In this article we discuss model in ASP.Net Core MVC. You can go through my previous article of Set up MVC in ASP.NET Core application. To learn step by step features about ASP .NET Core form beginners to advanced level please go through our article and videos on this link.
What is a Model in ASP.NET Core MVC ?
Model in ASP.NET Core MVC contains the business logic layer that can communicate with underlying data source such as database,XML,Json etc. and return the data to controller. So in the simple word we can say that the model in MVC is used to manage the data i.e. the state of the application in memory. It is not mandatory, but it is a good programming practice to store all model classes within the Models folder.
Adding Models in Folder
Right click on Solution Project then click Add=> New Folder and rename it into “Models“. Then add here a class name as “Customer.cs” and the customer information property.
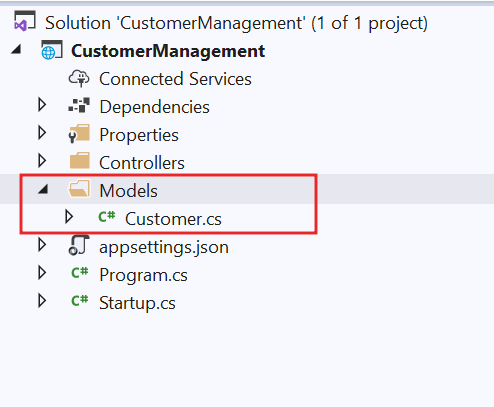
Models
The Customer class should be like below;
public class Customer { public int custid { get; set; } public string name { get; set; } public string address { get; set; } public string phone { get; set; } public string email { get; set; } public string gender { get; set; } }
So in above we declare the customer class and we need to create a interface and implement a class that return the set of customers information.
Creating ICustomerRepository interface:
Let’s create a folder named as “Repository” inside the Models folder and create a interface like below.
public interface ICustomerRepository { Customer GetCustomerId(int CustomerId); }
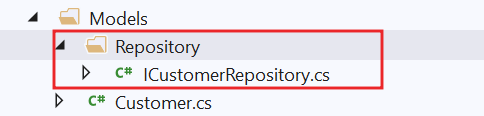
Creating CustomerRepository class
Let us create an implementation class for the above ICustomerRepository interface. In our upcoming article, we will discuss how to retrieve the customer details from a database. But for this demo, lets hard-coded the customer details. So, create a class file with the name CustomerRepository within the Repository folder and implement this class into the interface ICustomerRepository.
public class CustomerRepository:ICustomerRepository { private List<Customer> _customerList; public CustomerRepository() { _customerList = new List<Customer>() { new Customer() { custid = 001, name = "John Smith", address = "USA", phone="6737540477", email = "john.smith@outlook.com", gender = "Male" }, new Customer() { custid = 002, name = "Lori Orona", address = "USA", phone="6729837538", email = "lori.orona@gmail.com", gender = "Female" }, new Customer() { custid = 003, name = "Steve Oman", address = "UK", phone="4252573576", email = "steve333@yahoo.com", gender = "Male" }, new Customer() { custid = 004, name = "Renna Sky", address = "USA", phone="5426589045", email = "renna@outlook.com", gender = "Female" }, new Customer() { custid = 005, name = "Steve Khan", address = "UAE", phone="4252573576", email = "steve333_k@yahoo.com", gender = "Male" } }; } public Customer GetCustomerId(int CustomerId) { return this._customerList.FirstOrDefault(e => e.custid == CustomerId); } }
Access the model information in Controller
To access the model repository in Controller we need to modify the controller like below and simply return the Json data like below;
public class HomeController : Controller { public JsonResult GetCustomerDetails(int Id) { ICustomerRepository repository = new CustomerRepository(); Customer customerDetails = repository.GetCustomerId(Id); return Json(customerDetails); } }
Let’s run the application and pass the customer id then you can see the output like below.

On above, we see how the model can communicate with repository and return back the result to controller. In this example we take the customer dummy data, on future article we can see how the data will fetch using database.
The way we implemented the GetCustomerDetails method of Home Controller is not loosely coupled. That means tomorrow if the implementation class of ICustomerRepository is changed then we need to change the code in Home Controller class as both are tightly coupled. We can overcome this problem by implementing Dependency Injection design pattern.
On our next article we will learn how to use Dependency Injection in ASP .NET Core application.
Video Summary
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