Introduction
When it comes to Angular interviews one of the most asked questions is around Rxjs. RxJs even though very simple when it comes to expressing answers and explanation it has its own challenges. This article will cover important RxJS Interview Questions which will help you fly in your Angular interviews. Happy learning and Happy job hunting.
Please read Angular Interview Questions here.
What is RxJs and why do we need it ?
RxJs stands for Reactive extensions for JavaScript. RxJs helps to handle asynchronous data stream with ease.
It is a JavaScript library that uses observables to work with reactive programming and deals with asynchronous data calls, callbacks and event-based programs. RxJS has introduced the concept of “reactive programming” to the web. It implements a reactive extension for TypeScript and JavaScript.
Assume you have an entity which is streaming async data, now this entity which is streaming async data can be a HTTP response , Port which is streaming data , Timer emitting data and so on. This Async stream is coming in undecided intervals like stream of data. As a developer you would like to listen to this stream , run some logic on these stream and so on. RxJs makes this task easy.
What should we know before going to learn RxJS?
Before learning RxJS, we must have a basic knowledge of JavaScript, JavaScript frameworks, and Angular. You can easily understand this technology if you have a basic understanding of JS.
What is Reactive Programming?
Reactive programming is a declarative programming paradigm which deals with asynchronous data streams. Reactive programming facilitates us to create data streams of anything, not just from click and hover events.
What are the biggest advantages of Reactive Programming?
- Reactive programming provides a lot of operators that can simplify our work.
- Reactive programming is very simple to compose streams of data.
- It can be used to avoid “callback problems”.
- In Reactive programming, it is very simple to do async and threaded task.
- It makes complex threading very easy.
- By using Reactive programming, we can get a more cleaner and readable code base.
- In Reactive programming, it is easy to implement back-pressure.
What are the Pros and Cons of using RxJS?
Pros of RxJS:
- RxJS can be used with other Javascript libraries and frameworks. It is supported by javascript and also with typescript. Few examples are Angular, ReactJS, Vuejs, nodejs etc.
- RxJS is an awesome library when it comes to the handling of async tasks. RxJS uses observables to work with reactive programming that deals with asynchronous data calls, callbacks and event-based programs.
- RxJS offers a huge collection of operators in mathematical, transformation, filtering, utility, conditional, error handling, join categories that makes life easy when used with reactive programming.
Cons of RxJS:
- Debugging the code with observables is a little difficult.
- As you start to use Observables, you can end up with your full code wrapped under the observables.
What is Stream in RxJS?
- A stream is a sequence of data elements made available over time.
- A stream can be thought of as items on a conveyor belt being processed one at a time rather than in large batches.
- The reason for it being called a stream is that you should think of the data as continuous and not really having an end, unless you explicitly define an end.
Where did you use RxJs in Angular?
Most of the times rxJs is used in http calls with angular. As http streams asynchronous data we can subscribe , apply filters to the http streams. Below is a simple sample code of how RxJs can be used with HTTP calls.
var stream1 = httpc.get("https://www.myapi.com/somedata"); var stream2 = stream1.pipe(filter(x=>x>3)); stream2.subscribe(res=>this.Success(res),res=>this.Error(res));
What are observables and observers?
RxJs library helps to handle async data stream easily. But in order to access the async stream it has to be exposed as a rxJs Observable object. The listener who is interested in accessing the Observable stream is exposed as an observer.
In simple word observable represents async stream of data and observer subscribes to the observable to receive the stream.
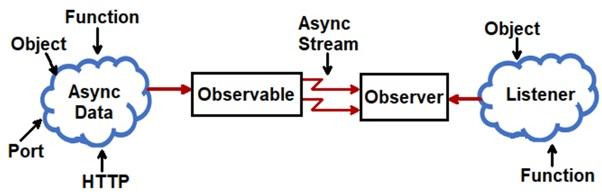
We have to follow the following three steps to complete the RxJS observable:
- Create Observable
- Subscribe Observable
- Execute Observable
What is the difference between RxJS Observables and Promises?
