In this article we are going to discuss, Developer Exception page in ASP .NET Core. Please go through our previous article How to rename wwwroot folder name in ASP.NET Core. The UseDeveloperExceptionPage extension method adds middleware into the request pipeline which displays developer friendly exception detail page.
The exception handling is one of the key features of any application. As a developer you should check the exception at development level in deeply and when the application is ready to serve on staging or production server it should be error free. So in development stage the application is need to trace the development errors, in this post we will cover how to trace these development errors in ASP .NET Core application using Developer exception page.
UseDeveloperExceptionPage Middleware
- UseDeveloperExceptionPage middleware must call before any middleware that you want to catch exceptions.
- Enable the Developer Exception Page only when the app is running in the Development environment. You don’t want to share detailed exception information publicly when the app runs in production.
- You don’t want to share detailed exception information publicly when the app runs in production
The page includes the following information about the exception and the request:
- Stack trace
- Query string parameters (if any)
- Cookies (if any)
- Headers
Developer Exception code should be add on Startup.cs class of configure() method like below;

How to use Developer Exception Page Middleware in ASP.NET Core Application
Let’s consider the following code in Configure() method in Startup class.
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseFileServer(); app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapGet("/", async context => { await context.Response.WriteAsync("Hello World!"); }); }); }
When we run the application you can see instead of response “Hello World” it open default page as we set the default page as “Index.html”, this is because due to UseFileServer() middleware. UseFileServer() middleware combines the functionality of UseDefaultFiles and UseStaticFiles middleware. In our previous videos in this series we have included a default html document with name index.html in wwwroot folder.
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } // app.UseFileServer(); app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapGet("/", async context => { throw new Exception("Error Occurred while processing your request"); await context.Response.WriteAsync("Hello World!"); }); }); }
Here you can see, we block the app.UseFileServer(); and the custom exception in response message in MapGet() method. As the app.UseFileServer() middleware is blocked it directly point to MapGet() method.
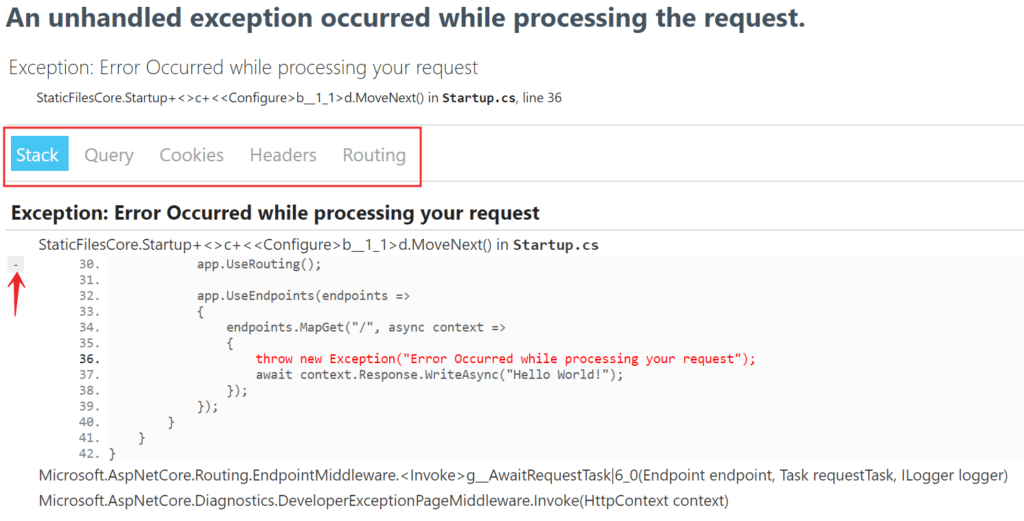
If you have any experience with previous version of .NET (ASP.Net, ASP .NET MVC), then you must be very familiar with this page. This is similar to that yellow screen of death in classic asp.net.
As the name implies, this Developer Exception Page contains exception details like
- Stack trace including the file name and line number that caused the exception
- Query String, Cookies and HTTP headers
You can navigate to other tabs like Query String, Cookies, Headers and Routing etc.
