In this article we will learn about NgModelChange & Change Event in Angular. ngModelChange
is an Angular specific event, which we can use to listen for changes to the user input. It is the @Output
property of the ngModel
directive, ngModel
raises the NgModelChange
event, whenever the model changes. Another way to listen for change is to use the change DOM event. We also learn how to use them and also the difference between change & ngModelChange
. Please read my previous article Two Way Data Binding in Angular.
NgModelChange Syntax
The following is the simple example of ngModelChange
. We assign the method in the component class (handler function) to the ngModelChange using the event binding syntax
<input type="text" name="name" ngModel (ngModelChange)="nameChanged($event)">
nameChanged
is the handler function, which we need to define in the component class. We can access the new value by using the $event
as an argument to the handler function.
//Component
nameChanged(arg) {
console.log("modelchanged " + arg);
}
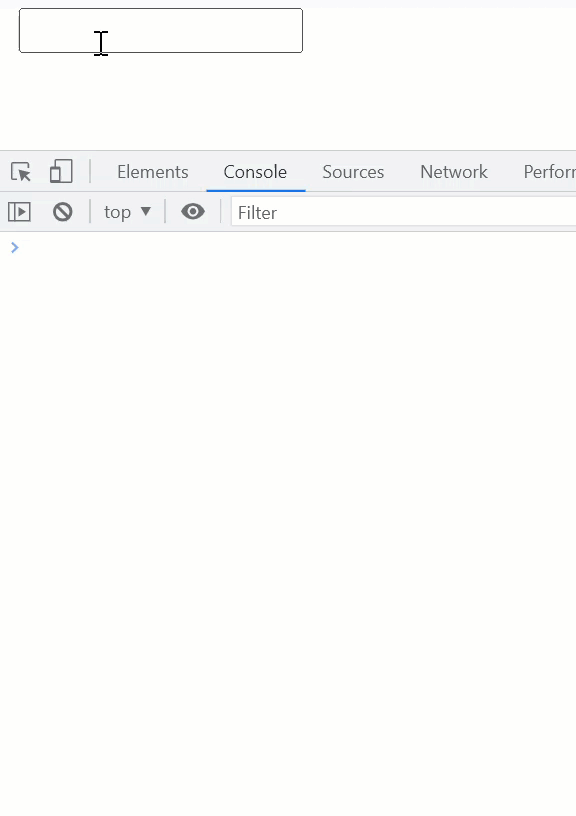
ngModel
We usually use the ngModel as follows to achieve Two Way Data Binding in Angular. [(ngModel)]="email"
keeps the email property in the component class in sync with the template.
<input type="text" name="email" [(ngModel)]="email">
Angular internally converts the preceding syntax to the following syntax.
<input [ngModel]="email" (ngModelChange)="email = $event">
The component property email is bound to the input element using the Property Binding [ngModel]="email"
. Any changes made to the input is updated in the component using the (ngModelChange)="email = $event"
Event binding in Angular.
Change Event
The (change) is a DOM event fires when changes to the form fields like <input>, <select> and <textarea>
is committed by the user.
- This event fires when user changes the input & moves the focus away from the text box (blur event).
- On
<select>
it fires when the user selects a new option either by a mouse click or using a keyboard. - Fires when the state of a check box or radio button change due to users action.
Example of Change Event
The change event for text element fires when we move the focus away from the element (blurred the input). This is different from the ngModelChange
, which fires the event for each input change.
The other import point is the $event
parameter. In the ngModelChange
, it is always the new value. But in the case of a change event, it is event data. The event data is an object containing data about the event. We need to use the target.value
to access the value.
Name :
<input type="text" name="name1" (change)="name1Changed($event)">
<br>
country :
<select name="country1" (change)="country1Changed($event)" >
<option [ngValue]="null" disabled>Select Country</option>
<option *ngFor="let country of countries" [ngValue]="country.id">{{country.name}}</option>
</select>
import { Component, EventEmitter, Input, Output } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
countries = [
new country(1, 'India'),
new country(2, 'USA'),
new country(3, 'UK'),
new country(4, 'Germany')
]
name1Changed(arg: any) {
console.log("name1Changed " + arg.target.value);
console.log(arg);
}
country1Changed(arg: any) {
console.log("country1Changed " + arg.target.value);
console.log(arg);
}
}
class country {
id: number = 0;
name: string = '';
constructor(id: any, name: any) {
this.id = id;
this.name = name
}
}
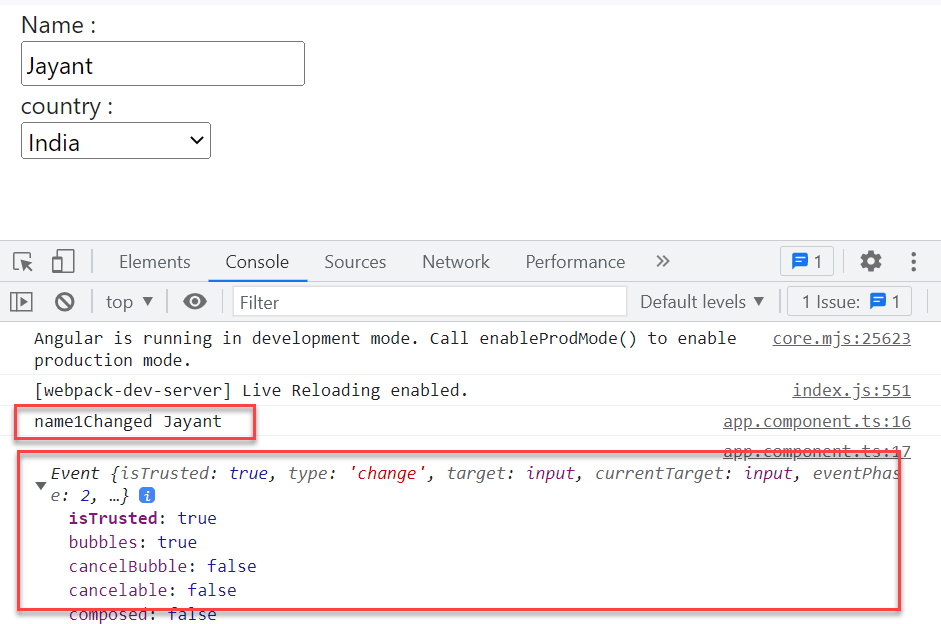
NgModelChange | Change Event |
NgModelChange is Angular specific event | Change is a DOM Event and has nothing to do with the Angular. |
We must use the ngModelChange along with the ngModel directive | You can use change event on <input>, <select>, and <textarea> form elements. |
ngModelChange event passes new value | Change event passes event parameter, Use the target.value to access the new value |
ngModelChange will trigger with each input change | Change event fires when you remove the focus from input text after changing the content. |
Conclusion
Here we discussed about NgModelChange & Change Event in Angular. ngModelChange
is an Angular specific event, which we can use to listen for changes to the user input. It is the @Output
property of the ngModel
directive, ngModel
raises the NgModelChange
event.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.