Observable
An observer is like a stream and allows you to pass at least zero or more events where the callback is needed for each event.
- Observable is favored over Promise, it can emits multiple values over a time.
- The “Observable” is cold. It’s not called until we’re registered to it.
- You may cancel an Observable with the unsubscribe() method
- Observable provides a lot of efficient operators like map, foreach, filter, reduce, retry, retryWhen etc.
Promise
A Promise emits a single event at the completion or failure of an async operation.
- promise emits a single value
- A promise is Not Lazy A Promise cannot be cancelled
RxJS Observables | Promises |
---|---|
Observables are used to run asynchronously, and we get the return value multiple times. | Promises are used to run asynchronously, and we get the return value only once. |
Observables are lazy. | Promises are not lazy. |
Observables can be canceled. | Promises cannot be canceled. |
Observables provide multiple future values. | Promises provide a single future value. |
Example of RxJS Observables and Promises
Observables Example
const { Observable } = require('rxjs'); let observable = new Observable((observer) => { setTimeout(() => { observer.next("some value") }, 1000) }) observable.subscribe(value => { console.log(value) })
output
some value
Unlike the Promise, Observables aren’t native to JavaScript. This requires us to import the RxJS library:
const { Observable } = require('rxjs');
While you can implement the observer design pattern yourself, the “Observable” in JavaScript usually refers to the RxJS implementation.
Promises Example
let promise = new Promise((resolve) => { setTimeout(() => { resolve("some value") }, 1000`) }) promise.then(value => { console.log(value) })
output
some value
A Promise works by taking a function with (optionally) two arguments resolve and reject. The passed in function executes some async code and either resolves or rejects.
Once the Promise resolves, its then() method executes a handler function with the emitted value.
What is the difference between Cold and Hot Observables in RxJS?
In simple words, the concept of cold and hot Observable can be defined as the following:
When the data is produced by the Observable itself, t is called the cold Observable. When the data is produced outside the Observable, it is called hot Observable.
Let’s see the differences between Cold Observables and Hot Observables:
Cold Observables | Hot Observables |
---|---|
We can call an Observable “cold” when the data is produced inside the Observable. | We call the Observable “hot” when the data is produced outside the Observable. |
Cold observables start to run upon subscription. | Hot observables produce values even before a subscription is made. |
The Cold observable sequence only starts pushing values to observers when subscribe is called. | Hot observables such as mouse move events, stock pickers or WebSocket connections are already produced in values even before the subscription is active. |
The cold Observable starts running upon subscription. | The hot Observable produces values before subscriptions. |
The cold Observable sequence starts pushing values. | In cold Observable, the data producer is outside the Observable. |
In cold Observable, the data is produced inside the Observable so, we cannot share the data between multiple subscribers. Two Observables that subscribe at more or less the same may receive two different values. We call this behavior “unicasting.” | As we know that the data is produced outside the Observable in hot Observable, so it can share data between multiple subscribers in hot Observable. This behavior is “multicasting.” |
What is difference between BehaviorSubject and Observable?
The difference between BehaviourSubject and Observable are below –
Observable | BehaviourSubject |
---|---|
Observable is stateless | BehaviourSubject is stateful |
Observable creates copy of data | BehaviourSubject shares data |
Observable is unidirectional | BehaviourSubject is bidirectional |
What is the use of subscribe in RxJs?
“Subscribe” function starts the stream from observable to the observer function. You can see in the below code how the subscribe function takes a function as reference. So when data is streamed from Observable its received by the Listener function.
var observ = Observable.create(AsyncStream); observ.subscribe(res=>Listener(res)); function Listener(res) console.log(res); }
How to unsubscribe from the stream?
We need to get reference of the “subscription” object. This subscription object is returned when we call the “subscribe” function. To unsubscribe we can call “unsubscribe” on the “sunscription” object.
var subscription = observ.subscribe(res=>Listener(res)); subscription.unsubscribe();
What is Subject ?
