In this article we will learn JavaScript form validation, JavaScript provides facility to validate the form on the client-side so data processing will be faster than server-side validation. Most of the web developers prefer JavaScript form validation. Please read my previous article Redis Caching in ASP.NET Core.
Form validation normally used to occur at the server, after the client had entered all the necessary data and then pressed the Submit button. If the data entered by a client was incorrect or was simply missing, the server would have to send all the data back to the client and request that the form be resubmitted with correct information. This was really a lengthy process which used to put a lot of burden on the server, so that JavaScript provides a way to validate form’s data on the client’s computer before sending it to the web server.
JavaScript Form Validation Example
In this example, we are going to validate the name, email and password. Here we create a HTML file with style and JavaScript that responsible for validate the form. You can see the demo on this link.
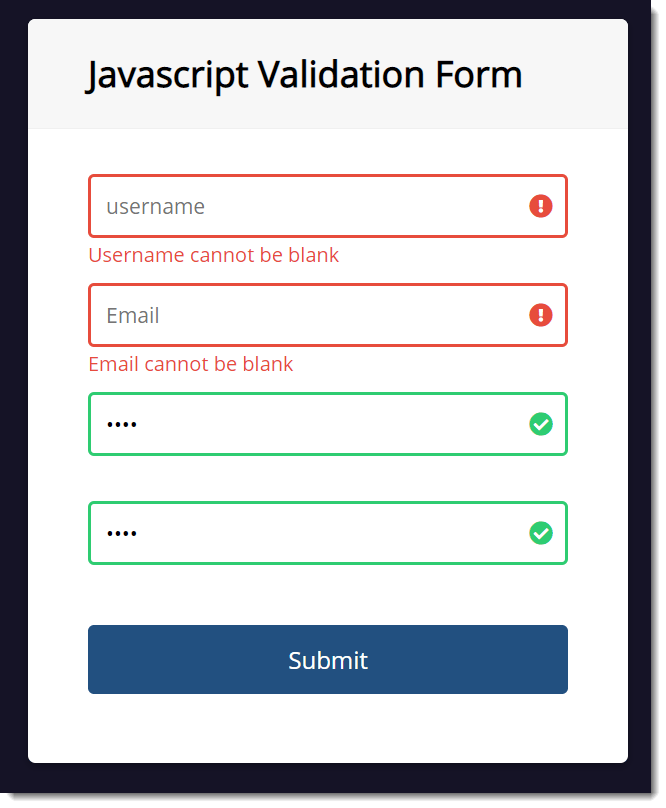
Forms are used in webpages for the user to enter their required details that further send it to the server for processing. A form is also known as a web form or HTML form.
Index.html
<html> <head> <link rel="stylesheet" media="all" href="/style.css" /> <link rel="stylesheet" media="all" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.10.2/css/all.min.css" /> <script src="/validation.js" async defer crossorigin="anonymous"></script> </head> <body> <div class="container"> <div class="header"> <h2>Javascript Validation Form</h2> </div> <form id="form" class="form"> <div class="form-control"> <input type="text" placeholder="username" id="username" /> <i class="fas fa-check-circle"></i> <i class="fas fa-exclamation-circle"></i> <small>Error message</small> </div> <div class="form-control"> <input type="email" placeholder="Email" id="email" /> <i class="fas fa-check-circle"></i> <i class="fas fa-exclamation-circle"></i> <small>Error message</small> </div> <div class="form-control"> <input type="password" placeholder="Password" id="password" /> <i class="fas fa-check-circle"></i> <i class="fas fa-exclamation-circle"></i> <small>Error message</small> </div> <div class="form-control"> <input type="password" placeholder="Confirm Password" id="cnfpassword" /> <i class="fas fa-check-circle"></i> <i class="fas fa-exclamation-circle"></i> <small>Error message</small> </div> <button>Submit</button> </form> </div> </body> </html>
Code Explanation
- Line #3-5 : Here we use stylesheet and javascript file
- Rest are the forms element.
