These questions are guidelines to assess the candidate about ASP.Net Core Interview Questions. These interview question covers basic to advance and will help you to prepare for the interviews, quick revision and provide strength to your technical skills.
Here we discuss the most asked interview questions of
- .NET Core Basics
- ASP.NET Core MVC
- Authentication & Authorization
Basics of .NET Core
What is .NET Core?
.NET Core is a newer version of .NET, which is cross-platform, supporting Windows, MacOS and Linux, and can be used in device, cloud, and embedded/IoT scenarios.
The following characteristics best define .NET Core:
- Flexible deployment: Can be included in your app or installed side-by-side user or machine-wide.
- Cross-platform: Runs on Windows, MacOS and Linux; can be ported to other OSes. The supported Operating Systems (OS), CPUs and application scenarios will grow over time, provided by Microsoft, other companies, and individuals.
- Command-line tools: All product scenarios can be exercised at the command-line.
- Compatible: .NET Core is compatible with .NET Framework, Xamarin and Mono, via the .NET Standard Library.
What are the differences between .NET Core and .NET Framework?Â
.NET Core | .NET Framework |
Completely open-source. | Few components are open-source. |
Compatible with Linux, Windows, and Mac operating systems. | Compatible with only Windows. |
Does not support desktop application development. | Supports web and desktop application development. |
Supports microservices development. | Does not support microservices development. |
Lightweight for Command Line Interface(CLI). | Heavy for Command Line Interface. |
What is ASP.NET Core?
ASP.NET Core is an open-source, cross-platform and high performance platform that allows you to build modern, Internet-connected and cloud enabled applications. It is much faster, configurable, modular, scalable, extensible, and has cross-platform support. It can work with both .NET Core and .Net framework via the .NET standard framework. It is best suitable for developing cloud-based such as web applications, mobile applications, and IoT applications.
With ASP.NET Core you can
- Build web applications, IoT (Internet of things) apps, services and mobile Backends.
- Run on .Net Core.
- You can do your development on Linux, Windows and MacOS.
- Deploy your code to cloud or on-premises.
What are the features provided by ASP.NET Core?
Following are the core features that are provided by the ASP.NET Core
- Built-in supports for Dependency Injection
- Built-in supports for the logging framework and it can be extensible
- Introduced a new, fast and cross-platform web server – Kestrel. So, a web application can run without IIS, Apache, and Nginx.
- Multiple hosting ways are supported
- It supports modularity, so the developer needs to include the module required by the application. However, the .NET Core framework is also providing the meta package that includes the libraries
- Command-line supports to creating, building, and running of the application
- There is no
web.config
file. We can store the custom configuration into anappsettings.json
file - There is no
Global.asax
file. We can now register and use the services in the startup class - It has good support for asynchronous programming
- Support
WebSocket
andSignalR
- Provide protection against CSRF (Cross-Site Request Forgery)
Describe the Servers in ASP.NET Core.
Server is required to run any application. ASP.NET Core provides an in-process HTTP server implementation to run the app. This server implementation listen for HTTP requests and surface them to the application as a set of request features composed into an HttpContext.
ASP.NET Core use the Kestrel web server by default. ASP.NET Core comes with:
- Default Kestrel web server that’s cross platform HTTP server implementation.
- IIS HTTP Server that’s in-process server for IIS.
- HTTP.sys server that’s a Windows-only HTTP server and it’s based on the HTTP.sys kernel driver and HTTP Server API.
What is Kestrel?
Kestrel is an event-driven, I/O-based, open-source, cross-platform, and asynchronous server which hosts .NET applications. It is provided as a default server for .NET Core therefore, it is compatible with all the platforms and their versions which .NET Core supports.

It is really fast, secure and good enough to use it without a reverse proxy server. However, it is still recommended that you use IIS, Nginx or Apache or something else.
Usually, it is used as an edge-server, which means it is the server which faces the internet and handles HTTP web requests from clients directly. It is a listening server with a command-line interface.
Advantages of Kestrel are:
- Lightweight and fast.
- Cross-platform and supports all versions of .NET Core.
- Supports HTTPS
- Easy configuration
What is WebListener?
ASP.NET Core ships two server implementations Kestral and WebListener. WebListener
is also a web server for ASP.NET Core that runs only on Windows. It’s built on the Http.Sys
kernel mode driver. WebListener
is an alternative to Kestrel that can be used for direct connection to the Internet without relying on IIS as a reverse proxy server.
What is ASP.NET Core Module (ANCM)?
