In this article we are going to discuss how to create custom pipes in Angular. If you are new to Angular then please explore our angular tutorials where we discussed about angular from basic to advance level, you can find here.
What is a Pipe in Angular ?
Every application runs doing a simple task : get data, transform them, and show them to users. Introducing Angular pipes, a way to write display-value transformations that you can declare in your HTML.
Built-in Pipes Provided by Angular
- CurrencyPipe
- DatePipe
- DecimalPipe
- JsonPipe
- LowerCasePipe
- UpperCasePipe
- PercentPipe
- SlicePipe
- AsyncPipe etc..
Create a Custom Pipe in Angular
We take a example of how a description box have a minimal filed using custom pipes. Let’s say a description text is about 500 characters but you want to show it only 50 chars. and on tool tip you may show all the characters.
Let’s create a Angular 9 application using Visual Studio Code.
- Open the VS Code
- Open the VS Code Terminal Window
- Add the below command to create a new project
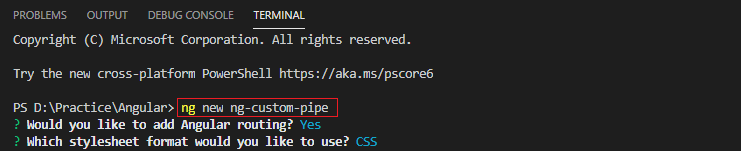
After creation of the project then move into the project folder using below command.
cd ng-custom-pipe
Create a new component named as “product” using below command
ng g c product
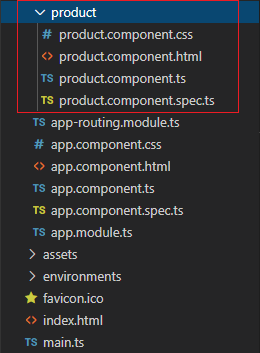
After adding the product component, it automatically add in the module.ts in the root folder.
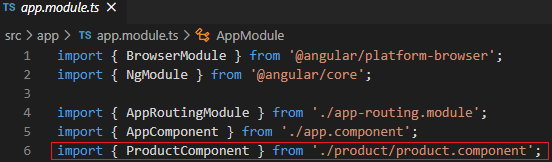
Then remove all the predefined HTML body content in app.component.html.
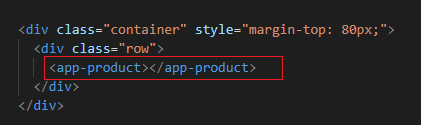
Open the Product.ts file add the dummy product information like this.
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-product', templateUrl: './product.component.html', styleUrls: ['./product.component.css'] }) export class ProductComponent implements OnInit { products: any[]; constructor() { this.products = [ { id: '001', name: 'Laptop', model: 'HP-Pavilion', price: 62000, quantity: 1, description: 'HP Pavilion 15 is a Windows 10 laptop with a 15.60-inch display that has a resolution of 1366x768 pixels. It is powered by a Core i7 processor and it comes with 8GB of RAM. The HP Pavilion 15 packs 1TB of HDD storage. Graphics are powered by Nvidia GeForce 940MX'}, { id: '002', name: 'Mobile', model: 'Samsung-Note10', price: 125000, quantity: 2, description: 'Samsung Galaxy Note 10 is powered by a 1.9GHz octa-core Samsung Exynos 9825 processor that features 4 cores clocked at 1.9GHz, 2 cores clocked at 2.4GHz and 2 cores clocked at 2.7GHz. It comes with 8GB of RAM. The Samsung Galaxy Note 10 runs Android 9 Pie and is powered by a 3500mAh battery.'} ]; } ngOnInit(): void { } }
<h2>Product Information</h2> <table class="table table-bordered"> <thead> <tr> <th>Id</th> <th>Name</th> <th>Model</th> <th>Price</th> <th>Quantity</th> <th>Description</th> </tr> </thead> <tbody> <tr *ngFor='let prod of products'> <td>{{prod.id}}</td> <td>{{prod.name}}</td> <td>{{prod.model}}</td> <td>{{prod.price}}</td> <td>{{prod.quantity}}</td> <td>{{prod.description}}</td> </tr> <tr *ngIf="!products || products.length==0"> <td colspan="7"> No Products to display </td> </tr> </tbody> </table>
Run the application and you can see the output like below; You can see the description is printed here with full text characters.
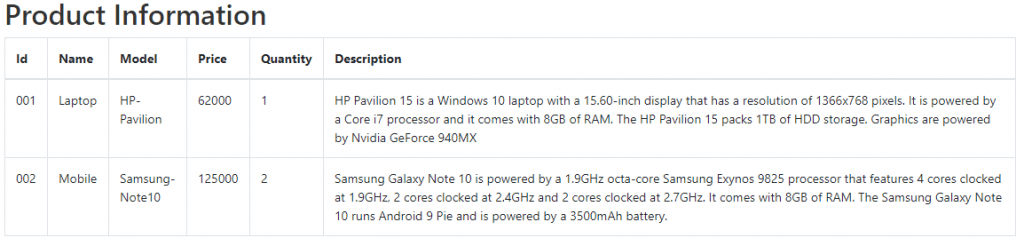
How to use Custom Pipes
Let’s introduce with custom pipes and do the description with minimal characters. Let’s create a new folder named as “CustomPipe” and add a angular pipe using below command.
ng g pipe custom-text-length
import { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'customTextLength' }) export class CustomTextLengthPipe implements PipeTransform { transform(value: string, limit?: number) { if (!value) { return null; } const actuallimit = (limit) ? limit : 75; return value.substr(0, actuallimit) + '......'; } }
Code Explanation
We create the custom pipe named as customTextLength
and here you can see we add the limit text as 75 characters that means when we add this custom pipe into angular interpolation it limit the filed.
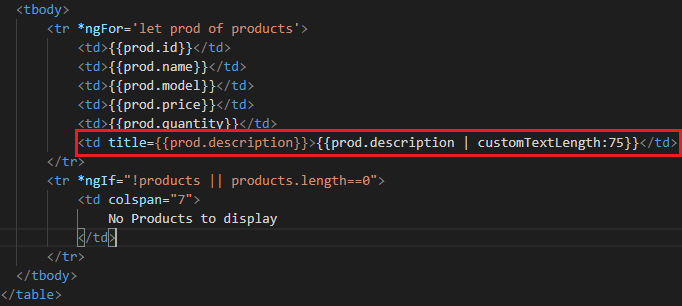
Let’s run the application and see the description field now is limited to 75 characters and on tool tip you can see full text.
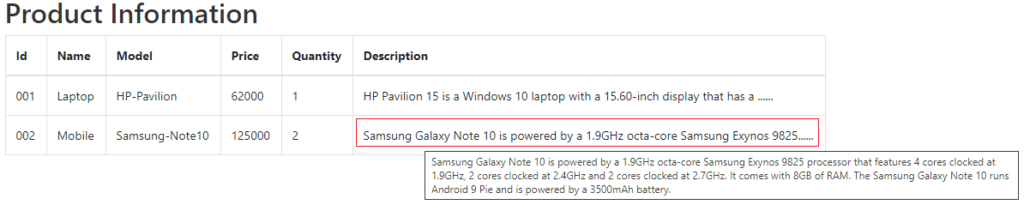
Above image you can see now the custom pipe is worked and it display only 75 characters as per our requirement and on mouseover it shows full text.
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.