In this article we will discuss about Building Blocks of Angular Forms. Please read my previous article of Angular Template Driven vs. Reactive Forms.
The Angular Forms module consists of three Building blocks, irrespective of whether you are using Template driven forms in Angular or Reactive forms approach.
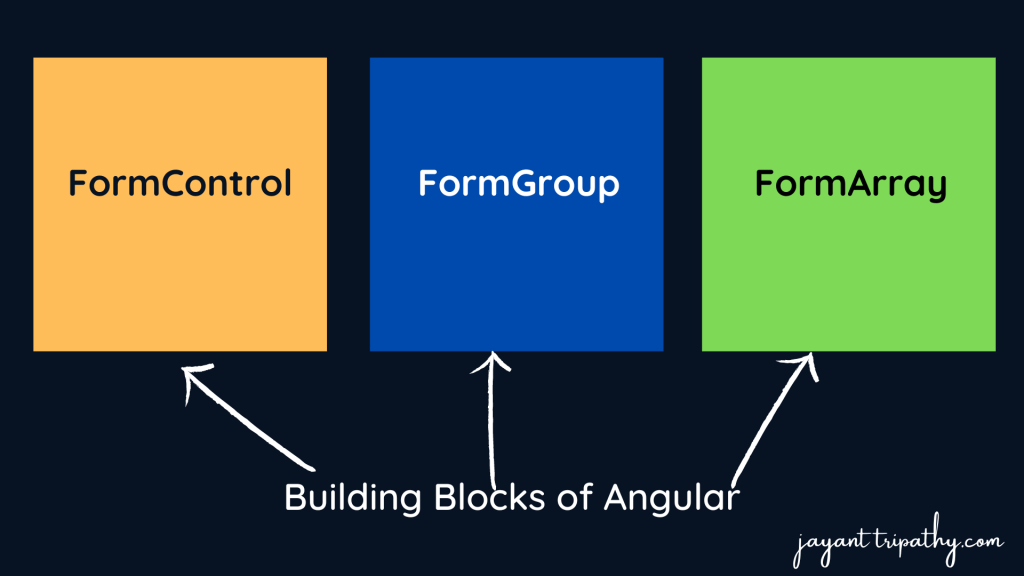
FormControl
A FormControl
represents a single input field in an Angular form. Let’s say, we would like to know the current value in the Text box. You would also be like to know if the value is valid or not. If the user has changed the value(dirty) or is it unchanged. You would like to be notified when the user changes value.
Consider a simple Text input box
Name : <input type="text" name="Name" />
The FormControl
is an object that encapsulates all this information related to the single input element. It Tracks the value and validation status of each of these control.
The FormControl
is just a class. A FormControl
is created for each form field. We can refer them in our component class and inspect its properties and methods.
FormControl
to set the value of the Form field, find the status of form field like (valid/invalid, pristine/dirty, touched/untouched ) etc & add validation rules to it.The above input field is created using the FormControl as shown below
let name= new FormControl(); //Creating a FormControl in a Reactive forms
Then, you can retrieve the current value in the input field using the value property
name.value //Returns the value of the first name field
You can check the validation status of the Name element as shown below
name.errors // returns the list of errors
name.dirty // true if the value has changed (dirty)
name.touched // true if input field is touched
name.valid // true if the input value has passed all the validation
FormGroup
FormGroup is a collection of FormControls
. Each FormControl
is a property in a FormGroup
. with the control name as the key. Often forms have more than one field. It is helpful to have a simple way to manage the Form controls together. Consider the following Form. we have three input fields street, city & Pincode.
<div ngModelGroup="address">
<div class="row mt-2">
<div class="col-sm-4">
<label for="city">City:</label>
</div>
<div class="col-sm-8">
<input type="text" name="city" class="form-control" ngModel>
</div>
</div>
<div class="row mt-2">
<div class="col-sm-4">
<label for="street">Street:</label>
</div>
<div class="col-sm-8">
<input type="text" name="street" class="form-control" ngModel>
</div>
</div>
<div class="row mt-2">
<div class="col-sm-4">
<label for="pincode">Pin Code:</label>
</div>
<div class="col-sm-8">
<input type="text" name="pincode" class="form-control" ngModel>
</div>
</div>
</div>
FormGroup
solve’s this issue by providing a wrapper interface around a collection of FormControls A FormGroup
tracks the status of each child FormControl
and aggregates the values into one object. with each control name as the key
We can group these input fields under the group address as shown below
let address= new FormGroup({
street : new FormControl(""),
city : new FormControl(""),
pinCode : new FormControl("")
})
In the above example, the address is our FormGroup
, consisting of 3 Form Controls city, street, and Pincode. Now we can check the validity of the entire group together. For example, if the state is invalid, then the address FormGroup returns the invalid state.
We can read the value of an address using the value method
// We can get the value using below code
address.value
//You can access child control as
address.get("street")
// We can check the validation error as
address.errors // returns the list of errors
address.dirty // true if the value of one of the child control has changed (dirty)
address.touched // true if one of the child control is touched
address.valid // true if all the child controls passed the validation
The Angular form is itself a FormGroup
FormArray
FormArray
is an array of form controls. It is similar to FormGroup
except for one difference. In FormGroup
each FormControl
is a property with the control name as the key. In FormArray
is an array of form controls.
We define the FormArray as shown below,
contactForm = new FormGroup( {
name: new FormControl(''),
cities:new FormArray([
new FormControl('Mumbai'),
new FormControl('Delhi')
])
});
You can get the reference to the cities
from the contactForm.get
method
cities() :FormArray {
return this.contactForm.get("cities") as FormArray
}
Check the Validation status as follows
cities.errors // returns the list of errors
cities.dirty // true if the value of one of the child control has changed (dirty)
cities.touched // true if one of the child control is touched
cities.valid // true if all the child controls passed the validation
Conclusion
In this tutorial, we learned what is Angular Forms all about. We looked at the basic building blocks of Angular Forms i.e. FormGroup
, FormControl
& FormArray
. The Angular allows us to build Forms using two different approaches.
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.