In this article, we are going to discuss about Modules in Angular Application. Please follow our previous article Creating Angular Project using Visual Studio Code.
What is a Module in Angular?
In Angular, a module is a container. In order words, we can say that a module is a mechanism to group components, services, directives, pipes. So, the grouping of related components, services, pipes, and directives is nothing but a module in angular.
Why we need Modules in Angular?
Modular development is one of the most important aspects of software development. Good software will always have self-contained modules. So, in Angular, we would like to create separate physical typescript or JavaScript files that will have self-contained code. Then definitely you would have some kind of reference mechanism by using which the modules can be referred from the places where you want to use these modules.
In TypeScript, we can do this by using the “import” and “export” keywords. That means the modules which need to be exposed should be declared using the “export” keyword while the modules which want to import the exported modules should have the import keyword.
Understanding modules in angular:
When we create a new angular project using the Angular CLI command, one module i.e. AppModule
(within the
file) is created by default within the app folder which you can find inside the src folder of your project as shown in the below image.app.module.ts
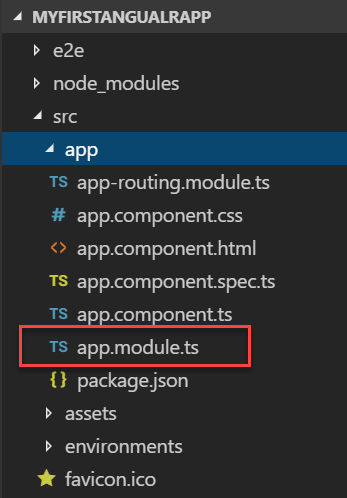
For any angular application, there should be at least one module. The AppModule (app.module.ts file) is the default module or root module of our angular application. Let us first understand this default module and then we will see how to create and use our own modules. Once you open the app.module.ts file, then you will find the following code in it.
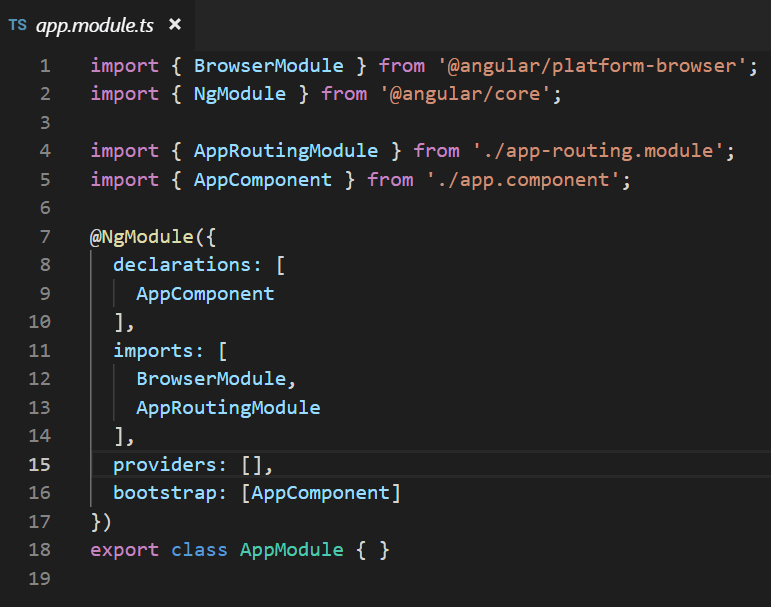
Here, AppModule is the module name and it is decorated with the @NgModule decorator. The @NgModule imported from the angular core library i.e. @angular/core. For better understanding please have a look at the following image. Here, we call NgModule a decorator because it is prefixed with @ symbol. So, whenever you find something in angular, that is prefixed with @ symbol, then you need to consider it as a decorator. In the next article, we will discuss the decorator in detail.
