These questions are guidelines to assess the candidate about ASP.Net MVC Interview Questions. These interview question covers basic to advance and will help you to prepare for the interviews, quick revision and provide strength to your technical skills.
What is MVC?
MVC stands for Model-View-Controller. It is a software design pattern which was introduced in 1970s. Also, MVC pattern forces a separation of concerns, it means domain model and controller logic are decoupled from user interface (view). As a result maintenance and testing of the application become simpler and easier.
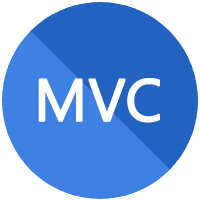
Explain MVC design pattern?
MVC design pattern splits an application into three main aspects: Model, View and Controller
Model – The Model represents a set of classes that describe the business logic i.e. business model as well as data
access operations i.e. data model. It also defines business rules for data means how the data can be changed and
manipulated.
View – The View represents the UI components like CSS, jQuery, html etc. It is only responsible for displaying the
data that is received from the controller as the result. This also transforms the model(s) into UI.
Controller – The Controller is responsible to process incoming requests. It receives input from users via the View,
then process the user’s data with the help of Model and passing the results back to the View. Typically, it acts as
the coordinator between the View and the Model.
Today, this pattern is used by many popular framework like as Ruby on Rails, Spring Framework, Apple iOS
Development and ASP.NET MVC.
What is Domain Driven Design and Development?
Domain-Driven Design (DDD) is a collection of principles and patterns that help developers to take design decisions to develop elegant systems for different domains. It is not a technology or methodology.
The main components of DDD are: Entity, Value Object, Aggregate, Service and Repository.
- Entity– An object that has an identity- it is unique within the system, like Customer, Employee etc.
- Value Object– An object that has no identity within the system like Rate, State etc.
- Note– A value object can become an entity depending on the situation.
- Aggregate– An aggregate root is a special kind of entity that consumers refer to directly. All consumers of then aggregate root are called as aggregate. The aggregate root guarantees the consistency of changes being made within the aggregate.
- Service– A service is a way of dealing with actions, operations and activities within your application.
- Repository- A repository is responsible to store and to retrieve your data. It is not a concern how and where data will be persist. So, it can be SQL server, oracle, xml, text file or anything else. Repository is not a Data Access Layer but it refers to a location for storage, often for safety or preservation.
What is MVVM pattern?
MVVM stands for Model-View-View Model
. This pattern supports two-way data binding between view and View model. This enables automatic propagation of changes, within the state of view model to the View. Typically, the view model uses the observer pattern to notify changes in the view model to model.
Model – The Model represents a set of classes that describes the business logic and data. It also defines business rules for data means how the data can be changed and manipulated.
View – The View represents the UI components like CSS, jQuery, html etc. It is only responsible for displaying the data that is received from the controller as the result. This also transforms the model(s) into UI.
View Model – The View Model is responsible for exposing methods, commands, and other properties that helps to maintain the state of the view, manipulate the model as the result of actions on the view, and trigger events in
the view itself.
This pattern is commonly used by the WPF, Silverlight etc.
Key Points about MVVM Pattern
- User interacts with the View.
- There is many-to-one relationship between View and ViewModel means many View can be mapped to
one ViewModel. - View has a reference to ViewModel but View Model has no information about the View.
- Supports two-way data binding between View and ViewModel.
What is ASP.NET MVC?
ASP.NET MVC is an open source framework built on the top of Microsoft .NET Framework to develop web application that enables a clean separation of code. ASP.NET MVC framework is the most customizable and extensible platform shipped by Microsoft.
How MVC pattern works in ASP.NET MVC?
The Model in ASP.NET MVC
The Model in ASP.NET MVC can be broken down into several different layers as given below:
- Objects or ViewModel or Presentation Layer – This layer contains simple objects or complex objects which are used to specify strongly-typed view. These objects are used to pass data from controller to strongly typed view and vice versa. The classes for these objects can have specific validation rules which are defined by using data annotations. Typically, these classes have those properties which you want to display on corresponding view/page.
- Business Layer – This layer helps you to implement your business logic and validations for your application. This layer make use of Data Access Layer for persisting data into database. Also, this layer is directly invoked by the Controller to do processing on input data and sent back to view.