- Subjects are unique types of Observers, that enables you to subscribe to other Observables and also listen to published data
- Listening to the stream is called subscribing.
What does a subject do in RxJS?
Subject helps in allowing the values to be multicast for many observers. They are multicast instead of plain observables, it is the equivalent of an event emitter and also the only way of multicasting a value or event or multiple observers.
Subjects implements observable and observer interfaces. very subject is an observer. It means that you have next, error, and complete methods, so you can send values, error subject or completed.
There are 3 types of subjects:
- ReplaySubject
- BehaviorSubject
- AsyncSubject
What are the differences between Subject, BehaviorSubject and ReplaySubject in RxJS?
Subject
In the RxJS Subject, Observers who are subscribed later do not obtain the data values emitted before their subscription.
ReplaySubject
In RxJS ReplaySubject, Observers who are subscribed at a later point receives data values issued before their subscription. It operates by using a buffer that holds the values emitted and re-emits them once new Observers are subscribed.
BehaviorSubject
BehaviorSubject functions similar to ReplaySubject but only re-issues the last emitted values. So, it should be used when you are interested in the observer’s last/current value.
What is RxJS concatMap?
ConcatMap is a combination of 2 operators(concat and map), it lets us mapping a value from a source observable to an observale stream. When the inner observable lets it value and pass it down to an observable. It helps in collecting all the emitted values from all of the inner observables and emits it into the subscribers.
Concat is used for taking each form value and in transforming into an observer HTTP known as inner observer. It also helps in subscribing to the inner observable and also sends the output to the observable result.
What are the operators in RxJS ?
Some of the operators in RxJS are as follows-
- tap()
- catchError()
- switchAll()
- finalize()
- throwError() etc..
What are switchMap, mergeMap and concatMap?
These are the operators used to flatten the observables, they are applicable in different scenarios. Switch and Merge maps are the most powerful and they are frequently used as the operators.
Mergemap used in creating an observable immediately for any other source item and it keeps the observables alive.
Concatmap are used for crating a new observable.
Switchmap helps in completing the previous observable and creates the next observable.
concatMap() Operators
this.form.valueChanges .pipe( concatMap(formValue => this.http.put("/api/book/",formValue)) ) .subscribe( response => ... handle successful ..., err => ... handle error ... );
The two main benefits of using concatMap() operator are that we no longer have to use nested subscribes with higher-order mapping operator, and the second is, all http requests are sent to the backend sequentially.
This is how the concatMap operator ensures that the requests still occur in sequence:
- concatMap takes each form value and transforms it into an observer HTTP, known as an inner observer.
- concatMap subscribes to the inner Observable and sends its output to the Observable result
- The second form of value can come more quickly than is needed to request in the backend the previous form value. When this occurs, the new form value is not converted to an HTTP request immediately.
mergeMap() Operator
Unlike the RxJS concatMap operator, mergeMap() will not wait until the Observable finishes until the next Observable is subscribed.
This is how the mergeMap operator works:
- In mergeMap operator, every Observable source value is mapped in an internal Observable.
- The inner Observable is then subscribed by mergeMap.
- When the inner observables emit new values, the output Observable immediately reflects them.
- In the mergeMap, unlike the concatMap operator, we do not need to wait until the previous inner observable is completed.
switchMap() Operator
Unlike the mergeMap operator, in the switchMap operator, we unsubscribe the previous Observable before subscribing to the new Observable if the new Observable begins to emit the values.
What is the difference between Imperative, Functional and Reactive Programming?
Imperative Programming: Imperative programming is a programming paradigm where each line of code is sequentially executed to produce the desired result. This programming paradigm forces programmers to write “how” a program will solve a certain task.
Functional Programming: Functional programming is a programming paradigm where we can set everything as a result of a function that avoids changing states and mutating data.
