In this article we will discuss about in depth about In-Memory Caching in ASP.Net Core. Here we discuss about all the possibilities of caching and will build a simple endpoint that can help demonstrate of cache entries from the in-memory Cache, and finally we will check after enabling the caching the application performance. Please read my previous article How to show only specific APIs on swagger in ASP.Net Core.
Prerequisites
– Basic knowledge of Caching
– Visual Studio 2019/2022
– .NET Core SDK 5.0 ( You can use SDK 3.1/6.0)
What is Caching ?
Caching is the process of storing copies of files in a cache, or temporary storage location, so that they can be accessed more quickly.
A cache is a hardware or software component that stores data so that future requests for that data can be served faster; the data stored in a cache might be the result of an earlier computation or a copy of data stored elsewhere. In simply we can say the most frequently used data are stored in a temporary storage so that it can be accessed much faster for the future calls from the client. This technique will boost the performance of the application drastically by removing unnecessary and frequent requests to the data source.
Caching can significantly improve the performance and scalability of an app by reducing the work required to generate content and it works best with data that changes infrequently and is expensive to generate. Caching makes a copy of data that can be returned much faster than from the source. Apps should be written and tested to never depend on cached data.
- On left hand side, you can see every time the resources are called to the datasource that may burden to the server and counter performance issue.
- Whereas on right side part, you can see when a user is call to a datasource then it cached and it provide the result set from cached datasource only not the main datasource, so from performance point of view it should be much faster.
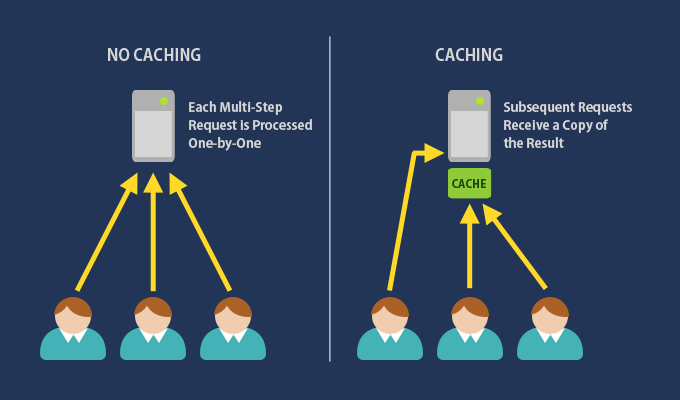
Caching In ASP.NET Core
ASP.NET Core support for various types of caching as follows.
- In-Memory Caching – Where the data is cached within the server’s memory.
- Distributed caching – The data is stored external to the application in sources like Redis cache etc., we will discuss it later.
In-Memory Caching in ASP.NET Core ?
In ASP.NET Core, it is now possible to cache the data within the application, this is known as In-Memory Caching in ASP.NET Core. The simplest cache is based on the IMemoryCache. IMemoryCache represents a cache stored in the memory of the web server. Apps running on a server farm (multiple servers) should ensure sessions are sticky when using the in-memory cache. Sticky sessions ensure that subsequent requests from a client all go to the same server.
In-memory caching is a service that’s referenced from an app using Dependency Injection. So, we first need to register this service to the built-in IoC container of ASP.NET Core by modifying ConfigureServices
method of Startup.cs
.
Cache Implementation in ASP.NET Core application
We have created the ASP.NET Core web API application that have set up an API and configured Entity Framework Core. This API will return a list of all customers in the database. In this example we created a ASP .NET Core web API 5.0 project and connect with EF along with customer database.
In-Memory Caching in ASP.NET Core is a Service that should be registered in the service container of the application.
