In this article we are going to discuss about ViewData in ASP.Net Core MVC. To learn step by step from beginner to advance level about ASP.Net Core MVC then please follow this link.
In ASP.NET Core MVC application, we can pass the data from a controller action method to a view in many different ways such as by using ViewBag, ViewData, TempData and using strongly typed model. In this article, we will discuss how to use ViewData to pass the data from the controller action method to a view.
What is ViewData in ASP.NET Core MVC Application ?
The ViewData in MVC is a dictionary of weakly typed objects which is derived from the ViewDataDictionary class. As the ViewData is a dictionary object, so it will store the data in the form of key-value pairs where each key must be a string.
[ViewDataDictionary] public ViewDataDictionary ViewData { get; set; }
You can access the string data from the ViewData dictionary without casting the data to string type. But if you are accessing data other than the string type then you need to explicitly cast the data to the type you are expecting.
Access a string data using ViewData
To access the string data in View page we need to do following things.
- Declare the ViewData string in Controller.
- Then access the ViewData in .cshtml page like in the below image.

Access a model data using ViewData
To access model data in View page using ViewData we declare the ViewData in Controller and access it in View Page. Let’s declare a customer data in a controller and this customer details add in ViewData.
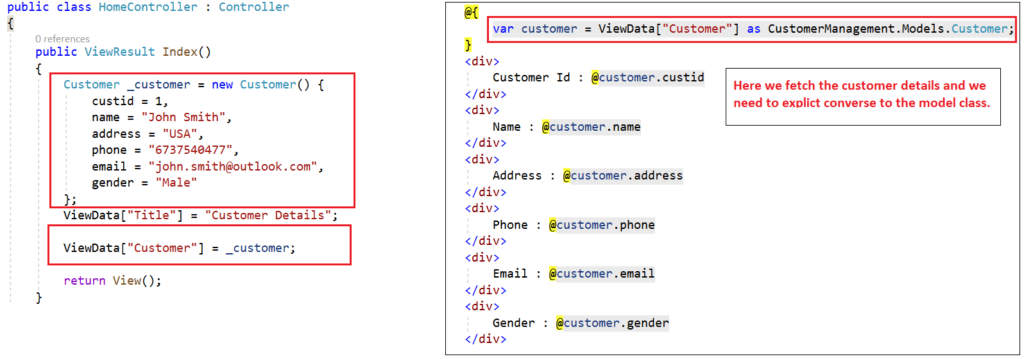
ViewData Example in ASP.NET Core MVC Application
Let’s see how to pass data from controller to view and see the code. Here we pass the data using ViewData of a string and model class.
public class HomeController : Controller { public ViewResult Index() { ViewData["Title"] = "Customer Details"; Customer _customer = new Customer() { custid = 1, name = "John Smith", address = "USA", phone = "6737540477", email = "john.smith@outlook.com", gender = "Male" }; ViewData["Customer"] = _customer; return View(); } }
To access the ViewData in view page(.cshtml) we need to access like below. To show the model data instead of div we use bootstrap table.
<html> <head> <meta name="viewport" content="width=device-width" /> <title>Customer Management</title> <script src="~/lib/jquery/jquery.js"></script> <link href="~/lib/twitter-bootstrap/css/bootstrap.css" rel="stylesheet" /> </head> <body> @{ var customer = ViewData["Customer"] as CustomerManagement.Models.Customer; } <div class="container"> <h2>Page Title is : @ViewData["Title"] </h2> <table class="table table-dark"> <tr> <td> Customer Id </td> <td> @customer.custid </td> </tr> <tr> <td> Name </td> <td> @customer.name </td> </tr> <tr> <td> Address </td> <td> @customer.address </td> </tr> <tr> <td> Phone </td> <td> @customer.phone </td> </tr> <tr> <td> Email </td> <td> @customer.email </td> </tr> <tr> <td> Gender </td> <td> @customer.gender </td> </tr> </table> </div> </body> </html>
Let’s run the application and see the output like below.
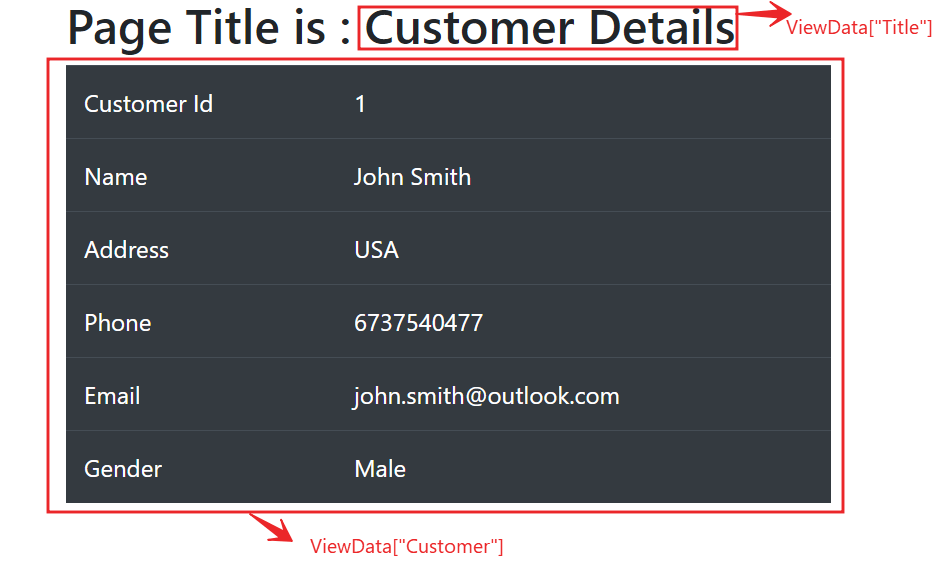
The ViewData is dynamically resolved at runtime, as a result, it does not provide compiles time error checking as well as we do not get any intelligence. For example, if we miss-spell the key names then we wouldn’t get any compile-time error. We get to know about the error only at runtime
The ViewData only transfers the data from the controller action method to a view, but not vice-versa. That means it is valid only during the current request.
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.