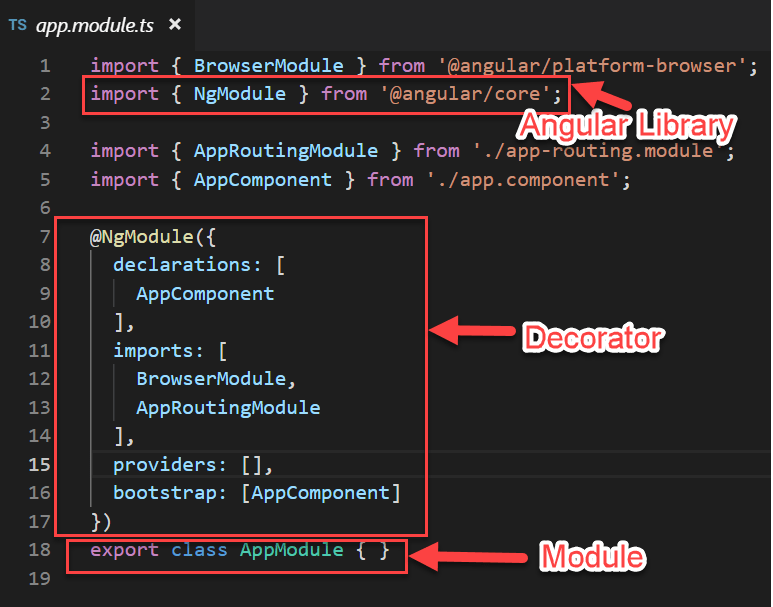
As you can see in the above image, the @NgModule decorator accepts one object and that object contains some properties in the form of arrays. By default, it has included 4 arrays (declarations, imports, providers, and bootstrap). Let us understand each of these properties of the @NgModule decorator.
Declarations Array (declarations):
The declarations array is an array of components, directives, and pipes. Whenever you add a new component, first the component needs to be imported and then a reference of that component must be included in the declarations array. By default AppComponent (app.component.ts file) is created when you create a new angular project and you can find this file within the app folder which you can find inside the src folder. If you open the app.component.ts file, then you will find the following code.
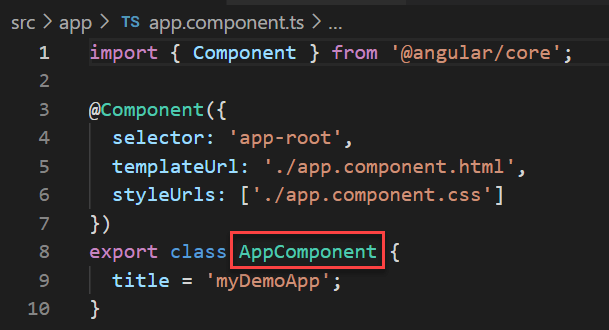
As you can see in the above image, the component name is AppComponent. And in the root module, first, this component (AppComponent) is imported and then its reference is included in the declarations array as shown in the below image.
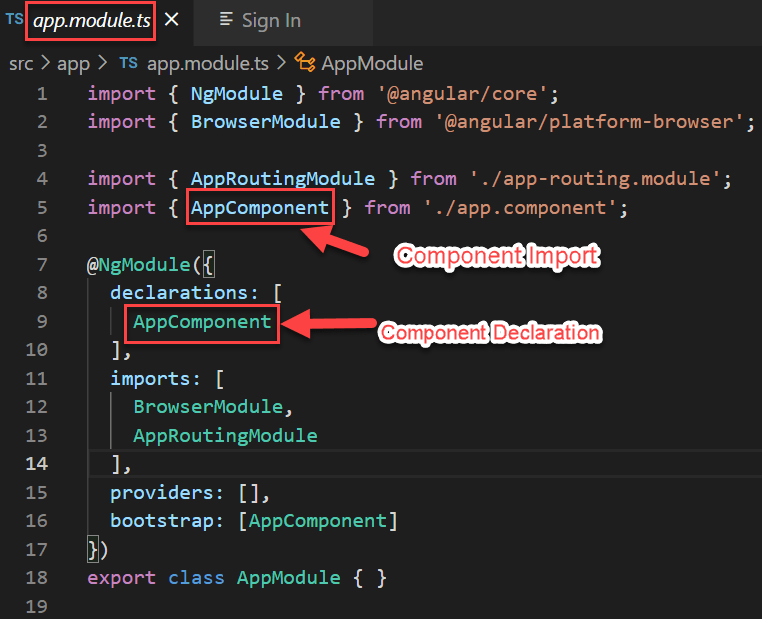
Imports Array (imports):
In the imports section, we need to include other modules (Except @NgModule). By default, it includes two modules i.e. BrowserModule and AppRoutingModule. Like the components, here also, first you need to import the modules, and then you need to include a reference of that module in the imports section as shown in the below image.
