In this article we will learn about Angular Interpolation. This is also known as string interpolation. As the data flow from the component to the view, there is only one way. Please read my previous article Data Binding in Angular.
What is Angular Interpolation?
We can use interpolation to include expressions as part of any string literal in our HTML. Angular converts the expressions into strings, replaces them in the original strings, and updates the view. Interpolation is available wherever a string literal is used in the view. String interpolation is another name for angular interpolation. Because you use expressions within another string.
The Interpolation in Angular allows you to place the component property name in the view template, enclosed in double curly braces i.e. {{propertyName}}
. So, the Angular Interpolation is a technique that allows the user to bind a value to a UI element.
Interpolation syntax
The Angular uses the {{ }}
(double curly braces) in the template to denote the interpolation. The syntax is as :
{{ templateExpression }}
Template Expression refers to the content between the double braces {{}}
.
The Template Expression is first evaluated and converted into a string by Angular. The result is then used to replace the Template expression in the original string in the HTML. Angular updates the original string whenever the template expression changes.
On the below image you can see, we used Angular Interpolation
- In the
app.component.ts
file we declared two values FirstName and LastName that we want to show in HTML. - In the
app.component.html
file we used the angular interpolation using braces{{}}
that render the crated object in Typescript file. - And finally the output like below that render the string that we declared.
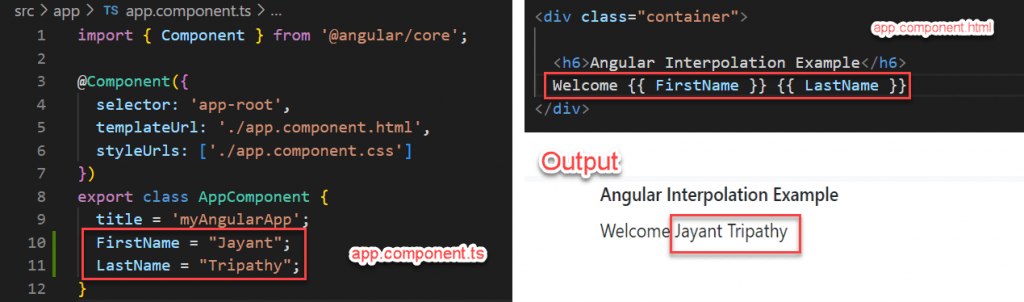
Angular Interpolation Examples
Angular interpolation with hardcoded string
In Angular, you can also concatenate a hardcoded string value with the property value. Let us add the hard-coded string First Name before the FirstName property and Last Name before the LastName property to better understand this. If this is your requirement, you could do it as shown in the image below.
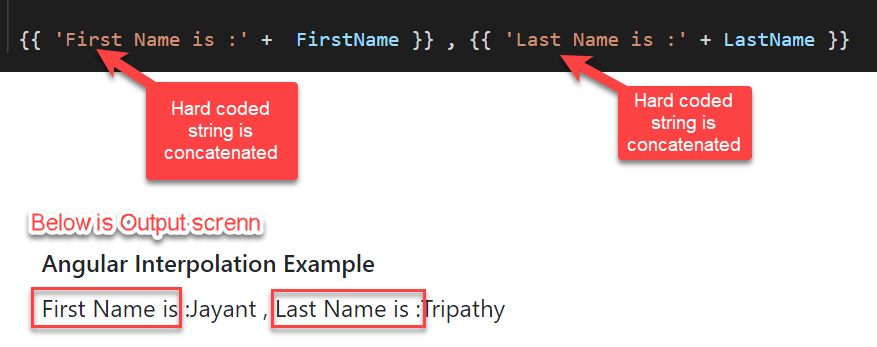
Angular Interpolation with Expression
When using Interpolation in Angular (i.e. double curly braces), you can also specify a valid expression. For example, if you want to perform mathematical calculations, you can do so using interpolation, as shown in the image below.
