In this article I will explain you how to create Autocomplete Using JQuery, Ajax & WCF in MVC. I am creating application using VS 2017. Create a MVC Application. We will include the WCF Service on the said project. Please read my previous article How to Create ASP.NET Core MVC application using VS Code.
Creating ASP.Net MVC application
Create a MVC Application. We will include the WCF Service on the said project. Save your project with the specified location.
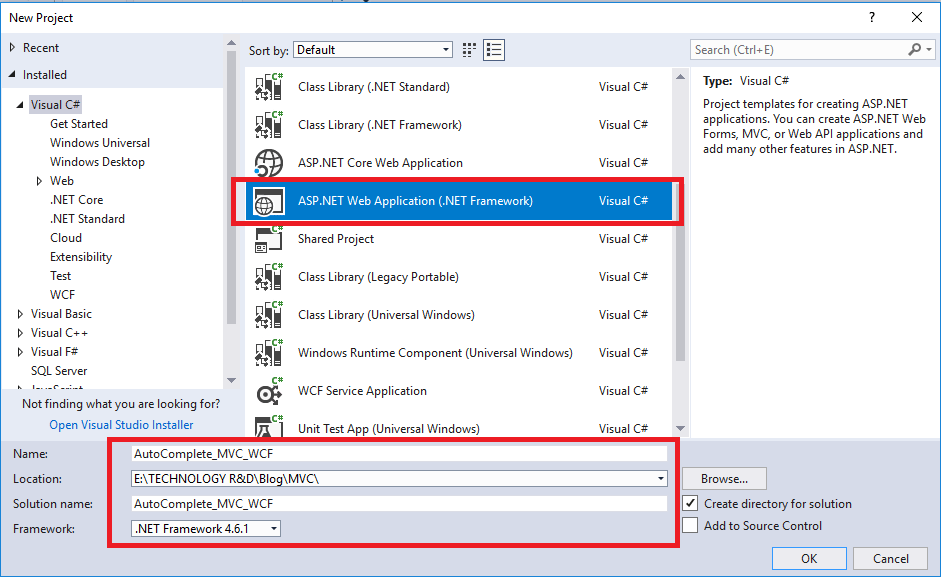
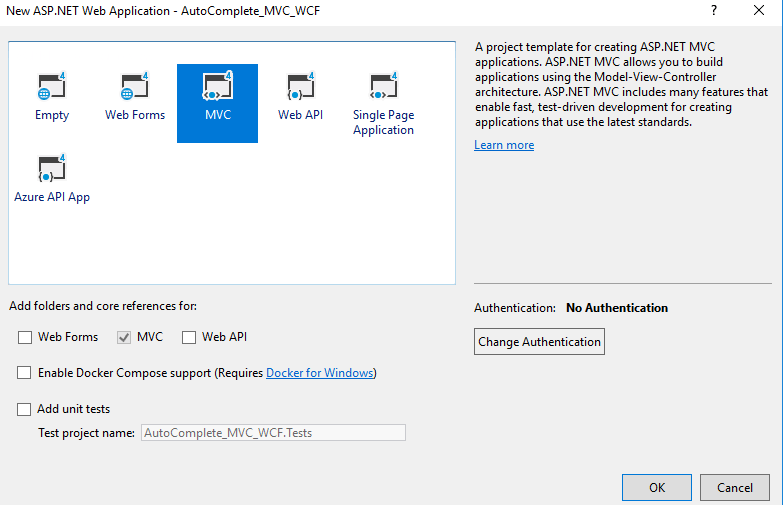
Creating WCF Service and Database script
The MVC application is ready now we create the WCF Service. For creating the Service, Right Click on the “Models” folder and the WCF Service class like below.
