In this article we will learn about Angular Component Communication. We discussed how we can communicate or interact with each other among components. The component is the main building block of an Angular App. A typical Angular application consists of a lot of components. Each component handles a small part of the UI. These components must interact or communicate together to produce the complete user interface of the application. Please read my previous article Child/Nested component use in Angular.
Component Communication
There are few ways in which components can communicate or share data between them. And methods depend on whether the components have a Parent-child relationship between them are not.
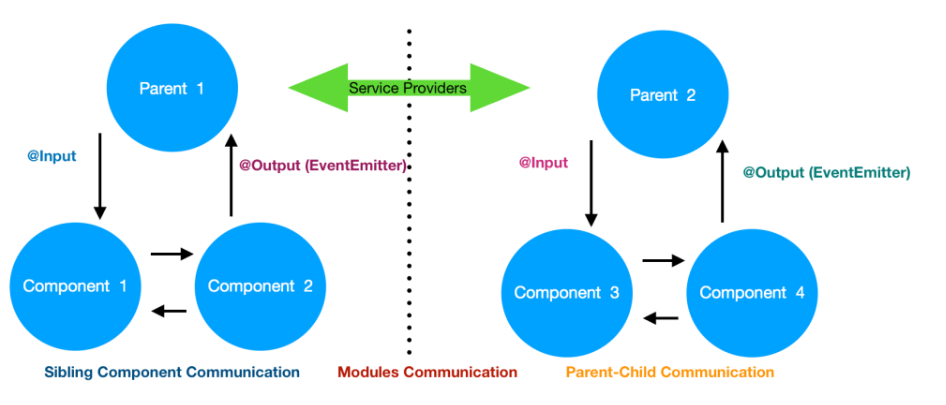
There are five ways to share data between components:
- Parent to child component
- Child to parent component
- Sharing data between sibling components
- Sharing data using ViewChild property
- Sharing data between not related components
Parent to Child Communication
If the Components have a parent-child relationship then, then the parent component can pass the data to the child using the @input
Property.
Using @Input Decorator to Pass Data
Create a property in the Child Component and decorate it with @Input()
. This will mark the property as input property.
export class ChildComponent {
@Input() SomeProperty: number;
}
And in the Parent Component Instantiate the Child Component. Pass the value to the SomeProperty using the Property Binding.
<child-component [someProperty]=value></child-component>`
Child to Parent Communication
The Child to Parent communication can happen in three ways.
- Listens to Events from Child
- Use Local Variable to access the child in the Template
- Use @ViewChild to get a reference to the child component
Listens to Child Event
This is done by the child component by exposing an EventEmitter
Property. We also decorate this Property with @Output
decorator. When Child Component needs to communicate with the parent it raises the emit event of the EventEmitter
Property. The Parent Component listens to that event and reacts to it.
Use Local Variable to access the child
Using Local Variable is to refer to the child component is another technique. For Example, Create a reference variable #child
to the Child Component.
<child-component #child></child-component>
Use @ViewChild to get the reference to the child component
<child-component></child-component>
Another way to get the reference of the child component is using the @ViewChild
query in the component class.
@ViewChild(ChildComponent) child: ChildComponent;
Sharing data between sibling components
Sharing data between siblings can be done by using points 1(Parent to child) and 2(Child to parent). First share data between the child to parent using @output
decorator and EventEmitter
. Once received data in parent component share it to another child component using @Input
decorator. So siblings can talk each other via parent components.
Sharing data using ViewChild decorator
@ViewChild
allows child component to be injected in parent component. So this make ViewChild
more powerful. It allows parents to controls the child’s methods and properties. But a parent can get access to the properties after view init
event. That means we have to implement ngAfterViewInit
life cycle hook in order to get the properties from parent components.
@ViewChild(AppChildComponent) child;
constructor() { }
ngAfterViewInit() {
this.product.productName=child.masterName; //<= This will set data
}
masterName is defined in the child component. ViewChild
gives a reference to the child component in parent components. And it sets the value of child masterName in parent productName.
Sharing data between not related components
If the Components do not share the Parent-child relationship, then the only way they can share data is by using the services and observable.
When there is no relation between the component we can not pass the data using the above four methods. This happens when your components are in different modules. There are other scenarios when you have list of products and click on a particular product and then redirect to product details components. In these kinds of scenarios, we have to use data service to share data between components.
The advantageous of using service is that
- We can share data between multiple components.
- Using observable, you can notify each component, when the data changes.
Conclusion
Here we discussed about Angular Component Communication. We discussed how we can communicate or interact with each other among components. The component is the main building block of an Angular App. A typical Angular application consists of a lot of components.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Related Articles
- Angular Interceptors- The Complete Guide
- Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular
- Introduction to Azure Cosmos DB
- How to create Cosmos DB in Azure
- Why ReactJS is used over plain JavaScript
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.