In this article we will discuss about Angular Services. We’ll demonstrate how to create a simple component that retrieves a list of products from an Angular Service and displays it in our template. A Service is a class that provides a service to a component, directive, or another service. The Service may be retrieving data from the back end, executing business logic, and so on.
What are Angular Services?
The Angular Services are the piece of code or logic that are used to perform some specific task. A service can contain a value or function or combination of both. The Services in angular are injected into the application using the dependency injection mechanism.
Our components need to access the data. You can write data access code in each component, but that is very inefficient and breaks the rule of single responsibility. The Component must focus on presenting data to the user. The task of getting data from the back-end server must be delegated to some other class. We call such a class a Service class. Because it provides the service of providing data to every component that needs it.
Why do we need a service in Angular?
- Reusable Components: When you need to reuse the same data and logic across multiple components of your application, angular service is the way to go. That is, if you see the same logic or data-access code repeated across multiple components, you should consider refactoring the same logic or data-access code into a service.
DRY (Don't Repeat Yourself)
is a software development principle, and using services in angular safely adheres to this principle. The logic or data is stored in a service, which can be used by multiple components of your angular application. So, services are a mechanism for sharing functionality between components. - Develop, Debug & Test in One place: Without Angular Services, you would have to repeat the same logic or code in multiple components wherever you want this code or logic. Now think about the overhead in terms of time and effort required to develop, debug, test and maintain the duplicated code across multiple places instead of having that duplicated code at one central place like a service and reusing that service where required.
- Features that are independent of components such a logging services.
- Encapsulate external interactions like data access.
Features of Angular Services
- Services in Angular are simply typescript classes with the
@injectible
decorator. This decorator tells angular that the class is a service and can be injected into components that need that service. They can also inject other services as dependencies. - As mentioned earlier, these services are used to share a single piece of code across multiple components. These services are used to hold business logic.
- Services are used to interact with the backend. For example, if you wish to make AJAX calls, you can have the methods to those calls in the service and use it as a dependency in files.
- In angular, the components are singletons, meaning that only one instance of a service that gets created, and the same instance is used by every building block in the application.
- A service can be registered as a part of the module, or as a part of the component. To register it as a part of the component, you’ll have to specify it in the providers’ array of the module.
Understanding Angular Service with an example
An Angular service is simply a Javascript function. All we need to do is to create a class and add methods & properties. We can then create an instance of this class in our component and call its methods.
One of the best uses of services is to get the data from the data source. Let us create a simple service, which gets the product data and passes it to our component.
To create an angular service class we use below command, here product is the service name, You can select according to yours choice.
ng g service product
The angular service is composed of three things. You need to create an export class and you need to decorate that class with @Injectable decorator and you need to import the Injectable decorator from the angular core library. The syntax to create angular service is given below.
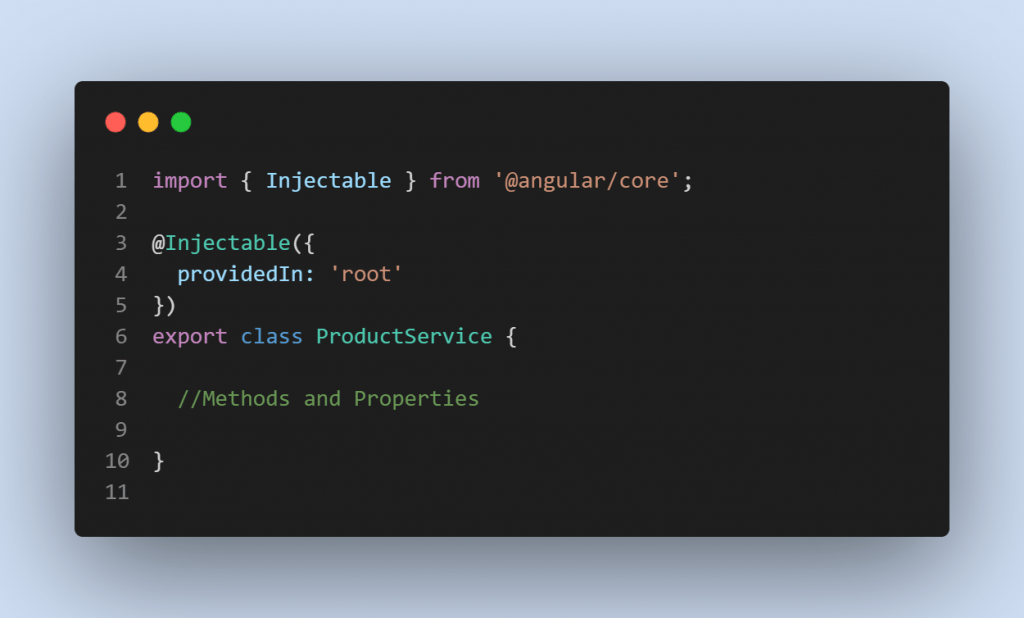
In our example we use the angular service that return us the product items and we display into component template.
