In this article we are going to discuss Difference between AddMVC() and AddMvcCore(). Please read my previous article IHostingEnvironment and IWebHostEnvironment in ASP.Net Core.
Along with the AddMvc() method, we can also use the AddMvcCore() method to Set up the MVC in the ASP.NET Core application. So, in short, we can say that the IServiceCollection interface provides two methods such as AddMvc() and AddMvcCore() to set up MVC in an ASP.NET Core application.
Before we discuss the difference between AddMvc() and AddMvcCore() methods, let’s modify the following HomeController to return JSON formatted data, instead of a simple string.
At the moment, there is no base class specified for the the HomeController class. It works for us now because we are returning a simple string from the Index() action method like below;
public class HomeController : Controller { public string Index() { return "Hello from MVC | Coreprogram"; } }
But this is not going to be worked if we want to return an HTML View or JSON Data from the Index action method. If you want to return the JSON Data or HTML View from an action method then the Controller class should inherit from the base Controller class which is provided by the ASP.NET Core framework.
The base Controller class which is belongs to Microsoft.AspNetCore.Mvc namespace provides the supports to return different types of results such as JSONResult, ViewResult, etc.
public class HomeController : Controller { public JsonResult Index() { return Json(new { Name = "John Smith", Country = "USA", Designation = "Software Engineer" }); } }
And as like below image we added services.AddMvc(); in the ConfigureServices() method.
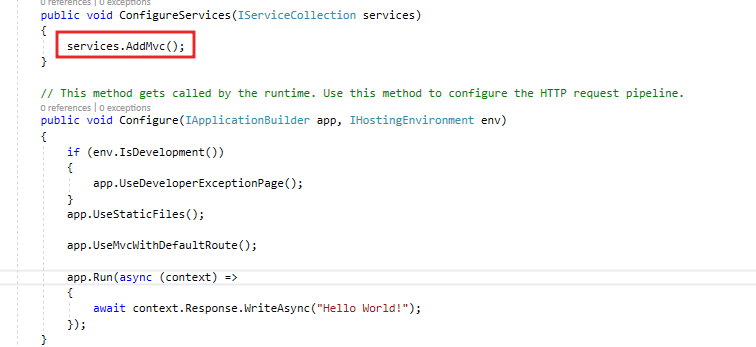
When we run the application you can see we got the Json result as expected.
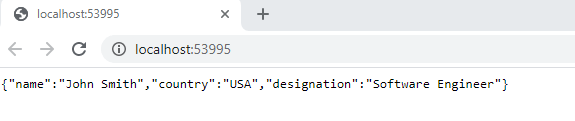
Using AddMvcCore() method
Now let us modify the ConfigureServices() method of the Startup class as shown below to use the AddMvcCore() method to register the MVC services to the dependency injection container.
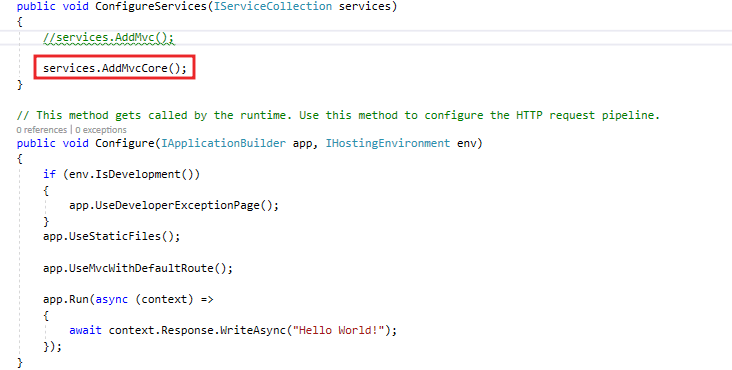
With the above changes in the ConfigureServices()
method, when you run the application you can find the below error.
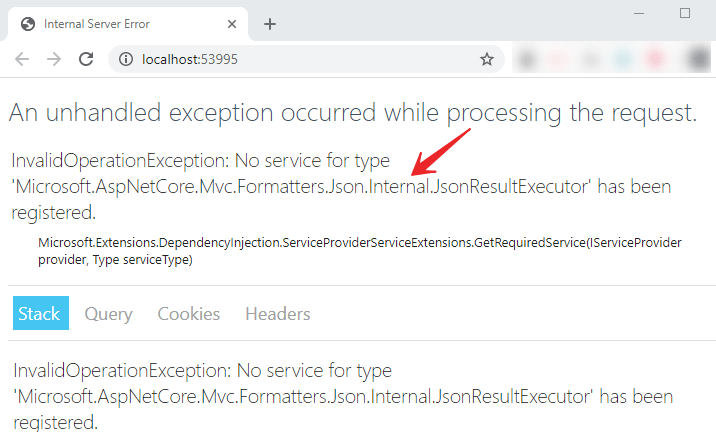
Let’s understand why the above issue is came when we use AddMvcCore()
The reason is that AddMvc() method registered the Json Formatters within the dependency injection container but not the AddMvcCore() method. So that when we run the application it shows the straight forward error.
As asp.net core is open source, so you can find the source code of the AddMvc() method by navigating to the following GitHub link.
So let’s modify the ConfigureServices() method of the Startup class as shown below to register the JsonFormatters with AddMvcCore() method to return JSON data.
public void ConfigureServices(IServiceCollection services) { var builder = services.AddMvcCore(); builder.AddJsonFormatters(); } // This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IHostingEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseStaticFiles(); app.UseMvcWithDefaultRoute(); app.Run(async (context) => { await context.Response.WriteAsync("Hello World!"); }); }
Now, when we run the application we can see the Json result as expected.
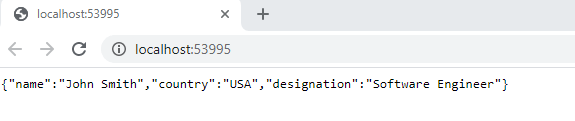
What we learn so far
AddMvcCore() method adds only the required MVC services to the dependency injection container whereas the AddMvc() method adds all the required MVC services.
- As the name implies, AddMvcCore() method only adds the core MVC services.
- On the other hand, AddMvc() method adds all the required MVC services. AddMvc() method calls AddMvcCore() method internally, to add all the core MVC services.
- So if we are calling AddMvc() method there is no need to explicitly call AddMvcCore() method again.
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.