In this article we are going to discuss Views in ASP.NET Core MVC. Before starting this article please go through the previous article Model in ASP.NET Core MVC. To learn step by step about ASP .NET Core MVC from beginner to advanced level please follow the ASP.NET Core MVC Tutorials.
What are Views in ASP.NET Core MVC Application ?
- In the Model-View-Controller (MVC) pattern, the View is the component that contains logic to represent the model data (the model data provided to it by a controller) as a user interface with which the end-user can interact.
- View is a HTML template with embedded razor markup.
- A view file has .cshtml extension if the programming language is C#.
Where are View files stored in ASP.NET Core MVC ?
- The View files are stored in Views folder by default.
- View files that belong to a specific controller are stored in a sub-folder in the Views folder and that sub-folder has the same name as the controller.
- The view file has the same name as that of the controller action method with a .cshtml extension.
Let’s say, we have an ASP.NET Core MVC application with two controllers i.e. HomeController and CustomerController. The HomeController is created with the following three action methods.
- Index()
- AboutUs()
- ContactUs()
On the other hand, the CustomerController is created with the following four action methods.
- Create()
- Edit()
- Update()
- Delete()
Views Folder Structure
The views folder are structured like in the below diagram. According to Controller it creates the folder inside Views and according to action method it create Views.cshtml.
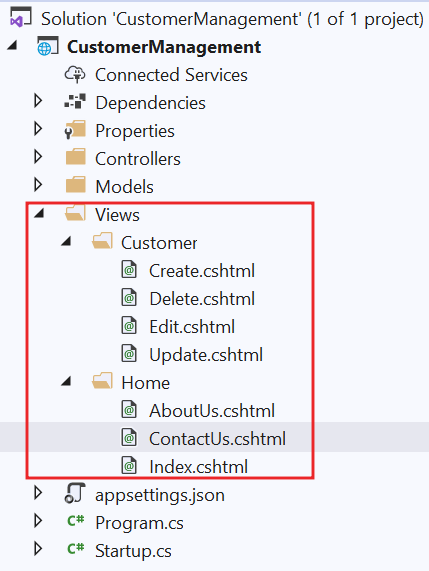
- For each Controller we have a separate folder in the “Views” folder.
- All the HomeController views are in Home folder in the “Views” folder.
- All the CustomerController views are in Customer folder in the “Views” folder.
- Each view file has the same name as the controller action method.
In addition to action-specific views, we also have provided with partial views, layouts, and view components that can also be used to reduce the repetition and allow for reuse within the application’s views. We will discuss all these things in our upcoming articles.
Understanding Views with an Example
To understand Views properly let’s modify in HomeController and a dd a new action method like below;
public class HomeController : Controller { public ViewResult Details() { return View(); } }
In the above HomeController, we have the action method i.e. Details. As the return type of the Details() action method is ViewResult, so this action method is going to return a view. In order to return a view, here we are using the View() extension method which is provided by Microsoft.AspNetCore.Mvc.Controller Base class.
Let’s run the application by navigating the URL “http://localhost:50375/Home/Details“. You can see the issue found due to their is no views folder inside views.
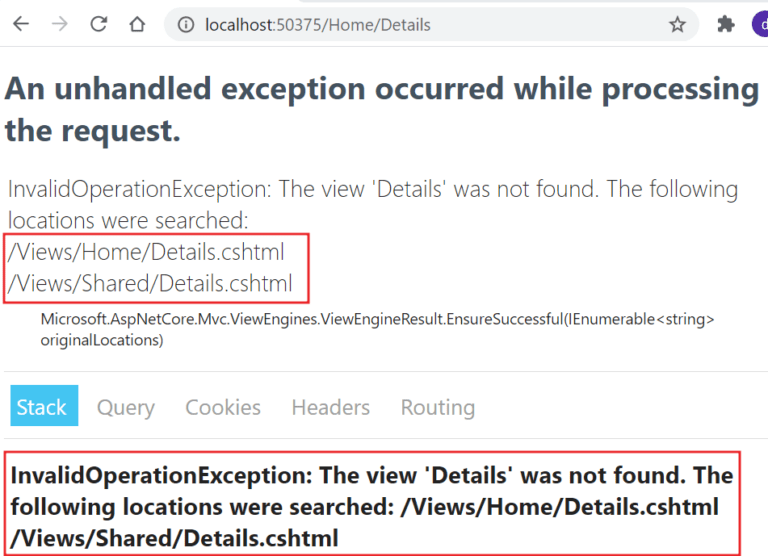
Let us understand why we got the above error
As we are returning a view from the Details action method of Home Controller, by default the ASP.NET Core MVC Framework will look for a file with the name Details.cshtml in the following three locations.
The .cshtml is the combination of csharp+html.
- First it search in “/Views/Home/” folder because the Controller name is HomeController
- Then it search in “/Views/Shared/” folder
How to create Views in ASP.NET Core MVC Application
You can create views in two ways
- Manually create Views
- By creating view from controller action method
Manually Create Views
- First we need to create a folder name as “Views” in the root level project.
- Under the Views folder we need to create folder name as “Home” as we have the controller name as HomeController.
