In this article, we will learn about Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular. We’ll look at how to set up Azure Blob Storage and build services in ASP.NET Core Web API to upload, list, download, and delete files from Azure Blob Storage. To accomplish these activities from the client side, we will develop an Angular application. Please read my previous article on How to upload files to Azure Blob Storage using Asp.Net Core Web API.
Please find the source code on this GitHub link.
Setting up Azure Blob Storage
Let us first create a basic Azure Blob Storage resource on the Azure interface to which we will upload our files.
Creating an Azure storage account
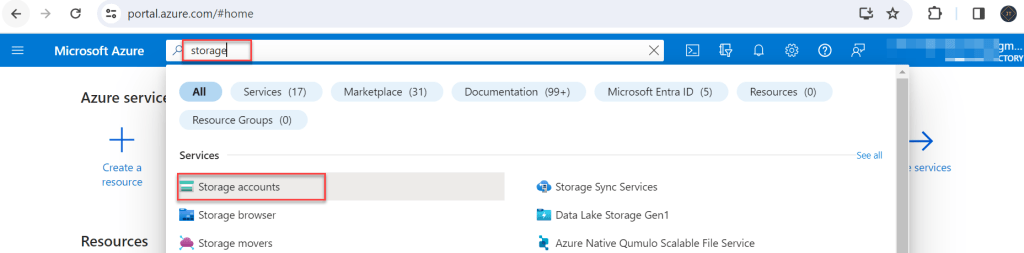
- Go to the Azure portal (portal.azure.com) and log in with your account.
- Search for “Storage account”. Click on the “Storage account” option, and then click the “Create” button.
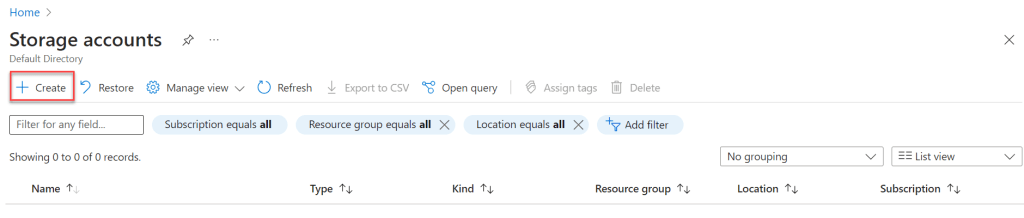
- Click on the Create button to create the storage accounts.
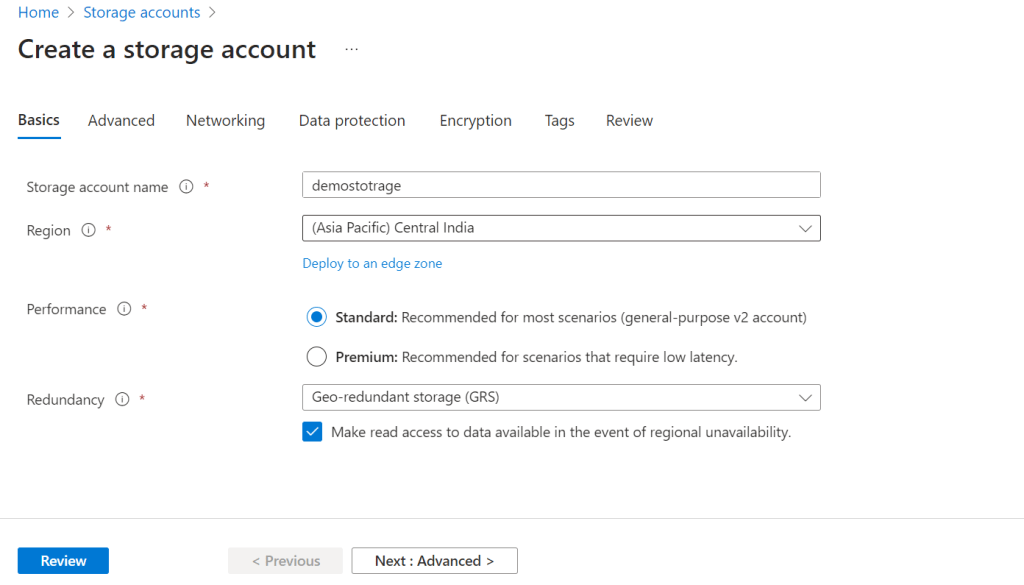
- Set the Storage account name and set the Region according to your convenient location.
