In this article we discuss about how to use ASP.Net Core Web API with MongoDB CRUD. It’s complete beginners tutorial to learn how we can integrate mongoDB in ASP.NET Core web API project. Now-a-days we hear a common word about NoSQL databases. These are being very strongly promoted by all vendors. One of the most common NoSQL databases these days is MongoDB. In this article we will discuss what a NoSQL database is, when it must be used and then we will look at an example to install, create and populate this database. Finally, we will look at how to access data from this database using a ASP.NET Core Web API. Also please read my previous article Integrate SMS in ASP.NET Core using Twilio.
At the end of article we will have hands on to learn how to use ASP.Net Core Web API with MongoDB CRUD.
What is a NoSQL database?
NoSQL database is a collection of documents with no real defined structure as in an RDBMS or SQL database. That means that we do not have any pre-defined columns as we do have in a SQL table. We simply store each row with its own number of columns or data fields as we may call them.
Advantages of NoSQL database?
- The common advantages of NoSQL database is the data for each document is in one place and faster to read.
- As there is no pre-defined structure we can store documents with different fields in one place and do not have to worry about adding new columns each time where most rows could contain NULLS as in a SQL database. Also, it saves us from creating new tables with relationships.
What is MongoDB?
MongoDB is one of the most common NoSQL databases. MongoDB can be downloaded and run from your desktop computer. It runs as a service. It also comes with a client called “MongoDB Compass Community”. This is a nice compact client which allows you to connect to the instance running, view the databases, collections, and documents inside it. It also allows you to create new databases, new collections inside the database, and new documents inside the collection using the JSON format. Being a Microsoft developer, another well known NoSQL database is Cosmos DB in addition to the tables storage mechanism in Microsoft Azure.
Installing MongoDB Server
MongoDB allows to host our own instance locally in our infrastructure. They offer Enterprise as well as community editions of their installable server. You will have to install both the server and a GUI Application (MongoDB Compass) to manage the MongoDB, very similar to SQL Management Studio.
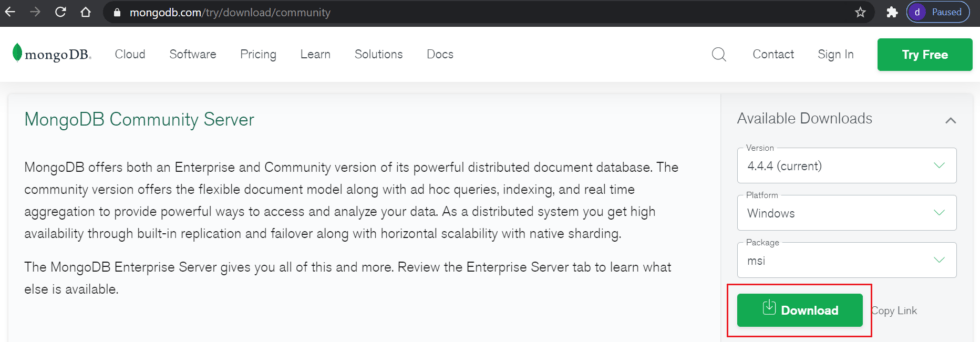
Once you have downloaded the MSI package, you can install it as shown below:
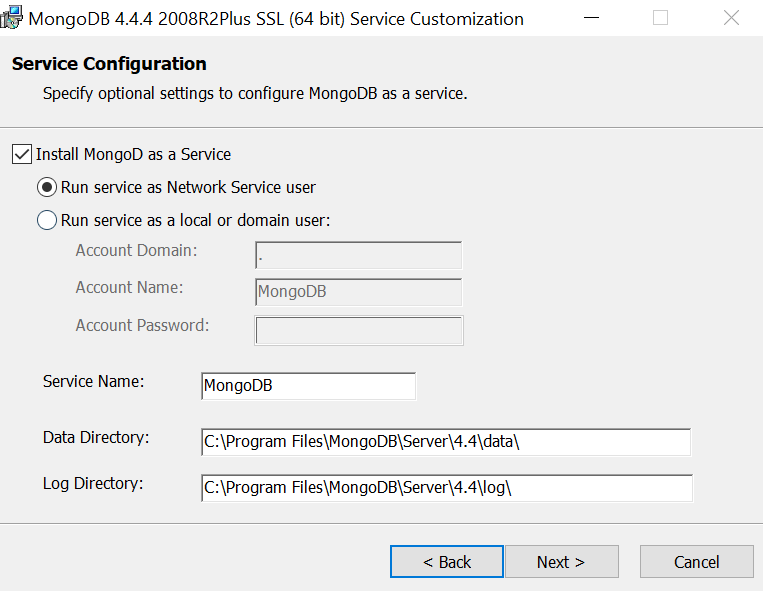
After installation, Open up MongoDB Compass. With Compass, you could specify the connection string to any authorized server and start browsing the database quite easily, But since we have no connection string with us, we can connect to the local MongoDB Server. For this you don’t have to specify the connection string, just press CONNECT.