ASP.NET Core Module (ANCM) lets you run ASP.NET Core applications behind IIS and it works only with Kestrel. it isn’t compatible with WebListener. ANCM is a native IIS module that hooks into the IIS pipeline and redirects traffic to the backend ASP.NET Core application. ASP.NET Core applications run in a process separate from the IIS worker process, ANCM also does process management.
ANCM starts the process for the ASP.NET Core application when the first request comes in and restarts it when it crashes. In short, it sits in IIS and routes the request for ASP.NET Core application to Kestral.
Can ASP.NET Core application work with full .NET 4.x Framework?
Yes, ASP.NET core application works with full .NET framework via the .NET standard library.
What is the startup class in ASP.NET core?
The startup class is the entry point of the ASP.NET Core application. Every .NET Core application must have this class. This class contains the application configuration related items. It is not necessary that the class name must be “Startup”, it can be anything, we can configure the startup class in the Program class.
public class Program { public static void Main(string[] args) { CreateWebHostBuilder(args).Build().Run(); } public static IWebHostBuilder CreateWebHostBuilder(string[] args) => WebHost.CreateDefaultBuilder(args) .UseStartup<TestClass>(); }
What is a Host and what’s the importance of Host in ASP.NET Core application?
ASP.NET Core apps require a host in which to execute. The host is responsible for application startup and lifetime management. Other responsibility of host’s includes ensuring the application’s services and the server are available and properly configured. The host is responsible for starting the app and its management, where the server is responsible for accepting HTTP requests. The host is configured to use a particular server; the server is unaware of its host. The host is typically created using an instance of a WebHostBuilder
, which builds and returns a WebHost
instance. The WebHost
references the server that will handle requests.
What does WebHost.CreateDefaultBuilder() do?
WebHost.CreateDefaultBuilder() configure the app to use Kestrel as web server. It Specify to use the current project directory as root directory for the application. Setup the configuration sub-system to read setting from appsettings.json and appsettings.{env}.json to environment specific configuration.
Configure logging to read from the Logging section of the appsettings.json file and log to the Console and Debug window. It can configure integration with IIS
What is the role of Startup class?
Startup class is responsible for configuration related things as below.
- It configures the services which are required by the app.
- It defines the app’s request handling pipeline as a series of middleware components.
Startup class is specified inside the CreateHostBuilder
method when the host is created.
Multiple Startup classes can also be defined for different environments, At run time appropriate startup classes are used.
// Startup class example public class Startup { public Startup(IConfiguration configuration) { Configuration = configuration; } public IConfiguration Configuration { get; } public void ConfigureServices(IServiceCollection services) { services.AddRazorPages(); } public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } else { app.UseExceptionHandler("/Error"); app.UseHsts(); } app.UseHttpsRedirection(); // other middleware components } }
What is the role of ConfigureServices and Configure method?
ConfigureServices method is optional and defined inside startup class as mentioned in above code. It gets called by the host before the ‘Configure’ method to configure the app’s services. The Configure
 method is used to specify how the ASP.NET application will respond to HTTP requests. The request pipeline is configured by adding middleware components to an IApplicationBuilder
instance that is provided by dependency injection. There are some built-in middlewares
for error handling, authentication, routing, session and diagnostic purpose. Highlighted lines in below code, are built-in Middleware with ASP.NET Core 1.0.
You can configure the services and middleware components without the Startup class and it’s methods, by defining this configuration inside the Program class in CreateHostBuilder
method.
Where to keep configuration information in ASP.NET Core.
In ASP.NET Core web.config
is not available. So here we have to store configuration information in appsetting.json file, which is a plain text file, it keeps information as key-value pair in JSON format.
{ "Logging": { "IncludeScopes": false, "LogLevel": { "Default": "Warning" } }, "Data": { "ConnectionString": "Data Source=KIITs\\SQLEXPRESS;Initial Catalog=testdb;Integrated Security=True" } }
What is the role of IHostingEnvironment interface in ASP.NET Core?
ASP.NET Core offers an interface named IHostingEnvironment
, allows you to programmatically retrieve the current environment so you can have an environment-specific behavior. By default, ASP.NET Core has 3 environments Development, Staging, and Production. Previously, the developers have to build the application differently for each environment (Staging, UAT, Production) due to dependency on config file sections and the preprocessor directive applicable at compile time. ASP.NET Core takes a different approach and uses IHostingEnvironment
to retrieve the current environment.
What is middleware?
It is software that is injected into the application pipeline to handle requests and responses. They are just like chained to each other and form as a pipeline. The incoming requests are passed through this pipeline where all middleware is configured, and middleware can perform some action on the request before passing it to the next middleware. Same as for the responses, they are also passing through the middleware but in reverse order.
