In this article we are going to discuss about Static files in ASP .NET Core. Before starting this article please go through our previous article How to set Connection String Globally in ASP .NET Core.
All the web applications have the feature to serve the static files from the file system, the static files are HTML, Images, CSS and Javascript. In ASP .NET Core these static files are not directly serve to the client browser, to enable the access of static files we need to do some configuration in ASP .NET Core application so that it serve the files directly. Let’s discuss the below points to achieve this.
Where the static files are store in ASP .NET Core Application ?
In ASP .NET Core the default directory to store the static files is “wwwroot” folder and this folder should be present on the project root folder. By default in “wwwroot” folder the static files are serve in .NET Core application, however if we want to change it then we can do it by using UseWebRoot method (we will discuss it in our next article).
How to add the static files in wwwroot folder ?
We are going to start it from scratch so create a .NET Core application choosing empty template and lets named as “StaticFiles”. After creating the application the template is look like below;
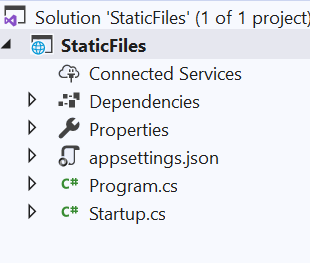
- Right click on the solution the add a new folder named as “wwwroot“.
- Under “wwwroot” folder let’s add a static image into it and access the image in browser.
- Copy the image into your physical location and paste inside “wwwroot” folder.
- The static image name in our case is “image1.png”
To be able to access the image file from the browser we need to follow the URL to be http://localhost:60070/image1.png. (The port number may be different on your machine).
When you run the application with the above URL you can see that there is no image is display but I still see the response produced by the middleware. This is due to we are registered using the Map() method but the image image1.png is not showing this is because at the moment when the application is request the processing pipeline does not have the required middleware that can serve static files.
UseStaticFiles()
Let’s add the UseStaticFiles()
middleware in Configure() method to serve the application’s request processing pipeline as shown below.
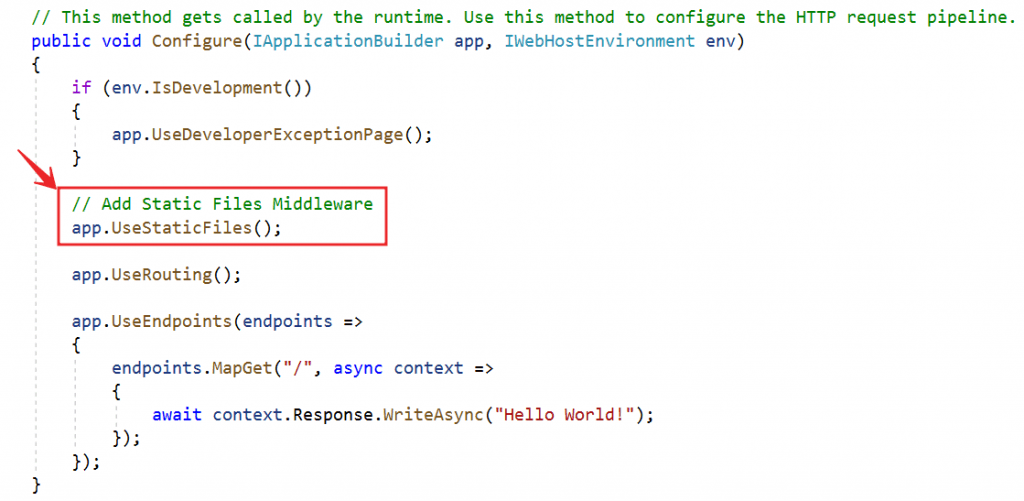
After adding this now the image is accessible to following the URL http://localhost:60070/image1.png.
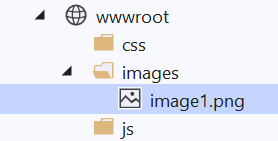
To able to access the image,m we should append the “images” folder tag as the image1.png are in images folder http://localhost:60070/image1.png.
How to navigate default document of ASP .NET Core application ?
Most of the web applications have a default document and is displayed when a user visits the root URL of your application. For example, you have a file with name default.html and you want to serve it when the user visits the root url of your application i.e http://localhost:60070/
When we run the application you can see the response produced by the middleware that we are using Map() method. We don’t see the content of the default document default.html. To be able to serve default page we have to plug in the UseDefaultFiles() middleware in our application’s request processing pipeline.
