In this article, we will learn How to integrate GraphQL in .Net Core. To integrate GraphQL in a .NET Core application, we can use the HotChocolate
library, which is a popular GraphQL server implementation for .NET. We will discuss a step-by-step guide on how to integrate GraphQL into a .NET Core application using HotChocolate. Please read my previous article on Introduction to GraphQL.
Here we learn the basic setup for using GraphQL in a.NET Core application with HotChocolate. You can extend this by defining more complicated kinds, integrating with databases, and incorporating authentication as needed for your application. For more sophisticated features and options, the HotChocolate documentation is an excellent resource.
What helps to use GraphQL
- Instead of many requests, make one.
- Instead of numerous endpoints, use one.
- Versioning should be avoided.
- Instead of having “ALL” we want to have exactly what we desire.
- Avoid over- and under-fetching.
- Being mobile-friendly as opposed to desktop-friendly
- Instead of waiting for backend developers to add/remove fields, we can iterate quickly.
Creating ASP.Net Core Web API Project
- Launch the Visual Studio IDE and click on “Create new project”.
- In the “Create new project” window, select “ASP.NET Core Web API” from the template list.
- Click Next. In the “Configure your new project” window, specify the name and location for the new project and then click Create.
- In the “Create New ASP.NET Core Web API” window shown next, select .NET Core as the runtime and .NET 7.0 from the drop-down list at the top. Select “API” as the project template to create a new ASP.NET Core API application.
- Ensure that Authentication is set as “No Authentication” as we won’t be using authentication either and Click Create.
Install the HotChocolate NuGet package:
Run the following command to install the HotChocolate NuGet package:
dotnet add package HotChocolate.AspNetCore
Unlike REST, GraphQL doesn’t have many verbs to learn.
They are only available in three steps:
- Query: Equal to HTTP GET in REST
- Mutation: Equivalent to POST, PUT, DELETE, PATCH
- Subscription: No REST equivalent
Adding the class Query
Add the below class named as “Query” with the following code that returns the name.
public class Query
{
public string GetName()
{
return "Jayant Tripathy";
}
}
Modify the Program.cs file
using GraphQLDontNetCore.Models;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllers();
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
builder.Services.AddGraphQLServer().AddQueryType<Query>();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.UseAuthorization();
app.MapControllers();
app.MapGraphQL();
app.Run();
- To handle GraphQL queries, we need to build a GraphQL server. ( AddGraphQLServer)
- To define the exact type, we need to provide our class as a query type ( AddQueryType). To run and map queries, we need to use MapGraphQL() middleware.
Run the application using /graphQL
the route we can see this screen like below, then click on Create Document
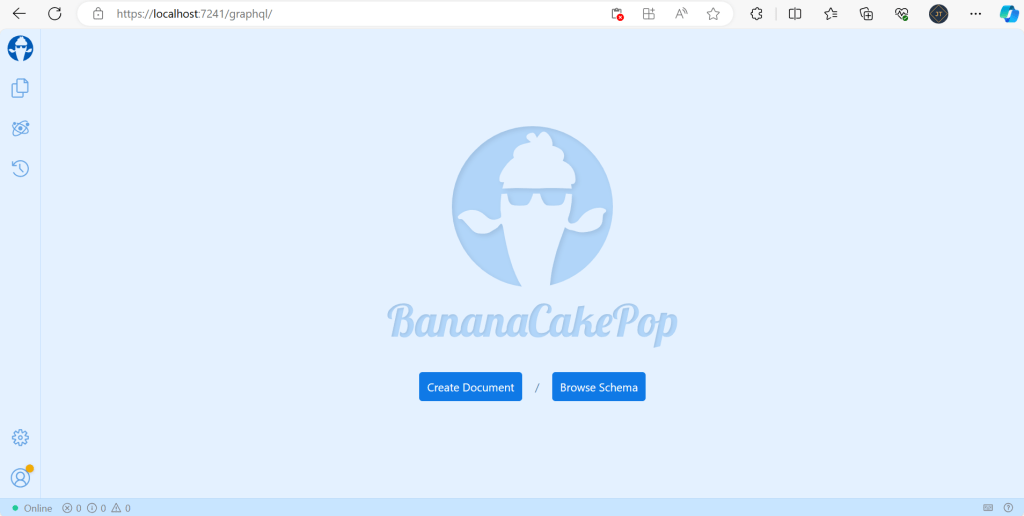
We have one Query object in our schema with a “name
” property.
It’s a slightly modified version of our “GetName()
” method.
HotChocolate has the ability to remove verbs from the prefix. As a result, it eliminates “GET” and other verbs from the “field” automatically.