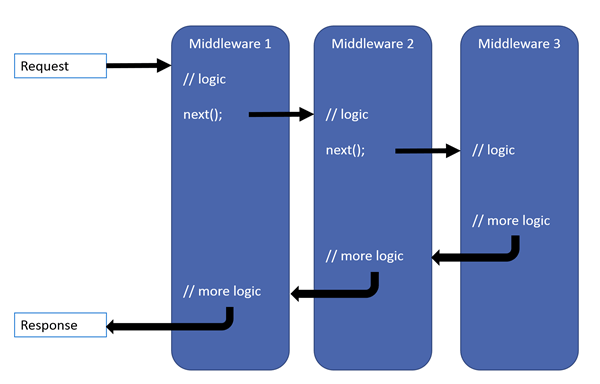
The Request handling pipeline is a sequence of middleware components where each component performs the operation on request and either call the next middleware component or terminate the request. When a middleware component terminates the request, it’s called Terminal Middleware as It prevents next middleware from processing the request. You can add a middleware component to the pipeline by calling .Use… extension method as below.
app.UseHttpsRedirection(); app.UseStaticFiles(); app.UseRouting();
Describe the URL Rewriting Middleware in ASP.NET Core
URL rewriting is the act of modifying request URLs based on one or more predefined rules. URL rewriting creates an abstraction between resource locations and their addresses so that the locations and addresses aren’t tightly linked
URL rewriting is valuable in several scenarios to:
- Move or replace server resources temporarily or permanently and maintain stable locators for those resources.
- Split request processing across different apps or across areas of one app.
- Remove, add, or reorganize URL segments on incoming requests.
- Optimize public URLs for Search Engine Optimization (SEO).
- Permit the use of friendly public URLs to help visitors predict the content returned by requesting a resource.
- Redirect insecure requests to secure endpoints.
- Prevent hotlinking, where an external site uses a hosted static asset on another site by linking the asset into its own content.
What is Request delegate?
Request delegates handle each HTTP request and are used to build request pipeline. It can configured using Run, Map and Use extension methods. An request delegate can be a in-line as an anonymous method (called in-line middleware) or a reusable class. These classes or in-line methods are called middleware components.
What is the difference between IApplicationBuilder.Use() and IApplicationBuilder.Run()?
We can use both the methods in Configure methods of the startup class. Both are used to add middleware delegates to the application request pipeline. The middleware adds using IApplicationBuilder.Use may call the next middleware in the pipeline whereas the middleware adds using IApplicationBuilder.The run method never calls the subsequent middleware. After IApplicationBuilder.Run method, system stop adding middleware in the request pipeline.
What is the use of the “Map” extension while adding middleware to the ASP.NET Core pipeline?
It is used for branching the pipeline. It branches the ASP.NET Core pipeline based on request path matching. If the request path starts with the given path, middleware on to that branch will execute.
public void Configure(IApplicationBuilder app) { app.Map("/path1", Middleware1); app.Map("/path2", Middleware2); }
How to enable Session in ASP.NET Core?
The middleware for the session is provided by the package Microsoft.AspNetCore.Session
. To use the session in the ASP.NET Core application, we need to add this package to csproj
file and add the Session middleware to the ASP.NET Core request pipeline.
public class Startup { public void ConfigureServices(IServiceCollection services) { …. …. services.AddSession(); services.AddMvc(); } public void Configure(IApplicationBuilder app, IHostingEnvironment env) { …. …. app.UseSession(); …. …. } }
What is Dependency Injection?
Dependency Injection is the design pattern that help us to create application which loosely coupled. This means that object should only have those dependency that required during complete task. The main advantages of DI (Dependency Injection) is our application loosely coupled and has provide greater maintainability, testability and also re-usability. It is loosely coupled because dependency required by the class are injected from outer world rather than created them self directly win-in code.
Service Lifetime of Dependency Injection?
When Services are registered, there is a lifetime for every service. ASP.NET Core provides the following lifetimes.
- Transient – Services with transient lifetime are created each time they are requested from service container. So it’s best suited for stateless, light weight services.
services.AddTransient<IHelloWorldService, HelloWorldService>();
- Scoped – Services with scoped lifetime are created once per connection or client request. When using scoped service in middleware then inject the service via invoke or invokeAsync method. You should not inject the service via constructor injection as it treats the service behavior like Singleton.
services.AddScoped<IHelloWorldService, HelloWorldService>();
- Singleton – Service with singleton lifetime is created once when first time the service is requested. For subsequent requests same instance is served by service container.
services.AddSingleton<IHelloWorldService, HelloWorldService>();
How ASP.NET Core supports dependency injection into views?
