In this article we will learn about how to Using API Key Authentication To Secure ASP.Net Core Web API. API key authentication will keep a secure line between the API and clients. Please follow all the ASP.Net Core articles we will learn in this link. There are various type authentications mechanism in ASP.Net Core Web API application we can discuss it on my upcoming articles.
Creating an ASP.Net Core Web API App
Let’s create an ASP.Net Core Web API Application using .NET Core 6
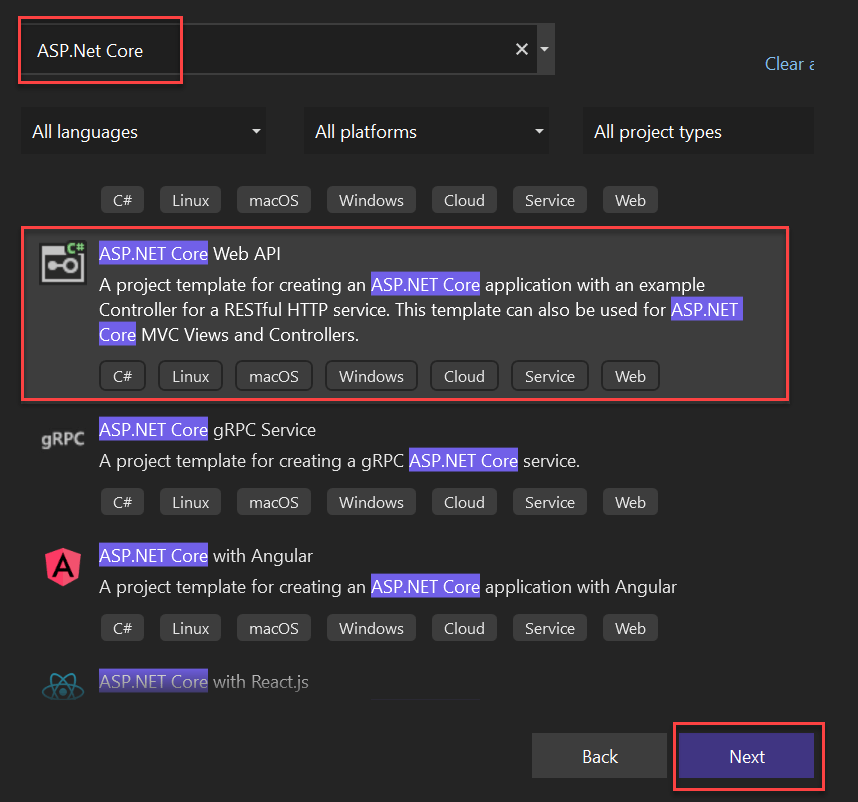
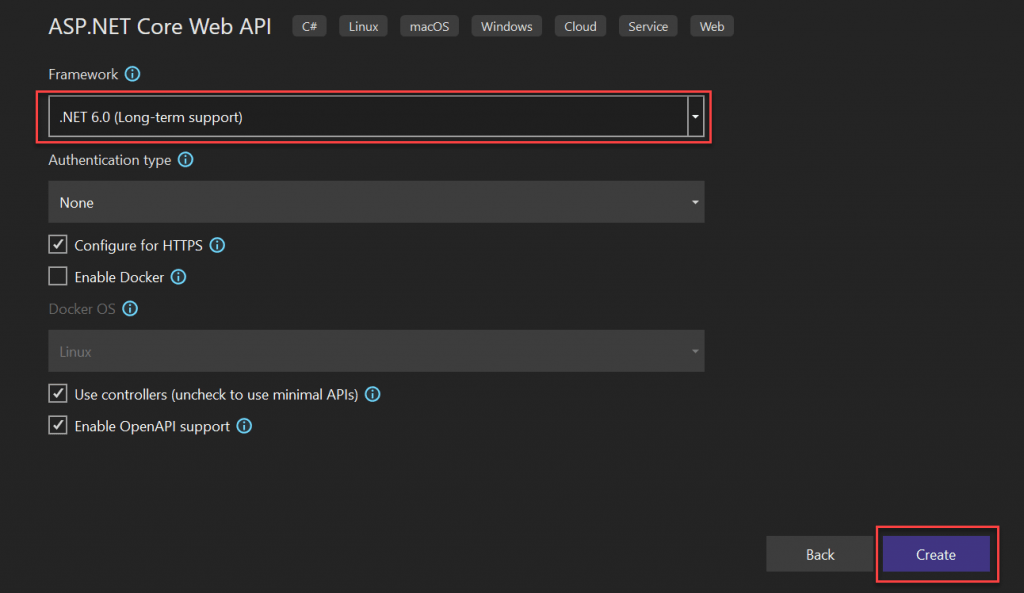
Once run the application we can see the application run in swagger and Postman
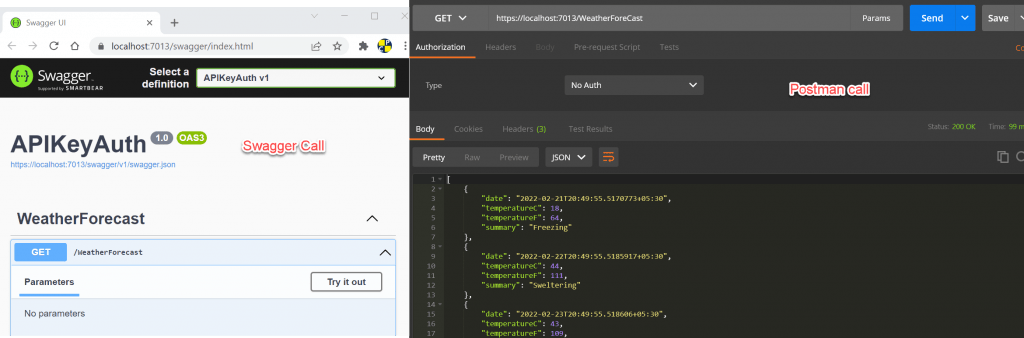
Applying API Key conditioned in API
Create a Middleware Folder, and add a new C# file. I named the new class as APIKeyMiddleware.cs
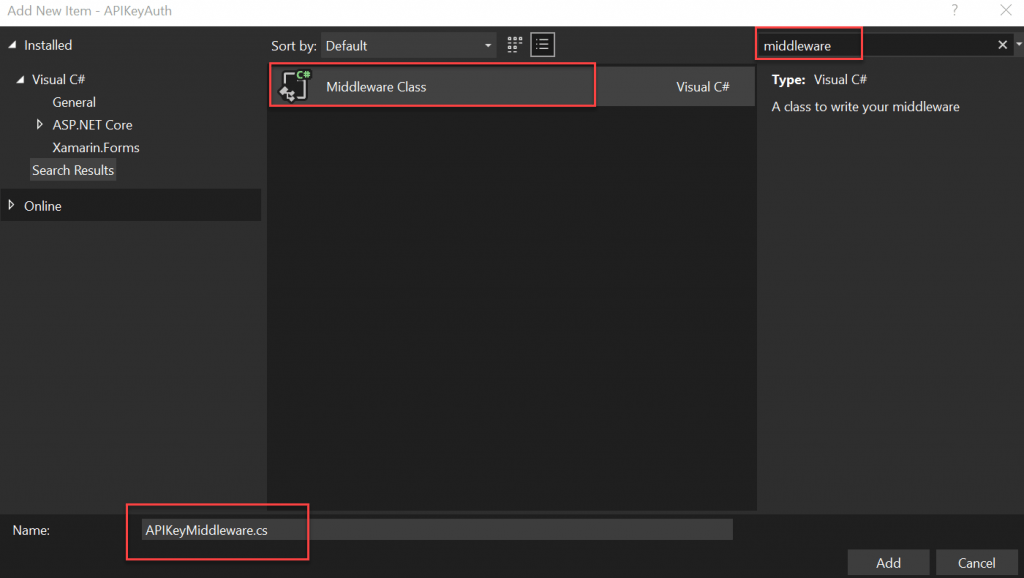
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Http;
using System.Threading.Tasks;
namespace APIKeyAuth.Middleware
{
// You may need to install the Microsoft.AspNetCore.Http.Abstractions package into your project
public class APIKeyMiddleware
{
private readonly RequestDelegate _next;
const string APIKEY = "x-authKey";
public APIKeyMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task Invoke(HttpContext httpContext)
{
if (!httpContext.Request.Headers.TryGetValue(APIKEY, out
var extractedApiKey))
{
httpContext.Response.StatusCode = 401;
await httpContext.Response.WriteAsync("Api Key missing ");
return;
}
var appSettings = httpContext.RequestServices.GetRequiredService<IConfiguration>();
var apiKey = appSettings.GetValue<string>(APIKEY);
if (!apiKey.Equals(extractedApiKey))
{
httpContext.Response.StatusCode = 401;
await httpContext.Response.WriteAsync("Wrong Auth Key : Unauthorized access");
return;
}
await _next(httpContext);
}
}
// Extension method used to add the middleware to the HTTP request pipeline.