- Click Review and then Create the Storage accounts.
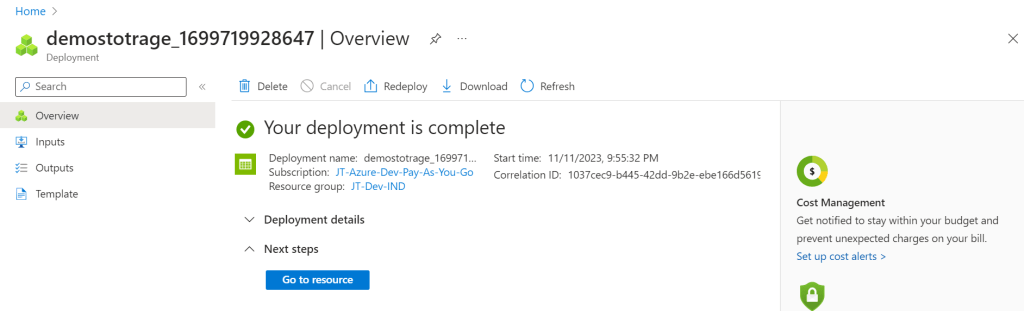
- Here we can see the deployment is complete, Go to Resource to access the Demo Storage.
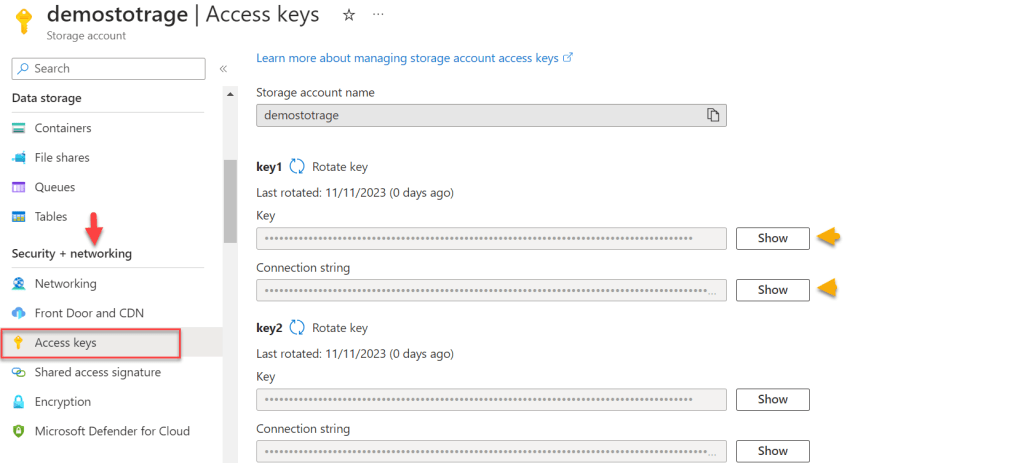
- On the Storage account, Go to
Security + Networking
and click on Access Keys. - Here we can get the Key and Connection String that need to be used in our .Net project, we can see the key and connection string values by clicking on the
Show
button.