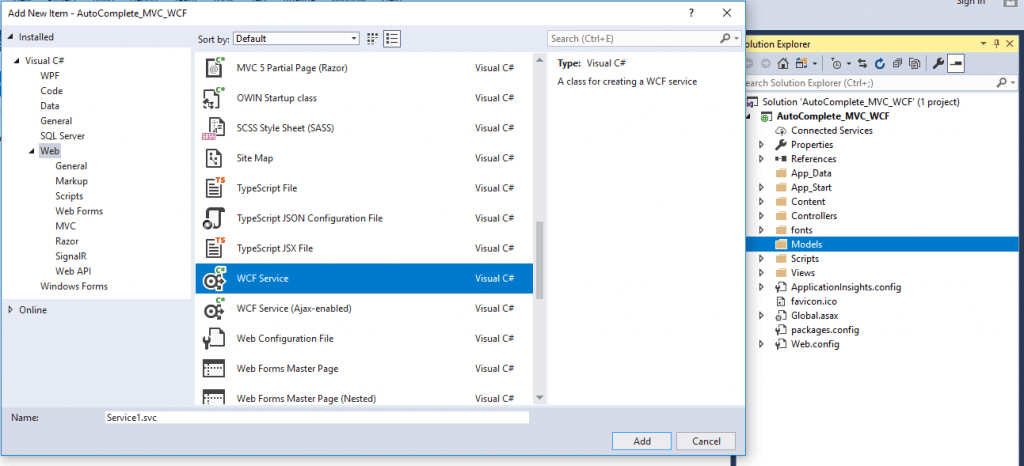
Add the connection String in the Web.Config File and Create a database with Dummy Data look like below.
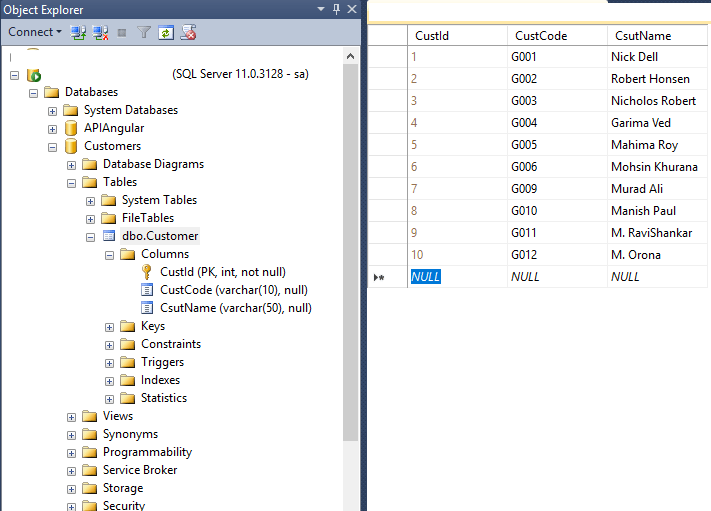
USE [Customers] GO /****** Object: Table [dbo].[Customer] Script Date: 7/1/2019 7:39:02 PM ******/ SET ANSI_NULLS ON GO SET QUOTED_IDENTIFIER ON GO SET ANSI_PADDING ON GO CREATE TABLE [dbo].[Customer]( [CustId] [int] IDENTITY(1,1) NOT NULL, [CustCode] [varchar](10) NULL, [CsutName] [varchar](50) NULL, CONSTRAINT [PK_Customer] PRIMARY KEY CLUSTERED ( [CustId] ASC )WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY] ) ON [PRIMARY] GO SET ANSI_PADDING OFF GO
In “Iservice1.cs” Interface method add the Service method and in “OperationContarct” add the below Invoke method as well. “System.ServiceModel.Web” reference must add.
namespace AutoComplete_MVC_WCF.Models { // NOTE: You can use the "Rename" command on the "Refactor" menu to change the interface name "IService1" in both code and config file together. [ServiceContract] public interface IService1 { [OperationContract] [WebInvoke(Method = "POST", ResponseFormat = WebMessageFormat.Json)] string GetCustomers(string AutoData); } }
Implement the method in “Service.cs” like below.
using System; using System.Collections.Generic; using System.Configuration; using System.Data.SqlClient; using System.Linq; using System.Runtime.Serialization; using System.ServiceModel; using System.ServiceModel.Activation; using System.Text; using System.Web.Script.Serialization; namespace AutoComplete_MVC_WCF.Models { // NOTE: You can use the "Rename" command on the "Refactor" menu to change the class name "Service1" in code, svc and config file together. // NOTE: In order to launch WCF Test Client for testing this service, please select Service1.svc or Service1.svc.cs at the Solution Explorer and start debugging. [AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)] public class Service1 : IService1 { public string GetCustomers(string Prefix) { List<object> customers = new List<object>(); using (SqlConnection conn = new SqlConnection()) { conn.ConnectionString = ConfigurationManager.ConnectionStrings["ConnectionString"].ConnectionString; using (SqlCommand cmd = new SqlCommand()) { cmd.CommandText = "SELECT CustCode,CsutName FROM dbo.Customer where " + "CsutName like @prefix + '%'"; cmd.Parameters.AddWithValue("@prefix", Prefix); cmd.Connection = conn; conn.Open(); using (SqlDataReader sdr = cmd.ExecuteReader()) { while (sdr.Read()) { customers.Add(new { CustCode = sdr["CustCode"], CsutName = sdr["CsutName"] }); } } conn.Close(); } return (new JavaScriptSerializer().Serialize(customers)); } } } }
Code Explanation
In this service call we call the database and fetch the customer details.
