In this article we will learn about Angular KeyValue Pipe. The KeyValue Pipe converts given Object or Map into an array of key-value pairs. We can use this with the ngFor to loop through the object keys. The keyValue accepts the one argument compare function, which we can use to set the custom sort to the pipe.
Let’s look at the following files to see the different ways of using it. We can use compare function if keys are complex or we want to display in a specific order
Syntax
{{ input_expression | keyvalue [ : compareFn ] }}
Example-1
This examples show how an Object or a Map can be iterated by ngFor with the use of this keyvalue pipe.
export class AppComponent implements OnInit {
object: { [key: number]: string } = { 2: 'foo', 1: 'bar' };
map = new Map([[2, 'foo'], [1, 'bar']]);
constructor() { }
ngOnInit(): void { }
}
<span>
<p>Object</p>
<div *ngFor="let item of object | keyvalue">
{{item.key}}:{{item.value}}
</div>
<p>Map</p>
<div *ngFor="let item of map | keyvalue">
{{item.key}}:{{item.value}}
</div>
</span>
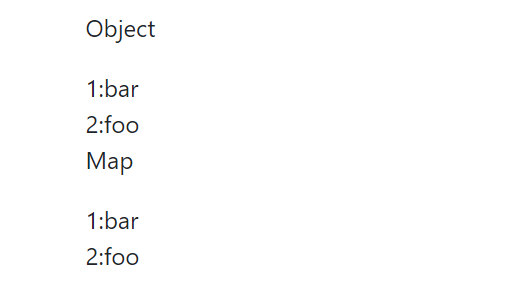
Example-2
export class AppComponent implements OnInit {
person = {firstName: 'John', lastName: 'Smith', age: 12};
persons = [{firstName: 'Ram', lastName: 'Sen', age: 35}, {firstName: 'David', lastName: 'Willey', age: 42}];
personMap = new Map([[0, this.persons[0]], [1, this.persons[1]]]);
names = {asit: 25, ramya: 45, stewart: 98, cherry: 109};
constructor() { }
ngOnInit(): void { }
orderByNames(key1: any, key2: any) {
if (key1.key < key2.key) {return -1; }
if (key1.key > key2.key) {return 1; }
return 0;
}
}
<div class="mt-2">
<h5>With Object:</h5>
<div *ngFor="let item of person | keyvalue">
{{item.key}} : {{item.value}}
</div>
</div>
<div class="mt-2">
<h5>With Map:</h5>
<div *ngFor="let item of personMap | keyvalue">
{{item.key}}:
<div *ngFor="let item of item.value | keyvalue">
{{item.key}} : {{item.value}}
</div>
</div>
</div>
<div class="mt-2">
<h5>With Compare Function:</h5>
<div *ngFor="let item of names | keyvalue:orderByNames">
{{item.key}} :: {{item.value}}
</div>
</div>
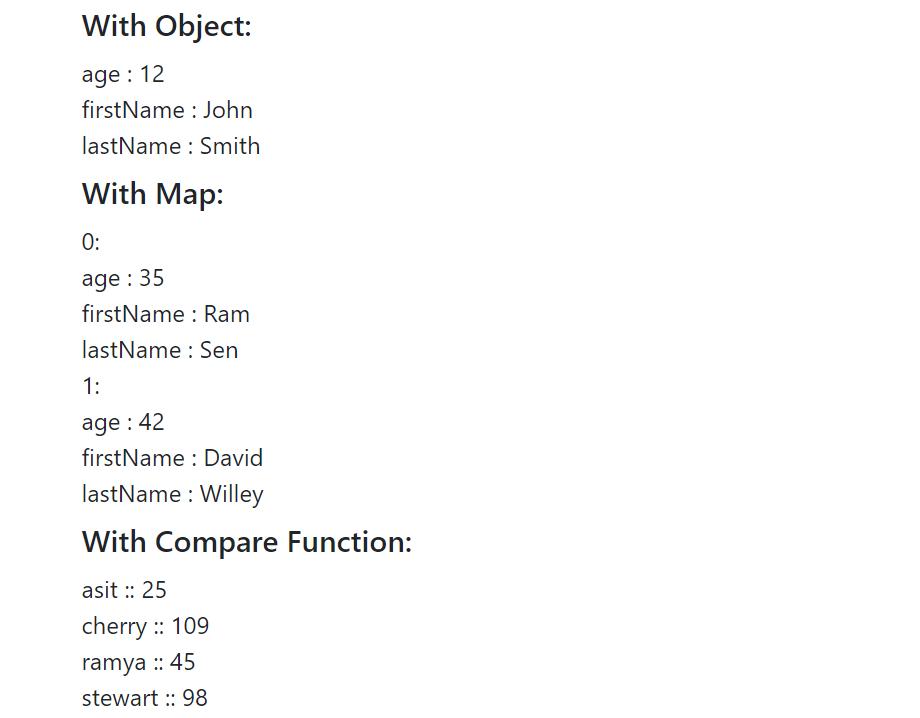
Conclusion
In this article we discussed about Angular KeyValue Pipe. The KeyValue Pipe converts given Object or Map into an array of key-value pairs. We can use this with the ngFor to loop through the object keys.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding š
Related Articles
- Angular Interceptors- The Complete Guide
- Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular
- Introduction to Azure Cosmos DB
- How to create Cosmos DB in Azure
- Why ReactJS is used over plain JavaScript
- Introduction to GraphQL
- Creating a Sample ReactJs Application using VS Code
- How to use Policy-based Authorization using JWT in .Net Core 7 and Angular
- BuildingĀ Single Page Applications(SPA) with ReactJS
- How to deploy Angular App in Azure Static Web App
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.