In this article we will learn about ngFor Directive in Angular. As you know that ngFor is the structural directive in angular, The Angular Directives are one of the most important features of Angular applications. They are used to extend the power of HTML attributes. Please read my previous article of Building Custom Directives.
Angular ngFor directive iterates over a collection of data like an array, list, etc.. and creates an HTML element for each of the items from an HTML template. It helps us to build lists or tables to display tabular data.
What is Angular ngFor Directive?
The Structural directive category includes the built-in ngFor
directive. It is used to change the structure of the DOM because it belongs to the structural directive category.
The ngFor
directive is very much similar to the “for loop” used in most of the programming languages. So, the NgFor
directive is used to iterate over a collection of data.
Syntax
<html-element *ngFor="let <item> of <items>;”>
<html-Template></html-Template>
</html-element>
<html-element>
is the element on which we apply ngFor directive. it repeats the <html-element>...</html-element>
. for each item of the collection.
*ngFor
: The syntax starts with *ngFor. The * here tells us that ngFor is an Angular structural directive.
let of <item> of <items>
item is the Template input variable. It represents the currently iterated item from the <items>. <items> is a collection, which we need to show to the user. It is usually a property on your component class and can be anything that you can iterate over.
ngFor Directive Example
Understanding Angular ngFor
Directive:
On the below example we can see how the list can iterate using HTML.
- cricketerList contains dummy data that would iterate in HTML.
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-cricketer-list',
templateUrl: './cricketer-list.component.html',
styleUrls: ['./cricketer-list.component.css']
})
export class CricketerListComponent implements OnInit {
cricketerList: Cricketer[] = [
{ id: 1, name: 'Rahuld Dravid', hundreds: 48, country: 'India' },
{ id: 2, name: 'Sachin Tendulkar', hundreds: 100, country: 'India' },
{ id: 3, name: 'Saurrav Ganguly', hundreds: 38, country: 'India' },
{ id: 4, name: 'Mahendra Singh Dhoni', hundreds: 16, country: 'India' },
{ id: 5, name: 'Virat Kohli', hundreds: 71, country: 'India' },
{ id: 6, name: 'Ricky Ponting', hundreds: 71, country: 'Australia ' },
{ id: 7, name: 'Steven Smith', hundreds: 27, country: 'Australia' },
{ id: 8, name: 'Kane Willimison', hundreds: 51, country: 'Newzealand' },
{ id: 8, name: 'Babar Azam', hundreds: 24, country: 'Pakistan' }
];
constructor() { }
ngOnInit(): void {
}
}
export class Cricketer {
id: number = 0;
name: string = '';
hundreds: number = 0;
country: string = '';
}
HTML Markup
- The highlighted line is iterate the dummy list
*ngFor="let cricketer of cricketerList;"
- And according to the details it iterates the field id,name , hundred and country etc..
ngFor
directive is used to iterate over a collection. In this example, the collection is an array of cricketers.- As the ngFor directive is a structural directive, so it is prefixed with * (star). So, the point that you need to remember is, all the structural directive are prefixed with a *.
- *ngFor=’let cricket of cricketers’ – In this statement, the ‘cricket‘ is called template input variable, which can be accessed by the element and any of its child elements.