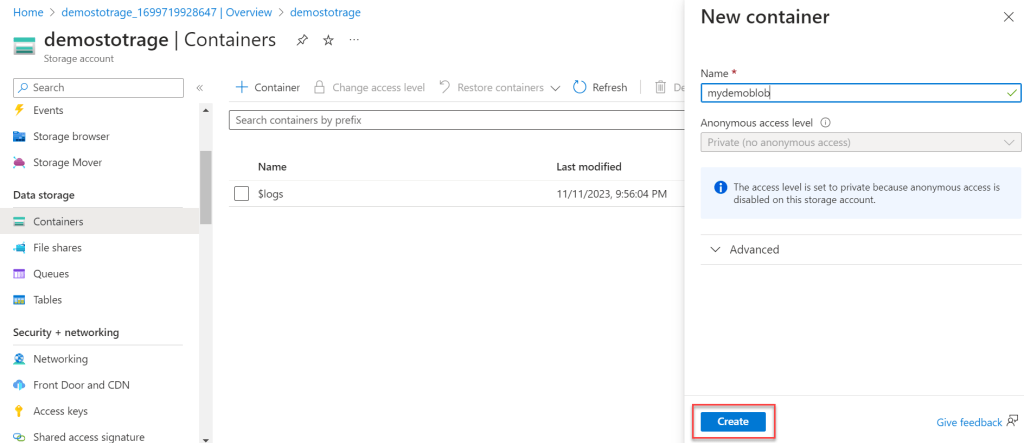
- Click on the Containers left menu, create on the Container button, and create the container where the image would be stored.
Setting up ASP.Net Core Web API Project
- Launch the Visual Studio IDE and click on “Create new project”.
- In the “Create new project” window, select “ASP.NET Core Web API” from the template list.
- Click Next. In the “Configure your new project” window, specify the name and location for the new project and then click Create.
- In the “Create New ASP.NET Core Web API” window shown next, select .NET Core as the runtime and .NET 7.0 from the drop-down list at the top. Select “API” as the project template to create a new ASP.NET Core API application.
- Ensure that Authentication is set as “No Authentication” as we won’t be using authentication either and Click Create.
Add the below Nuget Package
Install-Package Azure.Storage.Blobs
Install-Package Swashbuckle.AspNetCore
Install-Package WindowsAzure.Storage
Add the Azure Blob Storage info in appsettings.json
- Add the Azure Blob Storage info in appsettings.json and add the connection string and Key name like below.
- Connection String and Key name you can find from the Azure Storage Account that we mentioned earlier.
"BlobStorgaeInfo": {
"ConnectionStrings": "****************************",
"StorageName": "****************************"
}
Create the Azure Blob Service to handle file uploads
public class AzureBlobService
{
BlobServiceClient _blobClient;
private readonly IConfiguration _configuration;
BlobContainerClient _containerClient;
public AzureBlobService(IConfiguration Configuration)
{
_configuration = Configuration;
_blobClient = new BlobServiceClient(_configuration.GetSection("BlobStorgaeInfo:ConnectionStrings").Value.ToString());
_containerClient = _blobClient.GetBlobContainerClient(_configuration.GetSection("BlobStorgaeInfo:StorageName").Value.ToString());
}
public async Task<List<Azure.Response<BlobContentInfo>>> UploadFiles(IFormFile file)
{
var azureResponse = new List<Azure.Response<BlobContentInfo>>();
string fileName = file.FileName;
using (var memoryStream = new MemoryStream())
{
file.CopyTo(memoryStream);
memoryStream.Position = 0;
var client = await _containerClient.UploadBlobAsync(fileName, memoryStream, default);
azureResponse.Add(client);
}
return azureResponse;
}
public async Task<List<BlobItem>> GetUploadedBlobs()
{
var items = new List<BlobItem>();
var uploadedFiles = _containerClient.GetBlobsAsync();
await foreach (BlobItem file in uploadedFiles)
{
items.Add(file);
}
return items;
}
public async Task<CloudBlob> ReturnBlobsFile(string fileName)
{
if (CloudStorageAccount.TryParse(_configuration.GetSection("BlobStorgaeInfo:ConnectionStrings").Value.ToString(), out CloudStorageAccount storageAccount))
{
CloudBlobClient BlobClient = storageAccount.CreateCloudBlobClient();
CloudBlobContainer container = BlobClient.GetContainerReference(_configuration.GetSection("BlobStorgaeInfo:StorageName").Value.ToString());
if (await container.ExistsAsync())
{
CloudBlob file = container.GetBlobReference(fileName);
return file;
}
}
return null;
}
}
- Here on AzureBlobService, we used the constructor to get the Azure Blob Storage Connection String and Storage container name.
uploadfiles
is responsible for adding the file to Azure Container Service.