This gets you connected to the localhost:27017 port where your default mongodb service is running.
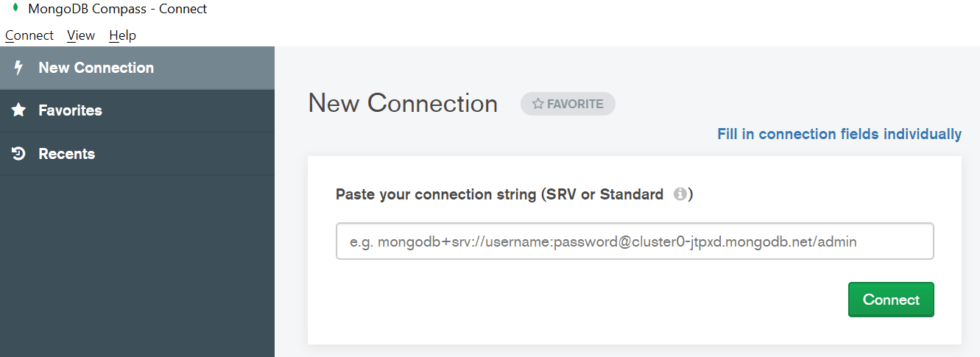
After click on connect you can navigate to this below screen.
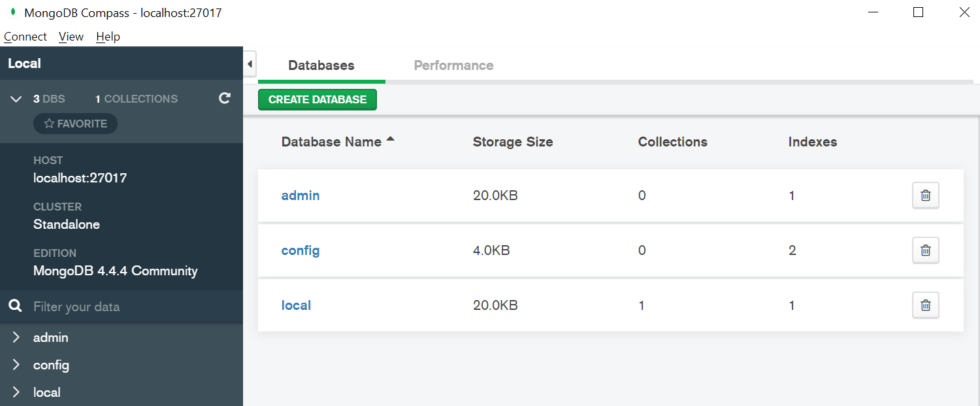
Create Database
Input database name as per your choice and set the collection name.
