In this article we are going to discuss about Controller in ASP.NET Core MVC. Before going to this please read our previous article Views in ASP.NET Core MVC. To learn step by step process of ASP .NET Core MVC from beginners level to advanced level then please follow the ASP.NET Core Tutorial.
Controllers in ASP.NET Core MVC Application ?
The Controllers in ASP.NET Core MVC application are the classes having a set of public methods and the public methods are called as actions (action methods). These action methods are the methods of a Controller which is actually going to handle the incoming HTTP Requests.
The Controllers in MVC application logically group similar types of actions together. It allows us to define sets of rules such as caching, routing, and authorization which is going to be applied collectively.
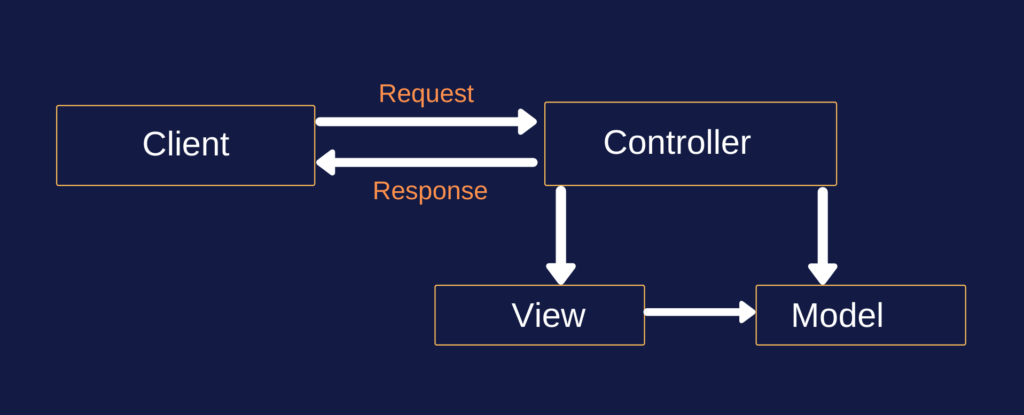
- The controller classes are available in Controllers folder in the root level project and the controllers are inherits from Microsoft.AspNetCore.Mvc.
- The controller class name is suffixed with word “Controller” as like HomeController, EmployeeController etc. and the class is decorated with the [Controller] attribute.
- When a request come from the browser, it is reach the controller in the MVC design pattern, that handles the incoming HTTP request and responds to the user action.
Understating Controller in Details
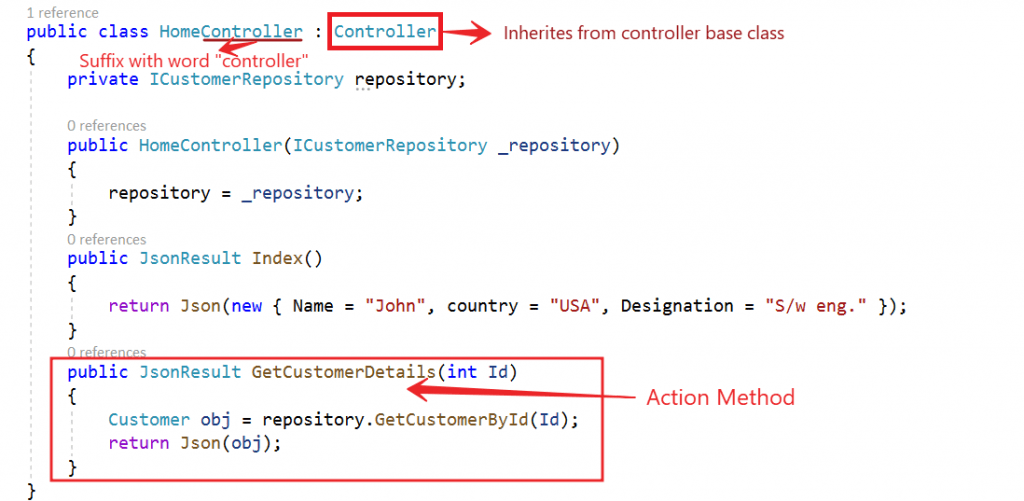
Let’s say the user has typed the following URL in the browser address bar and hit the ENTER key http://localhost:43265/Home/GetCustomerDetails/1
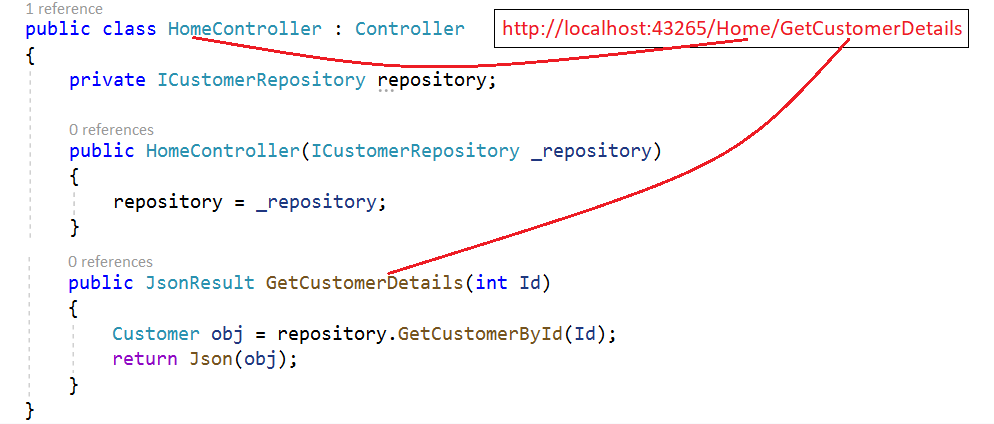
