In this article we will learn about C Programming- Recursion in C. A function that calls itself is known as a recursive function. And, this technique is known as recursion. Please read my previous article about C here about C Program to Add Two Numbers Using Functions.
- The recursion continues until some condition is met to prevent it.
- To prevent infinite recursion, if…else statement (or similar approach) can be used where one branch makes the recursive call, and other doesn’t.
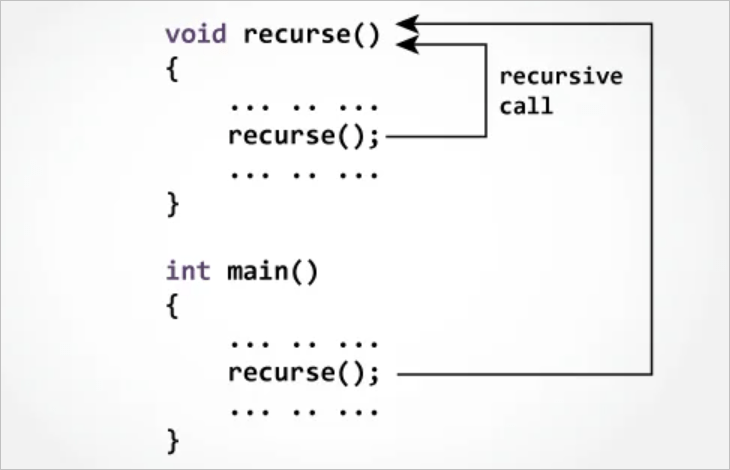
Example of Sum of Natural Numbers Using Recursion
#include<stdio.h>
int sum(int n);
int main(){
int number, result;
printf("Enter a positive integer: ");
scanf("%d", &number);
result= sum(number);
printf("sum= %d", result);
return 0;
}
// create recursiion function
int sum(int n){
if(n!=0){
//sum of function calls itself
return n + sum(n-1);
}else{
return n;
}
}
Output
Enter a positive integer:3 sum = 6
Code Explanation
- Initially, the
sum()
is called from themain()
function with number passed as an argument. - Suppose, the value of n inside
sum()
is 3 initially. During the next function call, 2 is passed to thesum()
function. This process continues until n is equal to 0. - When n is equal to 0, the if condition fails and the else part is executed returning the sum of integers ultimately to the
main()
function.
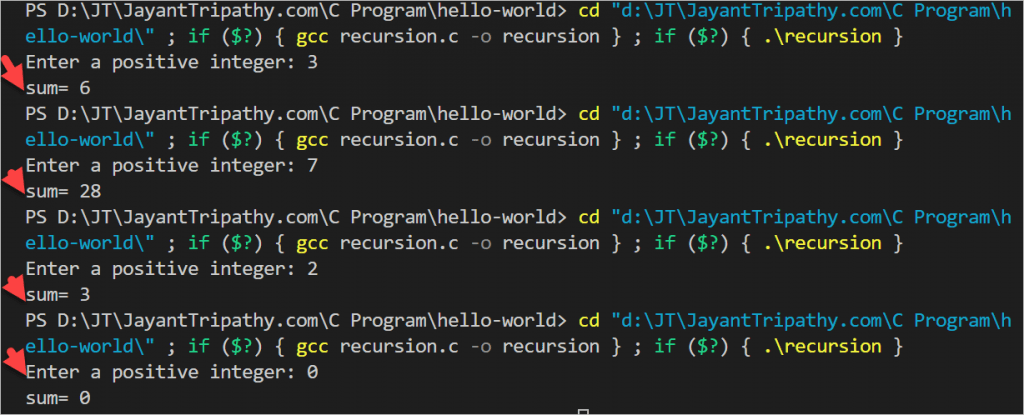
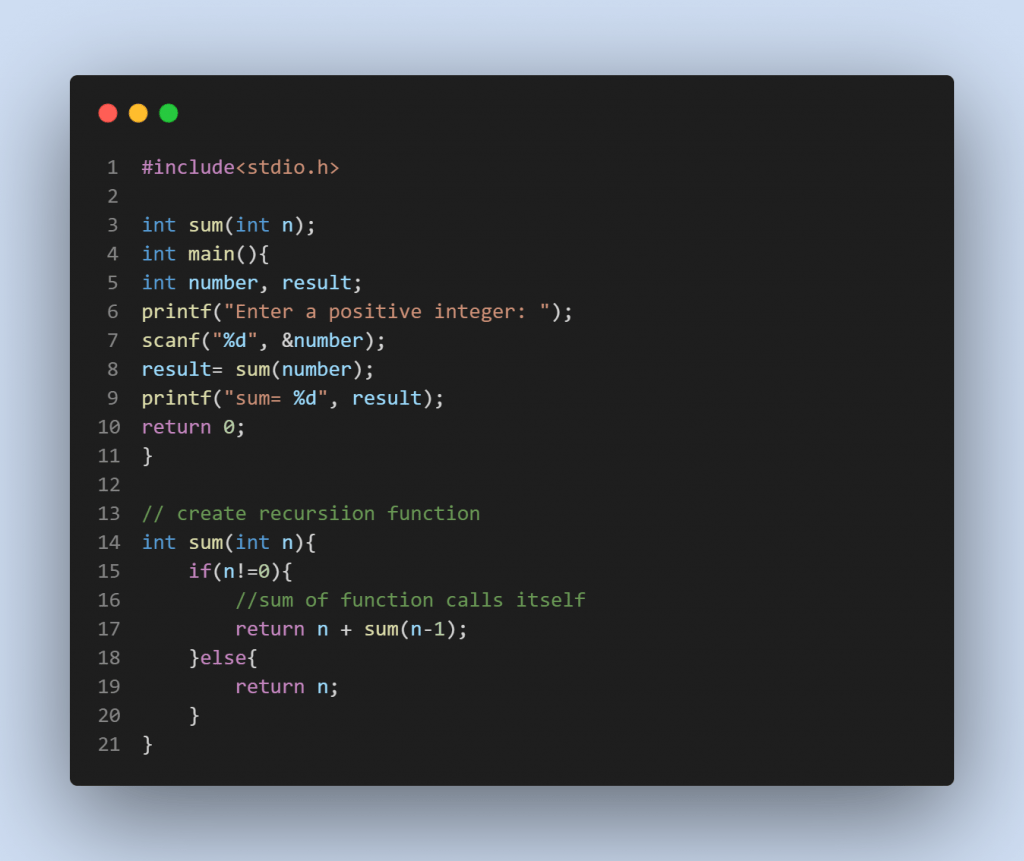
Advantages and Disadvantages of Recursion
Recursion makes program elegant. However, if performance is vital, use loops instead as recursion is usually much slower.
Conclusion
We discussed here about C Program to Add Two Numbers Using Functions.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding đŸ™‚
Related Articles
- Angular Interceptors- The Complete Guide
- How to install and configure AWS Toolkit for VS Code
- Building CI/CD Pipeline(YAML) using Azure DevOps – GitHub to Azure Web App (PaaS)
- How to use Response Compression in .NET Core
- How to send SMS using Amazon Simple Notification Services(SNS)
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.