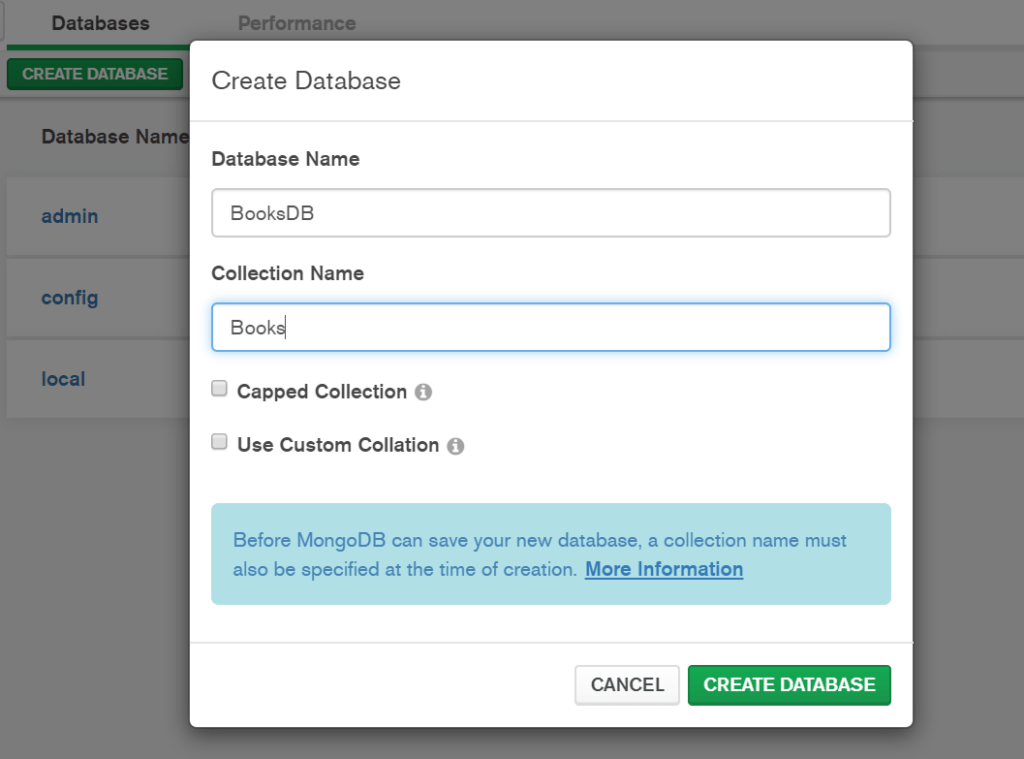
Let’s try to add a new Document to the Books Collection. Select the Books Collection and Add Data -> Insert Document.
{ "name": "Clean Architecture in .NET Core", "price" : 1000, "category" : "Computers", "author" : "JT" }
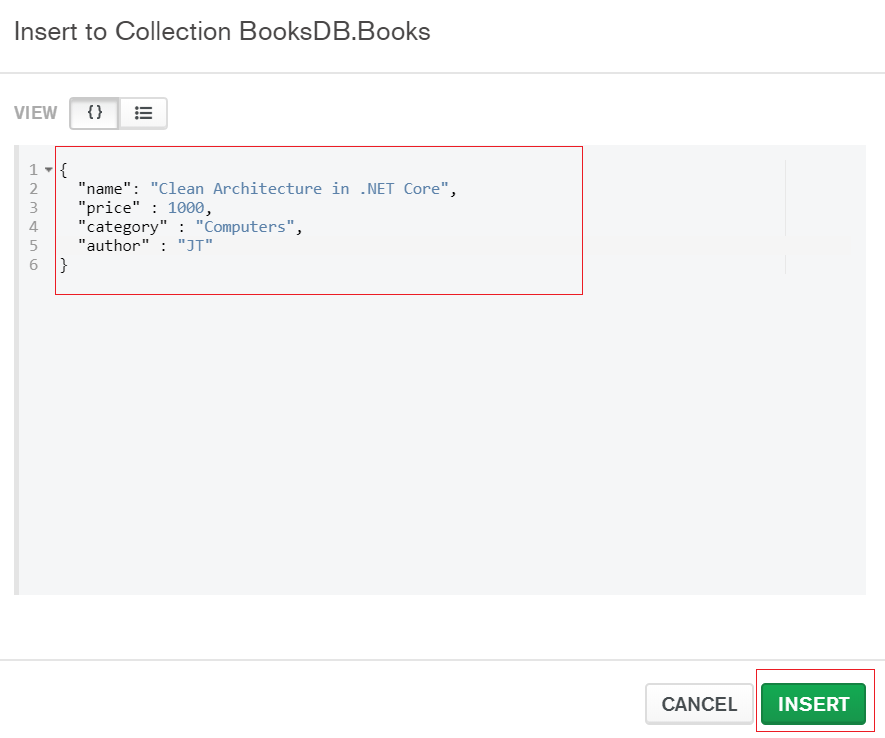
After click on insert button the JSON collection data is inserted in Books collection. You can note that the ID gets generated automatically with a random GUID.
