In this article we will learn about Building Serverless ASP.NET Core Web API with AWS Lambda using Function URLs. Serverless technologies enable developers to concentrate on what the application does without the hassle of managing where it runs and how it run. Serverless Api creates a collection of Amazon API Gateway resources and methods that can be invoked through HTTPS endpoints. Please read my previous article about AWS – Create An Amazon AWS Free Tier Account.
To make the API available on a public HTTP(S) endpoint, you will use Lambda function URLs instead of Amazon API Gateway.
Pre-requisites
- Visual Studio 2022
- AWS Toolkit for Visual Studio
- AWS Account
- .NET 6
Creating ASP.Net Core Web API Project
Open Visual Studio, create New Project and select ASP.NET Core Web API template to create a new Web API project.

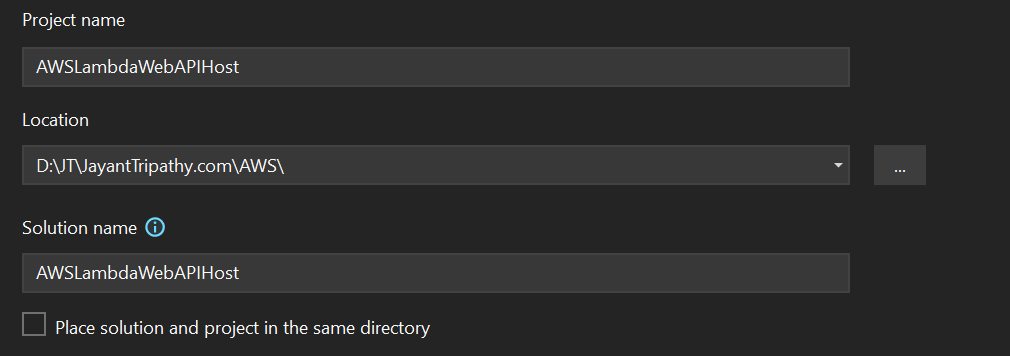
.NET 6 introduces a new style of writing ASP.NET Core applications called Minimal APIs. Minimal API makes use of C# 9’s new feature top-level statements. Minimal API allows you to define an entire ASP.NET Core application in a single file.
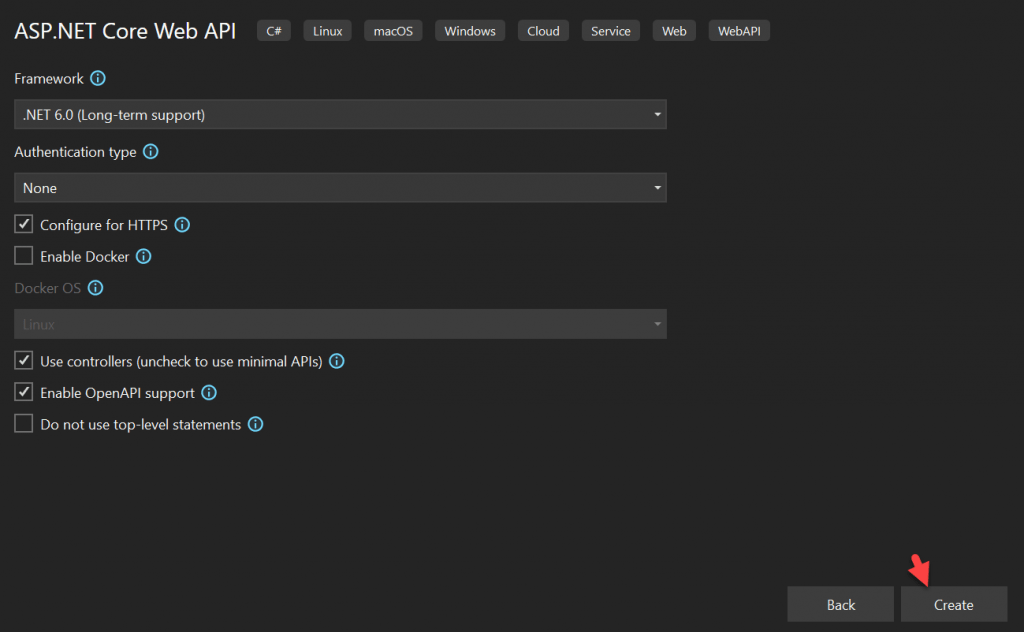
Install Amazon.Lambda.AspNetCoreServer.Hosting NuGet package
To deploy ASP.NET Core application using Minimal API to Lambda, we need to install Amazon.Lambda.AspNetCoreServer.Hosting
NuGet package on the existing application.
