In this article we will learn how simply we can use Toast Notifications in ASP.NET Core Razor Pages 5.0. Mainly on the MEAN stack project, we require to notify the end-user based on events. toastr.js library is very simple and easy to use. Importantly in today’s world of responsive sites, these notification are also responsive. Please follow our previous article Worker Service in .NET Core.
What is Toastr ?
Toastr is a simple JavaScript toast notification library that is small, easy to use, and extendable. It allows you to create simple toasts with HTML5 and JavaScript like this: Simply include the files in your HTML page and write a simple line of code like this: toastr.
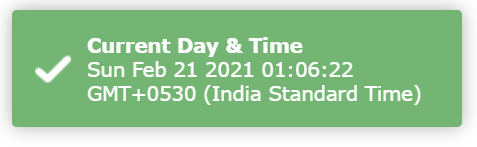
Simply include the files in your HTML page and write a simple line of code like this:
toastr.success(new Date(), 'Current Day & Time');
How to use Toast in ASP.NET Core Razor Pages ?
- Create a ASP.NET Core Razor page application and use toast like below.
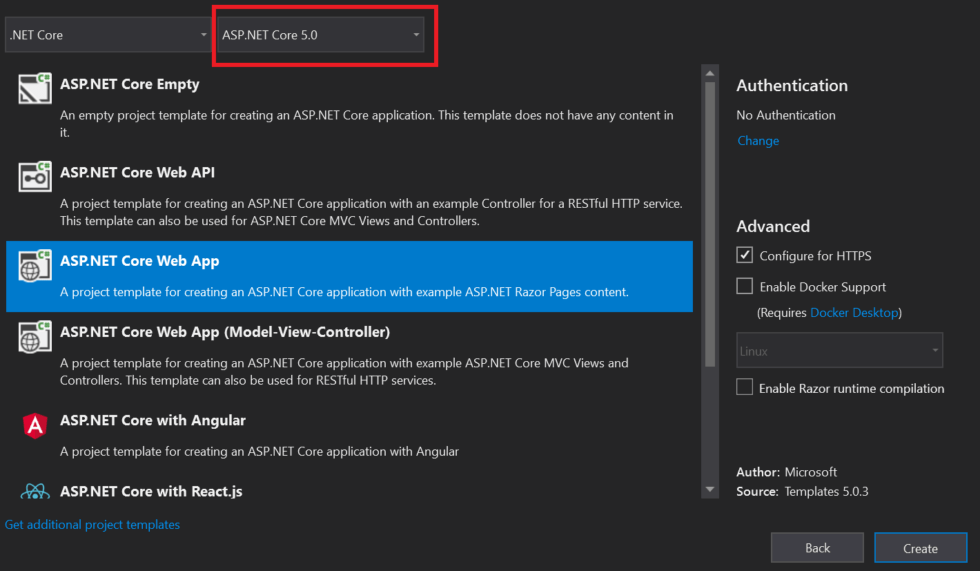
Razor Page
@page @model IndexModel @{ ViewData["Title"] = "Home page"; } <!DOCTYPE html> <html> <head> <meta http-equiv="content-type" content="text/html; charset=UTF-8"> <script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.4/jquery.min.js"> </script> <script type="text/javascript" src="js/toastr.js"> </script> <link rel="stylesheet" type="text/css" href="css/toastr.css"> <script type="text/javascript" src="js/toastrsample.js"></script> <link rel="stylesheet" type="text/css" href="css/toastrsample.css"> <title>Use Toastr in ASP .NET Core-5</title> </head> <body> <h2> How to Use Toastr Notifications </h2> <div class="control-group" id="toastrTypeGroup"> <div class="controls"> <label> Toast Type </label> <label class="radio"> <input type="radio" name="toasts" value="success" checked />Success </label> <label class="radio"> <input type="radio" name="toasts" value="info" />Info </label> <label class="radio"> <input type="radio" name="toasts" value="warning" />Warning </label> <label class="radio"> <input type="radio" name="toasts" value="error" />Error </label> </div> </div> <br /> <div class="control-group" id="toastrPositionGroup"> <div class="controls"> <label> Position </label> <label class="radio"> <input type="radio" name="positions" value="toast-top-right" checked />Top Right </label> <label class="radio"> <input type="radio" name="positions" value="toast-bottom-right" />Bottom Right </label> <label class="radio"> <input type="radio" name="positions" value="toast-bottom-left" />Bottom Left </label> <label class="radio"> <input type="radio" name="positions" value="toast-top-left" />Top Left </label> <label class="radio"> <input type="radio" name="positions" value="toast-top-full-width" />Top Full Width </label> <label class="radio"> <input type="radio" name="positions" value="toast-bottom-full-width" />Bottom Full Width </label> <label class="radio"> <input type="radio" name="positions" value="toast-top-center" />Top Center </label> <label class="radio"> <input type="radio" name="positions" value="toast-bottom-center" />Bottom Center </label> </div> </div> <br /> <button type="button" class="btn" id="showtoast" class="btn btn-primary"> Show Toastr Notification </button> </body> </html>
Create JavaScript file (toastrsample.js)
$(function() { $('#showtoast').click(function() { toastr.options = { "debug": false, "positionClass": $("#toastrPositionGroup input:radio:checked").val(), "onclick": null, "fadeIn": 300, "fadeOut": 100, "timeOut": 3000, "extendedTimeOut": 1000 } var d = Date(); toastr[$("#toastrTypeGroup input:radio:checked").val()](d, "Current Day & Time"); }); });
Run the application and see the output like below.
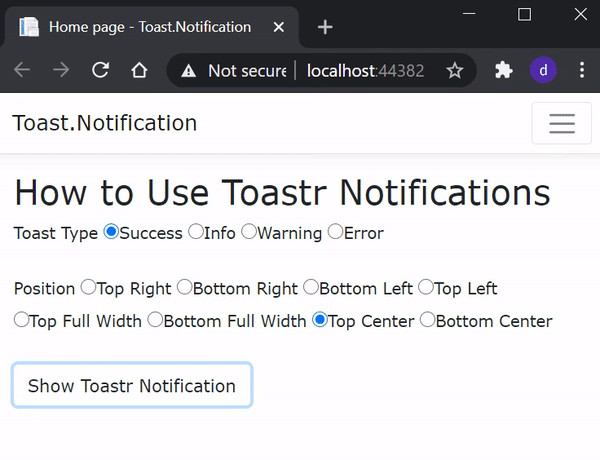
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Related Articles
- How to Integrate AdminLTE with ASP.NET Core
- How to run ASP.Net Core Web Application in Docker Container
- ChatGPT Integration in ASP.Net Core using OpenAI
- How to use cookies in ASP.Net Core- Complete Guide
- Deploy ASP.Net Core apps to Azure App Service
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.