- Data Access Layer – This layer provides objects to access and manipulate the database of your application. Typically, this layer is made by using ORM tools like Entity Framework or NHibernate etc.
The View in ASP.NET MVC
The view is only responsible for displaying the data that is received from the controller as a result. It also responsible for transforming a model or models into UI which provide all the required business logic and validation to the view. By default, views are stored in the Views folder of an ASP.NET MVC application.
The Controller in ASP.NET MVC
The Controller in ASP.NET MVC, respond to HTTP requests and determine the action to take based upon the content of the incoming request. It receives input from users via the View, then process the user’s data with the help of Model and passing the results back to the View. By default, controllers are stored in the Controllers folder an ASP.NET MVC application.
How Model, View and Controller communicate with each other in ASP.NET MVC?
There are following rules for communication among Model, View and Controller:
- User interacts with the Controller.
- There is one-to-many relationship between Controller and View means one controller can mapped to
multiple views. - Controller and View can have a reference to model.
- Controller and View can talk to each other.
- Model and View cannot talk to each other directly. They communicate to each other with the help of controller.
What are advantages of ASP.NET MVC?
There are following advantages of ASP.NET MVC over Web Forms (ASP.NET):
- Separation of concern – MVC design pattern divides the ASP.NET MVC application into three main aspects Model, View and Controller which make it easier to manage the application complexity.
- TDD – The MVC framework brings better support to test-driven development.
- Extensible and pluggable – MVC framework components were designed to be pluggable and extensible and therefore can be replaced or customized easier then Web Forms.
- Full control over application behavior – MVC framework doesn’t use View State or server based forms like Web Forms. This gives the application developer more control over the behaviors of the application and also reduces the bandwidth of requests to the server.
- ASP.NET features are supported – MVC framework is built on top of ASP.NET and therefore can use most of the features that ASP.NET include such as the providers architecture, authentication and authorization scenarios, membership and roles, caching, session and more.
- URL routing mechanism – MVC framework supports a powerful URL routing mechanism that helps to build a more comprehensible and searchable URLs in your application. This mechanism helps to the application to be more addressable from the eyes of search engines and clients and can help in search engine optimization.
History of ASP.NET MVC?
Here is the list of released version history of ASP.NET MVC Framework with theirs features.
ASP.NET MVC1
- Released on Mar 13, 2009
- Runs on .NET 3.5 and with Visual Studio 2008 & Visual Studio 2008 SP1
- MVC Pattern architecture with WebForm Engine
- Html Helpers
- Ajax helpers
- Routing
- Unit Testing
ASP.NET MVC2
- Released on Mar 10, 2010
- Runs on .NET 3.5, 4.0 and with Visual Studio 2008 & 2010
- Strongly typed HTML helpers means lambda expression based Html Helpers
- Templated Helpers
- UI helpers with automatic scaffolding & customizable templates
- Support for Data Annotations Attributes to apply model validation on both client and server sides
- Overriding the HTTP Method Verb including GET, PUT, POST, and DELETE
- Areas for partitioning a large applications into modules
- Asynchronous controllers
ASP.NET MVC3
- Released on Jan 13, 2011
- Runs on .NET 4.0 and with Visual Studio 2010
- The Razor view engine
- Enhanced Data Annotations attributes for model validation on both client and server sides
- Remote Validation
- Compare Attribute
- Session less Controller
- Child Action Output Caching
- Dependency Resolver
- Entity Framework Code First support
- Partial-page output caching
- ViewBag dynamic property for passing data from controller to view
- Global Action Filters
- Better JavaScript support with unobtrusive JavaScript, jQuery Validation, and JSON binding
- Use of NuGet to deliver software and manage dependencies throughout the platform
ASP.NET MVC4
- Released on Aug 15, 2012
- Runs on .NET 4.0, 4.5 and with Visual Studio 2010SP1 & Visual Studio 2012
- ASP.NET WEB API
- Enhancements to default project templates
- Mobile project template using jQuery Mobile
- Display Modes
- Task support for Asynchronous Controllers
- Bundling and minification
- Support for the Windows Azure SDK
ASP.NET MVC5
- Released on 17 October 2013
- Runs on .NET 4.5, 4.5.1 and with Visual Studio 2012 & Visual Studio 2013
- One ASP.NET
- ASP.NET Identity
- ASP.NET Scaffolding
- Authentication filters – run prior to authorization filters in the ASP.NET MVC pipeline
- Bootstrap in the MVC template
- ASP.NET WEB API2
What is difference between 3-layer architecture and MVC architecture?