Reactive Programming: Reactive programming is a programming paradigm with asynchronous data streams or event streams. An event stream can be anything like keyboard inputs, button taps, gestures, GPS location updates, accelerometer, iBeacon etc. Here, we can listen to a stream and react to it according to the situation.
What is the Reactive Manifesto?
Reactive Manifesto helps in defining the core principles of reactive programming and it underpins the principles of reactive programming.
Types of Reactive Systems are as follows:
- Responsive – helps in detecting and dealing the problems quickly, by providing rapid and consistent response times.It also simplifies error handling, builds end user confidence, and encourages further interaction.
- Resilient – it helps in recovering each componenet that are delegated with external component and also ensures high availibility by replication.
- Elastic – helps by implying designs which have no contention points in resulting the ability for sharding and replicating components.
- Message Driven – acts as an asynchronous message-passing for establishing a boundary between the components that ensures loosecoupling, isolation and location transparency.
What is NgRx?
NgRx stands for Angular Reactive Extensions, NgRx Store provides reactive state management for Angular apps inspired by Redux. NgRx has libraries for managing both global and local state. Isolation of side effects in order to achieve a more streamlined component architecture. Developer tooling that makes it easier for developers to construct a variety of applications
When we use zip and combineLatest and withLatestFrom?
zip
and combineLatest
are functions and withLatestFrom is operator that allows to combine a few observable sequences in a different ways, that are really helpful in real world application.
Composing functions and operators usually accept observables as their params and also they return observable that emits array with values produced by argument observables. This result observable emission logic is different depending on which operator or function we use.
What are Schedulers
A scheduler controls the execution of when the subscription has to start and be notified.
Types of schedulers: queueScheduler, asapScheduler, asyncScheduler, animationFrameScheduler
What is RxJS Map and What is Higher-Order Observable Mapping?
- RxJS Map: It’s a flattening operator used to transform current emitted data values to the desired data format.
const http$ : Observable<Books[]> = this.http.get('/api/books'); http$ .pipe( tap(() => console.log('HTTP request executed')), map(res => Object.values(res['payload'])) ) .subscribe( books => console.log("books", books) );
Higher-Order Mapping: These are Rxjs operators used to map source observable values into other observables.
What is RxJS exhaustMap?
- exhaust: Converts a higher-order Observable into a first-order Observable by dropping inner Observables while the previous inner Observable has not yet completed.
- It behaves like mergeAll. However, exhaust ignores every new inner Observable if the previous Observable has not yet completed. Once that one completes, it will accept and flatten the next inner Observable and repeat this process.
- ExhaustMap: Projects each source value to an Observable which is merged in the output Observable only if the previous projected Observable has completed.
const clicks = fromEvent(document, 'click'); const result = clicks.pipe( exhaustMap(ev => interval(1000).pipe(take(5))) ); result.subscribe(x => console.log(x));
What is Redux?
Open-Source JavaScript library for managing application state. Used in frontend framework such as React, Angular & Rxjs
What are the core principles of Redux?
–Single source of Truth: All application data is stored in a single object tree.
- State is read only: The state is immutable.
- State can only be change with Pure Functions: These pure functions are called Reduces and they specify how the object tree is should be updated.
Is there any similarity between Redux and RxJS?
- Redux uses reactive paradigm to mutate state of the store.
- RxJs uses reactive paradigm to accomplish asynchronous data processing.
What is the difference between Reactive Programming and Imperative Programming?
- Reactive programming is a programming paradime where data is being Pushed to subscribed observers in an application. Where as:
- Imperative programming is a programming paradime where data is being Pulled on request from an application.
What is the difference between BehaviorSubject and Observable in RxJS?
- Observable is a consumer/listener while a BehaviourSubject is a synchronous data source that emits data to observers.
- Observables are stateless while BehaviourSubject is stateful.
- Observables create a copy of the data while BehaviourSubject emits data to observers.
- Observables are unidirectional in nature while BehaviorSubject is bi-directional in nature.
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