In this article we will learn about Building Single Page Applications(SPA) with ReactJS. A Single-Page application (SPA) is a webpage that dynamically interacts with the web browser by rewriting the current web page with data from the webserver. As a result, the webpage does not reload during its execution and instead operates in a browser. Please read my previous lesson on React of How to create a react App.
Difference between Single Page applications and Multi Page applications?
Single-page applications are ideal for developing dynamic, fast-loading websites, particularly if you intend to create a mobile app. The most significant disadvantage of this model is that it has poor search engine optimization and ranks lower in search engines. As a result, it is suitable for social networks, secret groups, and SaaS apps that do not require SEO. Examples include Gmail, Google Maps, Facebook, and GitHub.
Multi-Page Applications, on the other hand, are useful for online stores, catalogs, and commercial websites because they can manage vast volumes of data that require multiple pages, features, and menus. React Js developers, on the other hand, are working on methods to allow single-page applications to handle enormous volumes of data.
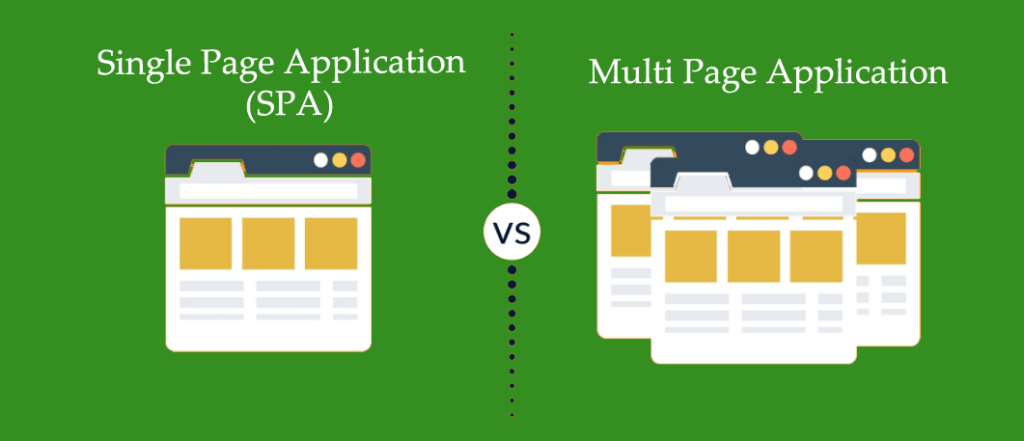
Create a Single Page Application using ReactJS
Step-1
- To create a React App use the below command.
- Although, it also generates a directory called “app-name” that contains some default files.
npx create-react-app app-name
Step-2: install react-router-dom using below command
npm install react-router-dom
Step-3 : Wrapping The App Component
React Router has 2 types of routers: BrowserRouter
and HashRouter
. We’re using BrowserRouter in this example because it makes the URL’s look like example.com/about rather than example.com/#/about. React Router uses a hash (hash) at the end of the URL to determine what page to load.
Index.js
import React from "react"
import { render } from "react-dom"
import { BrowserRouter } from "react-router-dom"
import App from "./App"
render(
<BrowserRouter>
<App />
</BrowserRouter>,
document.querySelector("#root")
)
We created two pages HomePage.js and Index.js
import React from 'react';
export default function HomePage() {
return (
<div style={{ padding: 10 }}>
<h3>HomePage Content</h3>
<p>This is your awesome HomePage subtitle</p>
</div>
);
}
import React from 'react';
import { useParams } from 'react-router-dom';
export default function UserPage() {
let { id } = useParams();
return (
<div style={{ padding: 10 }}>
<h3>UserPage Content</h3>
<p>This is your awesome UserPage subtitle</p>
</div>
);
}
App.js
Select and integrate the routers you want to use by using Switch and Route. Switch aggregates all routes and prioritizes them from top to bottom. The route, on the other hand, defines individual routes. Additionally, the routes must be included in your App.js file.
import './style.css';
import React from 'react';
import { Route, Routes, Switch, Link } from 'react-router-dom';
// We will create these two pages in a moment
import HomePage from '../pages/HomePage';
import UserPage from '../pages/UserPage';
export default function App() {
return (
<div>
<div className="p-2 w-100 d-flex flex-row border">
<Link to="/" className="m-2">
Home
</Link>
<Link to="/2" className="m-2">
User
</Link>
</div>
<div>
<Routes>
<Route path="/" element={<HomePage />} />
<Route path="/2" element={<UserPage />} />
</Routes>
</div>
</div>
);
}
That’s it. You can see the preview on below screen.
Get Source Code link here: https://github.com/JayantTripathy/reactjs-starter-app
What are the benefits of a Single-page application?
Some of the benefits of adopting single-page applications (SPAs) are as follows:
- Because SPA is faster, all webpage resources are loaded only once throughout the application, and data is the only resource transmitted.
- Furthermore, because it transmits just one request, maintains all data, and uses it even when offline, SPA effectively caches local storage.
- In fact, because it eliminates the need to write code to render pages on the server, SPA simplifies and streamlines development activities.
- Chrome makes it easy to troubleshoot SPAs by allowing you to explore page elements and monitor network activity.
When should single-page applications be avoided?
While SPA has its advantages, there are specific scenarios in which it should not be used. So, there are specific situations in which you should avoid using single-page apps. You may also hire a react js developer to advise you on where you should not utilize the library:
- SEO difficulties: Any web app is written in JavaScript loads data without reloading the page and only when the user requests it. This means there are no specific URLs optimized for search engines, and the content is not visible to search engines. The problem can only be solved by server-side rendering.
- Time to download: Users’ browsers will take longer to load content if the platform is sophisticated, vast, and poorly optimized.
- Support for JavaScript: You won’t be able to use the full functionality of an app without this feature. Users who disable JS in their browser will be unable to fully utilize the app.
Conclusion
In this article we discussed about Building Single Page Applications(SPA) with ReactJS. A Single-Page application (SPA) is a webpage that dynamically interacts with the web browser by rewriting the current web page with data from the webserver.