3-layer architecture separates the application into 3 components which consists of Presentation Layer, Business Layer and Data Access Layer. In 3-layer architecture, user interacts with the Presentation layer. 3-layer is a linear architecture.

MVC architecture separates the application into three components which consists of Model, View and Controller. In MVC architecture, user interacts with the controller with the help of view. MVC is a triangle architecture.
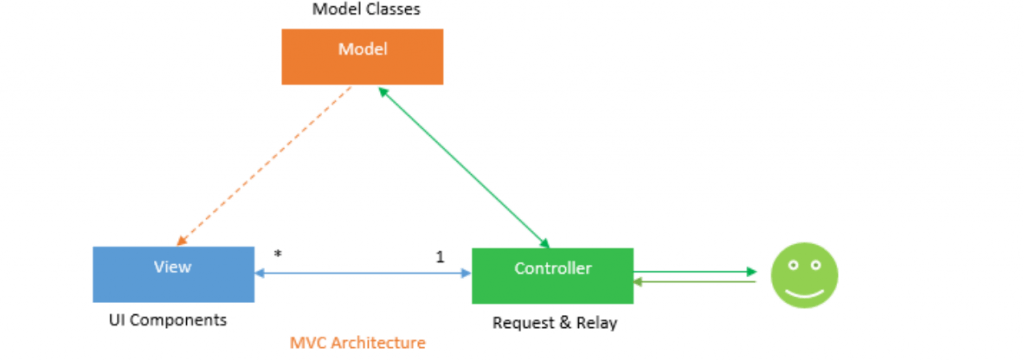
MVC does not replace 3-layer architecture. Typically 3-layer and MVC are used together and MVC acts as the Presentation layer.
What is difference between ASP.NET WebForm and ASP.NET MVC?
ASP.NET MVC | ASP.NET Web Forms |
---|---|
ASP.NET MVC is a lightweight and follow MVC (Model, View, and Controller) pattern based development model. | ASP.NET Web Form follows a traditional event driven development model. |
ASP.NET MVC has html helpers | ASP.NET Web Form has server controls. |
ASP.NET MVC has no automatic state management techniques. | ASP.NET Web Form has state management (like as view state, session) techniques. |
ASP.NET MVC has route-based URLs means URLs are divided into controllers and actions and moreover it is based on controller not on physical file. | ASP.NET Web Form has file-based URLs means file name exist in the URLs must have its physically existence. |
ASP.NET MVC follow customizable syntax (Razor as default) | ASP.NET Web Form follows WebForm Syntax |
In ASP.NET MVC, Views and logic are kept separately. | In ASP.NET Web Form, Web Forms (ASPX) i.e. views are tightly coupled to Code behind (ASPX.CS) i.e. logic. |
ASP.NET MVC has Layouts for consistent look and feels. | ASP.NET Web Form has Master Pages for consistent look and feels. |
ASP.NET MVC has Partial Views for code re-usability. | ASP.NET Web Form has User Controls for code reusability. |
ASP.NET MVC is lightweight, provide full control over mark-up and support many features that allow fast & agile development. Hence it is best for developing interactive web application with latest web standards | ASP.NET Web Form has built-in data controls and best for rapid development with powerful data access. |
ASP.NET Web MVC is an Open Source. | ASP.NET Web Form is not Open Source. |
What is ViewModel in ASP.NET MVC?
In ASP.NET MVC, ViewModel
is a class that contains the fields which are represented in the strongly-typed view. It is used to pass data from controller to strongly-typed view. Key Points about ViewModel
- ViewModel contain fields that are represented in the view (for LabelFor, EditorFor, DisplayFor helpers)
- ViewModel can have specific validation rules using data annotations.
- ViewModel can have multiple entities or objects from different data models or data source.
Explain ASP.NET MVC pipeline?
The detail ASP.NET MVC pipeline is given below:
Routing – Routing is the first step in ASP.NET MVC pipeline. Typically, it is a pattern matching system that matches the incoming request to the registered URL patterns in the Route Table.