Creating a Product Model Class
Using below command we can create the product model class and it holds some properties.
ng g class product
- We have added properties that holds Product Id, name, desc., price and Product image etc.
export class Product {
ProductId: number=0;
name: string='';
description: string='';
price: number=0;
imageName: string='';
constructor(productid:number, name: string, description: string, price: number, imageName: string) {
this.ProductId = productid;
this.name = name;
this.description= description;
this.price = price;
this.imageName = imageName;
}
}
Adding dummy items into Product Service
We have already create the Product service, so next is open the service and return the product Items.
- We import the
Product
model from theproduct.ts
. - The
getProducts
method returns the collection of the products. In this example, we have hardcoded the products. In real life, you would send an HTTP GET request to your back end API to get the data. The hardcoded product items hold name, description, Price with the image name.
import { Injectable } from '@angular/core';
import { Product } from '../class/product';
@Injectable({
providedIn: 'root'
})
export class ProductService {
products: Product[]=[];
constructor() { }
getProductDetails(){
this.products = [
new Product(1,'HP Spectre x360', 'HP Spectre x360 2-in-1 Laptop OLED Touch 13.5-ef0054TU.', 149999,'laptop.jpg'),
new Product(2,'iPhone 13 Pro', 'Apple iPhone 13 Pro (256 GB) - Alpine Green', 119900,'mobile.jpg'),
new Product(3,'SONY Camera', 'SONY ILCE-7M4K/BQIN5 Mirrorless Camera Single Lens: 28-70 mm (Black)', 230990,'camera.png'),
new Product(4,'SAMSUNG Crystal 4K', 'SAMSUNG Crystal 4K 138 cm (55 inch) Ultra HD (4K) LED Smart Tizen TV (UA55AUE60AKLXL)', 45990,'tv.png'),
];
return this.products;
}
}
Adding Images into the Assets folder
We have used some images to show in the product details page. I have added some static images inside this folder,
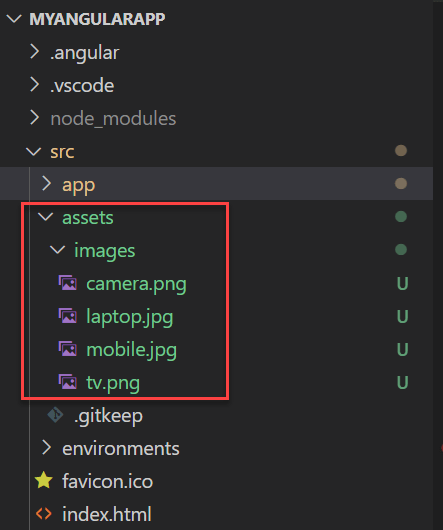
Invoking the ProductService on the Component
We have created one component named as “ProductComponent” and here we are invoking the Product Service.
- We imported Product class and Product Service inside the Product Component class.
- Added the Product Service on the constructor to initialize – In real-life Angular Apps, we use the Dependency Injection in Angular to inject the ProductSerivce in the constructor. We will learn that in the next tutorial.
