In this article we will learn about Async Pipe in Angular. The async pipe allows us to subscribe
to an observable
or Promise
from the template and returns the value emitted. The async pipes subscribe to the observable when the component loads. It unsubscribes when the component gets destroyed. Please read my previous article Angular KeyValue Pipe.
Syntax of Async Pipe
The following is the syntax of the async pipe. expression must return an observable or promise. It is followed by |
(pipe character) and the keyword async. We are using the async pipe with interpolation syntax.
{{expression | async}}
Async Pipe Example with Observables
The following example creates an observable. It returns 3000 after an delay. We use here the async pipe like below.
export class AppComponent implements OnInit {
constructor() { }
ngOnInit() {
}
obsValue = new Observable((observer) => {
setTimeout(() => { observer.next("I'm Observable content") }, 3000);
});
}
<p>Without use async Pipe: {{ obsValue }}</p>
<h3>Observable starts</h3>
<p>Observable Value is loading in some seconds : <span style="color: red;">{{ obsValue | async}}</span></p>
- When the components load, the angular automatically subscribes to the obsValue observable.
- The observable returns the value 3000 after a delay. When the value arrives, async pipe automatically triggers change detection. Hence you will see the return value on the screen.
- The observable is automatically unsubscribed when the component is destroyed. Thus avoiding any potential memory leaks

Use the async pipe with ngIf
The condition (obsValue | async) becomes true, when the observable returns a value. Until then the elseBlock is shown, which we use to display the loading indicator. In the example, it displays the message Observable is loading. Please wait.
When the observable returns with a value the ngIf condition becomes true and the pipe displays the returned value.
import { DecimalPipe, UpperCasePipe } from '@angular/common';
import { Component, EventEmitter, Input, OnInit, Output } from '@angular/core';
import { Observable } from 'rxjs';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
constructor(private httpclient: HttpClient) { }
ngOnInit() {
}
obsValue = new Observable((observer) => {
console.log("Observable starts");
setTimeout(() => {
observer.next("I'm Observable content");
console.log("Return the result");
}, 3000);
});
}
<div *ngIf="(obsValue | async); else elseBlock">
{{ obsValue | async}}
</div>
<ng-template #elseBlock>
Observable is loading. Please wait
</ng-template>
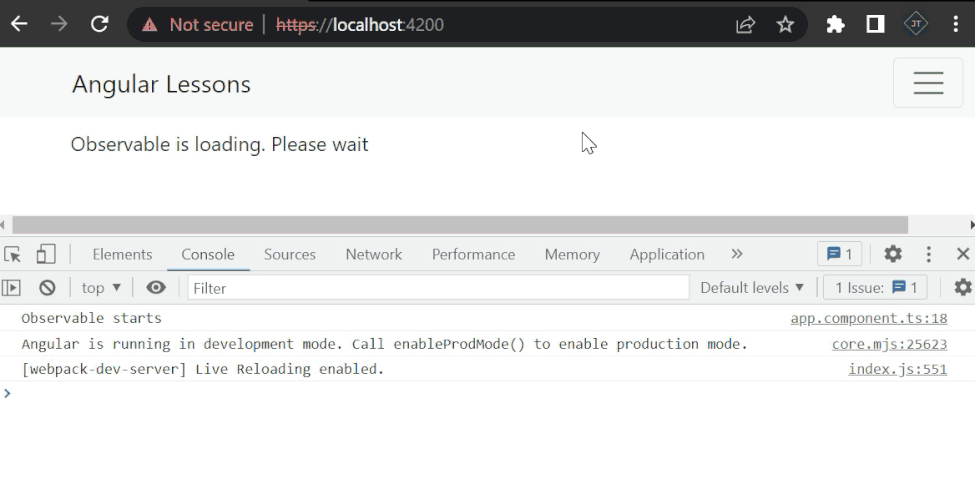
Async Pipe Example with ngFor
On the below example we will make use of httpclient library to make HTTP get request and display the results using the ngFor. Here we use the free HTTP end point https://dog.ceo/dog-api/documentation/. It returns the array of hound breeds as shown below (in the message array).