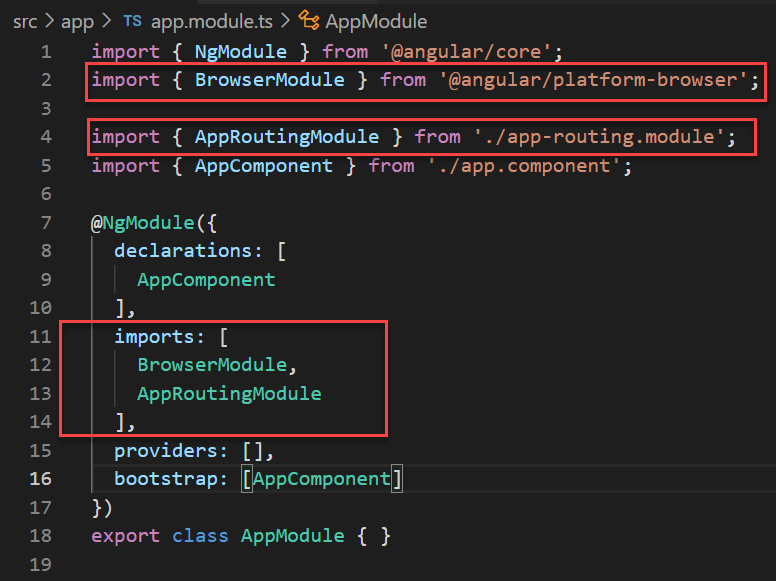
Bootstrap Array (bootstrap):
This section is basically used to bootstrap your angular application i.e. which component will execute first. At the moment we have only one component i.e. AppComponent and this is the component that is going to be executed when our application run. So, this AppComponent is included in the bootstrap array as shown in the below image.
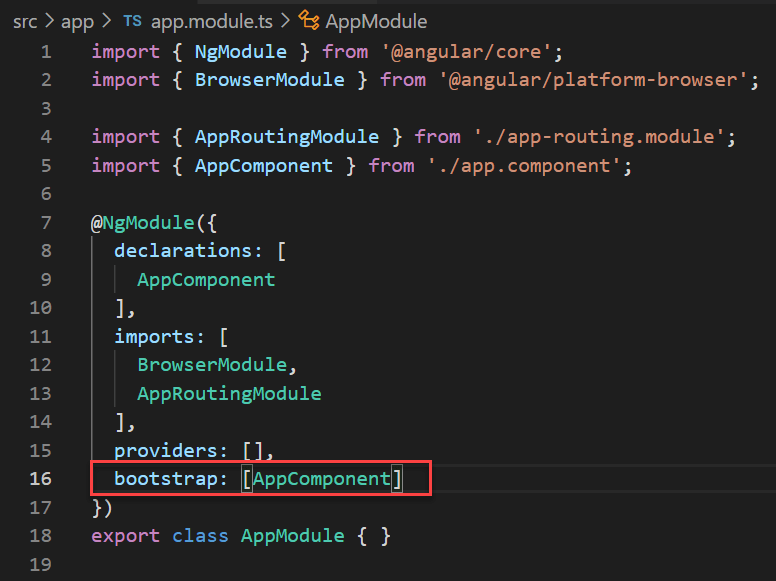
How to create a Module in Angular?
In order to create a module in angular using Angular CLI, you need to use the command as shown in the below image. Here g means generate and you use either g or generate to create the module.
ng generate module modulename ng g module modulename
Let say we want to create a module called Customer. So, first, open the terminal console in visual studio code and then type ng g module customer and press enter as shown in the below image.

You can see the customer module has been created with following module.ts
file. Like this, you can create multiple modules in a single angular application.
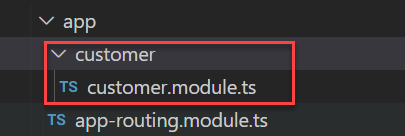
Creating Customer details Component within customer module folder
Here, we need to create the component within the customer folder. So, please type the following command and press enter. ng g c customer/customerdetails
When you execute the above command, it will create the customerdetails component within the customer folder as shown in the below image. In the same way, you can create multiple components in the same module.