ASP.NET Core supports dependency injection into views. This can be useful for view-specific services, such as localization or data required only for populating view elements. You should try to maintain separation of concerns between your controllers and views. Most of the data your views display should be passed in from the controller.
@using Microsoft.Extensions.Configuration @inject IConfiguration Configuration @{ string myValue = Configuration["root:parent:child"]; ... }
What is Host in ASP.NET Core?
Host encapsulates all the resources for the app. On startup, ASP.NET Core application creates the host. The Resources which are encapsulated by the host include:
- HTTP Server implementation
- Dependency Injection
- Configuration
- Logging
- Middleware components
Describe the Generic Host and Web Host.
The host setup the server, request pipeline and responsible for app startup and lifetime management. There are two hosts: .NET Generic Host
& ASP.NET Core Web Host
.NET Generic Host is recommended and ASP.NET Core template builds a .NET Generic Host on app startup.
ASP.NET Core Web host is only used for backwards compatibility.
How Configuration works in ASP.NET Core?
In ASP.NET Core, Configuration is implemented using various configuration providers. Configuration data is present in the form of key value pairs that can be read by configuration providers as key value from different configuration sources as below.
- appsettings.json – settings file
- Azure Key Vault
- Environment variables
- In-memory .Net objects
- Command Line Arguments
- Custom Providers
What is routing in ASP.NET Core?
Routing is functionality that map incoming request to the route handler. The route can have values (extract them from the URL) that are used to process the request. Using the route, routing can find a route handler based on the URL. All the routes are registered when the application is started. There are two types of routing supported by ASP.NET Core
- The conventional routing
- Attribute routing
The Routing uses routes to map incoming requests with the route handler and Generates URL that is used in response. Mostly, the application has a single collection of routes and this collection is used for the process of the request. The RouteAsync method is used to map incoming requests (that match the URL) with available in route collection.
app.UseRouting(); // It adds route matching to middlware pipeline // It adds endpoints execution to middleware pipeline app.UseEndpoints(endpoints => { endpoints.MapGet("/", async context => { await context.Response.WriteAsync("Hello World!"); }); });
Describe Attribute based routing
Attribute Routing gives you more control over the URIs in your web application. MVC 5 supports this attribute based routing where attributes are used to define the routes. You can manage resource hierarchies in better way using attribute based routing. Attribute based routing is used to create routes which are difficult to create using convention-based routing. For example below routes.
[Route("customers/{customerId}/orders")] public IEnumerable GetOrdersByCustomer(int customerId) { ... } . . . [Route("customers/{customerId}/orders/orderId")] public IEnumerable GetOrdersByCustomer(int customerId, int orderId) { ... }
How to handle errors in ASP.NET Core?
ASP.NET Core provides a better way to handle the errors in Startup class as below.
if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } else { app.UseExceptionHandler("/Error"); app.UseHsts(); }
For development environment, Developer exception page display detailed information about the exception. You should place this middleware before other middleware for which you want to catch exceptions. For other environments UseExceptionHandler
middleware loads the proper Error page.
You can configure error code specific pages in Startup class Configure
method as below.
app.Use(async (context, next) => { await next(); if (context.Response.StatusCode == 404) { context.Request.Path = "/not-found"; await next(); } if (context.Response.StatusCode == 403 || context.Response.StatusCode == 503 || context.Response.StatusCode == 500) { context.Request.Path = "/Home/Error"; await next(); } });
How ASP.NET Core serve static files?
In ASP.NET Core, Static files such as CSS, images, JavaScript files, HTML are the served directly to the clients. ASP.NET Core template provides a root folder called wwwroot
which contains all these static files. UseStaticFiles()
method inside Startup.Configure
enables the static files to be served to client.
Explain Session and State management in ASP.NET Core
HTTP is a stateless protocol. HTTP requests are independent and does not retain user values. There are different ways to maintain user state between multiple HTTP requests.
- Cookies
- Session State
- TempData
- Query strings
- Hidden fields
- HttpContext.Items
- Cache
What are the various JSON files available in ASP.NET Core?
There are the following JSON files in ASP.NET Core :
- global.json
- launchsettings.json
- appsettings.json
- bundleconfig.json
- bower.json
- package.json
What is tag helper in ASP.NET Core?