<table class='table table-stripped'>
<thead>
<tr>
<th>No</th>
<th>Name</th>
<th>No. of Hundreds</th>
<th>Country</th>
</tr>
</thead>
<tbody>
<ng-container *ngFor="let cricketer of cricketerList;">
<tr>
<td>{{cricketer.id}}</td>
<td>{{cricketer.name}}</td>
<td>{{cricketer.hundreds}}</td>
<td>{{cricketer.country}}</td>
</tr>
</ng-container>
</tbody>
</table>
And run the application and we can see the output like below,
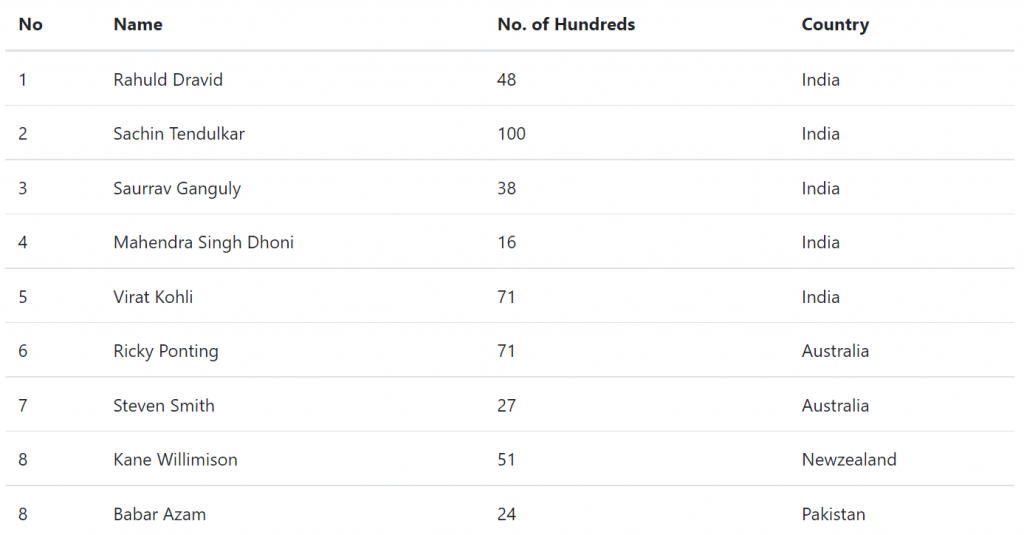
How to identify the even and odd elements in a collection in an angular application?
To identify the First and Last elements in a collection, you need to use the first and last properties of the ngFor
directive respectively. Let us understand this with an example. We want to display the student data along with whether that cricketer is the first or the last cricketer as shown in the below image.
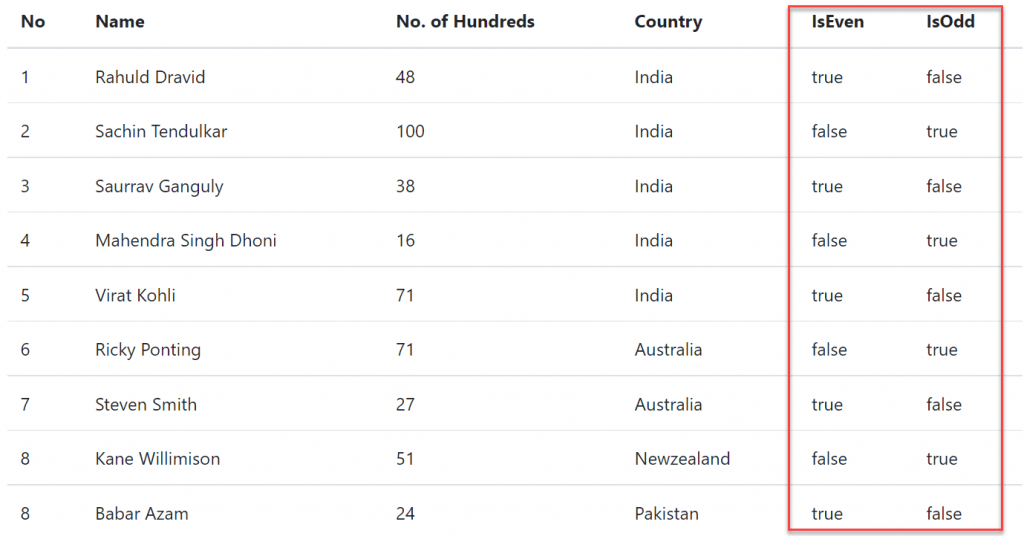
Modify the HTML mark up to implement odd & even
<table class='table table-stripped'>
<thead>
<tr>
<th>No</th>
<th>Name</th>
<th>No. of Hundreds</th>
<th>Country</th>
<th>IsEven</th>
<th>IsOdd</th>
</tr>
</thead>
<tbody>
<ng-container *ngFor="let cricketer of cricketerList;let isEven = even; let isOdd = odd">
<tr>
<td>{{cricketer.id}}</td>
<td>{{cricketer.name}}</td>
<td>{{cricketer.hundreds}}</td>
<td>{{cricketer.country}}</td>
<td>{{isEven}}</td>
<td>{{isOdd}}</td>
</tr>
</ng-container>
</tbody>
</table>
Local Variables
ngFor
exposes several values, which help us to fine-tune display. We assign these values to the local variable and use it in our template
The list of exported values provided by ngFor directive
- index: number: The zero-based index of the current element in the collection.