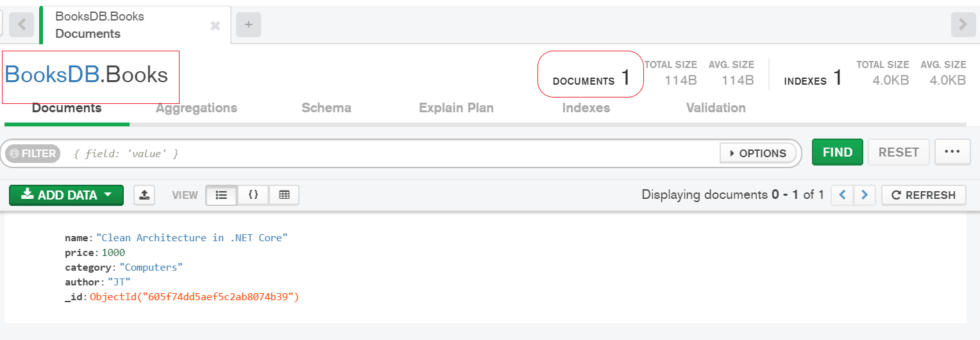
MongoDB In ASP.NET Core Web API – Getting Started
MongoDB is highly cross platform and can be seamlessly integrated with ASP.NET Core to take the maximum advantage of this awesome document based NOSQL Database. Let’s walkthrough step by step on working with MongoDB in ASP.NET Core. We will be setting up MongoDB Server and ultimately building a ASP.NET Core 5.0 WebAPI that does CRUD operations on a MongoDB Instances.
Create ASP.NET Core Web API project
Open Visual studio and create a new ASP.NET Core Web API and select target framework as 5.0 and also tick Open API support to enable swagger on our project. Using swagger we can test the API.
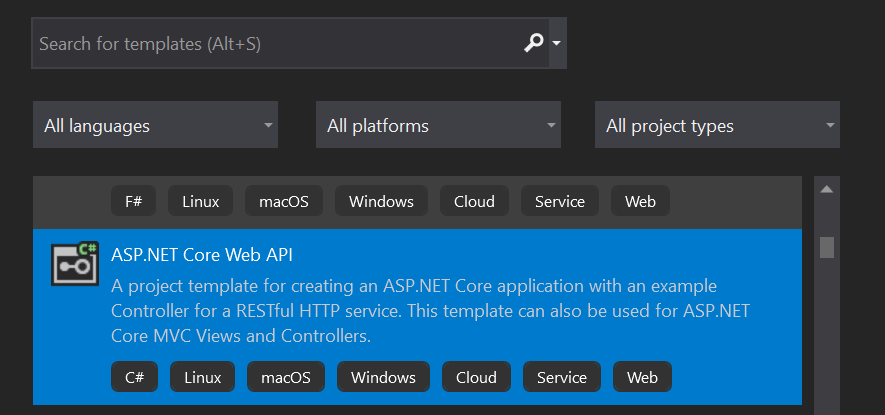
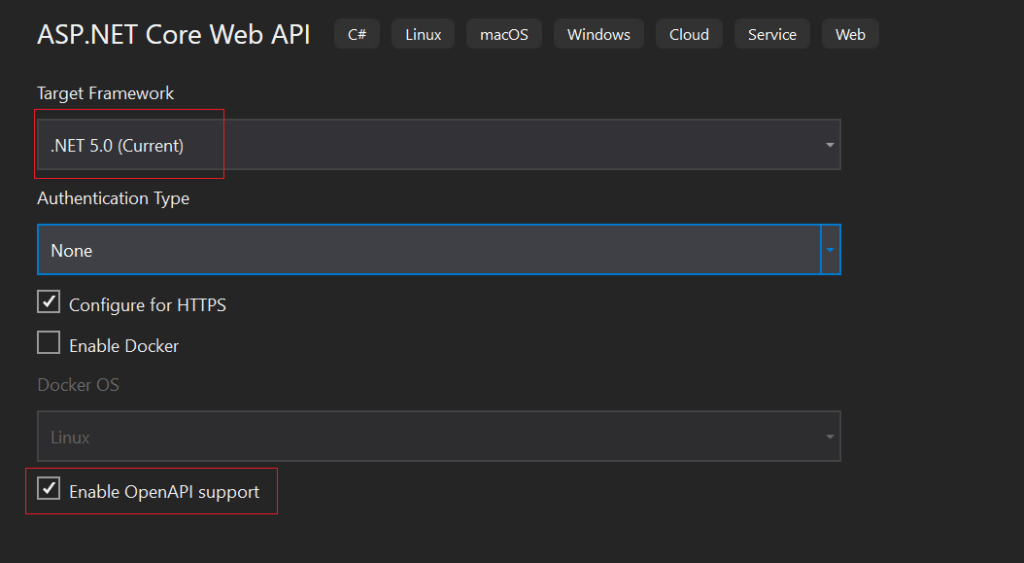
Add MongoDB Driver package in the project
Let’s add MongoDB package using package manager console.
