In this article we will learn about Styling in Angular Components. Angular applications are styled with standard CSS. That means you can apply everything you know about CSS stylesheets, selectors, rules, and media queries directly to Angular applications. Please read my previous lesson of Angular Template VS TemplateUrl in Angular.
Additionally, Angular can bundle component styles with components, enabling a more modular design than regular stylesheets.
Like Template
(Template VS TemplateUrl) , in style we can also use Style in two ways.
- style
- styleUrls
How to use style in Angular Components
The styles must be specified in the component file using the @component
decorator’s style property. So, open the app.component.ts
file and use the following code into it. As you can see, we’re using the @Component
decorator’s styles property to specify the required styles.
- You can see the we use the inline styles of table, tr & td
- HTML code are static that could render in browser and when you run the application see the output.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styles: [`table, td, th {border: 1px solid black;}table {border-collapse: collapse;}
td {text-align: center;}`]
})
export class AppComponent {
title = 'myAngularApp';
}
<table>
<tr>
<td>Name</td>
<td>Jayant</td>
</tr>
<tr>
<td>Gender</td>
<td>Male</td>
</tr>
<tr>
<td>Qualification</td>
<td>MCA</td>
</tr>
<tr>
<td>Age</td>
<td>35</td>
</tr>
<tr>
<td>Country</td>
<td>India</td>
</tr>
</table>
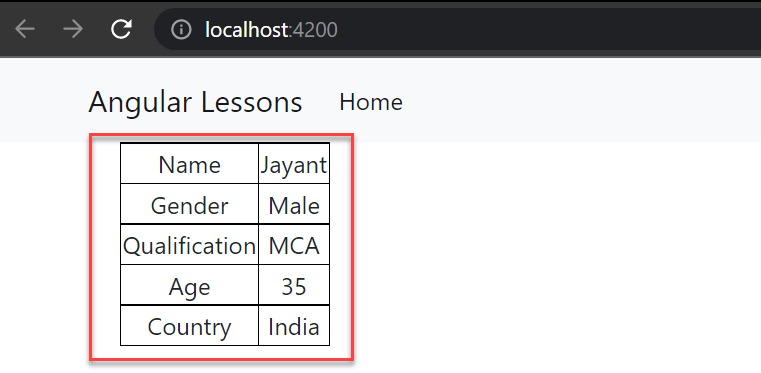
Advantages
- Application maintenance is simplified. The reason for this is that if you need to change or modify the styles, you only need to do so once.
- Styles specified using this approach are local to the component (i.e. the HTML File) and thus do not conflict with styles used elsewhere in the application.
Disadvantages:
- Code completion, Intelligence, and formatting are not available in the Visual Studio Code editor.
Styling using the @component decorator styleUrls
property
In this case, you should have an external stylesheet, and you should specify that external stylesheet using the @component
decorator’s styleUrls
property. The styleUrls
property in this case is actually an array of strings that contains the external stylesheet URLs. That is, you can specify multiple external stylesheets here.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'myAngularApp';
}
As you can see we define the external css files for styling so let’s add the css in app.component.css
table,
td,
th {
border: 1px solid black;
}
table {
border-collapse: collapse;
}
td {
text-align: center;
}
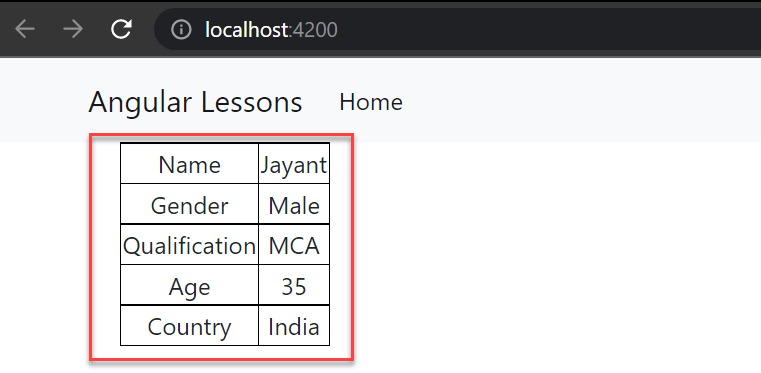
Advantages
- Because the stylesheet and its path are both included in the component, it is easy to reuse.
- Application upkeep becomes a breeze. The reason for this is that if you need to change the styles, you only need to do so once.
- Formatting, Intellisense, and Code completion are also included in the Visual Studio Code editor.
- Styles specified using this approach are component-specific, so they will not conflict with styles used elsewhere in the application.
Conclusion
In this article we will learnt about Styling Angular Components. Angular applications are styled with standard CSS. That means you can apply everything you know about CSS stylesheets, selectors, rules, and media queries directly to Angular applications.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.