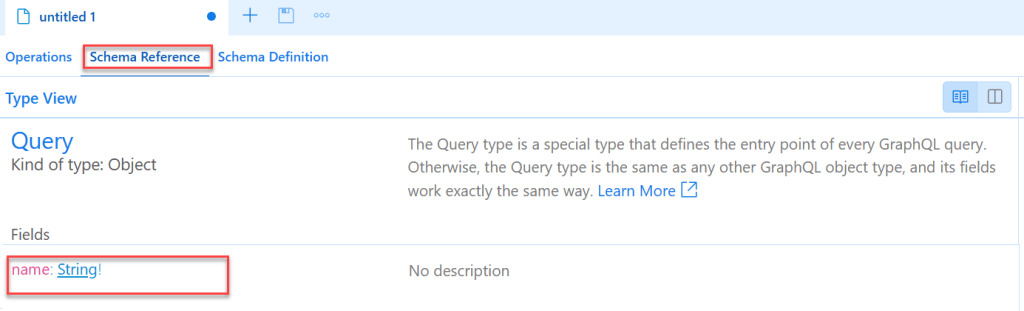
Let’s Run the query like below screen we can see the result output like below.
- Why did I refer to it as a field – Because they are pure properties/fields from the standpoint of GraphQL.
- Every method in a Query class will be converted into a field.
- C# methods are resolvers, and they assist us in determining the field we need to access.
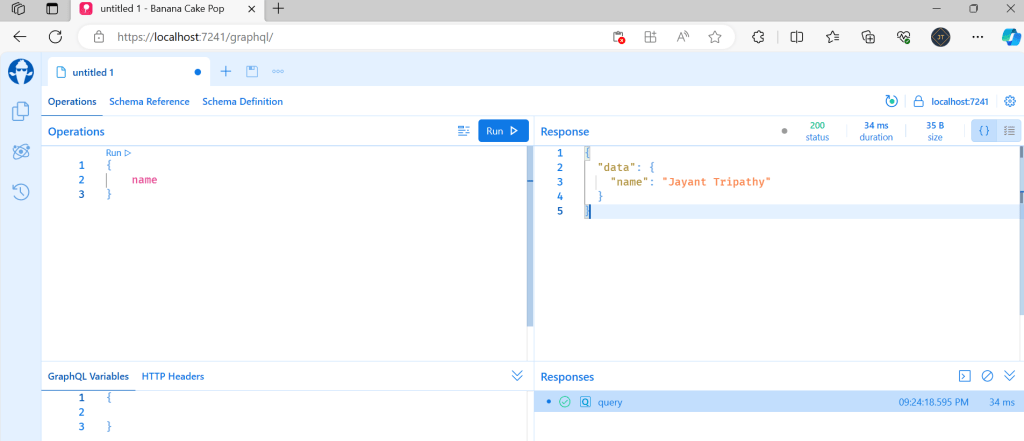
Let’s extened the Query class by adding another resoolver class into it.
public class Query
{
public string GetName()
{
return "Jayant Tripathy";
}
public IEnumerable<Country> GetCountries()
{
return new List<Country>
{
new Country(1,"India"),
new Country(2,"USA"),
new Country(3,"UK"),
new Country(4,"Austrillia"),
new Country(5,"Newzeland"),
new Country(6,"Russia")
};
}
public record Country(int Id, string Name);
}
- We now have a differnt data type named
Country
, and our method returns a list of them. In the preceding example, all methods are resolvers. - This “annotation-based approach” allows us to go into the specifics of scheme definition and “extract” necessary type models from C# code.
- In general, even without delving into type details, using GraphQL with hotchocolate is a piece of cake. However, in the future, If you need to modify more GraphQL endpoints, you will run into challenges that require a good type comprehension.