Install-Package MongoDB.Driver
Create the Model class to manage CRUD
Let’s create the model class inside the Entity folder named as Books.
public class Books { [BsonId] [BsonRepresentation(BsonType.ObjectId)] public string id { get; set; } public string name { get; set; } public int price { get; set; } public string category { get; set; } public string author { get; set; } }
- Line no. 3&4 – For Id property, we set the primary key of MongoDB document, this is represented as [BsonId]. MongoDB recognizes object Id and not string/int as the primary key. Thus we have to manually set the property so that MongoDB converts the string to object Id.
- Line no. 5-9 – The properties of Books collection that we are added.
Add Connection string in Appsettings.json file
"BooksConnectionSettings": { "BooksCollectionName": "Books", "ConnectionString": "mongodb://localhost:27017", "DatabaseName": "BooksDB" }
- Line #1 : Connection setting name declare.
- Line #2-4 : We declared connection collection name, connection string and Database name.
Add a configuration class
Let’s add a configuration class inside configuration folder.
public class BooksConfiguration { public string BooksCollectionName { get; set; } public string ConnectionString { get; set; } public string DatabaseName { get; set; } }
Add the Service class to complete CRUD
Let’s create a Interface to hold the CRUD operation method.
public interface IBookService { Task<List<Books>> GetAllAsync(); Task<Books> GetByIdAsync(string id); Task<Books> CreateAsync(Books book); Task UpdateAsync(string id, Books book); Task DeleteAsync(string id); }
Create the Book service class and implement the IBookService inside it.
using CoreAPI.Mongo.Configuration; using CoreAPI.Mongo.Entity; using MongoDB.Driver; public class BookService: IBookService { private readonly IMongoCollection<Books> _book; private readonly BooksConfiguration _settings; public BookService(IOptions<BooksConfiguration> settings) { _settings = settings.Value; var client = new MongoClient(_settings.ConnectionString); var database = client.GetDatabase(_settings.DatabaseName); _book = database.GetCollection<Books>(_settings.BooksCollectionName); } public async Task<List<Books>> GetAllAsync() { return await _book.Find(c => true).ToListAsync(); } public async Task<Books> GetByIdAsync(string id) { return await _book.Find<Books>(c => c.id == id).FirstOrDefaultAsync(); } public async Task<Books> CreateAsync(Books book) { await _book.InsertOneAsync(book); return book; } public async Task UpdateAsync(string id, Books book) { await _book.ReplaceOneAsync(c => c.id == id, book); } public async Task DeleteAsync(string id) { await _book.DeleteOneAsync(c => c.id == id); } }
- Line #3 – Defining the MongoCollection of Books.
- Line #4 – Since we are using the IOptions Pattern to read our MongoDB Connection Configuration from the appsetting.json, we will have to inject the configuration class to the constructor.
- Line #8 – Injecting the IOptions of the Configuration to the constructor.
- Line #12-13 – Getting the Configuration Information and initializing the Books collection.
- Line #15-35 – Here we added the CRUD methods.
Add the Dependency Injection inside startup
Add the Dependency Injection inside ConfigureServices class on startup class.
public void ConfigureServices(IServiceCollection services) { services.AddControllers(); services.AddSwaggerGen(c => { c.SwaggerDoc("v1", new OpenApiInfo { Title = "CoreAPI.Mongo", Version = "v1" }); }); services.Configure<BooksConfiguration>(Configuration.GetSection("BooksConnectionSettings")); services.AddScoped<IBookService, BookService>(); }
- Line #4-7 – Swagger configuration.