It is a feature provided by the Razor view engine that enables us to write server-side code to create and render the HTML element in view (Razor). The tag-helper is a C# class that is used to generate the view by adding the HTML element. The functionality of the tag helper is very similar to the HTML helper of ASP.NET MVC.
//HTML Helper @Html.TextBoxFor(model => model.FirstName, new { @class = "form-control", placeholder = "Enter Your First Name" }) //content with tag helper <input asp-for="FirstName" placeholder="Enter Your First Name" class="form-control" /> //Equivalent HTML <input placeholder="Enter Your First Name" class="form-control" id="FirstName" name="FirstName" value="" type="text">
How to disable Tag Helper at the element level?
We can disable Tag Helper at the element level using the opt-out character (“!”). This character must apply to open and close the Html tag.
<!span asp-validation-for="phone" class="divPhone"></!span>
What are Razor Pages in ASP.NET Core?
This is a new feature introduced in ASP.NET Core 2.0. It follows a page-centric development model just like ASP.NET web forms. It supports all the features of ASP.NET Core.
@page <h1> Hello, Book Reader!</h1> <h2> This is Razor Pages </h2>
The Razor pages start with the @page directive. This directive handle request directly without passing through the controller. The Razor pages may have code behind files, but it is not really a code-behind file. It is a class inherited from PageModel class.
How can we do the automatic model binding in Razor pages?
The Razor pages provide the option to bind property automatically when posting the data using the Bind Property attribute. By default, it only binds the properties only with non-GET verbs. we need to set Supports Get property to true to bind a property on getting a request.
public class Test1Model : PageModel { [BindProperty] public string Name { get; set; } }
How can we inject the service dependency into the controller?
There are three easy steps to add a custom service as a dependency on the controller.
Step 1: Create the service
public interface IHelloWorldService { string SaysHello(); } public class HelloWorldService: IHelloWorldService { public string SaysHello() { return "Hello "; } }
Step 2: Add this service to the Service container (service can either be added by singleton, transient, or scoped)
public void ConfigureServices(IServiceCollection services) { …. … services.AddTransient<IHelloWorldService, HelloWorldService>(); … … }
Step 3: Use this service as a dependency in the controller
public class HomeController: Controller { IHelloWorldService _helloWorldService; public HomeController(IHelloWorldService helloWorldService) { _helloWorldService = helloWorldService; } }
What are the different ways for bundling and minification in ASP.NET Core?
Ans: There are different ways for doing bundling and minification in ASP.NET Core.
- Gulp – was the default choice for ASP.NET Core till beta versions. Later it was removed due to performance and speed issue and replaced with BundlerMinifier.
- BundlerMinifier – is a Visual Studio extension and it’s default choice now. You should see bundleconfig.json file in your default template.
- ASP.NET Core Web Optimizer – ASP.NET Core middleware for bundling and minification of CSS and JavaScript files at runtime.
- Grunt – can also be used with ASP.NET Core.
Explain Model Binding in ASP.NET Core
Controllers and Razor pages work with data that comes from HTTP requests. For example, route data may provide a record key, and posted form fields may provide values for the properties of the model. Writing code to retrieve each of these values and convert them from strings to .NET types would be tedious and error-prone. Model binding automates this process. The model binding system:
- Retrieves data from various sources such as route data, form fields, and query strings.
- Provides the data to controllers and Razor pages in method parameters and public properties.
- Converts string data to .NET types.
- Updates properties of complex types.
Model binding for HTTP GET requests
By default, properties are not bound for HTTP GET requests. Typically, all you need for a GET request is a record ID parameter. The record ID is used to look up the item in the database. Therefore, there is no need to bind a property that holds an instance of the model. In scenarios where you do want properties bound to data from GET requests, set the SupportsGet
property to true
:
[BindProperty(Name = "ai_user", SupportsGet = true)] public string? ApplicationInsightsCookie { get; set; }
How to access HttpContext in ASP.NET Core?
ASP.NET Core apps access HttpContext through the IHttpContextAccessor interface and its default implementation HttpContextAccessor. It’s only necessary to use IHttpContextAccessor when you need access to the HttpContext inside a service.
Use HttpContext from Razor Pages
The Razor Pages PageModel exposes the PageModel.HttpContext property:
public class IndexModel : PageModel { public void OnGet() { var message = HttpContext.Request.PathBase; // ... } }
The same property can be used in the corresponding Razor Page View:
@page @model IndexModel @{ var message = HttpContext.Request.PathBase; // ... }
Explain the Caching or Response caching in ASP.NET Core
Caching significantly improves the performance of an application by reducing the number of calls to actual data source. It also improves the scalability. Response caching is best suited for data that changes infrequently. Caching makes the copy of data and store it instead of generating data from original source.