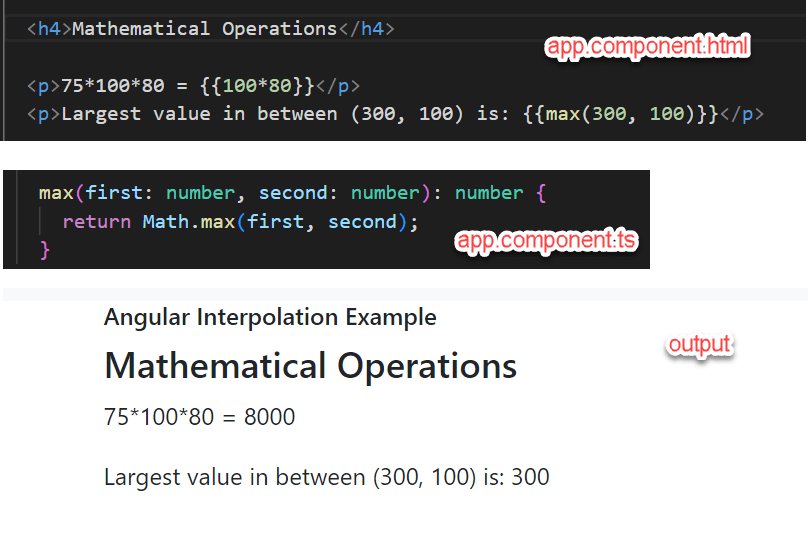
<h4>Mathematical Operations</h4>
<p>75*100*80 = {{100*80}}</p>
<p>Largest value in between (300, 100) is: {{max(300, 100)}}</p>
max(first: number, second: number): number {
return Math.max(first, second);
}
Interpolation in Angular with Ternary Operator
The expression that is enclosed in the double curly braces is commonly called Template Expression and the template expression can also be a ternary operator.
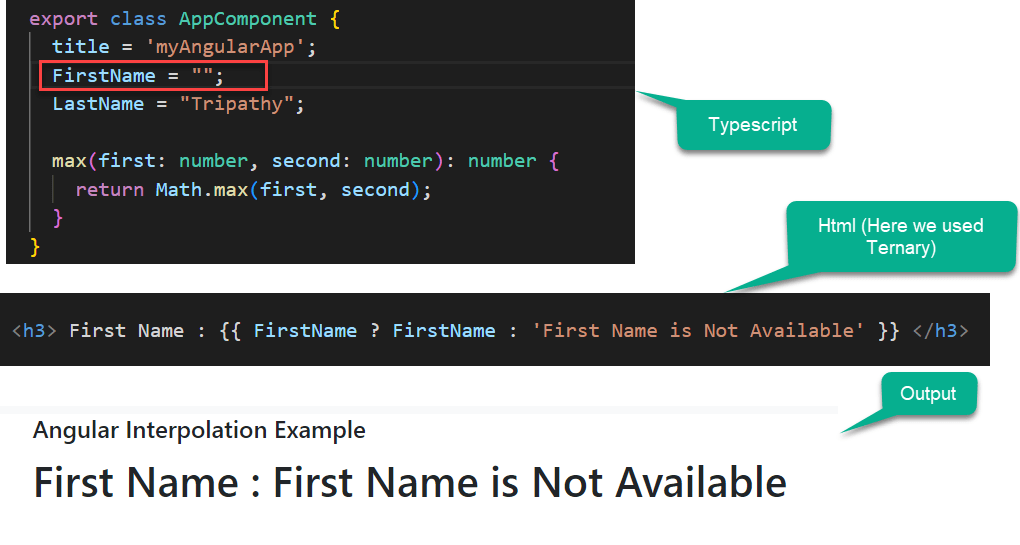
<h3> First Name : {{ FirstName ? FirstName : 'First Name is Not Available' }} </h3>
Method Interpolation in an Angular Application
First, let’s look at how to create a method with typescript, and then we’ll look at how to call a class method with interpolation. So, as shown in the image below, we will create one method called GetFullName()
that will return the full name by combining the first name and last name properties. This operator is used to access the class member in this case.
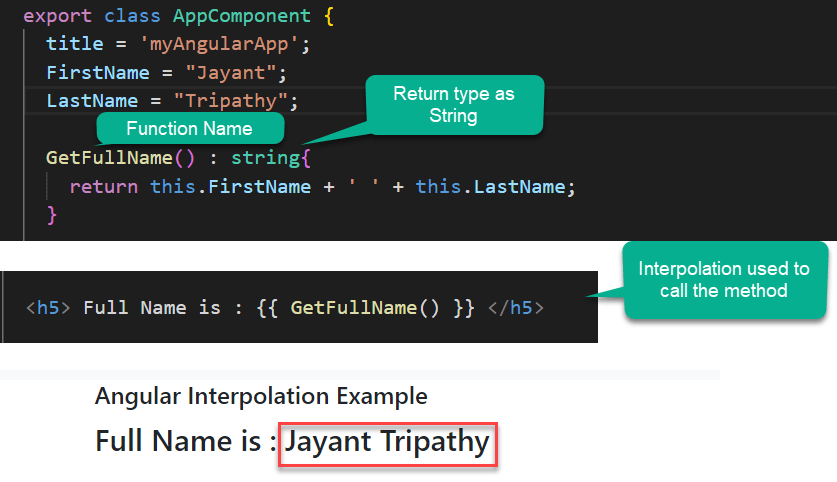
export class AppComponent {
title = 'myAngularApp';
FirstName = "Jayant";
LastName = "Tripathy";
GetFullName() : string{
return this.FirstName + ' ' + this.LastName;
}
}
Displaying Images using Angular Interpolation
It is also possible in angular to display images using Interpolation. Let us say we want to display the image from the link. https://jayanttripathy.com/wp-content/uploads/2022/04/cropped-JT.png
