In this article we will learn about Class binding in Angular. Class binding in Angular makes it very easy to set the class property of a view element. We can set or remove the CSS class names from an element’s class attribute with the help of class binding. Please read my previous article Attribute binding in Angular.
What is Class binding in Angular?
The Angular Class Binding is used to add or remove classes to and from the HTML elements. It is also possible in Angular to add CSS Classes conditionally to an element, which will create the dynamically styled elements and this is possible because of Angular Class Binding.
Syntax
<element [class] = "typescript_class_property">
Approach to use the class binding
- Define a property element in the
ts
file. - In the
html
file, set the class of the HTML element by assigning the property value to thets
file element.
Understanding Class Binding in Angular Application
Let’s say we have the below css
in app.component.css
file
.customClass
{
color: red;
text-decoration: underline;
font-weight: bold;
}
Add in the app.component.html
file and here we use the css class like below and we see the output,
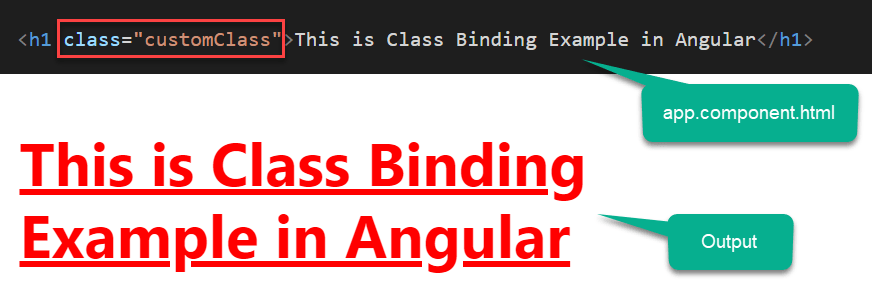
Class binding with ClassName
The ClassName is the property name of HTML Element. Hence we can make use of Property binding to assign the class name to any HTML element. The following example assigns CSS Class to the div element.
<div [className]="'customClass'">This is Class Binding Example in Angular</div>
You can also add more than one class by separating them using like below,
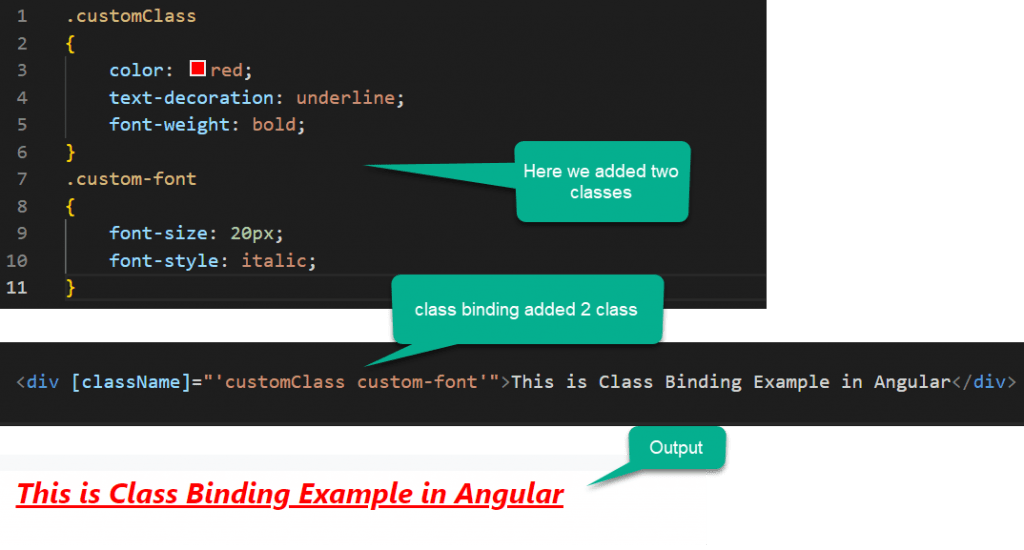
Should we can use both class and [className]
in the class attribute
Mixing both class
and [className]
results in removal of class
attribute. We cannot use both, you can see the customclass attribute is now work to us.
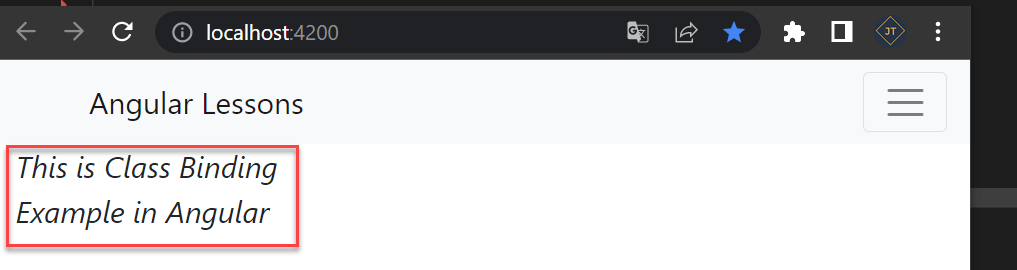
Conditionally apply Classes
We can also bind the class name dynamically. To do that first create a variable in your component class like below,
cssStringVar = 'customClass custom-font';
<div [className]="cssStringVar">This is Class Binding
Example in Angular</div>
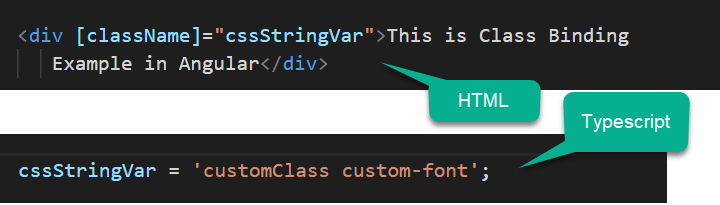
Class based on some condition
Also we can create a function, which returns the class based on some condition.
getClass() {
return 'customClass custom-font';
}
<div [className]="getClass()">This is Class Binding
Example in Angular</div>
Class based using Ternary Operator
We can use ternary operator like below, if hasError()
is true then it call first css else 2nd css.
hasError() {
return false
}
<div [className]="hasError() ? 'customClass' : 'custom-font'">This is Class Binding
Example in Angular</div>
Class binding with Class
There are another shorthand way to bind CSS Class to HTML element.
<div [class.<className>]="condition"></div>
ClassName is name of the class, which you want to bind. condition must return true or false. A return value of true adds the class and a false removes the class.
<div [class.customClass]="true" [class.custom-font]="true">Test</div>
Conditionally binding class
To dynamically or conditionally bind a class, First create a variable in the component class as shown below.
<div [class.customClass]="hasError" [class.custom-font]="hasError">This is Class Binding
Example in Angular</div>
Conclusion
Here we discuss about Class binding in Angular. Class binding in Angular makes it very easy to set the class property of a view element. We can set or remove the CSS class names from an element’s class attribute with the help of class binding.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.