In this article we will learn about How to use ChatGPT Integration in ASP.Net Core using OpenAI. We can incorporate ChatGPT into the ASP.NET Web API project in two ways. The first is by utilizing the OpenAI API, and the second is by utilizing the OpenAI library. We do not need to use an API endpoint in the second method. Integrating ChatGPT completions into a C# application can provide users with a natural language experience for text generation based on their input. Please read my previous article How to Implement AutoMapper in ASP.Net Core – Beginner’s Guide.
What is ChatGPT?
OpenAI created ChatGPT, a conversational AI model. It is a subset of the GPT-3 transformer-based language model (Generative Pretrained Transformer 3). It is capable of generating human-like text responses to natural language queries after being trained on a large corpus of text data. The model has been fine-tuned for text generation tasks such as sentence and paragraph completion, as well as generating entire articles based on given prompts.
ChatGPT is used to provide a natural language interface to users in a variety of applications, including chatbots, content generation, and text completion tools. ChatGPT’s versatility makes it a valuable tool for a wide range of applications, including chatbots, content generation, and text completion tools. ChatGPT enables seamless and intuitive communication between humans and technology.
What is Text Completion?
Text completion is a language processing task that involves generating the missing portion of a partially given text sequence. It is a type of text generation task where the model is given an incomplete text promptly and is asked to generate the missing text based on the context.
Generating API Key
To generate an OpenAI API key, follow these steps:
- Signup for an OpenAI account. Go to the OpenAI website and create a new account.
- Confirm your email address.
- Now, Log in to your account and navigate to the ‘View API keys’ section as given below and click on
create new secret key
and copy the secret keys.
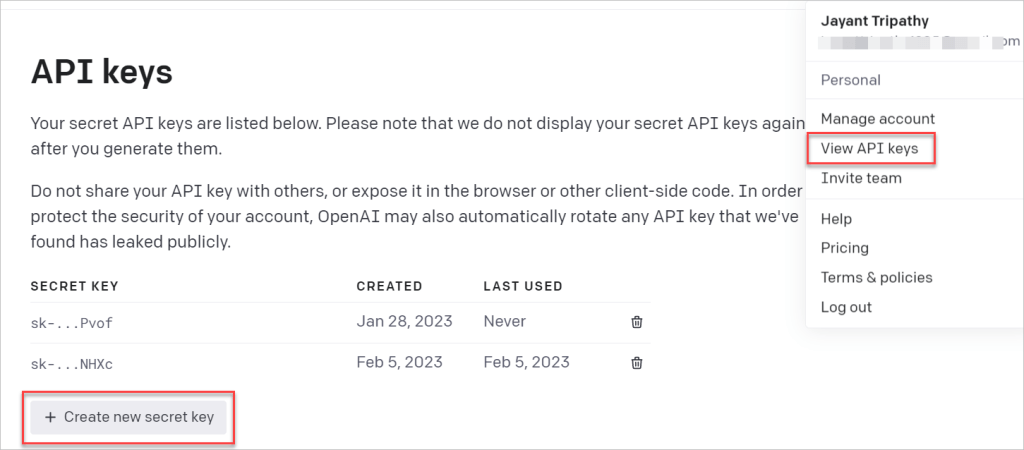
Store your API key in a secure location, as it will be required to access the OpenAPI completions. You can copy the key and save it for future use. OpenAI has usage and resource limitations for its API, so be sure to check its documentation here for details on usage and pricing.
Creating an ASP.Net Core Web Application
- Launch the Visual Studio IDE and click on “Create new project”.
- In the “Create new project” window, select “
ASP.NET Core Web Application
” from the list of templates displayed. - Click Next. In the “Configure your new project” window, specify the name and location for the new project and then click Create.
- In the “Create New ASP.NET Core API” window shown next, select .NET Core as the runtime and
.NET 7.0
from the drop-down list at the top. Select “API” as the project template to create a new ASP.NET Core API application. - Ensure that the check boxes “Enable Docker Support” is disabled s we won’t be using those features here and “Configure for HTTPS” are checked.
- Ensure that Authentication is set as “No Authentication” as we won’t be using authentication either and Click Create.
Adding the OpenAI NuGet package

Modifying the View templates to get the ChatGPT Answers
- Here we have taken a Input field and have a button, when we write your text and search the button then it gives the result.
