In this article we are going to discuss how to How to Show only specific APIs on Swagger in ASP.Net Core. Please read my previous article Deploy ASP.NET Core Web App in Azure Virtual Machine.
It is pretty common to have Web APIs with tons of Controllers and methods and, sometimes, it is necessary to hide non-public APIs or expose in the Swagger documentation only specific endpoints for any reason. There are a few ways to achieve this object in Asp.Net Core applications. In this article, I explain how to use Document Filters on Swagger in order to control the endpoints that are being shown on the documentation.
Prerequisites
– Basic knowledge of Caching & In-memory of Caching
– Visual Studio 2019/2022
– .NET Core SDK 5.0 ( You can use SDK 3.1 )
What is Swagger?
Swagger is an Interface Description Language for describing RESTful APIs expressed using JSON. Swagger is used together with a set of open-source software tools to design, build, document, and use RESTful web services. Swagger includes automated documentation, code generation, and test-case generation.
Swagger configuration for Asp.Net Core
First of all, we need to configure the Swagger in the Asp.Net Core project. We created a ASP.Net core 5.0 Web API template.
Install Swagger packages in Project
Let’s install the Swashbuckle packages in the project.
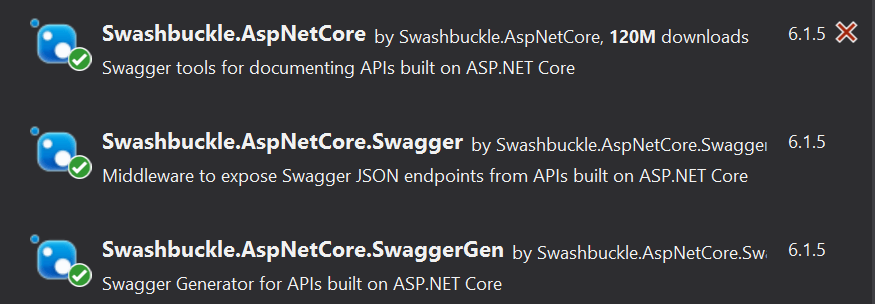
The Swagger service needs to be specified on the Startup class, as it follows
public class Startup { public Startup(IConfiguration configuration) { Configuration = configuration; } public IConfiguration Configuration { get; } // This method gets called by the runtime. Use this method to add services to the container. public void ConfigureServices(IServiceCollection services) { services.AddControllers(); services.AddSwaggerGen(c => { c.ResolveConflictingActions(apidesc => apidesc.First()); c.SwaggerDoc("v1", new OpenApiInfo { Title = "Core.SwaggerAPI", Version = "v1" }); }); } // This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); app.UseSwagger(); app.UseSwaggerUI(c => c.SwaggerEndpoint("/swagger/v1/swagger.json", "Core.SwaggerAPI v1")); } app.UseHttpsRedirection(); app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); } }
From that point, considering I’m using the default template for Web API, it already contains the WeatherForecastController. Apart from the standard get method, I created a second one called PublicGet,