- Line #8 – Configuration to read the connections.
- Line #9 – We inject the service class.
Create Books Controller to implement CRUD
public class BookController : ControllerBase { private readonly IBookService _bookService; public BookController(IBookService bookService) { _bookService = bookService; } [HttpGet] public async Task<IActionResult> GetAll() { return Ok(await _bookService.GetAllAsync()); } [HttpGet] public async Task<IActionResult> Get(string id) { var book = await _bookService.GetByIdAsync(id); if (book == null) { return NotFound(); } return Ok(book); } [HttpPost] public async Task<IActionResult> Create(Books book) { if (!ModelState.IsValid) { return BadRequest(); } await _bookService.CreateAsync(book); return Ok(book.id); } [HttpPut] public async Task<IActionResult> Update(string id, Books booksData) { var book = await _bookService.GetByIdAsync(id); if (book == null) { return NotFound(); } await _bookService.UpdateAsync(id, booksData); return NoContent(); } [HttpDelete] public async Task<IActionResult> Delete(string id) { var book = await _bookService.GetByIdAsync(id); if (book == null) { return NotFound(); } await _bookService.DeleteAsync(book.id); return NoContent(); } }
Line #3-6 – Inject the constructor dependency.
Testing the CRUD using Swagger
Let’s run the application and we should test the application using swagger.
Creating a New Book collection
Let’s create a POST request JSON like below and hit execute button.
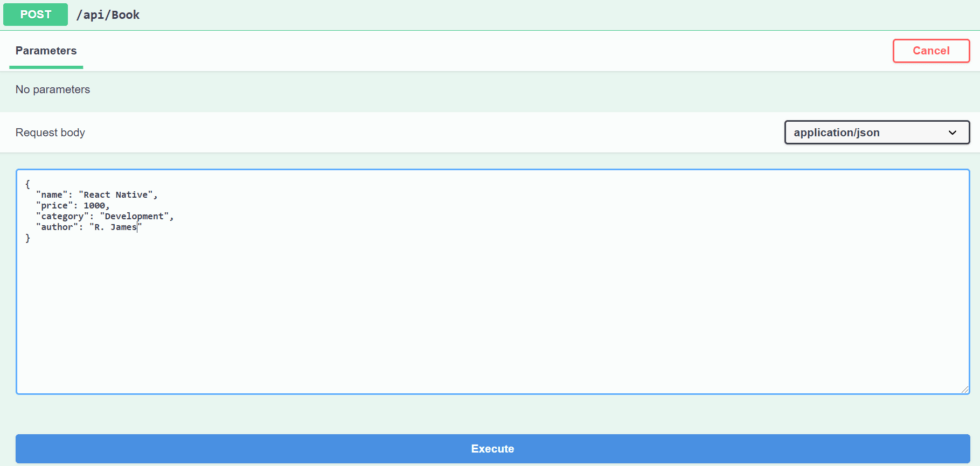
And you can see now the new book item has been added into our collection in MongDB database.
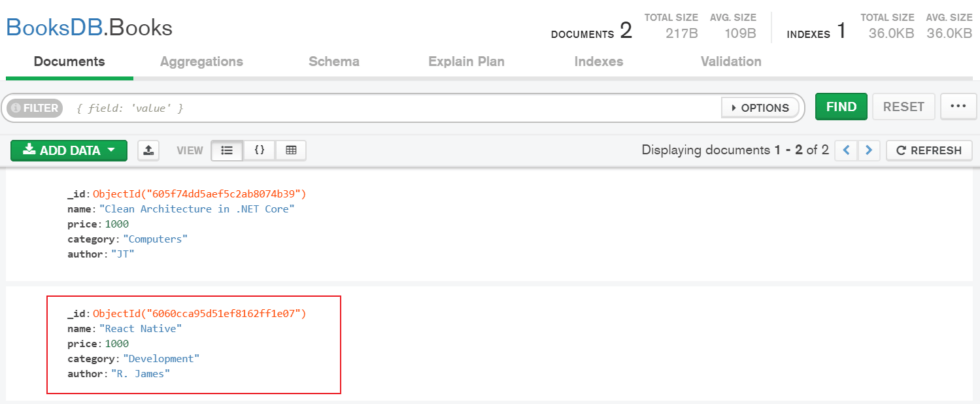
Read all book Collection
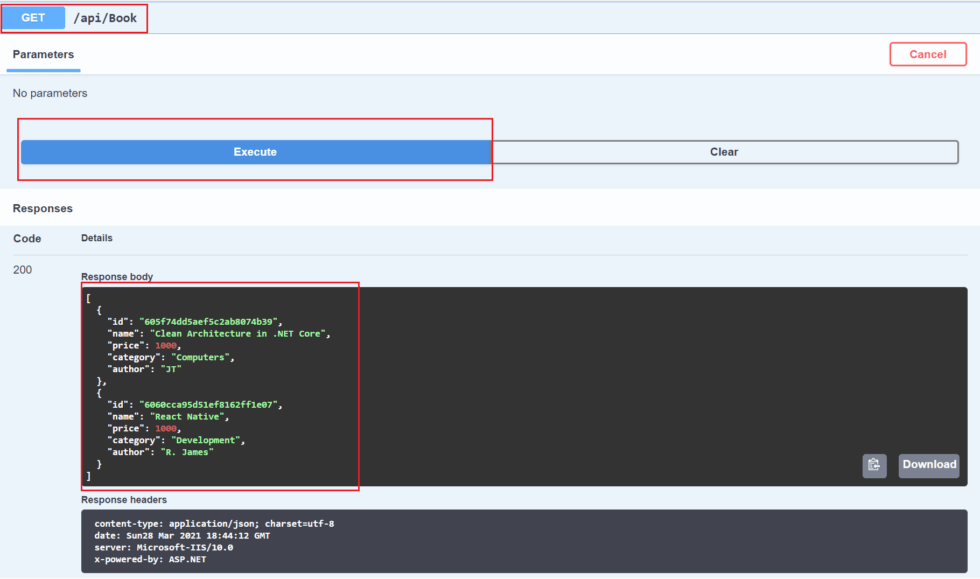
Get Books by Id
To get a Book by Id, send a GET Request to the same endpoint, but with an additional parameter, the Book id.
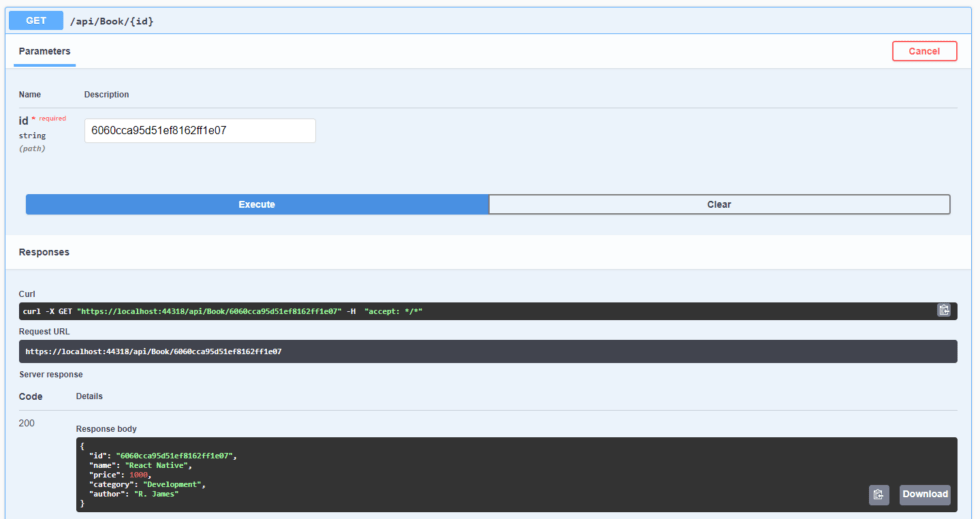
Update Books Data
Send the Book id as the parameter of the endpoint and also the Book data to be updated.