The UrlRoutingModule(System.Web.Routing.UrlRoutingModule) is a class which matches an incoming HTTP
request to a registered route pattern in the RouteTable(System.Web.Routing.RouteTable)
Controller Initialization – The MvcHandler initiates the real processing inside ASP.NET MVC pipeline by using
ProcessRequest method. This method uses the IControllerFactory instance (default is
System.Web.Mvc.DefaultControllerFactory) to create corresponding controller.
Action Execution – Action execution occurs in the following steps:
- When the controller is initialized, the controller calls its own InvokeAction() method by passing the details of the chosen action method. This is handled by the IActionInvoker.
- After chosen of appropriate action method, model binders(default is System.Web.Mvc.DefaultModelBinder) retrieves the data from incoming HTTP request and do the data type conversion, data validation such as required or date format etc. and also take care of input values mapping to that action method parameters.
- Authentication Filter was introduced with ASP.NET MVC5 that run prior to authorization filter. It is used to authenticate a user. Authentication filter process user credentials in the request and provide a corresponding principal. Prior to ASP.NET MVC5, you use authorization filter for authentication and authorization to a user.
- By default, Authenticate attribute is used to perform Authentication. You can easily create your own custom authentication filter by implementing IAuthenticationFilter.
- Authorization filter allow you to perform authorization process for an authenticated user. For example, Role based authorization for users to access resources.
- By default, Authorize attribute is used to perform authorization. You can also make your own custom authorization filter by implementing IAuthorizationFilter.
- Action filters are executed before (OnActionExecuting) and after (OnActionExecuted) an action is executed. IActionFilter interface provides you two methods OnActionExecuting and OnActionExecuted methods which will be executed before and after an action gets executed respectively. You can also make your own custom ActionFilters filter by implementing IActionFilter. For more about filters refer this article Understanding ASP.NET MVC Filters and Attributes.
- When action is executed, it process the user inputs with the help of model (Business Model or Data Model) and prepare Action Result.
Result Execution – Result execution occurs in the following steps:
- Result filters are executed before (OnResultExecuting) and after (OnResultExecuted) the ActionResult is executed. IResultFilter interface provides you two methods OnResultExecuting and OnResultExecuted methods which will be executed before and after an ActionResult gets executed respectively. You can also make your own custom ResultFilters filter by implementing IResultFilter.
- Action Result is prepared by performing operations on user inputs with the help of BAL or DAL. The Action Result type can be ViewResult, PartialViewResult, RedirectToRouteResult, RedirectResult, ContentResult, JsonResult, FileResult and EmptyResult.
- Various Result type provided by the ASP.NET MVC can be categorized into two category- ViewResult type and NonViewResult type. The Result type which renders and returns an HTML page to the browser, falls into ViewResult category and other result type which returns only data either in text format, binary format or a JSON format, falls into NonViewResult category.
View Initialization and Rendering – View Initialization and Rendering execution occurs in the following steps:
- ViewResult type i.e. view and partial view are represented by IView (System.Web.Mvc.IView) interface and rendered by the appropriate View Engine.
- This process is handled by IViewEngine (System.Web.Mvc.IViewEngine) interface of the view engine. By default ASP.NET MVC provides WebForm and Razor view engines. You can also create your custom engine by using IViewEngine interface and can registered your custom view engine in to your ASP.NET MVC application as shown below:
- Html Helpers are used to write input fields, create links based on the routes, AJAX-enabled forms, links and much more. Html Helpers are extension methods of the HtmlHelper class and can be further extended very easily. In more complex scenario, it might render a form with client side validation with the help of JavaScript or jQuery.
What is Routing in ASP.NET MVC?
Routing is a pattern matching system that monitor the incoming request and figure out what to do with
that request. At runtime, Routing engine use the Route table for matching the incoming request’s URL pattern
against the URL patterns defined in the Route table. You can register one or more URL patterns to the Route table
at Application_Start event.
When the routing engine finds a match in the route table for the incoming request’s URL, it forwards the request
to the appropriate controller and action. If there is no match in the route table for the incoming request’s URL, it
returns a 404 HTTP status code.
How to define a route in ASP.NET MVC?