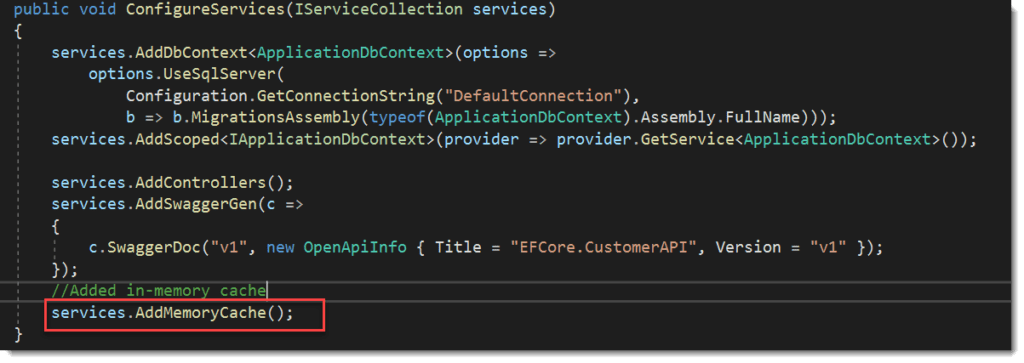
services.AddMemoryCache();
The above line of code should be add on configure services. This adds a non-distributed, in-memory implementation to the IServiceCollection. Now our Application eligible to provide in-memory caching.
Implement Cache in Controller Endpoint
[Route("api/[controller]")] [ApiController] public class CustomersController : ControllerBase { private readonly ApplicationDbContext _context; private readonly IMemoryCache memoryCache; public CustomersController(ApplicationDbContext context, IMemoryCache memoryCache) { _context = context; this.memoryCache = memoryCache; } // GET: api/Customers [HttpGet] public async Task<ActionResult<IEnumerable<Customer>>> GetCustomers() { var cacheKey = "Allcustomer"; if (!memoryCache.TryGetValue(cacheKey, out List<Customer> customerList)) { customerList = await _context.Customers.ToListAsync(); var cacheExpiryOptions = new MemoryCacheEntryOptions { AbsoluteExpiration = DateTime.Now.AddMinutes(5), Priority = CacheItemPriority.High, SlidingExpiration = TimeSpan.FromMinutes(2) }; memoryCache.Set(cacheKey, customerList, cacheExpiryOptions); } return customerList; } } }
Code Explanation
- #6 – IMemoryCache definition
- #16 – Here we are setting the cache key internally in our code.
- #17 – If there is a cache entry with the key as “Allcustomer”, then push this data to a List object. Else, if there is no such cache entry, call the database via the context object and set this value as a new cache entry along with the expiration parameters. The expiration date is according to you, you can according to your requirements.
SlidingExpiration
: Gets or sets how long a cache entry can be inactive (e.g. not accessed) before it will be removed. This will not extend the entry lifetime beyond the absolute expiration (if set).AbsoluteExpiration:
Gets or sets an absolute expiration date for the cache entry.
A cached item set with a sliding expiration only is at risk of becoming stale. If it’s accessed more frequently than the sliding expiration interval, the item will never expire. Combine a sliding expiration with an absolute expiration to guarantee that the item expires once its absolute expiration time passes
Test the In-Memory Cache in Postman
Let’s open the postman tool and test the cache performance.
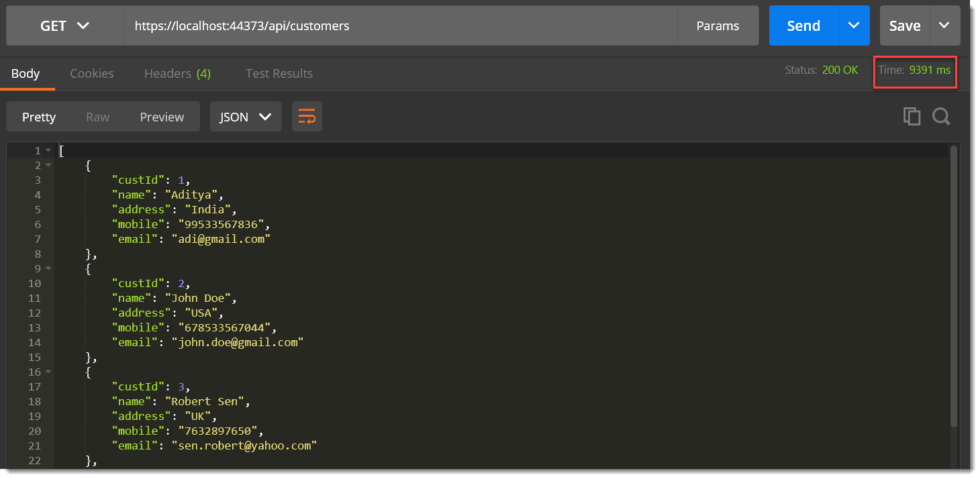
You can see when I call the customer API, it fetch around 50-60 records to take time 9391 ms.
