In this article we are going to discuss how to Create custom middleware in ASP.Net Core. Please go through our previous article Middleware in ASP .NET Core.
Creating Custom Middleware In ASP.Net Core. Middleware is the new “pipeline” for requests in asp.net core. Each piece of middleware can process part or all of the request, and then either choose to return the result or pass on down to the next piece of middleware.
If you want to execute your own logic and do some stuff like authentication/authorization, Error handling and maintain the application log information etc. in that scenario you can use Create custom middleware and use of it own.
Custom Middleware in ASP.NET Core
Visual Studio includes template for creating standard middleware class. For this, right click on the project or folder where you want to create middleware class and select Add -> New Item. This will open Add New Item popup. Search for word “middleware” in the top right search box as shown below.
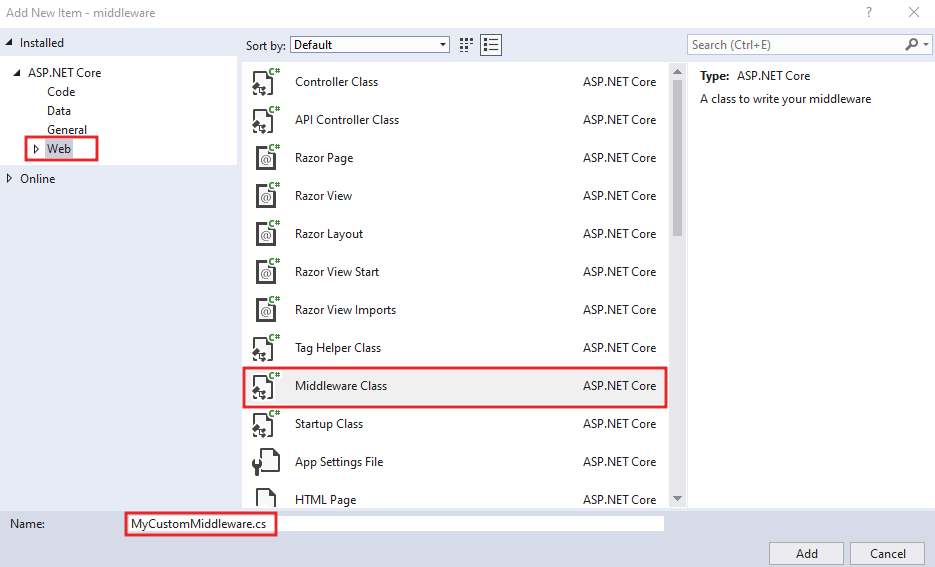
Once it added into the project then the middleware code looks like below;
// You may need to install the Microsoft.AspNetCore.Http.Abstractions package into your project public class MyCustomMiddleware { private readonly RequestDelegate _next; public MyCustomMiddleware(RequestDelegate next) { _next = next; } public Task Invoke(HttpContext httpContext) { return _next(httpContext); } } // Extension method used to add the middleware to the HTTP request pipeline. public static class MyCustomMiddlewareExtensions { public static IApplicationBuilder UseMyCustomMiddleware(this IApplicationBuilder builder) { return builder.UseMiddleware<MyCustomMiddleware>(); } }
The custom middleware component is like any other .NET class with Invoke() method. However, in order to execute next middleware in a sequence, it should have RequestDelegate type parameter in the constructor.
We need to Customize the middleware means we are going to add a logging mechanism to check the custom middleware process flow.
public class CustomLoggerMiddleware { private readonly RequestDelegate _next; private readonly ILogger _logger; public CustomLoggerMiddleware(RequestDelegate next, ILoggerFactory logFactory) { _next = next; _logger = logFactory.CreateLogger("MyMiddleware"); _logger.LogInformation("MyMiddleware is Started"); } public async Task Invoke(HttpContext httpContext) { _logger.LogInformation("MyMiddleware is executing.."); await _next.Invoke(httpContext); } }
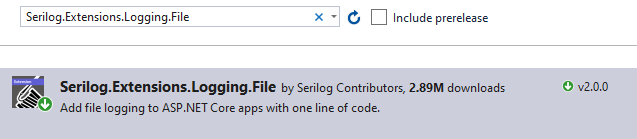
And after adding this we need to inject the logger in Configure() method in startup.cs class like below. Here the log is append in the text file and save into the folder named “Logs” in the root of the application.
public void Configure(IApplicationBuilder app, IHostingEnvironment env, ILoggerFactory logFactory) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseCustomLoggerMiddleware(); app.Run(async (context) => { await context.Response.WriteAsync("Hello World check from Run method!"); }); logFactory.AddFile("Logs/myapp-{Date}.txt"); }
When we run the application you can see the text file is created with the logging information.
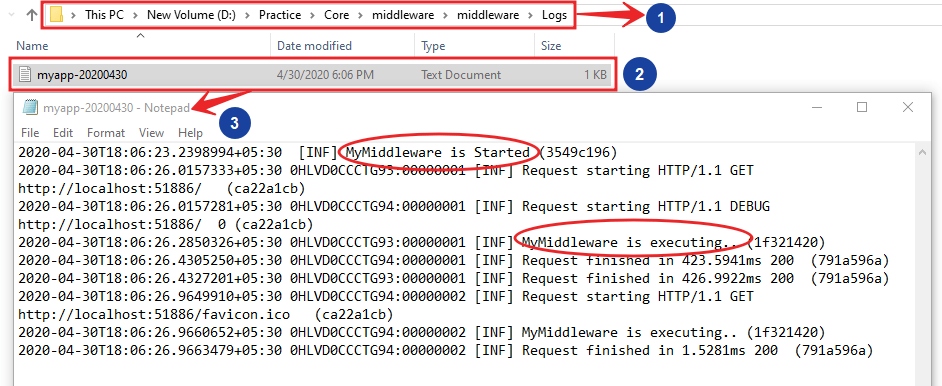
Another Example to discuss about Custom Middleware
public void Configure(IApplicationBuilder app, IHostingEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseCustomLoggerMiddleware(); app.Run(async (context) => { await context.Response.Body.WriteAsync(Encoding.ASCII.GetBytes("This is called from Configure() method response")); }); }
Then we add some text as well in our custom middleware in Invoke() like below;
public async Task Invoke(HttpContext httpContext) { await httpContext.Response.Body.WriteAsync(Encoding.ASCII.GetBytes("Called from Custom Middleware -- ")); await _next.Invoke(httpContext); }
Finally Run the application and see the output like below;
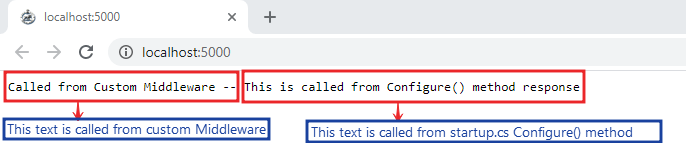
You can see both the text is append, this is due to we call app.UseCustomLoggerMiddleware()
and also the then Run() method will execute and the response is printed.
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.