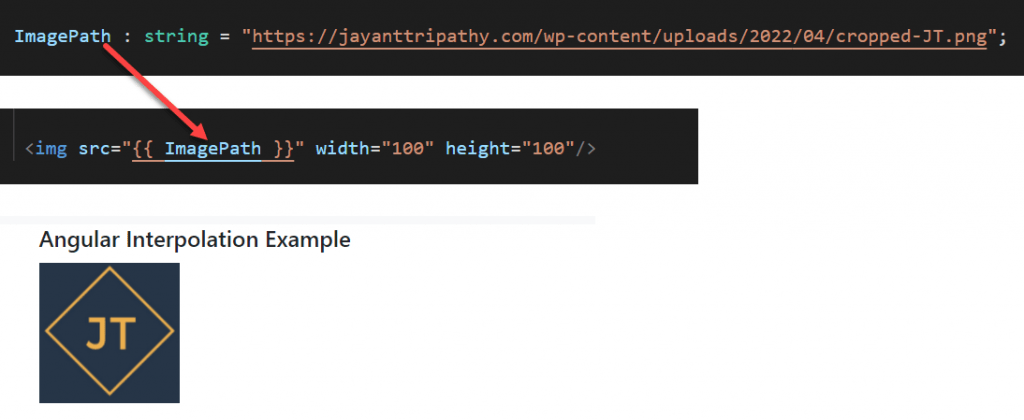
ImagePath : string = "https://jayanttripathy.com/wp-content/uploads/2022/04/cropped-JT.png";
<img src="{{ ImagePath }}" width="100" height="100"/>
Make use of a template reference variable
The template reference variable can also be used. The example below adds a template variable #name
to an input box. It can be used to retrieve the value of the input field {{name.value}}
.
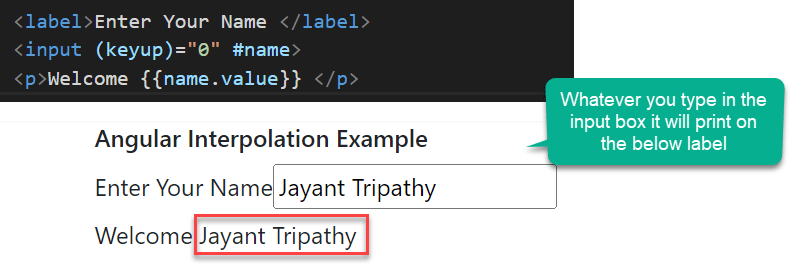
<label>Enter Your Name </label>
<input (keyup)="0" #name>
<p>Welcome {{name.value}} </p>
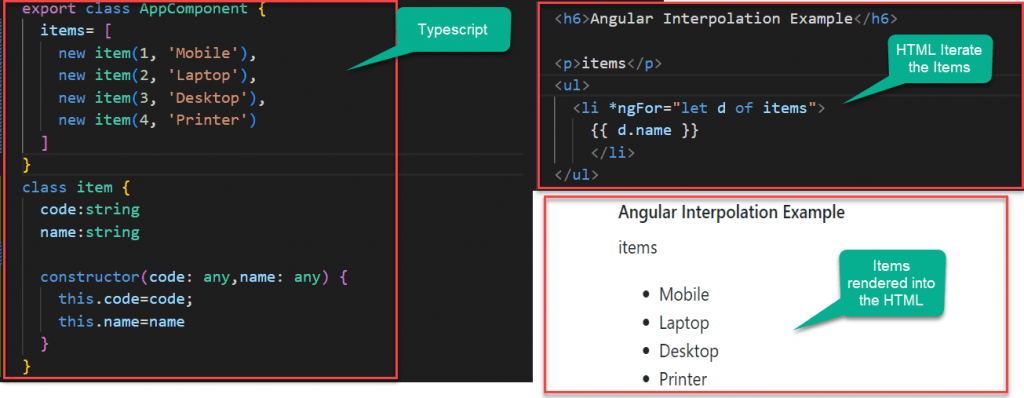
export class AppComponent {
items= [
new item(1, 'Mobile'),
new item(2, 'Laptop'),
new item(3, 'Desktop'),
new item(4, 'Printer')
]
}
class item {
code:string
name:string
constructor(code: any,name: any) {
this.code=code;
this.name=name
}
}
<h6>Angular Interpolation Example</h6>
<p>items</p>
<ul>
<li *ngFor="let d of items">
{{ d.name }}
</li>
</ul>
Cross-site scripting or XSS
Angular Sanitizes everything before inserting it into the DOM, preventing Cross-Site Scripting Security flaws (XSS). Values from the component’s property, attribute, style, class binding, or interpolation, for example, are sanitized. The script in the following example is not invoked, but rather displayed in its entirety.