Response caching headers control the response caching. ResponseCache
attribute sets these caching headers with additional properties.
What is In-memory cache?
In-memory cache is the simplest way of caching by ASP.NET Core that stores the data in memory on web server.
Apps running on multiple server should ensure that sessions are sticky if they are using in-memory cache. Sticky Sessions responsible to redirect subsequent client requests to same server. In-memory cache can store any object but distributed cache only stores byte[].
IMemoryCache
interface instance in the constructor enables the In-memory caching service via ASP.NET Core dependency Injection.
What is Distributed caching?
Applications running on multiple servers (Web Farm) should ensure that sessions are sticky. For Non-sticky sessions, cache consistency problems can occur. Distributed caching is implemented to avoid cache consistency issues. It offloads the memory to an external process. Distributed caching has certain advantages as below.
- Data is consistent across client requests to multiple server
- Data keeps alive during server restarts and deployments.
- Data does not use local memory
IDistributedCache
interface instance from any constructor enable distributed caching service via Dependency Injection.
What is XSRF or CSRF? How to prevent Cross-Site Request Forgery (XSRF/CSRF) attacks in ASP.NET Core?
Cross-Site Request Forgery (XSRF/CSRF)Â is an attack where attacker that acts as a trusted source send some data to a website and perform some action. An attacker is considered a trusted source because it uses the authenticated cookie information stored in browser.
For example a user visits some site ‘www.abc.com’ then browser performs authentication successfully and stores the user information in cookie and perform some actions, In between user visits some other malicious site ‘www.bad-user.com’ and this site contains some code to make a request to vulnerable site (www.abc.com). It’s called cross site part of CSRF.
How to prevent CSRF?
- In ASP.NET Core 2.0 or later FormTaghelper automatically inject the antiforgery tokens into HTML form element.
- You can add manually antiforgery token in HTML forms by using
@Html.AntiForgeryToken()
and then you can validate it in controller byValidateAntiForgeryToken()
method.
How to prevent Cross-Site Scripting (XSS) in ASP.NET Core?
How to enable Cross-Origin Requests (CORS) in ASP.NET Core?
Explain the Filters.
Filters provide the capability to run the code before or after the specific stage in request processing pipeline, it could be either MVC app or Web API service. Filters performs the tasks like Authorization, Caching implementation, Exception handling etc. ASP.NET Core also provide the option to create custom filters. There are 5 types of filters supported in ASP.NET Core Web apps or services.
- Authorization filters run before all or first and determine the user is authorized or not.
- Resource filters are executed after authorization.
OnResourceExecuting
filter runs the code before rest of filter pipeline andOnResourceExecuted
runs the code after rest of filter pipeline. - Action filters run the code immediately before and after the action method execution. Action filters can change the arguments passed to method and can change returned result.
- Exception filters used to handle the exceptions globally before wrting the response body
- Result filters allow to run the code just before or after successful execution of action results.
What is View components in ASP.NET Core
ViewComponent was introduced in ASP.NET Core MVC. It can do everything that a partial view can and can do even more. ViewComponents are completely self-contained objects that consistently render html from a razor view.
ViewComponents are very powerful UI building blocks of the areas of application which are not directly accessible for controller actions. Let’s suppose we have a page of social icons and we display icons dynamically. We have separate settings for color, urls and names of social icons and we have to render icons dynamically. For more details please visit this official Microsoft link.
How View compilation works in ASP.NET Core?
For both ASP.NET Core and ASP.NET Core MVC project, a Razor file having a .cshtml extension are compiled at both build and publish time. Furthermore, runtime compilation is optionally enabled, and this can entirely be enabled if you have configured your project correctly. This means you can allow runtime compilation, giving you the ability to see any modified UI changes in real-time without ever restarting your application.
You can install below package in Nuget to allow the View/Razor compilation
Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation
Then Add the below code into ConfigureServices() method to allow runtime compilation.
// This method gets called by the runtime. Use this method to add services to the container. public void ConfigureServices(IServiceCollection services) { services.AddControllersWithViews().AddRazorRuntimeCompilation(); }
Explain Buffering and Streaming approaches to upload files in ASP.NET Core
ASP.NET Core supports uploading one or more files using buffered model binding for smaller files and unbuffered streaming for larger files. Two general approaches for uploading files are buffering and streaming.
Buffering
The entire file is read into an IFormFile. IFormFile
is a C# representation of the file used to process or save the file.