How to Customize the UseDeveloperExceptionPage Middleware in ASP.NET Core?
Like other middleware components we also make to customize the UseDeveloperExceptionPage() middleware, Whenever you want to customize a middleware component, always remember you may have the respective OPTIONS object.
Middleware | Options |
UseDeveloperExceptionPage | DeveloperExceptionPageOptions |
UseDefaultFiles | DefaultFilesOptions |
UseStaticFiles | StaticFileOptions |
UseFileServer | FileServerOptions |
Middleware with Options
So, to customize UseDeveloperExceptionPage middleware, use DeveloperExceptionPageOptions object as shown below.
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { DeveloperExceptionPageOptions developerExceptionPageOptions = new DeveloperExceptionPageOptions { SourceCodeLineCount = 5 }; app.UseDeveloperExceptionPage(developerExceptionPageOptions); } app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapGet("/", async context => { throw new Exception("Error Occurred while processing your request"); await context.Response.WriteAsync("Hello World!"); }); }); }
SourceCodeLineCount
property specifies how many lines of code to include before and after the line of code that caused the exception.
How UseDeveloperExceptionPage Middleware works
We need to configure the UseDeveloperExceptionPage() Middleware as early as possible in the application’s request processing pipeline so that it can handle the unhandled exception and then display the Developer Exception Page with the detailed information about the exception.
Let us see what happened when we configure the UseDeveloperExceptionPage() middleware after the middleware using is Registered using the MapGet() method. Please modify the Configure() method as shown below.
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapGet("/", async context => { throw new Exception("Error Occurred while processing your request"); await context.Response.WriteAsync("Hello World!"); }); }); if (env.IsDevelopment()) { DeveloperExceptionPageOptions developerExceptionPageOptions = new DeveloperExceptionPageOptions { SourceCodeLineCount = 10 }; app.UseDeveloperExceptionPage(developerExceptionPageOptions); } // app.UseFileServer(); }
When we run the application, it will not display the developer’s exception page instead of simply returns the default error status code. This is the reason why we need to configure the UseDeveloperExceptionPage()
middleware as early as possible to handle the unhandled exception of the application in the request processing pipeline.
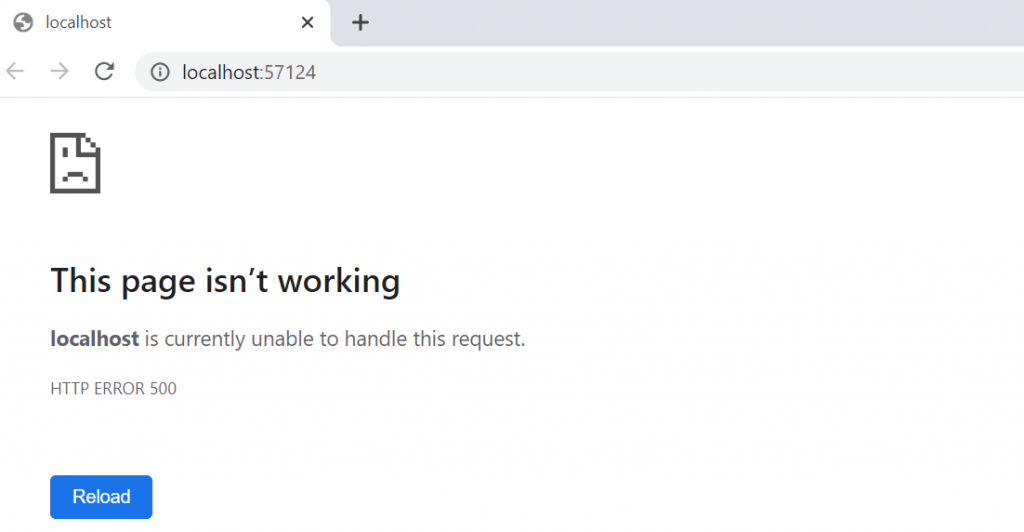
You can go through the below video to see more,
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.