- Then according to the action method name we should create the cshtml page, in our case the action name is Details so we need to create the view page as “Details.cshtml“
Right Click on Home folder then add View, select the Razor view like below;
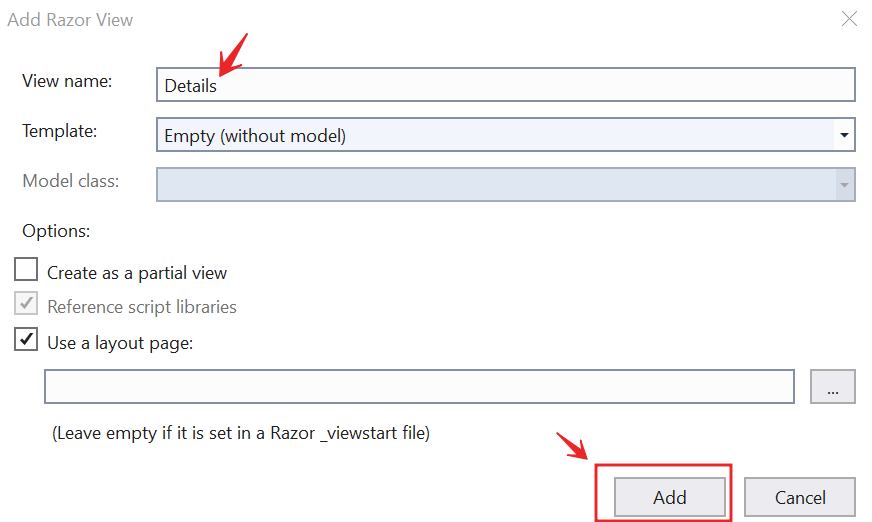
After adding the view page run the application and you will see the view is rendered like below; On the left we have the html content and on right side the view content is there.

By creating view from controller action method
You can also create the view page from controller action method using below steps.
- Right click on the action method
- Select Add View option like below
- Then a popup window open the select razor page/razor page with empty according to your choice.
- Finally, give the page name then add the page
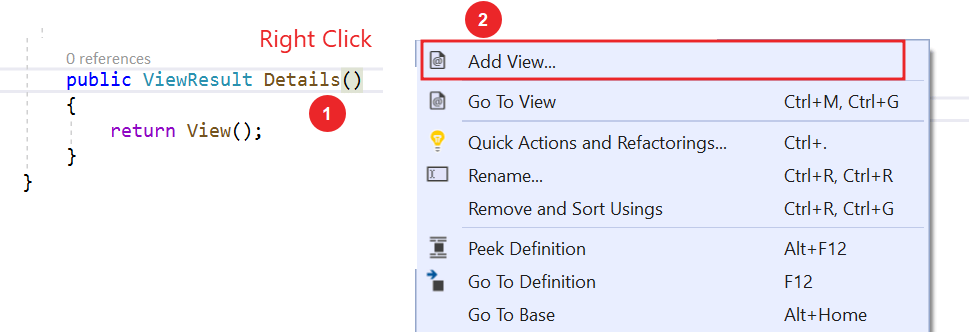
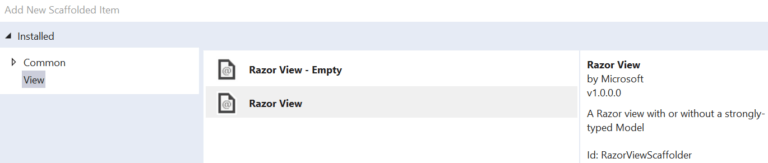
Difference between View() and View(object model) Extension Methods
When you go to definition of controller base class you can find their View and View with Object model.
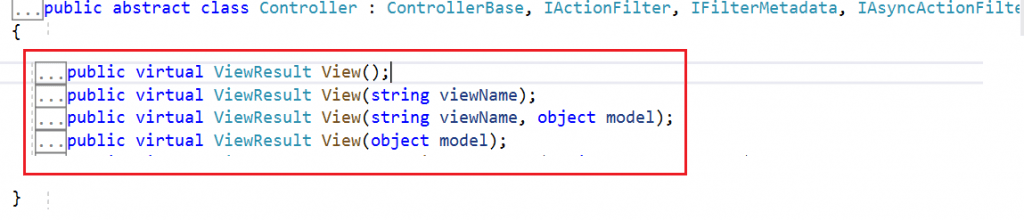
The difference is you can return single View() or you can return View(object model) or View(“SamplePage”) like this. On our upcoming article we go through the details.
How to specify the Absolute view file path ?
You can also specify absolute path of a view page like below;
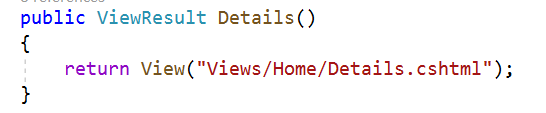
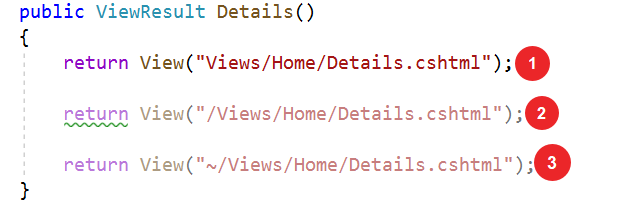
Advantages of Using Views in ASP.NET Core MVC
The Views in MVC application provides the separation of concerns, It separates the user interface from the business logic or you can say from the rest of the application. The ASP.NET Core MVC views use the Razor syntax which makes it easy to switch between the HTML markup and C# code. The Common or repetitive aspects of the application’s user interface can be easily reused between views using layout and shared directives or partial views.
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