- On the OnInit() method we get access the Product Service getProducts() method.
import { Component, OnInit } from '@angular/core';
import { Product } from 'src/app/class/product';
import { ProductService } from 'src/app/services/product.service';
@Component({
selector: 'app-product',
templateUrl: './product.component.html',
styleUrls: ['./product.component.css']
})
export class ProductComponent implements OnInit {
products: Product[]=[];
productService;
constructor() {
this.productService=new ProductService();
}
ngOnInit(): void {
this.products = this.productService.getProductDetails();
}
}
Adding the Product details on the Template
- We loop through the products via ngFor Directive in Angular that iterates through the items.
- Using Angular Interpolation we added bind the data.
- We use here bootstrap card for styling.
- We have passed 4 items into the product service with Product image, Product Name, Description and Price that are iterate into the cards.
- For the price we have added Angular Pipe to customize the currency according to Indian Rupees.
<h5>Product Details Page</h5>
<div class="card-deck mt-3">
<div class="card" style="width: 18rem;" *ngFor="let prod of products">
<img class="card-img-top" src="../../../assets/images/{{prod.imageName}}" alt="Card image cap">
<div class="card-body">
<h5 class="card-title">{{prod.name}}</h5>
<p class="card-text">{{prod.description}}</p>
</div>
<span style="padding: 10px;"> Price <strong>{{prod.price | currency:'INR':true:'4.2-2'}} </strong></span>
</div>
</div>
That’s it. Now everything looks good. Run the application and we can see the output like below,
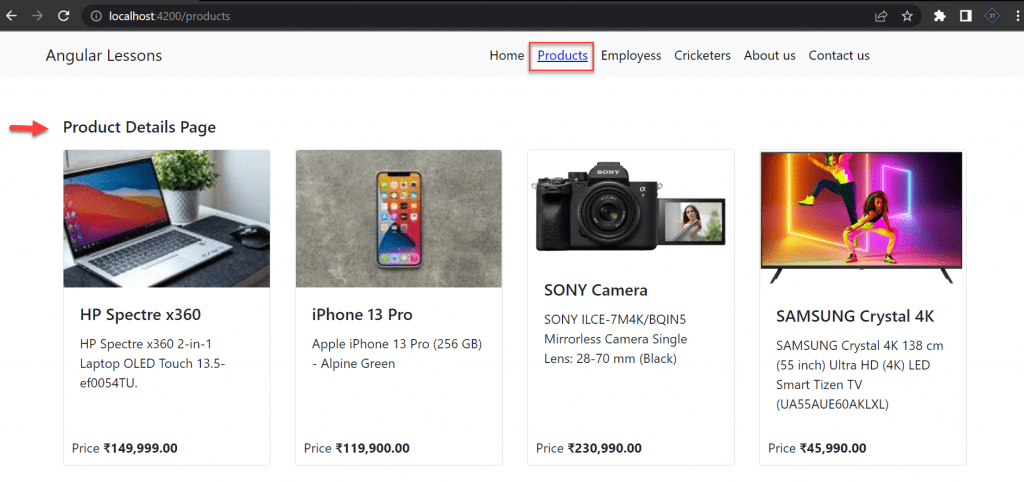
What is the issue causing on Injecting Service like above?
In the above example we instantiated the productService as this.productService=new ProductService()
.
The disadvantages of directly instantiating the service, as shown above, are numerous.
- The ProductService is tightly coupled to the Component. If we change the ProductService class definition, then we need to update every code where service is used.
- If we want to change ProductService with BetterProductService, then we need to search wherever the ProductService is used and manually change it.
- Makes Testing difficult. We may need to provide mockProductService for testing and use the ProductService for Production.
On the next article we discuss about Angular Dependency injection where we short out the above issue.
Conclusion
We discussed here about Angular Services, we learnt why & when it require to use the services in Angular. We create a product service example and bind the items into template.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Related Articles
- Angular Interceptors- The Complete Guide
- Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular
- Introduction to Azure Cosmos DB
- How to create Cosmos DB in Azure
- Why ReactJS is used over plain JavaScript
- Introduction to GraphQL
- Creating a Sample ReactJs Application using VS Code
- How to use Policy-based Authorization using JWT in .Net Core 7 and Angular
- Building Single Page Applications(SPA) with ReactJS
- How to deploy Angular App in Azure Static Web App
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.