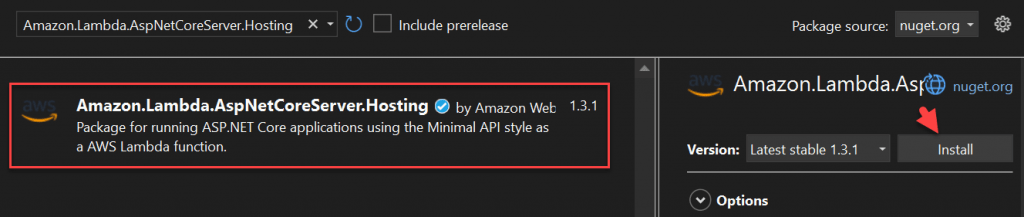
Adding AddAWSLambdaHosting
method when services are defined in your application.
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllers();
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
// Add AWS Lambda support.
builder.Services.AddAWSLambdaHosting(LambdaEventSource.HttpApi);
var app = builder.Build();
When the ASP.NET Core project is run locally, AddAWSLambdaHosting
does nothing, allowing the normal .NET Kestrel web server to handle the local experience. When running in Lambda, AddAWSLambdaHosting
swaps out Kestrel with Amazon.Lambda.AspNetCoreServer
allowing Lambda and API Gateway to act as the web server instead of Kestrel. Since Minimal APIs take advantage of top-level statements, AddAWSLambdaHosting
also starts the Lambda runtime client.
Adding AWSProjectType inside PropertyGroup
Right click on the project and click Edit .csproj
file and add Lambda in PropertyGroup
section.
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>net6.0</TargetFramework>
<Nullable>enable</Nullable>
<ImplicitUsings>enable</ImplicitUsings>
<AWSProjectType>Lambda</AWSProjectType>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Amazon.Lambda.AspNetCoreServer.Hosting" Version="1.3.1" />
<PackageReference Include="Swashbuckle.AspNetCore" Version="6.2.3" />
</ItemGroup>
</Project>
This will let AWS Toolkit for Visual Studio know that your project is an AWS project. Now, right-click on your project, you will start getting Publish to AWS. Please read my previous article How to get AWS Toolkit for visual studio.
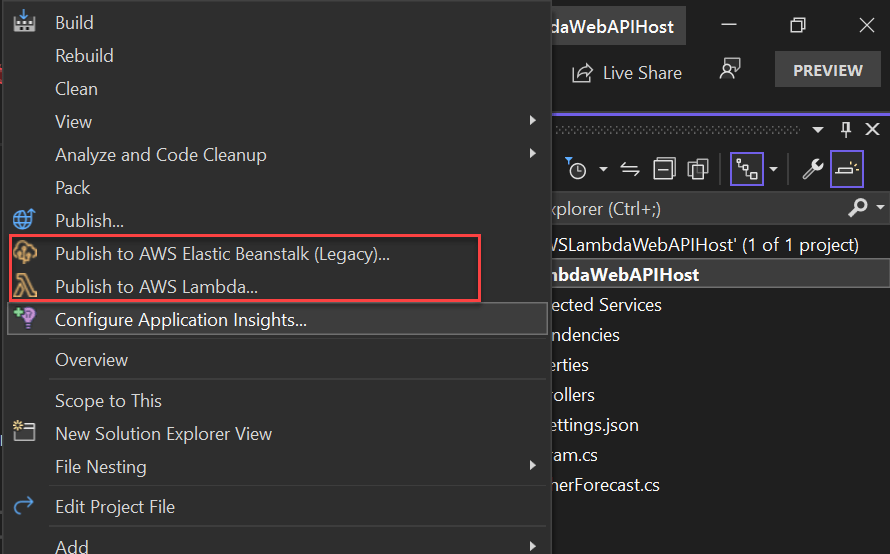
Deploy ASP.Net Core Web API on AWS Lambda
Follow the instructions below to deploy Web API on AWS Lambda.