In this call, we are directly calling the database, which may be slow depending on the traffic, connection status, size of the response, and so on. We have configured our API to parallelly store these records to the cache as well.
So in the second call, the response is expected at much more better time.
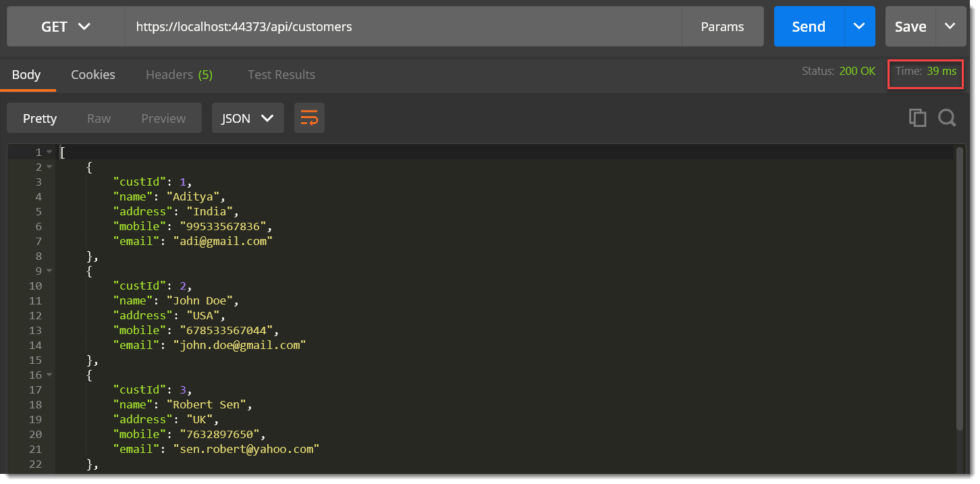
As you can see, on second API call it takes 39 ms. You can see the drastic improvement in the performance, this is how awesome Caching is.
Pros of In-Memory Cache
- Much Quicker than other forms of distributed caching as it avoids communicating over a network.
- Highly Reliable and best suited for Small to Mid Scale Applications.
Cons of In-Memory Cache
- If configured incorrectly, it can consume your server’s resources.
- With the scaling of application and longer caching periods, it can prove to be costly to maintain the server.
- If deployed in the cloud, maintaining consistent caches can be difficult.
Points To Remember about Caching
- Application should never depend on the Cached data as it is highly probable to be unavailable at any given time. Traditionally it should depend on your actual data source. Caching is just an enhancement that is to be used only if it is available/valid.
- Try to restrict the growth of the cache in memory. This is crucial as Caching may take up your server resources if not configured properly. You can make use of the Size property to limit the cache used for entries.
- Use Absolute Expiration / Sliding Expiration to make your application much faster and smarter. It also helps restricts cache memory usage.
- Try to avoid external inputs as cache keys. Always set your keys in code.
How to improve Cache Entry
To do improvements on caching, we can use Background Jobs to update cache at a regular interval. If the Absolute Cache Expiration is set to 10 minutes, then we can can run a recurring job every 11 minutes of interval to update the cache entry. You can use Hangfire
to achieve the same in ASP.NET Core Applications.
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Related Articles
- How to use Response Compression in .NET Core
- How to Integrate GraphQL in .Net Core
- Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular
- How to upload files to Azure Blob Storage using Asp.Net Core Web API
- How to store app secrets in ASP.NET Core using Secret Manager
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.