The disk and memory used by file uploads depend on the number and size of concurrent file uploads. If an app attempts to buffer too many uploads, the site crashes when it runs out of memory or disk space. If the size or frequency of file uploads is exhausting app resources, use streaming.
Any single buffered file exceeding 64 KB is moved from memory to a temp file on disk.
Temporary files for larger requests are written to the location named in the ASPNETCORE_TEMP
 environment variable. If ASPNETCORE_TEMP
 is not defined, the files are written to the current user’s temporary folder.
Buffering small files is covered in the following sections of this topic:
- Physical storage
- Database
Streaming
The file is received from a multipart request and directly processed or saved by the app. Streaming doesn’t improve performance significantly. Streaming reduces the demands for memory or disk space when uploading files.
Streaming large files is covered in the Upload large files with streaming section.
For more details about the buffer & Streaming concept visit this link.
How does bundling and minification work in ASP.NET Core?
Bundling and minification are two techniques you can use in ASP.NET to improve page load performance for your web application. Bundling combines multiple files into a single file. Minification performs a variety of different code optimizations to scripts and CSS, which results in smaller payloads.
For more details visit this link.
What is the Area?
Area is used to divide large ASP.NET MVC application into multiple functional groups. In general, for a large application Models, Views and controllers are kept in separate folders to separate the functionality. But Area is a MVC structure that separate an application into multiple functional groupings. For example, for an e-commerce site Billing, Orders, search functionalities can be implemented using different areas.
ASP.NET Core MVC Interview Questions
What is ASP.NET MVC? Explain its componentsÂ
It is a lightweight and open-source web development framework, which is used to decouple data(Model), interface (View), and logic(Controller). It provides a pattern-based way to create dynamic websites and supports TDD-based development.
An MVC(Model-View-Controller) architectural pattern separates the application into three components and provides separation of concerns.
- Model: Represents the state of application/logic, where the business logic and implementation logic is encapsulated.
- View: It is responsible for providing the view through the user interface.
- Controller: Handles user interaction, works in tandem with model and view components.
It provides the latest web standards and many features like routing, model binding, model validation, dependency injection, web APIs, razor view engine, filters, etc.
What are the advantages of ASP.NET MVC?Â
- Provides full control over HTML rendering.
- Provides separation of concerns(SoC).
- Reduction of complexity by dividing the application into three components.
- Supports test-driven development(TDD).
- Easy integration with JavaScript, JSON, jQuery, etc.
- Uses the latest technology and supports the latest trends.
What is the difference between ViewData and ViewBag in ASP.NET MVC?Â
ViewData and ViewBag in ASP.NET MVC are used for transferring data from controller to view. Below are the differences between them:
ViewData | ViewBag |
It is a dictionary object of the ‘ViewDataDictionary’ class having key-value. | It is a wrapper around ViewData and is a dynamic property. |
Faster than ViewBag. | Slower than ViewData. |
Type conversion code is required. | Dynamic hence type conversion code is not required. |
Describe the request flow in the ASP.NET MVC frameworkÂ
The request flow has below stages in the MVC framework:
- Routing: It is the first step which matches the pattern of the request’s URL against the URL present in the route table.
- MvcHandler: It starts the processing of the request using the ProcessRequest method.
- Controller: Uses ‘IControllerFactory’ instance and calls the ‘Execute’ method, where ‘IControllerFactory’ is a default controller factory or a custom factory can be defined.
- Action execution: After controller instantiation, ‘ActionInvoker’ defines which action to be performed on the controller.
- View result: The ‘action’ method prepares the response and then returns a result.
- View engine: ‘IViewInterface’ of the view engine selects a view engine to render the result.
- View: ‘ViewResult’ returns and renders an HTML page on the browser.
Explain Partial Views
A partial view is a Razor markup file ( . cshtml ) without an @page directive that renders HTML output within another markup file’s rendered output. The term partial view is used when developing either an MVC app, where markup files are called views, or a Razor Pages app, where markup files are called pages
When to use partial views
Partial views are an effective way to:
- Break up large markup files into smaller components.In a large, complex markup file composed of several logical pieces, there’s an advantage to working with each piece isolated into a partial view. The code in the markup file is manageable because the markup only contains the overall page structure and references to partial views.
- Reduce the duplication of common markup content across markup files.When the same markup elements are used across markup files, a partial view removes the duplication of markup content into one partial view file. When the markup is changed in the partial view, it updates the rendered output of the markup files that use the partial view.
How routing works in MVC application?
Basically, routing is used for handling HTTP requests and searching matching action methods, and then executing the same. It constructs outgoing URLs that correspond to controller actions. Routing the map request with Controller’s Action Method.
