In this article we discuss read external JSON file in Jquery Ajax. There are many ways to store JSON data in our application. We can store JSON in an array or in an external file. There are many ways to read JSON data from external files, here we see how it be done using Jquery Ajax. Please read my previous article Download Json array as File using Jquery.
What is Ajax ?
Ajax is a set of web development techniques using many web technologies on the client side to create asynchronous web applications. With Ajax, web applications can send and retrieve data from a server asynchronously without interfering with the display and behavior of the existing page.
Let’s you have a external JSON file named as sample.json and it holds the JSON value like below;
{"first_name" : "John", "last_name" : "Smith", "location" : "USA"}
How to read JSON file in Jquery AJAX
Here let’s create a HTML and use Jquery Ajax to read the json.
<html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <script> $.ajax({ type: "GET", url: "./json/sample.json", success: function (response) { console.log(response) $("div").append("First Name : " + response.first_name+ "<br/>Last Name : " +response.last_name+ "<br/> Address : " + response.location); } }); </script> <body> <div></div> </body> </head> </html>
Code Explanation
On the above we create a AJAX method to read the external JSON fileby passing in the ajax URL. After getting response print the result in the DIV tag. Also if we open the browser console window you can see the output like below;
After run the application you can see the output like below;
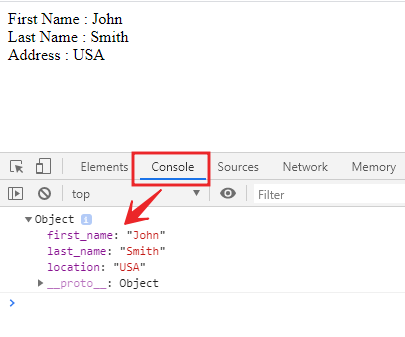
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.