- When the URL /Home/GetCustomerDetails is mapped to the “GetCustomerDetails” public action method in the HomeController. This mapping is done by the routing rules defined in our application.
- int Id is the parameter this need to pass to filter out the customer details.
- In ASP.NET Core MVC the action methods of a controller can return different types of data such as JSON, View, String, Object, XML, etc.
- The request arrives at a controller action method. As part of processing that request, the controller creates the Model. To retrieve model data, the controller depends on a service.
- For example, in our case to retrieve Customer data, HomeController depends on ICustomerReporsitory service.
- ICustomerReporsitory service is injected into the HomeController using the constructor. This is called Dependency Injection.
- We are assigning the injected dependency to a read-only field. This is a good practice as it prevents accidentally assigning another value to it inside a method.
- Once the controller has the required model data, it can simply return that model data if we are building a RESTful service or an API.
Controller Action Method Returning JSON Data
The GetCustomerDetails action method always going to returns the data in JSON format irrespective of the content negotiation. This is because of the return type of the GetStudentDetails() method which is set to JsonResult. In this case, it is going to ignore the Accept Header values.
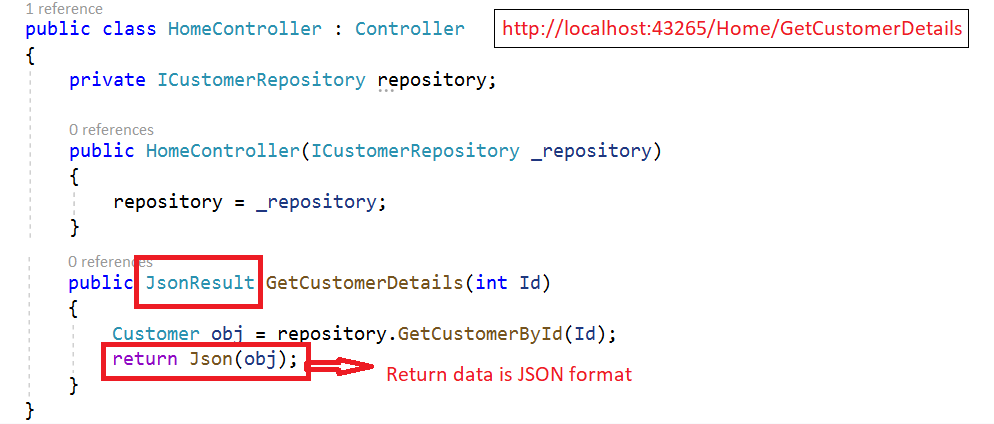
Controller Action Method returning ObjectResult
In the below example, it looks for the Accept Header value and if the value is set to application/xml, then it returns the data in XML format whereas if the value is set to application/json, then the data is going to return in JSON format.