Style.css
@import url('https://fonts.googleapis.com/css?family=Muli&display=swap'); @import url('https://fonts.googleapis.com/css?family=Open+Sans:400,500&display=swap'); * { box-sizing: border-box; } body { background-color: #151326; font-family: 'Open Sans', sans-serif; display: flex; align-items: center; justify-content: center; min-height: 100vh; margin: 0; } .container { background-color: #fff; border-radius: 5px; box-shadow: 0 2px 5px rgba(0, 0, 0, 0.3); overflow: hidden; width: 400px; max-width: 100%; } .header { border-bottom: 1px solid #f0f0f0; background-color: #f7f7f7; padding: 20px 40px; } .header h2 { margin: 0; } .form { padding: 30px 40px; } .form-control { margin-bottom: 10px; padding-bottom: 20px; position: relative; } .form-control label { display: inline-block; margin-bottom: 5px; } .form-control input { border: 2px solid #f0f0f0; border-radius: 4px; display: block; font-family: inherit; font-size: 14px; padding: 10px; width: 100%; } .form-control input:focus { outline: 0; border-color: #777; } .form-control.success input { border-color: #2ecc71; } .form-control.error input { border-color: #e74c3c; } .form-control i { visibility: hidden; position: absolute; top: 13px; right: 10px; } .form-control.success i.fa-check-circle { color: #2ecc71; visibility: visible; } .form-control.error i.fa-exclamation-circle { color: #e74c3c; visibility: visible; } .form-control small { color: #e74c3c; position: absolute; bottom: 0; left: 0; visibility: hidden; } .form-control.error small { visibility: visible; } .form button { background-color: #225080; border: 2px solid #225080; border-radius: 4px; color: #fff; display: block; font-family: inherit; font-size: 16px; padding: 10px; margin-top: 20px; width: 100%; cursor: pointer; } .floating-btn { border-radius: 26.5px; background-color: #001F61; border: 1px solid #001F61; box-shadow: 0 16px 22px -17px #03153B; color: #fff; cursor: pointer; font-size: 16px; line-height: 20px; padding: 12px 20px; position: fixed; bottom: 20px; right: 20px; z-index: 999; } .floating-btn:hover { background-color: #ffffff; color: #001F61; } .floating-btn:focus { outline: none; } .floating-text { background-color: #001F61; border-radius: 10px 10px 0 0; color: #fff; font-family: 'Muli'; padding: 7px 15px; position: fixed; bottom: 0; left: 50%; transform: translateX(-50%); text-align: center; z-index: 998; } .floating-text a { color: #FF7500; text-decoration: none; } @media screen and (max-width: 480px) { .social-panel-container.visible { transform: translateX(0px); } .floating-btn { right: 10px; } }
Validation.js
const form = document.getElementById('form'); const username = document.getElementById('username'); const email = document.getElementById('email'); const password = document.getElementById('password'); const cnfpassword = document.getElementById('cnfpassword'); form.addEventListener('submit', e => { e.preventDefault(); checkInputs(); }); function checkInputs() { const usernameValue = username.value.trim(); const emailValue = email.value.trim(); const passwordValue = password.value.trim(); const cnfpasswordValue = cnfpassword.value.trim(); if(usernameValue === '') { setErrorFor(username, 'Username cannot be blank'); } else { setSuccessFor(username); } if(emailValue === '') { setErrorFor(email, 'Email cannot be blank'); } else if (!isEmail(emailValue)) { setErrorFor(email, 'Not a valid email'); } else { setSuccessFor(email); } if(passwordValue === '') { setErrorFor(password, 'Password cannot be blank'); } else { setSuccessFor(password); } if(cnfpasswordValue === '') { setErrorFor(cnfpassword, 'Confirm Password cannot be blank'); } else if(passwordValue !== cnfpasswordValue) { setErrorFor(cnfpassword, 'Passwords does not match'); } else{ setSuccessFor(cnfpassword); } } function setErrorFor(input, message) { const formControl = input.parentElement; const small = formControl.querySelector('small'); formControl.className = 'form-control error'; small.innerText = message; } function setSuccessFor(input) { const formControl = input.parentElement; formControl.className = 'form-control success'; } function isEmail(email) { return /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/.test(email); }
Code Explanation
- Line #1-5 : Hold the form fields value.
- When submit button is hit it call the checkInputs method and check the validation accordingly.
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.