{"message":["afghan","basset","blood","english","ibizan","plott","walker"],"status":"success"}
- We consumed the API with using observable and returned the result.
- In the template use the
(hounds | async)
to subscribe to thehounds
observable. We are using a safe navigation operator?
before the property namemessage
. i.e because initially, it is null until the result arrives and without?
you will see errors in your console.
import { DecimalPipe, UpperCasePipe } from '@angular/common';
import { Component, EventEmitter, Input, OnInit, Output } from '@angular/core';
import { Observable } from 'rxjs';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
constructor(private httpclient: HttpClient) { }
ngOnInit() {
}
hounds: Observable<any> = this.getHoundList();
getHoundList(): Observable<any> {
return this.httpclient.get<any>("https://dog.ceo/api/breed/hound/list")
}
}
<h5>Async Pipe Example with ngFor</h5>
<ul>
<li *ngFor="let breed of (hounds | async)?.message">{{breed}}</li>
</ul>
Also we need to add the HttpClientModule in the app.module.ts file.
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { BrowserModule } from '@angular/platform-browser';
@NgModule({
declarations: [
AppComponent,
EmployeeComponent,
CricketerListComponent,
FilteredListComponent,
ParentComponent,
ChildComponent,
HighlightDirective
],
imports: [
BrowserModule,
AppRoutingModule,
FormsModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
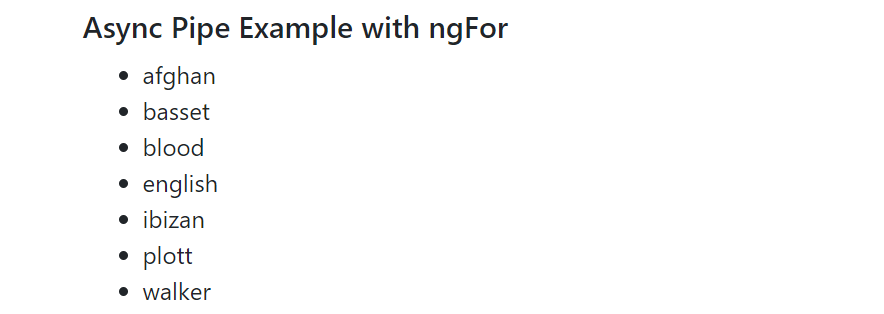
ShareReplay
We use the shareReplay
when you want subscribers to share the observable and access previously emitted values. i.e. the observable is subscribed only once and for every subsequent subscription, the previously received value is used.
obsValue = new Observable((observer) => {
console.log("Observable starts");
setTimeout(() => {
observer.next("I'm Observable content");
console.log("Return the result");
}, 3000);
}).pipe(shareReplay());
<div *ngIf="(obsValue | async); else elseBlock">
{{ obsValue | async}}
</div>
<ng-template #elseBlock>
Observable is loading. Please wait
</ng-template>
<div *ngIf="(obsValue | async);">
observable has recevied data
</div>

Conclusion
So far in this article we discussed about Async Pipe in Angular. Here we discussed why and when we can use async pipe with example of ngIf, ngFor etc..
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Related Articles
- Deploying Angular apps in Azure Blob Storage with CI/CD Integration 🚀
- How to resolve Function App Cannot Create within Azure Portal
- Building a Chatbot in Angular using Gemini API
- Create a responsive sidebar with Angular Material- Angular 18 Example Series
- How to Deploy Angular 17/18 App in GitHub Pages
- How to convert Text To Speech With Azure Cognitive Services using Angular and .Net Core
- Angular Interceptors- The Complete Guide
- Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular
- Introduction to Azure Cosmos DB
- How to create Cosmos DB in Azure
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.