Creating File upload controller
namespace BlobStorageAPI.Controllers
{
[Route("api/v1/")]
[ApiController]
public class FileUploadController : ControllerBase
{
AzureBlobService _service;
public FileUploadController(AzureBlobService service)
{
_service = service;
}
[HttpPost("insertfile")]
public async Task<bool> InsertFile(IFormFile asset)
{
try
{
var response = await _service.UploadFiles(asset);
return true;
}
catch
{
return false;
}
}
[HttpGet]
[Route("listfiles")]
public async Task<IActionResult> GetBlobs()
{
var response = await _service.GetUploadedBlobs();
return Ok(response);
}
[HttpGet]
[Route("DownloadFile/{fileName}")]
public async Task<IActionResult> DownloadFile(string fileName)
{
MemoryStream ms = new MemoryStream();
var responsefile = await _service.ReturnBlobsFile(fileName);
if (await responsefile.ExistsAsync())
{
await responsefile.DownloadToStreamAsync(ms);
Stream blobStream = responsefile.OpenReadAsync().Result;
return File(blobStream, responsefile.Properties.ContentType, responsefile.Name);
}
else
{
return Content("File does not exist");
}
}
[Route("DeleteFile/{fileName}")]
[HttpGet]
public async Task<bool> DeleteFile(string fileName)
{
try
{
var responsefile = await _service.ReturnBlobsFile(fileName);
if (await responsefile.ExistsAsync())
{
await responsefile.DeleteAsync();
}
return true;
}
catch
{
return false;
}
}
}
}
- Register the dependency
AzureBlobService
on the constructor. - Add the insert files POST bypassing
IFormFile
- Delete File and Download file method is used for deleting and downloading files from blob storage.
Register the Depndancy Service in Program.cs file
builder.Services.AddSingleton<AzureBlobService>();
Setting up Angular Project
Create a new Angular application using the below CLI command
ng new NGBlobAction
We need “bootstrap”, “font-awesome”, and “file-save” libraries in this project. We can install these libraries one by one using the below command.
npm install bootstrap font-awesome --save
npm install file-saver --save
Create a new component to hold Blob Action
ng c c blob-action
blob-action.component.ts
- Here we have added to call the API by using methods ( Insertfile, Download, Delete and Show)
import { HttpClient } from '@angular/common/http';
import { Component } from '@angular/core';
@Component({
selector: 'app-blob-action',
templateUrl: './blob-action.component.html',
styleUrls: ['./blob-action.component.css']
})
export class BlobActionComponent {
constructor(private http: HttpClient) { }
files: any[] = [];
fileToUpload!: FormData;
fileUpload: any;
fileUpoadInitiated!: boolean;
fileDownloadInitiated!: boolean;
private baseUrl = 'https://localhost:7107/api/v1';
ngOnInit(): void {
this.showBlobs();
}
showBlobs() {
this.http.get<string[]>(this.baseUrl + '/listfiles').subscribe(result => {
this.files = result;
console.log(this.files)
console.log(this.files[0])
}, error => console.error(error));
}
addFile() {
if (!this.fileUpoadInitiated) {
document.getElementById('fileUpload')?.click();
}
}
handleFileInput(event: Event) {
const target = event.target as HTMLInputElement;
const files = target.files as FileList;
let formData: FormData = new FormData();
formData.append("asset", files[0], files[0].name);
this.fileToUpload = formData;
this.onUploadFiles();
}
onUploadFiles() {
if (this.fileUpoadInitiated) {
return;
}
this.fileUpoadInitiated = true;