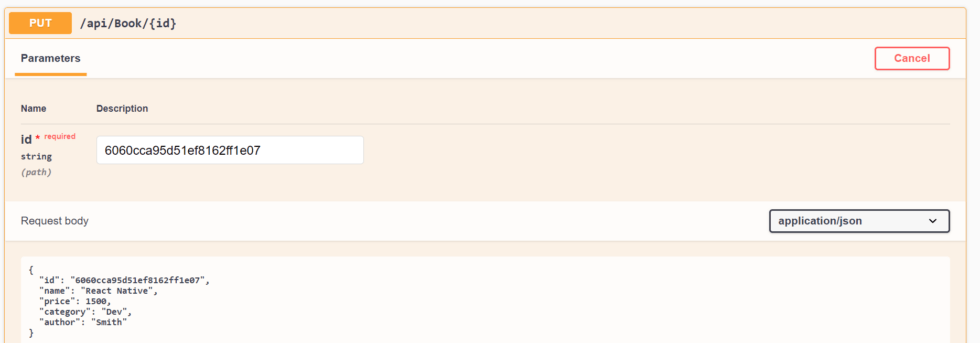
And you can see the update data is there.
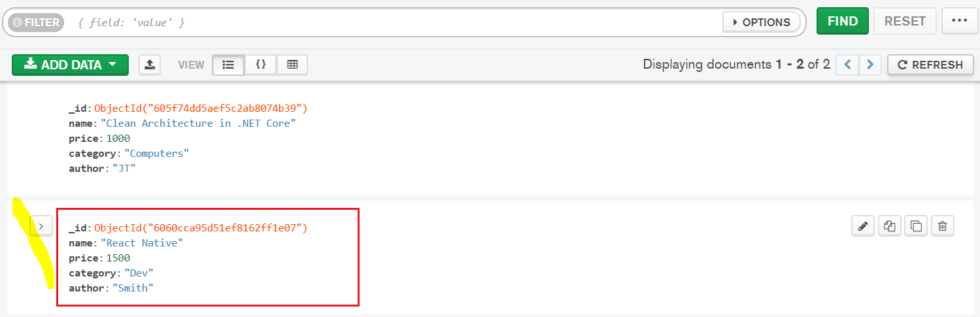
Delete Book Data
To delete a Books data, simply send in the id as the parameter with DELETE Request like below.
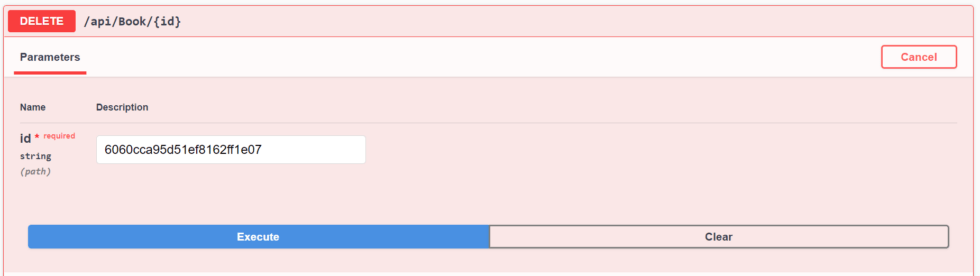
Now, let’s refresh the MongoDB compass and we can see that the book data is removed from the collection.
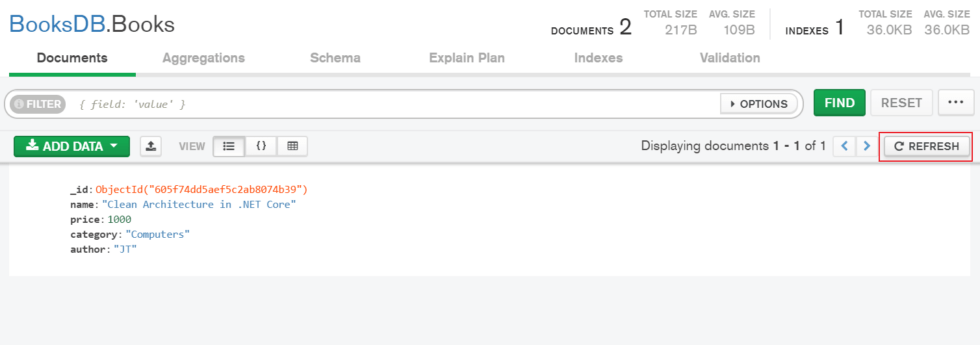
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Related Articles
- How to use Response Compression in .NET Core
- How to Integrate GraphQL in .Net Core
- Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular
- How to upload files to Azure Blob Storage using Asp.Net Core Web API
- How to store app secrets in ASP.NET Core using Secret Manager
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.