A route is a URL pattern. Routing is a pattern matching process that monitors the requests and determines what to do with each request. In other words we can say Routing is a mechanism for mapping requests within our MVC application. The Routing mechanism passes the request to the handler. A handler may be a physical path such as .aspx or may be a class capable of processing our request such as controller in MVC.
Describe Attribute based routing
Attribute Routing gives you more control over the URIs in your web application. MVC 5 supports this attribute based routing where attributes are used to define the routes. You can manage resource hierarchies in better way using attribute based routing. Attribute based routing is used to create routes which are difficult to create using convention-based routing. For example below routes.
Route("customers/{customerId}/orders")] public IEnumerable GetOrdersByCustomer(int customerId) { ... } . . . [Route("customers/{customerId}/orders/orderId")] public IEnumerable GetOrdersByCustomer(int customerId, int orderId) { ... }
Explain dependency injection for controllers
ASP.NET Core MVC controllers request dependencies explicitly via constructors. ASP.NET Core has built-in support for dependency injection (DI). DI makes apps easier to test and maintain. Services are added as a constructor parameter, and the runtime resolves the service from the service container. Services are typically defined using interfaces.
For more details please visit this link
How ASP.NET Core supports dependency injection into views?
ASP.NET Core supports dependency injection into views. This can be useful for view-specific services, such as localization or data required only for populating view elements. You should try to maintain separation of concerns between your controllers and views. Most of the data your views display should be passed in from the controller.
@using Microsoft.Extensions.Configuration @inject IConfiguration Configuration @{ string myValue = Configuration["root:parent:child"]; ... }
What is Cache Tag Helper in ASP.NET Core MVC?
The Cache Tag Helper provides the ability to improve the performance of your ASP.NET Core app by caching its content to the internal ASP.NET Core cache provider.
The following Razor markup caches the current date:
<cache>@DateTime.Now</cache>
The first request to the page that contains the Tag Helper displays the current date. Additional requests show the cached value until the cache expires (default 20 minutes) or until the cached date is evicted from the cache.
How validation works in MVC and how they follow DRY Pattern?
One of the core design tenets of ASP.NET MVC is DRY (“Don’t Repeat Yourself”). ASP.NET MVC encourages you to specify functionality or behavior only once, and then have it be reflected everywhere in an application. This reduces the amount of code you need to write and makes the code you do write less error prone and easier to maintain.
The validation support provided by ASP.NET MVC and Entity Framework Code First is a great example of the DRY principle in action. You can declaratively specify validation rules in one place (in the model class) and the rules are enforced everywhere in the application.
Describe the complete request processing pipeline for ASP.NET Core MVC
Request delegates are configured using Run, Map, and Use extension methods. An individual request delegate can be specified in-line as an anonymous method (called in-line middleware), or it can be defined in a reusable class. These reusable classes and in-line anonymous methods are middleware, also called middleware components. Each middleware component in the request pipeline is responsible for invoking the next component in the pipeline or short-circuiting the pipeline. When a middleware short-circuits, it’s called a terminal middleware because it prevents further middleware from processing the request.
The Endpoint middleware in the preceding diagram executes the filter pipeline for the corresponding app type—MVC or Razor Pages.
The Routing middleware in the preceding diagram is shown following Static Files. This is the order that the project templates implement by explicitly calling app.UseRouting. If you don’t call app.UseRouting
, the Routing middleware runs at the beginning of the pipeline by default. For more information, see Routing.
TempData in ASP.NET Core
TempData in MVC is used to pass data from Controller to Controller or Controller to view and it is used to pass data that persists only from one request to the next. TempData requires Typecasting. And also check for Null value before reading the value from it.
Various Method in TempData
- Keep : – Marks all keys in the dictionary for retention.
- Keep (with Key): – Marks the specified key in the dictionary for retention.
- Peek (with Key): – Read data from key without marking the key for deletion.
Conclusion
Here we discussed frequently asked ASP.NET Core Interview Questions and answers to help you for your Interview. All these Essential ASP.NET Core Interview Questions are targeted for mid level of experienced Professionals and freshers.
Give the comments, if need to add other questions related to ASP .NET Core MVC.
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.
Thanks for your blog, nice to read. Do not stop.
Nice Article!
Thanks for sharing with us 🙂
snowflake training in hyderabad
Nice Article!
Thanks for sharing with us 🙂
mulesoft training in hyderabad
Nice Article!
Thanks for sharing with us 🙂
Azure Admin Training in HyderabadÂ