if (this.fileToUpload == undefined) {
this.fileUpoadInitiated = false;
return false;
}
else {
return this.http.post(this.baseUrl + '/insertfile', this.fileToUpload)
.subscribe((response: any) => {
this.fileUpoadInitiated = false;
this.fileUpload = '';
if (response == true) {
this.showBlobs();
}
else {
alert('Error occured!');
this.fileUpoadInitiated = false;
}
},
err => console.log(err),
);
}
}
downloadFile(fileName: string) {
this.fileDownloadInitiated = true;
return this.http.get(this.baseUrl + '/downloadfile/' + fileName, { responseType: "blob" })
.subscribe((result: any) => {
if (result.type != 'text/plain') {
var blob = new Blob([result]);
let saveAs = require('file-saver');
let file = fileName;
saveAs(blob, file);
this.fileDownloadInitiated = false;
}
else {
this.fileDownloadInitiated = false;
alert('File not found in Blob!');
}
}
);
}
deleteFile(fileName: string) {
var del = confirm('Are you sure want to delete this file');
if (!del) return;
this.http.get(this.baseUrl + '/deletefile/' + fileName).subscribe(result => {
if (result != null) {
this.showBlobs();
}
}, error => console.error(error));
}
}
blob-action.component.html
- We used the table format to show the files uploaded using API.
- We can see the file name along with download & delete the files.
<div class="blobstorage-section">
<div class="row">
<div class="col-sm-1">
<i class="fa fa-plus fa-2x" style="cursor: pointer; color: darkslateblue;" (click)="addFile()"></i>
</div>
<div class="col-sm-11">
Click here to Add new files Store into Azure Blob
</div>
</div>
<input style="display: none;" type="file" id="fileUpload" #selectedFile [(ngModel)]="fileUpload"
(click)="selectedFile.value" value="" (change)="handleFileInput($event)" />
<table class="table table-striped mt-3h">
<tr>
<th class="column1">Uploaded Files</th>
<th class="column2" style="text-align:center;">Download</th>
<th class="column3" style="text-align:center;">Delete</th>
</tr>
<tr *ngFor="let file of files">
<td class="column1">{{ file.name }}</td>
<td class="column2" style="text-align:center;cursor: pointer;" (click)="downloadFile(file.name)">
<i class="fa fa-download"></i></td>
<td class="column3" style="text-align:center;" (click)="deleteFile(file.name)">
<i class="fa fa-trash"></i>
</td>
</tr>
</table>
</div>
blob-action.component.css
.blobstorage-section {
font-family: Calibri;
box-shadow: 0 1px 4px 0 #9b9b9b;
background-color: #ffffff;
margin: auto;
margin-top: 50px;
padding: 30px;
width: 40%;
}
.column1{
width:450px;
}
.column2{
width:200px;
}
.column3{
width:200px;
}
.blobstorage-section th {
font-family: Calibri;
font-size: 14px;
font-weight: bold;
font-style: normal;
font-stretch: normal;
line-height: 1.57;
letter-spacing: normal;
color: #333333;
border-right: 1px solid #d7d7d7;
border-bottom: 1px solid #d7d7d7;
}
.blobstorage-section th i {
font-family: Calibri;
font-size: 16px;
}
.blobstorage-section tbody tr td {
border-right: 1px solid #d7d7d7;
border-bottom: 1px solid #d7d7d7;
}
.blobstorage-section tbody tr td a {
font-family: Calibri;
font-size: 15px;
font-weight: normal;
font-style: normal;
font-stretch: normal;
line-height: 1.2;
letter-spacing: normal;
color: #0091da;
text-decoration: underline;
}
.blobstorage-section tr td img:hover {
cursor: pointer;
}
app.component.html
We need to change app.component.html
and register the blob-action
component like below.