- On below we can see that two resolvers,
Name
andCountries
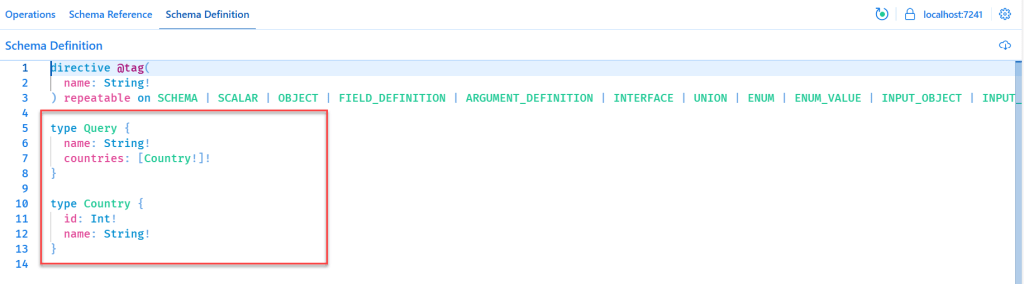
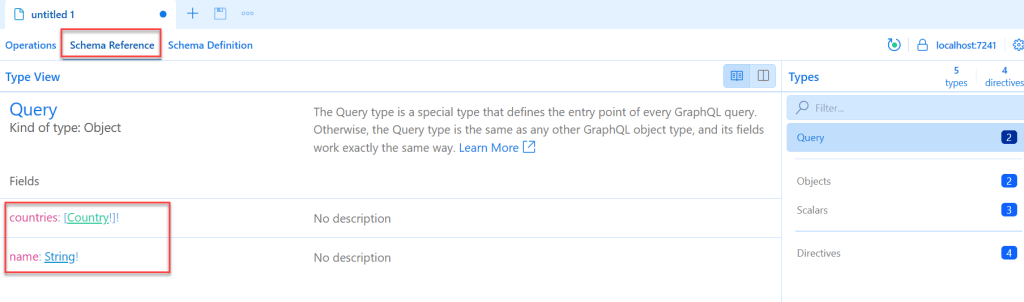
Run the application we can see the output like below, So in one call we can get two resolver methods. name & countries
.
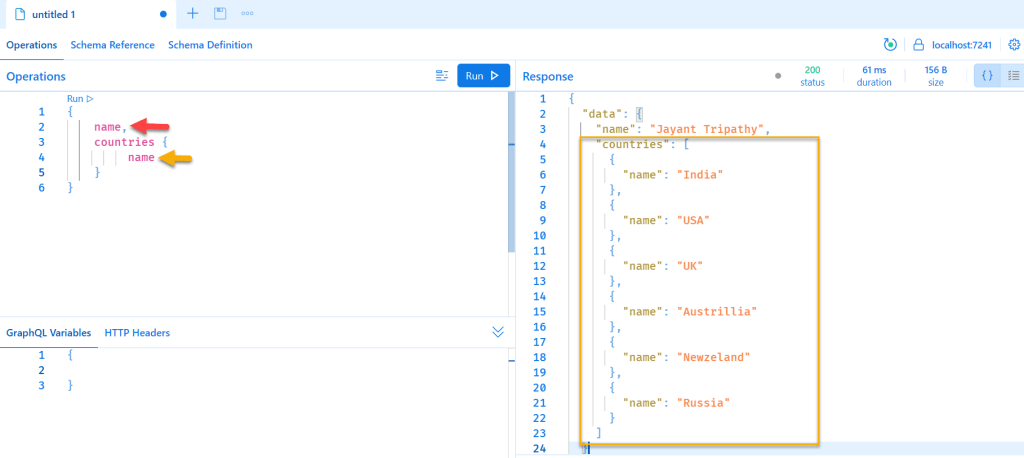
Request
{
name,
countries {
name
}
}
Response
{
"data": {
"name": "Jayant Tripathy",
"countries": [
{
"name": "India"
},
{
"name": "USA"
},
{
"name": "UK"
},
{
"name": "Austrillia"
},
{
"name": "Newzeland"
},
{
"name": "Russia"
}
]
}
}
GraphQL functions
The syntax of GraphQL is entirely about operations. “Operations” refers to all server requests. You can perform queries, mutations, and subscriptions. In the preceding examples, you may wonder where the operation is.
In that scenario, we’ll be using root curly brackets. Because GraphQL simplifies querying, we may avoid using the operation syntax directly. We primarily employ operation syntax.
- In the case of Query, if we wish to introduce some variable(s),
- Extensive logging. To record the precise query name
- To define the same endpoint query with various fields
{
name,
countries {
name
}
}
Is Equal to
query {
name,
countries {
name
}
}
Conclusion
In this article, we discussed How to integrate GraphQL in .Net Core and step by step implement GraphQL in .Net Core. GraphQL uses HTTP POST by default for both Query and Mutation. WebSockets are mostly used by GraphQL Subscription. However, server-side events can also be used for them.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Related Articles
- .NET 8 Authentication with Identity in a Web API using Bearer Tokens and Cookies
- How to convert Text To Speech With Azure Cognitive Services using Angular and .Net Core
- CRUD operation using the repository pattern with .Net 8, Ef-Core, and MySQL
- How to use Response Compression in .NET Core
- How to Integrate GraphQL in .Net Core
- Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular
- How to upload files to Azure Blob Storage using Asp.Net Core Web API
- How to store app secrets in ASP.NET Core using Secret Manager
- Logging into Azure App Service with ASP.Net Core
- Integrate Paging in ASP.Net Core Web API- Beginner’s Guide
SUPPORT ME
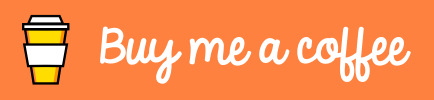