- Right-click on the project, and select Publish to AWS Lambda.
- In Upload Lambda Function dialog, ensure the following values are correct.
- Lambda Runtime should be .NET 6
- Configuration should be Release
- Handler must be the Assembly name of your application

In the Advanced Function Details dialog, you can configure the following things.
- IAM Role for the Lambda Function, when in doubt, select AWSLambdaBasicExecutionRole from the dropdown.
- Memory & Timeout values
- Environment variables
- DLQ & VPC details (if needed)
And click on the upload button.
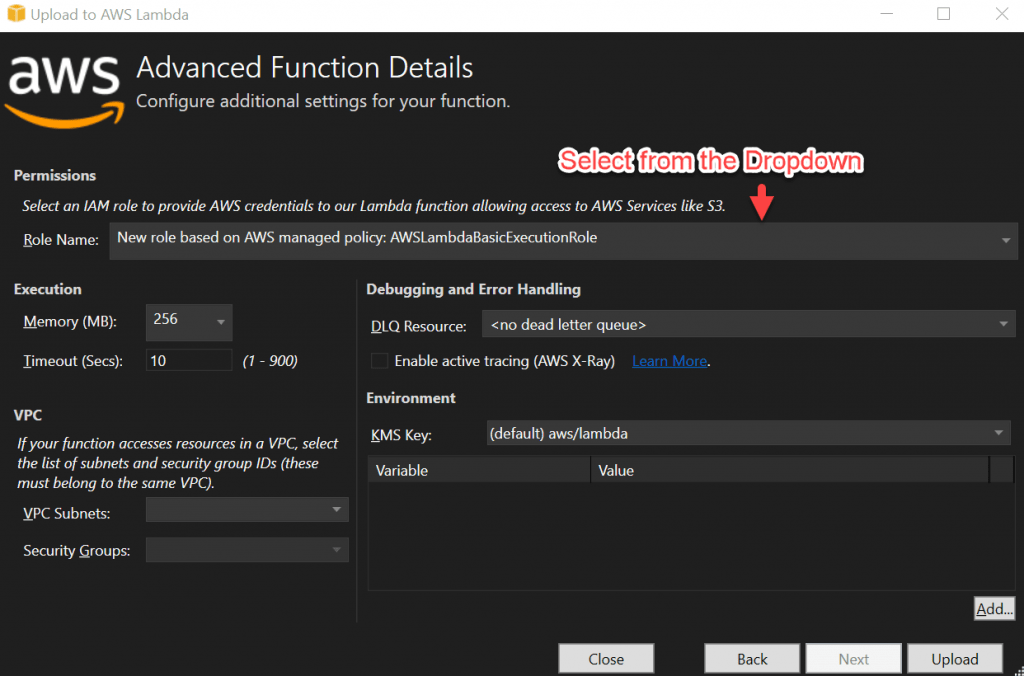
After clicking the upload button you can see it will getting publish the file in AWSLambda.

After uploading we can see our API is uploaded on AWSLambda.
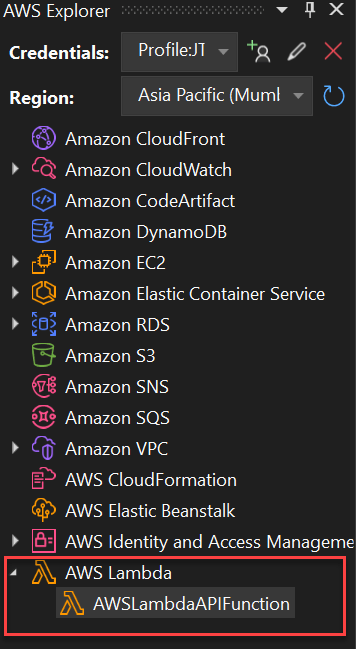
Enable Function Url for the Lambda
Follow the instructions below to enable Function Url for the Lambda.
- Go to AWS Console & navigate to Lambda service.
- You can see the deployed Lambda Function in AWS Console.