Now Configure the Service in “Web.config” File.
<system.serviceModel> <behaviors> <serviceBehaviors> <behavior name="ServiceBehavior"> <serviceMetadata httpGetEnabled="true"/> <serviceDebug includeExceptionDetailInFaults="true"/> </behavior> </serviceBehaviors> <endpointBehaviors> <behavior name="ServiceAspNetAjaxBehavior"> <enableWebScript/> </behavior> </endpointBehaviors> </behaviors> <serviceHostingEnvironment aspNetCompatibilityEnabled="true"/> <services> <service behaviorConfiguration="ServiceBehavior" name="AutoComplete_MVC_WCF.Models.Service1"> <endpoint address="" binding="webHttpBinding" contract="AutoComplete_MVC_WCF.Models.IService1" behaviorConfiguration="ServiceAspNetAjaxBehavior"> <identity> <dns value="localhost"/> </identity> </endpoint> <endpoint address="mex" binding="mexHttpBinding" contract="IMetadataExchange"/> </service> </services> </system.serviceModel>
Code Explanation
We have set the endpoint, the endpoint is AutoComplete_MVC_WCF.Models.IService1
The WCF Service is ready now, we need to set ready the Html. Already we have the MVC application project with Default “HomeController” with view “Index” page so we need to add the below html code. We use “CDN”.
@{ ViewBag.Title = "Home Page"; } <html xmlns="http://www.w3.org/1999/xhtml"> <head id="Head1" runat="server"> <title></title> <style> table, th, td { border: 1px solid grey; border-collapse: collapse; padding: 5px; } table tr:nth-child(odd) { background-color: #f1f1f1; } table tr:nth-child(even) { background-color: #ffffff; } </style> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <link href="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8.1/themes/base/jquery-ui.css" rel="stylesheet" type="text/css" /> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8.1/jquery-ui.min.js"></script> <script type="text/javascript"> $(document).ready(function () { $("#search12").click(function () { $.ajax({ type: "POST", contentType: "application/json; charset=utf-8", url: "../Models/Service1.svc/GetCustomers", data: '{"AutoData": "' + $("#AutoData").val() + '"}', processData: false, dataType: "json", success: function (response) { var customers = eval(response.d); var html = ""; html += ` <table > <tr> <td>ID</td> <td>Name</td> </tr>`; $.each(customers, function () { html += '<tr><td>' + this.CustCode + '</td><td>' + this.CsutName + '</td></tr>'; }); html += ` </table>`; $("#results").html(html == "" ? "No results" : html); }, error: function (a, b, c) { alert(a.responseText); } }); }); }); </script> </head> <body> <form id="form1" runat="server"> <br /> <input type="text" id="AutoData" /> <input id="search12" type="button" value="Search" /> <div id="results"></div> </form> </body> </html>
Code Explanation
We take here a Textbox and Button, When type any letter and click on the button then call AJAX method.
Here in POST method we call the WCF Service, ../Models/Service1.svc/GetCustomers
Then it return back the customer details according to the typed letter, autocomplete will do the rest and display like below.
Now run the application and when we put any related keyword and hit enter in Search button the Output look like below.
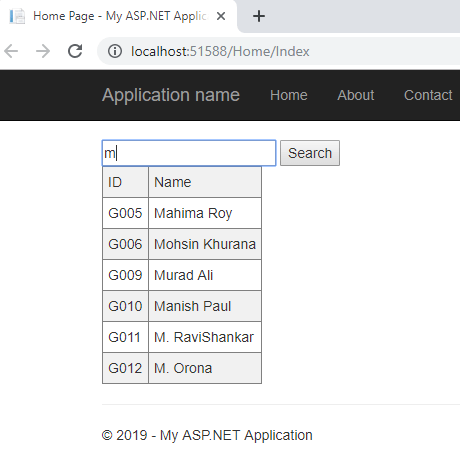
Conclusion
In this article we will see how to create Search box using Jquery and WCF. This article also I shared earlier in C-Sharp corner Creating Autocomplete Using JQuery, Ajax & WCF in ASP.Net