public static class APIKeyMiddlewareExtensions
{
public static IApplicationBuilder UseAPIKeyMiddleware(this IApplicationBuilder builder)
{
return builder.UseMiddleware<APIKeyMiddleware>();
}
}
}
Code Explanation
The middleware will check the API key in the header and validate the key by extracting it from the header and compare with the key defined in code.
InvokeAsync method is defined in this middleware so that it will contain the main process, in our case, the main process will be to search and validate the x-authKey header name and value within the httpcontext request headers collection.
if (!context.Request.Headers.TryGetValue
(APIKEY, out var extractedApiKey))
{ context.Response.StatusCode = 401;
await context.Response.WriteAsync("Api Key missing");
return; }
Add the middleware in Program file
app.UseMiddleware<APIKeyMiddleware>();
Add the below auth Key in Appsetting file
"x-authKey": "uynjsykkloye679km@~556HHTrMolews"
Run the application, and test the API using POSTMAN without passing the x-authKey in header, you will get “Api Key missing” message in payload, as shown in the below figure.
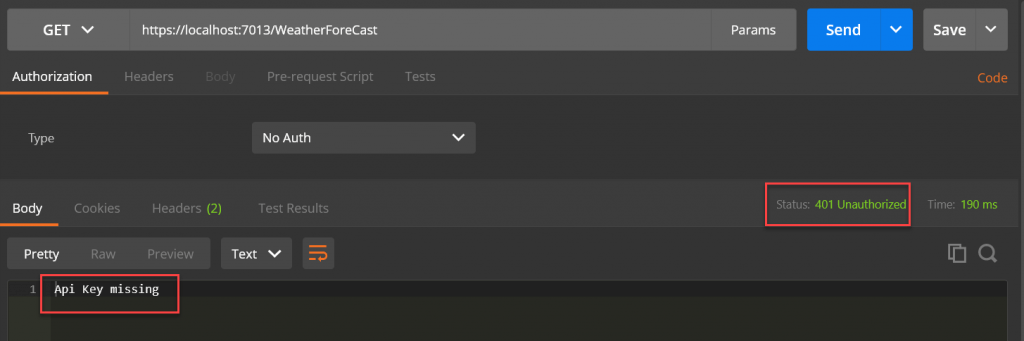
Let’s pass the right Auth key that we provided in Appsettings.json file like below and let’s see the result,
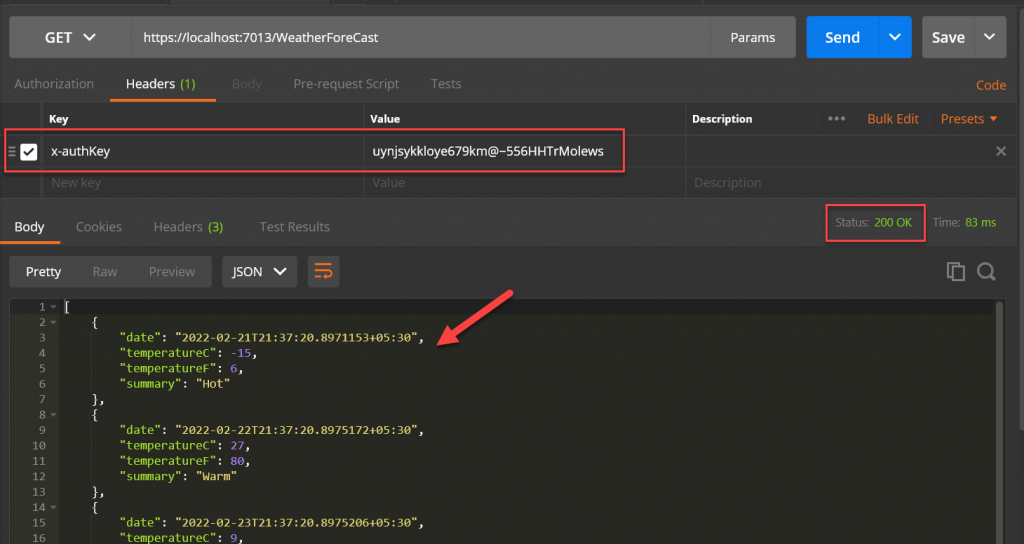
If we pass the wrong Auth key then it should block access the API key as below,
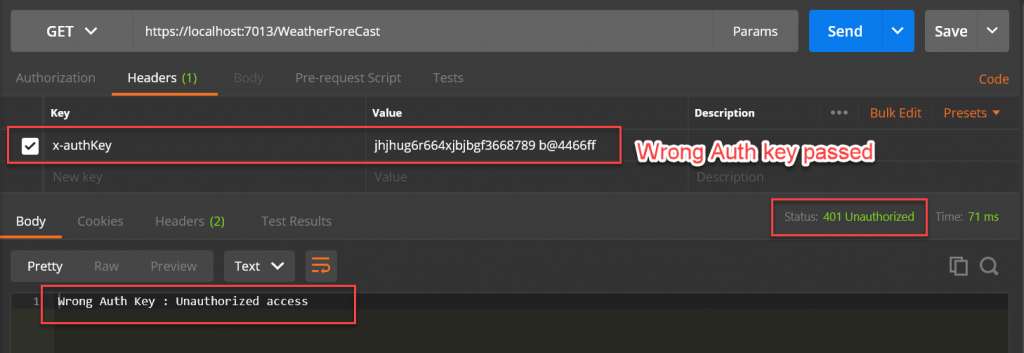
Conclusion
So far we discussed how to Using API Key Authentication To Secure ASP.Net Core Web API. Let us know if you have any additional question of this blog post.
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.
Great Tutorial! Sadly when i use the middleware i get cors problems. i enabled already all neccessary cors rules and without the middleware all is working fine.
This is what i get when enabling the middleware:
has been blocked by CORS policy: Response to preflight request doesn’t pass access control check: No ‘Access-Control-Allow-Origin’ header is present on the requested resource.
:44385/api/dinos:1 Failed to load resource: net::ERR_FAILED
Hey Carsten,
Please follow the below steps, I believe it will resolve your issue.
If you haven’t already, install the CORS nuget package.
Install-Package Microsoft.AspNetCore.Cors
In the ConfigureServices method of your startup.cs, add the CORS services.
public void ConfigureServices(IServiceCollection services)
{
services.AddCors(); // Make sure you call this previous to AddMvc
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
}
Then in your Configure method of your startup.cs, add the following :
public void Configure(IApplicationBuilder app, IHostingEnvironment env, ILoggerFactory loggerFactory)
{
// Make sure you call this before calling app.UseMvc()
app.UseCors(
options => options.WithOrigins(“http://example.com”).AllowAnyMethod()
);
app.UseMvc();
}