You can define a route in ASP.NET MVC as given below:
public static void RegisterRoutes(RouteCollection routes) { routes.MapRoute( "Default", // Route name "{controller}/{action}/{id}", // Route Pattern new { controller = "Home", action = "Index", id = UrlParameter.Optional }// Default values for above defined parameters ); } protected void Application_Start() { RegisterRoutes(RouteTable.Routes); //TODO: }
What is Attribute Routing and how to define it?
ASP.NET MVC5 and WEB API 2 supports a new type of routing, called attribute routing. In this routing,
attributes are used to define routes. Attribute routing provides you more control over the URIs by defining routes
directly on actions and controllers in your ASP.NET MVC application and WEB API.
Controller level routing – You can define routes at controller level which apply to all actions within the controller unless a specific route is added to an action.
[RoutePrefix("MyHome")] [Route("{action=index}")] //default action public class HomeController : Controller { //new route: /MyHome/Index public ActionResult Index() { return View(); } //new route: /MyHome/About public ActionResult About() { ViewBag.Message = "Your application description page."; return View(); } //new route: /MyHome/Contact public ActionResult Contact() { ViewBag.Message = "Your contact page."; return View(); } }
Action level routing – You can define routes at action level which apply to a specific action with in the controller.
public class HomeController : Controller { [Route("users/{id:int:min(100)}")] //route: /users/100 public ActionResult Index(int id) { //TO DO: return View(); } [Route("users/about")] //route" /users/about public ActionResult About() { ViewBag.Message = "Your application description page."; return View(); } //route: /Home/Contact public ActionResult Contact() { ViewBag.Message = "Your contact page."; return View(); } }
Note:
- Attribute routing should configure before the convention-based routing.
- When you combine attribute routing with convention-based routing, actions which do not have Route attribute for defining attribute-based routing will work according to convention-based routing. In above example Contact action will work according to convention-based routing.
- When you have only attribute routing, actions which do not have Route attribute for defining attribute-based routing will not be the part of attribute routing. In this way they can’t be access from outside as a URI.
When to use Attribute Routing?
The convention-based routing is complex to support certain URI patterns that are common in RESTful APIs. But by using attribute routing you can define these URI patterns very easily.
For example, resources often contain child resources like Clients have orders, movies have actors, books have
authors and so on. It’s natural to create URIs that reflects these relations like as: /clients/1/orders
This type of URI is difficult to create using convention-based routing. Although it can be done, the results don’t
scale well if you have many controllers or resource types.
With attribute routing, it’s pretty much easy to define a route for this URI. You simply add an attribute to the
controller action as:
[Route("clients/{clientId}/orders")] public IEnumerable<Order> GetOrdersByClient(int clientId) { //TO DO }
How to enable Attribute Routing in ASP.NET MVC?
Enabling attribute routing in your ASP.NET MVC5 application is simple, just add a call to routes.MapMvcAttributeRoutes() method with in RegisterRoutes() method of RouteConfig.cs file.
public static void RegisterRoutes(RouteCollection routes) { routes.IgnoreRoute("{resource}.axd/{*pathInfo}"); //enabling attribute routing routes.MapMvcAttributeRoutes(); }
You can also combine attribute routing with convention-based routing.
public class RouteConfig { public static void RegisterRoutes(RouteCollection routes) { routes.IgnoreRoute("{resource}.axd/{*pathInfo}"); //enabling attribute routing routes.MapMvcAttributeRoutes(); //convention-based routing routes.MapRoute( name: "Default", url: "{controller}/{action}/{id}", defaults: new { controller = "Home", action = "Index", id = UrlParameter.Optional }); } }
How to define Attribute Routing for Area in ASP.NET MVC?
You can also define attribute routing for a controller that belongs to an area by using the RouteArea attribute. When you define attribute routing for all controllers with in an area, you can safely remove the AreaRegistration class for that area.
[RouteArea("Admin")] [RoutePrefix("menu")] [Route("{action}")] public class MenuController : Controller { // route: /admin/menu/login public ActionResult Login() { return View(); } // route: /admin/menu/products [Route("products")] public ActionResult GetProducts() { return View(); } // route: /categories [Route("~/categories")] public ActionResult Categories() { return View(); } }