<nav class="navbar navbar-light bg-light" style="padding-left: 20px;">
<a class="navbar-brand" href="#">
<img src="https://cdn.jsdelivr.net/gh/devicons/devicon/icons/angularjs/angularjs-original.svg" width="30" height="30" alt="">
<img src="https://cdn.jsdelivr.net/gh/devicons/devicon/icons/dotnetcore/dotnetcore-original.svg" width="30" height="30" alt="">
<span class="navbar-brand mb-0 h1"> Azure Blob Storage Action ( Upload, Download, Delete )
using Angular and ASP.Net Core
</span>
</a>
</nav>
<app-blob-action></app-blob-action>That
That’s it the change, let’s run the application using ng s --o
and we can see the output below.
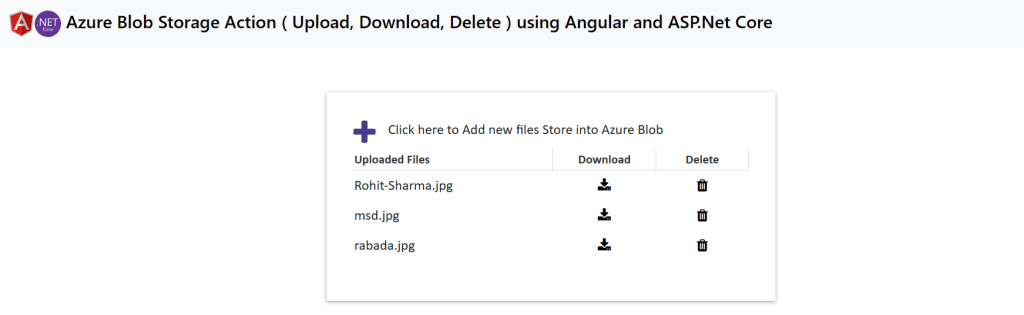
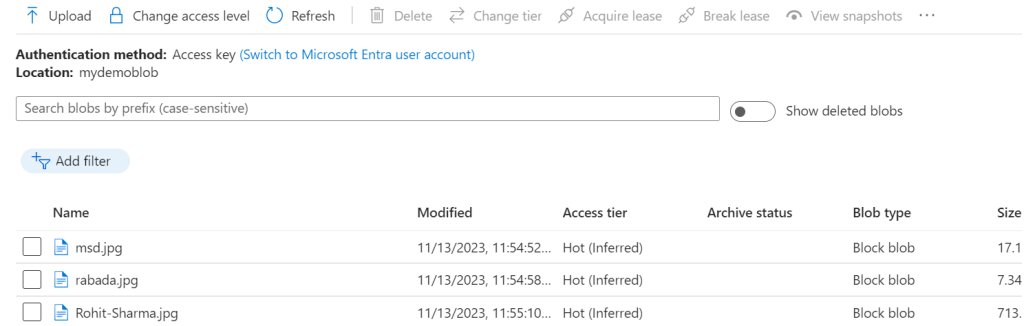
Please find the source code on this GitHub link.
Conclusion
In this article, we discussed Upload Download, and Delete files in Azure Blob Storage using ASP.NET Core and Angular. we have seen how to upload, list, download, and delete files from Azure Blob storage using the ASP.NET Core API project and we have consumed these services in an Angular client project.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Related Articles
- Building CI/CD Pipeline(YAML) using Azure DevOps – GitHub to Azure Web App (PaaS)
- Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular
- How to upload files to Azure Blob Storage using Asp.Net Core Web API
- Introduction to Azure Cosmos DB
- How to create Cosmos DB in Azure
- Logging into Azure App Service with ASP.Net Core
- Intro to Azure Logic Apps- Complete Guide
- How to use Azure Key Vault in ASP.Net Core
- How to create Azure Functions using Azure Portal
- How to Build ASP.Net Core API using Azure Cosmos DB Local Emulator
SUPPORT ME
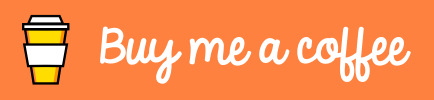