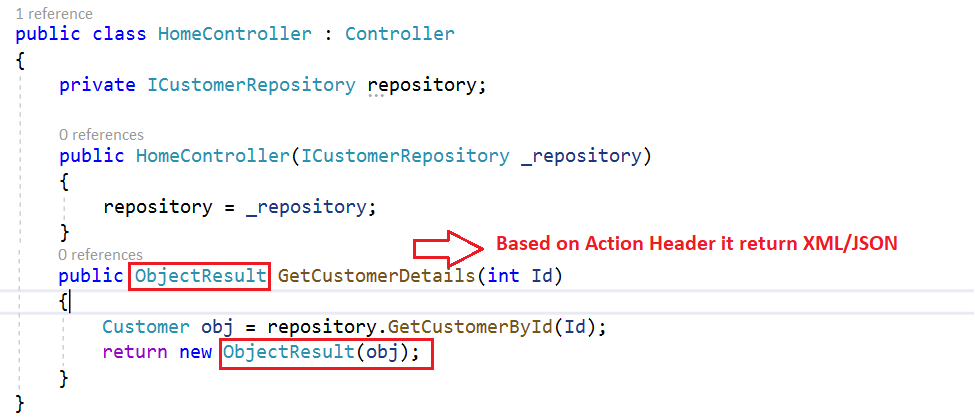
public void ConfigureServices(IServiceCollection services) { services.AddControllersWithViews().AddXmlSerializerFormatters(); services.AddTransient<ICustomerRepository, CustomerRepository>(); }
Controller Action Method returning View
In order to return a view from an action method in ASP.NET Core MVC, you need to use ViewResult as the return type of the action method. According to our example, the GetCustomerDetails action method is going to return a View as the return of the method is set to ViewResult
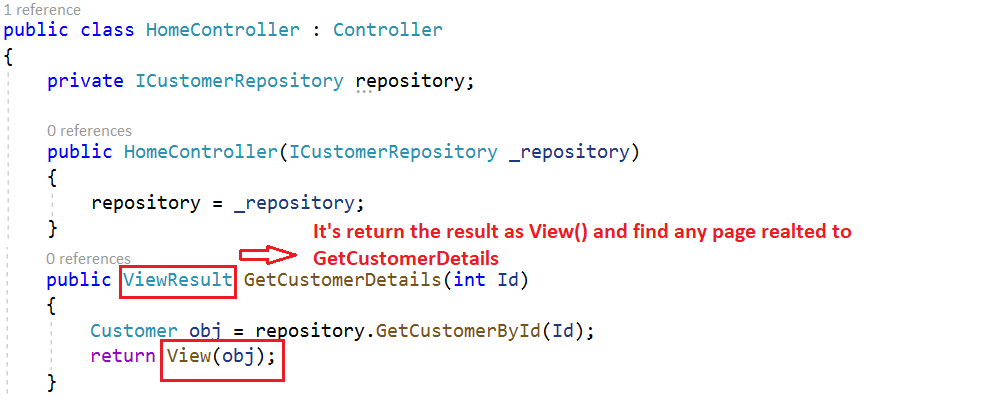
But when you run the application and navigate to “http://localhost:43265/Home/GetCustomerDetails“ URL, then you will get the following error.
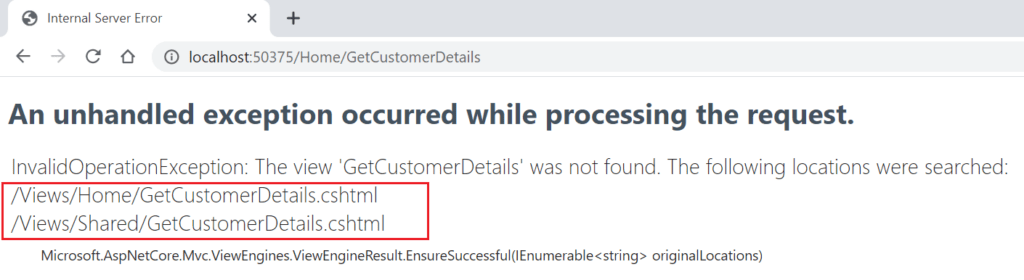
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.