@{
ViewData["Title"] = "Home Page";
}
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<div class="text-center">
<form asp-controller="Home" asp-action="Index" method="post">
<div class="panel panel-default">
<div class="panel-body">ChatGPT Completions In ASP.NET Core Web API</div>
<div class="panel-body">
<div class="col-sm-9">
<input type="text" name="searchText" value="@ViewBag.Text" class="form-control" autocomplete="off" />
</div>
<div class="col-sm-3" style="margin-left: -70px;">
<input type="submit" class="btn btn-primary" value="Search Answer" />
</div>
@if (ViewBag.Answer != null)
{
<div class="col-sm-12 mt-5">
@Html.Raw(ViewBag.Answer)
</div>
}
</div>
</div>
</form>
</div>
Modify the API Controller to implement the ChatGPT by OpenAI
- Add the copied OpenAI key like below.
completion.Prompt
holds the serachText that are coming from UI.- The result is collected from the loop, and provided the data using
ViewBag
[HttpPost]
public IActionResult Index(string searchText)
{
//your OpenAI API key
string apiKey = "XXXsk-6S92ywcjqqHOTFXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX";
string answer = string.Empty;
var openai = new OpenAIAPI(apiKey);
CompletionRequest completion = new CompletionRequest();
completion.Prompt = searchText;
completion.Model = OpenAI_API.Models.Model.DavinciText;
completion.MaxTokens = 4000;
var result = openai.Completions.CreateCompletionAsync(completion);
if (result != null)
{
foreach (var item in result.Result.Completions)
{
answer = item.Text;
}
}
ViewBag.Answer = answer;
ViewBag.Text = searchText;
return View();
}
The output result like below,

Conclusion
ChatGPT Integration in ASP.Net Core using OpenAI, ChatGPT is a revolutionary conversational AI model that is transforming the way we interact with technology. Its ability to generate human-like text responses and its versatility make it a valuable tool for various applications, and its development is a testament to the progress made in the field of AI and natural language processing. To integrate ChatGPT in ASP.NET Core first you need to generate the OpenAI API key and then use that API key in the code as given above.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Related Articles
- How to Integrate AdminLTE with ASP.NET Core
- How to run ASP.Net Core Web Application in Docker Container
- ChatGPT Integration in ASP.Net Core using OpenAI
- How to use cookies in ASP.Net Core- Complete Guide
- Deploy ASP.Net Core apps to Azure App Service
- How to detect whether page is load on Mobile or Desktop browser in ASP.Net Core
- AddTransient Vs AddScoped Vs AddSingleton Example in ASP.Net Core
- Razor file compilation in ASP.NET Core
- ViewData in ASP.Net Core MVC
- How controller finds a view (View Discovery)
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.
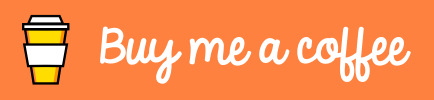
I using your source but I have an error
HttpRequestException: Error at completions (https://api.openai.com/v1/completions) with HTTP status code: TooManyRequests. Content: {
“error”: {
“message”: “You exceeded your current quota, please check your plan and billing details.”,
“type”: “insufficient_quota”,
“param”: null,
“code”: null
}
}
Hi MOHAMMAD, Thanks to reaching out to me.
This error message indicates that you have hit your maximum monthly spend (hard limit) for the API. This means that you have consumed all the credits or units allocated to your plan and have reached the limit of your billing cycle. This could happen for several reasons.
For more details please follow the Open AI help link : https://help.openai.com/en/articles/6891831-error-code-429-you-exceeded-your-current-quota-please-check-your-plan-and-billing-details
Would be really nice to know what files each of these code samples go into. And what the folder the “.proj” files goes into and so forth.
As an ASP.NET developer, I use a front page and a code-behind page, The front page may be named “mypage.aspx” and it presents an interface to the user, often containing a form. The code-behind file is named “mypage.aspx.vb” (could be a C# file instead) and the code-behind page does all the external integration – database read/write, file creation, email generation, etc. I need to also be able to pass the user’s prompt to OpenAI with my API key an receive a completion, which can then be displayed on the screen. That’s what ASP.NET is, and that’s how it needs to integrate with ChatGPT. What I see here is a collection of code and and files I have never heard of.
Hi Scott, Thanks for writing ….
“.proj” file is the project file extension. This project is developed on C# application so the the extension here is “csproj”. For .NET Core application all the added packages and dependencies are present in “csproj” file.
If we can open the file we can see all the dependencies of this project are resides here.
Please check this link
https://github.com/JayantTripathy/ChatGPT-Integration/blob/master/ChatGPT-Integration/ChatGPT-Integration.csproj
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.