[ApiController] [Route("[controller]")] public class WeatherForecastController : ControllerBase { private static readonly string[] Summaries = new[] { "Freezing", "Bracing", "Chilly", "Cool", "Mild", "Warm", "Balmy", "Hot", "Sweltering", "Scorching" }; private readonly ILogger<WeatherForecastController> _logger; public WeatherForecastController(ILogger<WeatherForecastController> logger) { _logger = logger; } [HttpGet] [Route("Get")] public IEnumerable<WeatherForecast> Get() { var rng = new Random(); return Enumerable.Range(1, 5).Select(index => new WeatherForecast { Date = DateTime.Now.AddDays(index), TemperatureC = rng.Next(-20, 55), Summary = Summaries[rng.Next(Summaries.Length)] }) .ToArray(); } [HttpGet] [Route("publicGetRange")] public WeatherForecast GetRange(int initialRange, int finalRange) { var rng = new Random(); return Enumerable.Range(initialRange, finalRange).Select(index => new WeatherForecast { Date = DateTime.Now.AddDays(index), TemperatureC = rng.Next(-20, 55), Summary = Summaries[rng.Next(Summaries.Length)] }) .FirstOrDefault(); } }
If we run the application and type endpoint “swagger” in the URL, we are already able to see the documentation with all the methods in the controller WeatherForecastController:
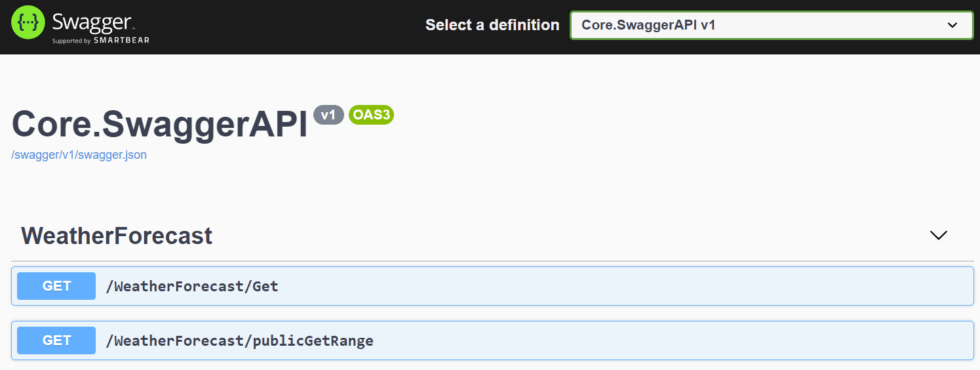
Now, imagine if we had a requirement specifying that only the PublicGet method needs to be shown on the documentation. We have to create a class implementing the IDocument interface presented in the namespace Swashbuckle.AspNetCore.SwaggerGen, as the representation in the code below:
public class CustomSwaggerFilter : IDocumentFilter { public void Apply(OpenApiDocument swaggerDoc, DocumentFilterContext context) { var nonMobileRoutes = swaggerDoc.Paths .Where(x => !x.Key.ToLower().Contains("public")) .ToList(); nonMobileRoutes.ForEach(x => { swaggerDoc.Paths.Remove(x.Key); }); } }
As you can see, the CustomSwaggerFilter class implements the IDocumentFilter interface, which contains a method called Apply. In this method, I specified a routine to remove from the routes every endpoint that does not have the word “public” as a part of the path. It could have other criteria according to your own scenario: controller name, etc.
In the Startup class a small change is necessary in order to specify the filter when the Swagger service is registered, as seen in the following image:
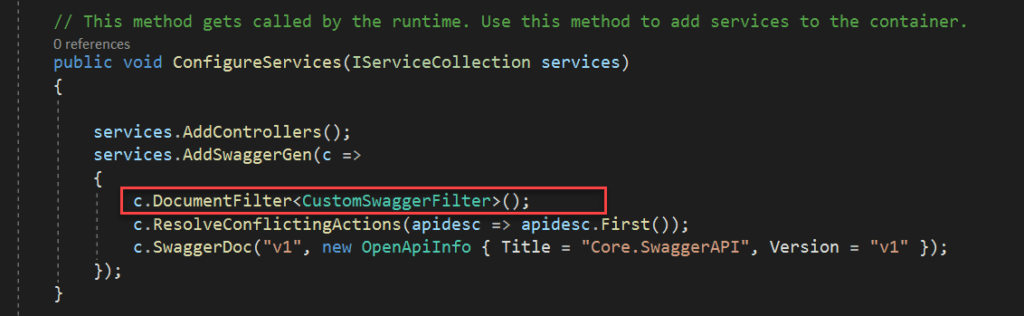
If we run the application again and call the swagger endpoint, only the PublicGet method is shown in the documentation, as seen in the following image:
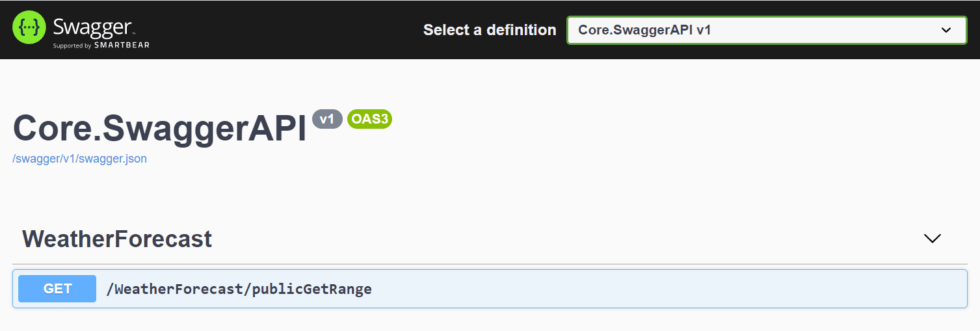
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Related Articles
- How to use Response Compression in .NET Core
- How to Integrate GraphQL in .Net Core
- Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular
- How to upload files to Azure Blob Storage using Asp.Net Core Web API
- How to store app secrets in ASP.NET Core using Secret Manager
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.