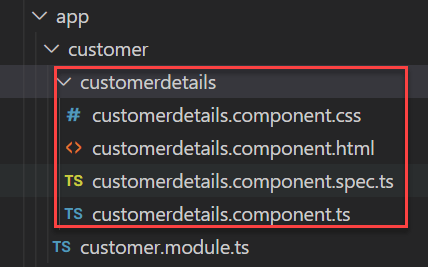
Adding CustomerDetails component reference in customer module
In the third step, you need to add the reference of CustomerDetails Component in the customer module. But, this work is automatically done by the angular framework for us. If you open the customer.module.ts file, then you will see that the import and declarations sections are automatically done as shown in the below image.
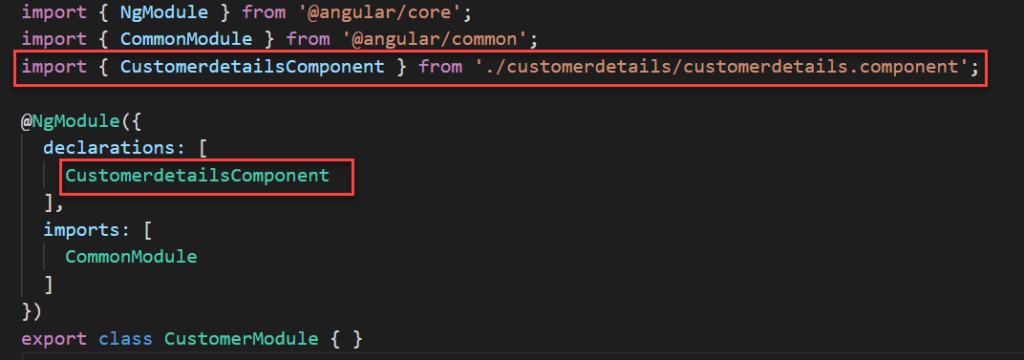
If you declare a component in one module, then you can’t declare the same component in another module. If you try then you will get an error when you run your application. At the moment you already declared the CustomerDetailsComponent within the customer module and if you try to declare it again on the AppModule module then you will get an error when you run your application.
Adding Employee module reference in AppModule import section
In order to use the CustomerDetailsComponent, you need to include the reference of the Customer module into the import section of your root module i.e. the AppModule as shown in the below image.
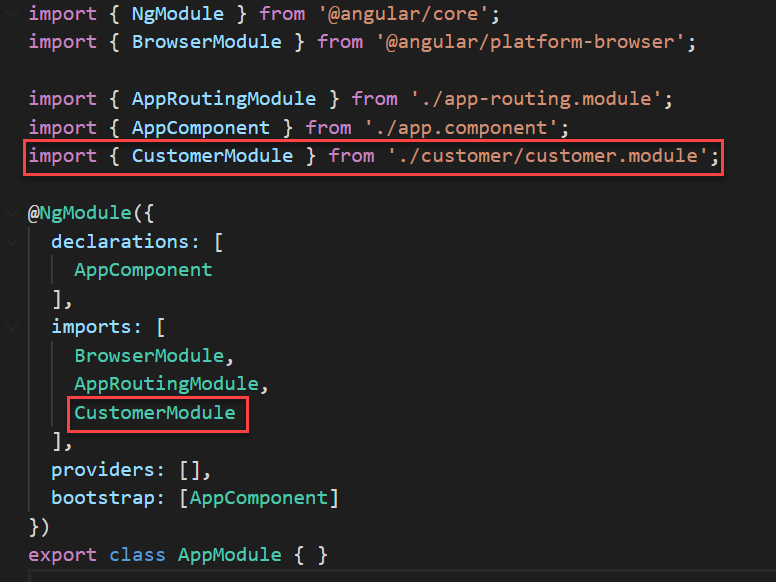
Conclusion
In the next article, we are going to discuss Angular Decorators in detail. Here, in this article, we discussed Modules in Angular Applications step by step with some examples.
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.