In this article we are going to discuss Introduction to ASP.NET Core MVC Framework. Please read my previous article where we discussed How to Create Custom Middleware in ASP.NET Core.
The main objective of the MVC design pattern is the separation of concerns, the Data Access Layer and Business logic are separated from the user interface (i.e. view). As a result, maintaining and testing the application becomes simpler and easier.
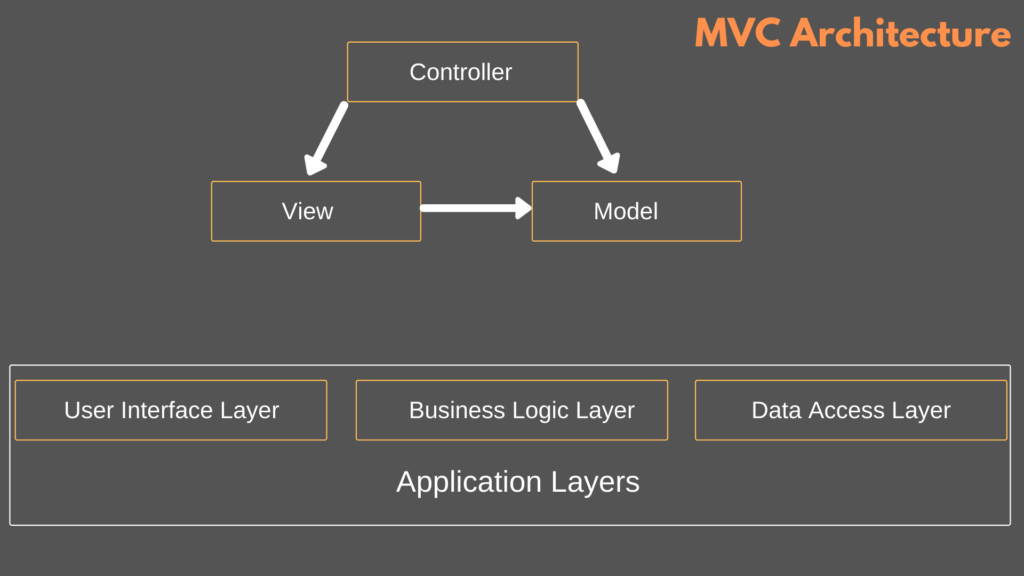
How MVC Design Pattern Works ?
Let us see an example to understand how the MVC pattern works in asp.net core MVC application. For example, we want to design an application, where we need to display the customer details in a web page as shown below.
Customer Details | |
---|---|
Customer Id | 1 |
Customer Name | Jhon Smith |
Customer Address | USA |
Customer Mobile no. | 6743289654 |
When we want to access the details from web browser the URL should be like this, https://jayanttripathy.com/customer/details/1
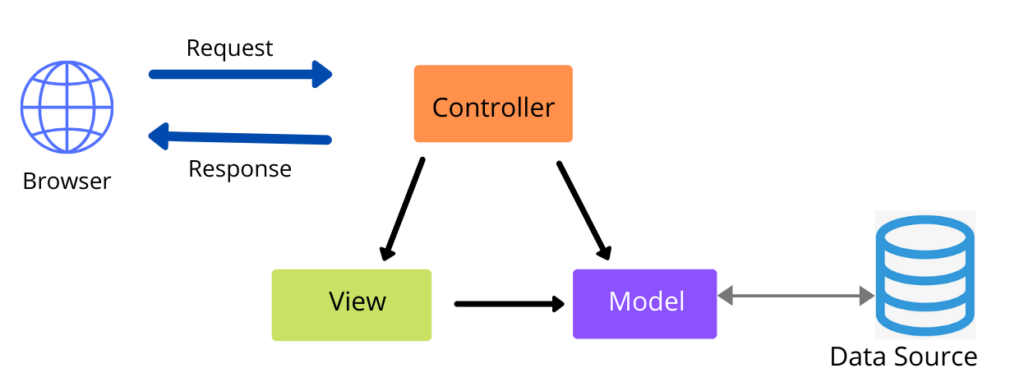
- When a user request through the server, it goes to the controller in the MVC design pattern that receives the request and handles it. The controller creates the model. The model has the classes that describe the data.
- In addition to the data itself, Model also contains the logic to retrieve data from the underlying data source such as a database.
- In addition to creating the Model, the controller also selects a View and passes the Model object to that View.
- The view is only responsible for presenting the model data. View, that generates the required HTML to present the model data i.e the customer data provided to it by the Controller.
- HTML is sent over the network to user who make the request.
Model
Model is the component in MVC Design pattern which is used to manage that data i.e. state of the application in memory. The Model represents a set of classes which is used to describe the applications validation logic, business logic, and data access logic. In our example, the model consists of customer class and the Customer Repository.
public class Customer { public int Id { get; set; } public string Name { get; set; } public string Address { get; set; } public string Mobile { get; set; } } public interface ICustomerRepository { Customer GetCustomer(int id); void Save(Customer customer); } public class CustomerRepository : ICustomerRepository { public Customer GetCustomer(int id) { // Logic to retrieve customer details } public void Save(Customer customer) { // Logic to save customer details } }
Here, in our example, we use the Customer class to hold the customer data in memory. The CustomerRepository class is use to manage the customer data i.e. going to perform the CRUD operation.
So, in short, we can say that a Model in MVC design pattern contains a set of classes which is use to represent the data and also contains the logic to manage those data. In our example, the Customer class is the class which is use to represent the data. The CustomerRepository is the class which is use to manage the customer data.
View
The view component in MVC Design pattern is use to contain the logic to represent the model data as a user interface with which the end-user can interact. Basically, the view is use to render the domain data (i.e. business data) which is provide to the controller.
For example, we want to display Customer data in a web page. In the following example, the Customer model carried the customer data to the view. As already discussed, the one and only responsibility of the view is to render that customer data. The following code does the same thing.
@model CustomerManagement.Customer <html> <head> <title>Customer Details</title> </head> <body> <table class="has-fixed-layout" style="width:50%"> <thead> <tr><th colspan="2">Customer Details</th></tr> </thead> <tbody> <tr><td>Customer Id</td><td>1</td></tr> <tr><td>Customer Name</td><td>Jhon Smith</td></tr> <tr><td>Customer Address</td><td>USA</td></tr> <tr><td>Customer Mobile no.</td><td>6743289654</td></tr> </tbody> </table> </body> </html>
Controller
The Controller is the component in an MVC application which is used to handle the incoming HTTP Request and based on the user action, the respective controller will work with the model and view and then sends the response back to the user who initially made the request. So, it is the one which will interact with both the models and views to control the flow of application execution.
In this case the user issued a request to the URL (/customer/details/1), So this request is mapped to the Details action method in the Customer Controller, passing it the Customer Id which in this case is 1. This mapping is follow by the Routing rules defined in our application.
public class CustomerController : Controller { private CustomerRepository _customerRepository; public CustomerController(ICustomerRepository customerRepository) { _customerRepository = customerRepository; } public IActionResult Details(int id) { Customer model = _customerRepository.GetCustomer(id); return View(model); } }
As you can see, from the code in the Details action method, the controller builds the model, in this case the Model is the Customer object. To retrieve the Customer data from the underlying data source, the controller is making use of the CustomerRepository class.
Video Summary
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.