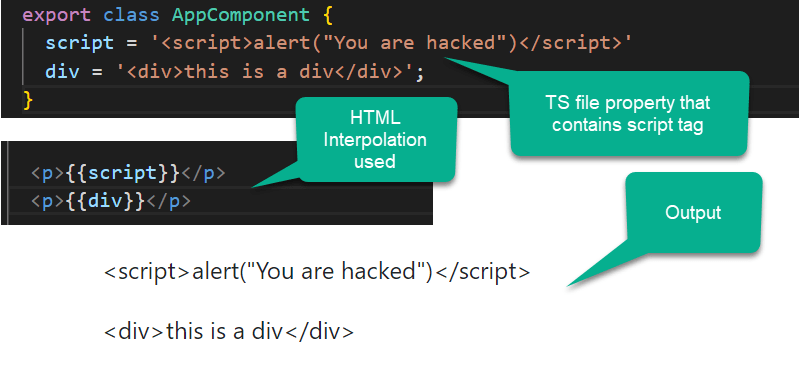
export class AppComponent {
script = '<script>alert("You are hacked")</script>'
div = '<div>this is a div</div>';
}
<p>{{script}}</p>
<p>{{div}}</p>
NgNonBindable
Use ngNonBindable
to tell Angular not to compile or bind the contents of the current DOM element. I.e any expression is not evaluated but shown as it is.
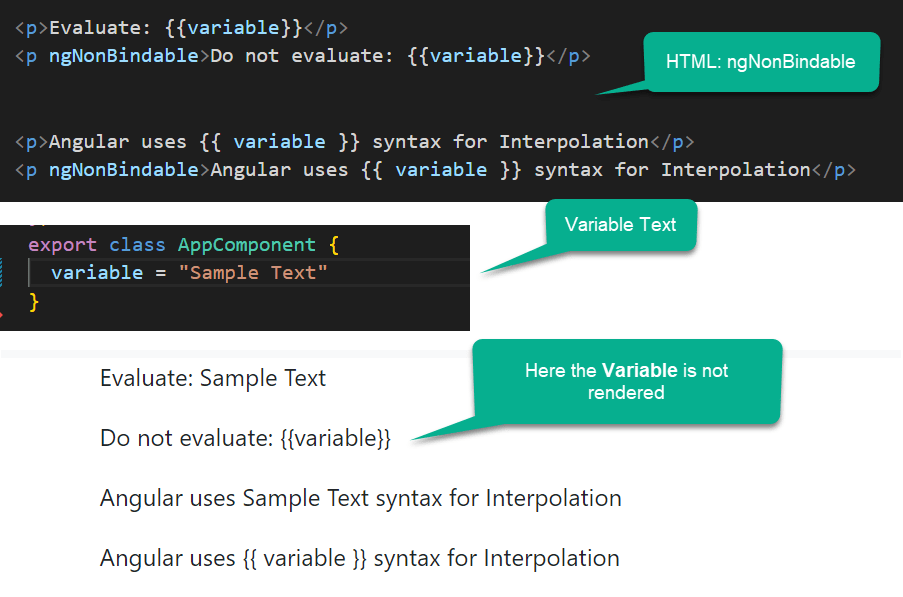
<p>Evaluate: {{variable}}</p>
<p ngNonBindable>Do not evaluate: {{variable}}</p>
<p>Angular uses {{ variable }} syntax for Interpolation</p>
<p ngNonBindable>Angular uses {{ variable }} syntax for Interpolation</p>
The safe navigation operator ( ? )
You can make use of a safe navigation operator ( ? ) to guards against null and undefined values.
<p>The item name is: {{nullItem.Name}}</p>
TypeError: Cannot read property 'itemName' of undefined
Use a safe navigation operator and the error goes away. Angular replace it with an empty string.
<p>The null item name is {{nullItem?.itemName}}</p>
Operator for non-null assertions
If you enable the –strictNullChecks flag in your tsconfig.json, Typescript will enforce strict null checking. Any variable that is not defined or is null results in a compiler error under strict null check. If the type checker cannot determine whether a variable will be null or undefined at runtime, it will throw an error.
You can tell typescript not to throw any compile errors by using the non-null assertion operator. It should be noted that this is a compile-time feature rather than a runtime feature.
The item's name is: {{item!.name}}
Conclusion
In this article we will learn about Angular Interpolation, Interpolation is a simple but powerful feature in Angular. It enables us to embed expressions in the string literal, allowing us to generate the string literal dynamically using values from the component class.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.