UseDefaultFiles is a URL rewriter that doesn’t actually serve the file. It simply rewrites the URL to the default document which will then be served by the Static Files Middleware. The URL displayed in the address bar still reflects the root URL and not the rewritten URL.
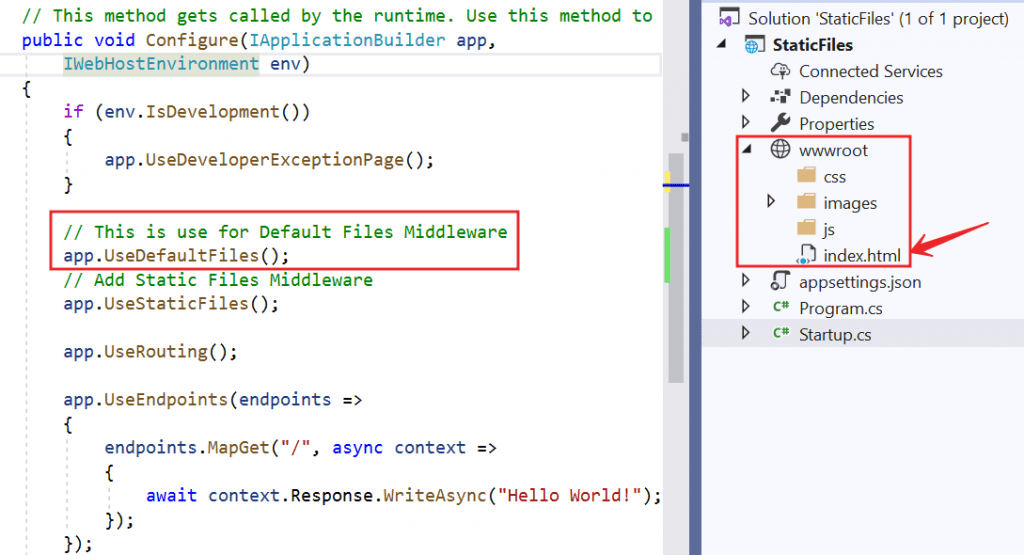
When we run the application you can see the “index.html” output as like below because we serve the UseDefaultFiles() middleware.
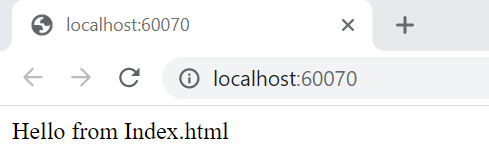
The following are the default files which UseDefaultFiles middleware looks for
index.htm
index.html
default.htm
default.html
If you want to set another document like custom.html as your default document, you can do so using the following code.
DefaultFilesOptions defaultFilesOptions = new DefaultFilesOptions(); defaultFilesOptions.DefaultFileNames.Clear(); defaultFilesOptions.DefaultFileNames.Add("custom.html"); // This is use for Default Files Middleware app.UseDefaultFiles(defaultFilesOptions);
Run the application now you can see the custom.html is the default page now.
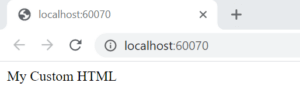
UseFileServer Middleware
UseFileServer combines the functionality of UseStaticFiles, UseDefaultFiles and UseDirectoryBrowser middleware. DirectoryBrowser middleware, enables directory browsing and allows users to see files within a specified directory. We could replace UseStaticFiles and UseDefaultFiles middlewares with UseFileServer Middleware. Let’s prove this.
FileServerOptions fileServerOptions = new FileServerOptions(); fileServerOptions.DefaultFilesOptions.DefaultFileNames.Clear(); fileServerOptions.DefaultFilesOptions.DefaultFileNames.Add("custom.html"); app.UseFileServer(fileServerOptions);
When we run the application, the custom.html is show as below;
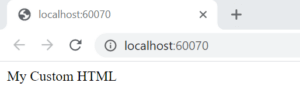
If you want to customise these middleware components, we use the respective OPTIONS object. For example notice the respective OPTIONS objects we use.
Middleware | Options Object |
UseDeveloperExceptionPage | DeveloperExceptionPageOptions |
UseDefaultFiles | DefaultFilesOptions |
UseStaticFiles | StaticFileOptions |
UseFileServer | FileServerOptions |
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.