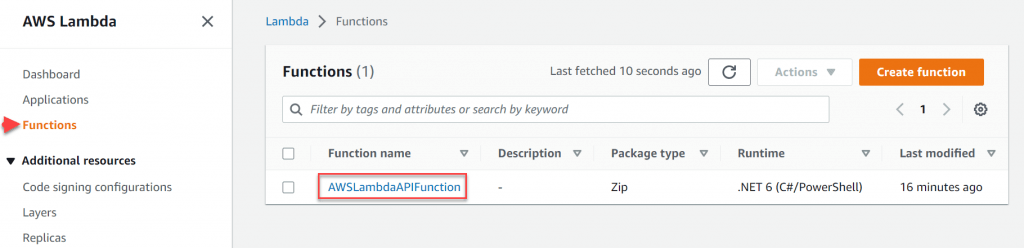
- Select your Lambda function to go to its detail page.
- Select Configuration tab and then select Function URL from the left menu.

- Click on Create function URL button.
- On Configure Function URL screen, select NONE as Auth type for now as I don’t want to any authentication for now.
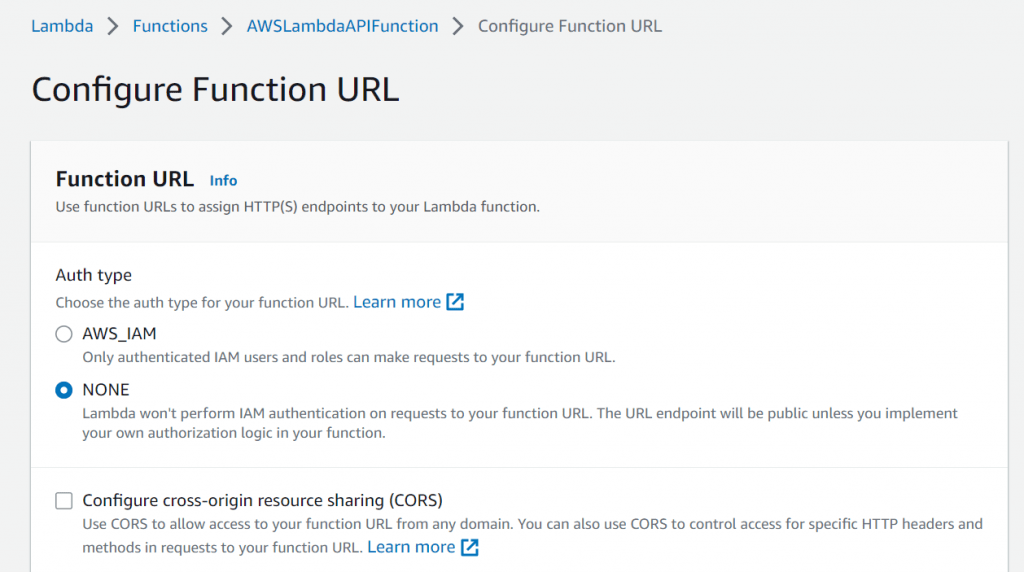
- Click on the Save button.
- You can see, Function URL has been created, and you can use it to access your Web API.

That’s it for the configuration of AWSLambda API.
Test the configured Lambda API
Follow the instructions below to verify that API is working.
- Copy the Function URL.
- Append WeatherForecast in the URL, as this is the only controller we have in the default ASP.NET Core Web API.
- Copy the new URL in the browser and see the result.
- If everything is fine, you will get the JSON response from the API like below.
https://nuekivwefikym4webmlq3w3fi40hwuwb.lambda-url.ap-south-1.on.aws/WeatherForecast
The above function URL I removed later, due to unavoidable calls.

Conclusion
In this post, we learned Building Serverless ASP.Net Core Web API with AWS Lambda using Function URLs. Running Web APIs on AWS Lambda helps you to make your system scalable as well as cost-effective.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
References
Related Articles
- How to install and configure AWS Toolkit for VS Code
- How to send SMS using Amazon Simple Notification Services(SNS)
- Send Emails from ASP.NET Core using Amazon SES
- How to Deploy Blazor WebAssembly to AWS Amplify
- How to host Angular app on AWS S3 bucket using CloudFront
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.