In this article we are going to discuss the difference between IHostingEnvironment
and IWebHostEnvironment
in ASP.Net Core. Before starting this article please go through the previous article How to Install .NET Core.
If you have work on previous version of .NET then we knew that to access the server path we used Server.MapPath
there but in .NET Core application Server.MapPath
is completely removed and introduce new interfaces IHostingEnvironment
for .Net Core 2.0 and IWebHostEnvironment
for .Net Core 3.0 and onward.
Difference between IHostingEnvironment and IWebHostEnvironment
As far we know both of these interfaces are use in .NET Core application to get the application server path and content root path. In earlier version of .NET Core application ( up to 2.0) IHostingEnvironment is used but after released of .NET Core 3.0 onward IWebHostEnvironment is used.
Both of these interfaces need to be injected as dependency in the Controller and then later used throughout the Controller. Both these interfaces have two properties.
- WebRootPath – Path of the www folder ( using this path you can access all static files)
- ContentRootPath – Path of the root folder which contains all the Application files. ( using this you can get all the application file in root folder)
Before using IHostingEnvironment and IWebHostEnvironment you need to import the below namespace.
using Microsoft.AspNetCore.Hosting;
IHostingEnvironment
The IHostingEnvironment is injected in the Controller and assigned to the private property Environment and then used to get the WebRootPath and ContentRootPath as on below;
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
private IHostingEnvironment _environment;
public HomeController(ILogger<HomeController> logger, IHostingEnvironment environment)
{
_logger = logger;
_environment = environment;
}
public IActionResult Index()
{
string wwwPath = this._environment.WebRootPath;
string contentPath = this._environment.ContentRootPath;
return View();
}
}
Here the .NET Core application is targeting to .NET Core 3.1 that’s why the IHostingEnvironment showing warning like below image.
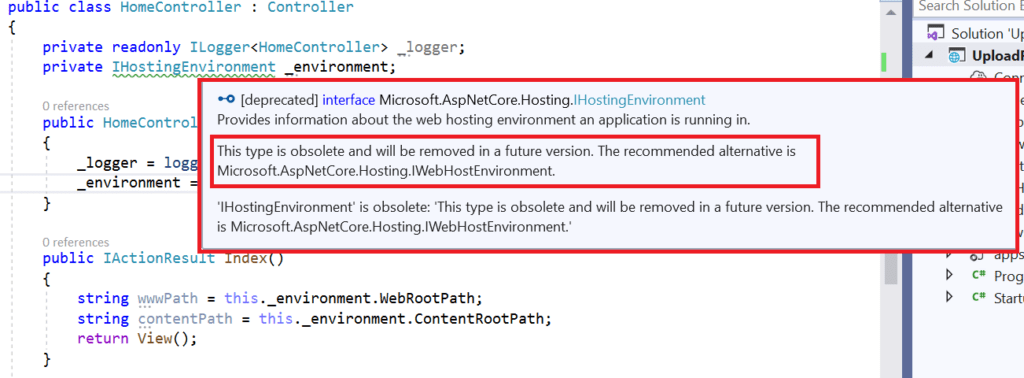
You can see that here showing as IHostingEnvironment is an obsolete means outdated due to the application environment is in .NET Core 3.1.
When you run the application you can see the result as below;
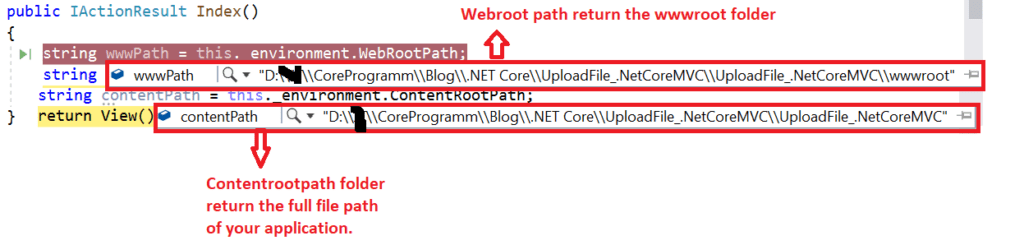
IWebHostEnvironment
IWebHostEnvironment is use on version 3.0 or above to fetch the server path or content root path in .NET Core. The IWebHostEnvironment is injected in the Controller and assigned to the private property Environment and then used to get the WebRootPath and ContentRootPath as on below;
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
private IWebHostEnvironment _environment;
public HomeController(ILogger<HomeController> logger, IWebHostEnvironment environment)
{
_logger = logger;
_environment = environment;
}
public IActionResult Index()
{
string wwwPath = this._environment.WebRootPath;
string contentPath = this._environment.ContentRootPath;
return View();
}
}
When we run the application you can see the same output as like in IHostingEnvironment but there is no warnings.
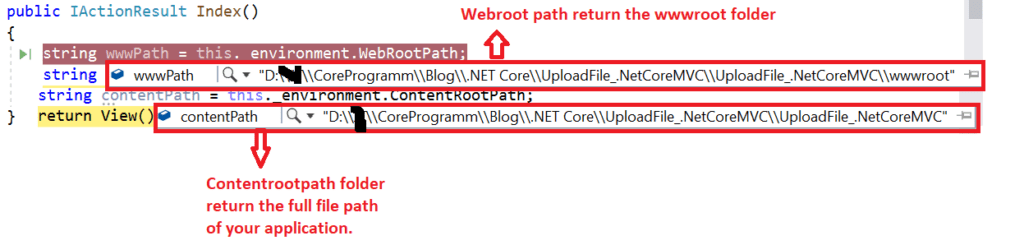
See the full Video that we illustrate the difference of IHostingEnvironment vs IWebHostEnvironment.
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.