- count: number: The total no of items in the collection
- first: boolean: True when the item is the first item in the collection.
- last: boolean: Is set to True, when the item is the last item in the collection.
- even: boolean: True when the item has an even index in the collection.
- odd: boolean: is set to True when the item has an odd index in the collection.
How to use ‘trackBy’ with ‘ngFor’
The purpose of using the trackBy is to set the identity of elements in an iterable. If Angular sees two elements with the same identity, it will proceed to check the content of the elements and will only repaint if the content is changed. Without the identity, Angular will rely on the object reference of the elements that usually change even when the content are the same, and thus Angular will repaint the elements because of different references.
We have the existing dummy cricketer-list of count is 8, and we have added two more items into it and adding a new button and when the button is click on demand it will display the new items, so the modifies
getAllCricketers(){
this.cricketerList= [
{ id: 1, name: 'Rahuld Dravid', hundreds: 48, country: 'India' },
{ id: 2, name: 'Sachin Tendulkar', hundreds: 100, country: 'India' },
{ id: 3, name: 'Saurrav Ganguly', hundreds: 38, country: 'India' },
{ id: 4, name: 'Mahendra Singh Dhoni', hundreds: 16, country: 'India' },
{ id: 5, name: 'Virat Kohli', hundreds: 71, country: 'India' },
{ id: 6, name: 'Ricky Ponting', hundreds: 71, country: 'Australia ' },
{ id: 7, name: 'Steven Smith', hundreds: 27, country: 'Australia' },
{ id: 8, name: 'Kane Willimison', hundreds: 51, country: 'Newzealand' },
{ id: 8, name: 'Babar Azam', hundreds: 24, country: 'Pakistan' }
];
}
getNewCricketers(){
this.cricketerList.push({ id: 9, name: 'David Warner', hundreds: 30, country: 'Australia' });
this.cricketerList.push({ id: 10, name: 'Md. Rizwan', hundreds: 14, country: 'Pakistan' });
}
And on the HTML Markup add the trackBy
<table class='table table-stripped'>
<thead>
<tr>
<th>No</th>
<th>Name</th>
<th>No. of Hundreds</th>
<th>Country</th>
<th>IsEven</th>
<th>IsOdd</th>
</tr>
</thead>
<tbody>
<ng-container *ngFor="let cricketer of cricketerList;let isEven = even; let isOdd = odd; trackBy:identify;">
<tr>
<td>{{cricketer.id}}</td>
<td>{{cricketer.name}}</td>
<td>{{cricketer.hundreds}}</td>
<td>{{cricketer.country}}</td>
<td>{{isEven}}</td>
<td>{{isOdd}}</td>
</tr>
</ng-container>
</tbody>
</table>
<button (click)="getNewCricketers()">More Items</button>
Now run the application and you can see on the page load it iterates 8 cricketer-list and on button click it will add 2 more items into list.

Conclusion
In this article we discussed about ngFor Directive in Angular. We discussed here how using ngFor we can iterate amoong the list and how we can set even & odd also discussed how to use trackBy in ngFor, The Angular Directives are one of the most important features of Angular applications.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Related Articles
- Angular Interceptors- The Complete Guide
- Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular
- Introduction to Azure Cosmos DB
- How to create Cosmos DB in Azure
- Why ReactJS is used over plain JavaScript
- Introduction to GraphQL
- Creating a Sample ReactJs Application using VS Code
- How to use Policy-based Authorization using JWT in .Net Core 7 and Angular
- Building Single Page Applications(SPA) with ReactJS
- How to deploy Angular App in Azure Static Web App
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.