In this article we will discuss how to make unit testing in ASP.Net csharp. Testing is an essential aspect of any programming language. Testing for ASP.Net applications is possible with the help of Visual Studio. Every successful software development follows a lifecycle known as SDLC (Software Development Life Cycle).As per Wikipedia: “SDLC is a process followed for a software project, within a software organization. It consists of a detailed plan describing how to develop, maintain, replace and alter or enhance specific software. The life cycle defines a methodology for improving the quality of software and the overall development process.” Please read my previous article of Automation Testing using Specflow and Selenium in .NET Framework.
As a developer we normally understand the requirements and write the code. Apart from those we also spend some time software testing. Software testing is a process of executing a program or application with the intent of finding the software bugs. It can also be described as the process of validating and verifying a software program or application or product.
Introduction to Unit testing for ASP.NET
The first level of testing an ASP.Net project is unit level testing. This test is the functionality of an application. The testing is conducted to ensure that the application behaves as expected. In ASP.Net, the first task is to create a test project in Visual Studio. The test project will contain the necessary code to test the application.
Let’s consider the below web page. In the page, we have the sample Calculator that can calculate the passing data (+,–,*,/). Now how can we confirm that the correct message is displayed when an ASP.Net project runs. This is done by adding a test project to the ASP.Net solution (used to develop web-based applications). This test project would ensure that the right message is displayed to the user.

Create Sample ASP.NET C# Web Application
Let’s Create a sample ASP.NET web application to following below steps.
- Choose ASP.NET Web application(.NET Framework)
- Choose Web Forms template as we want to do it in ASP.NET Web forms.
- We name the ASP.NET Web forms project name as “Demo.App”.
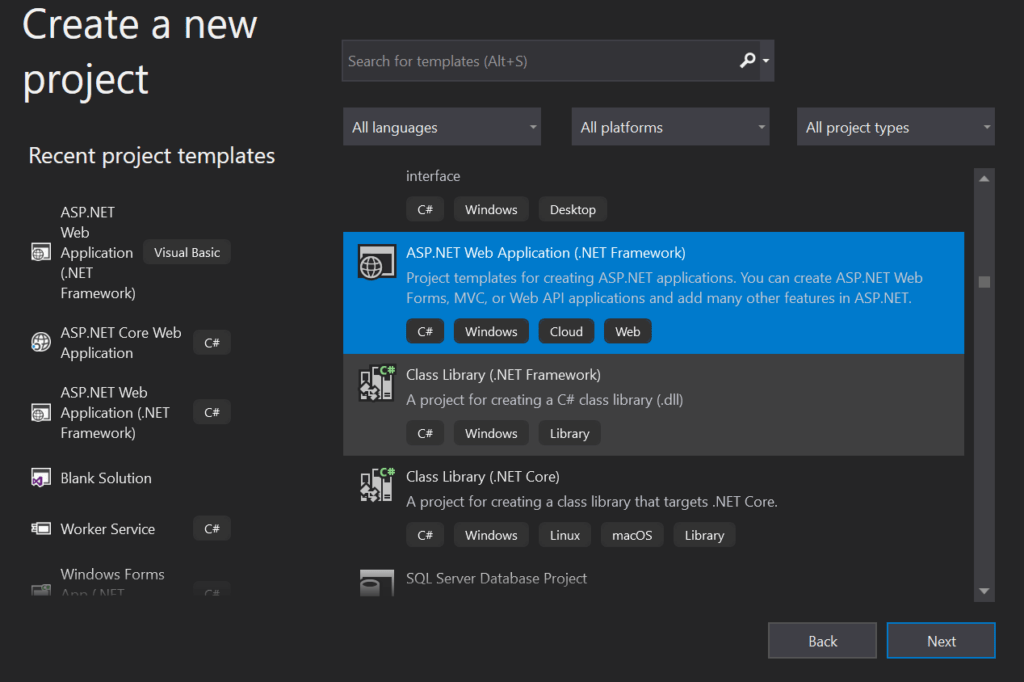
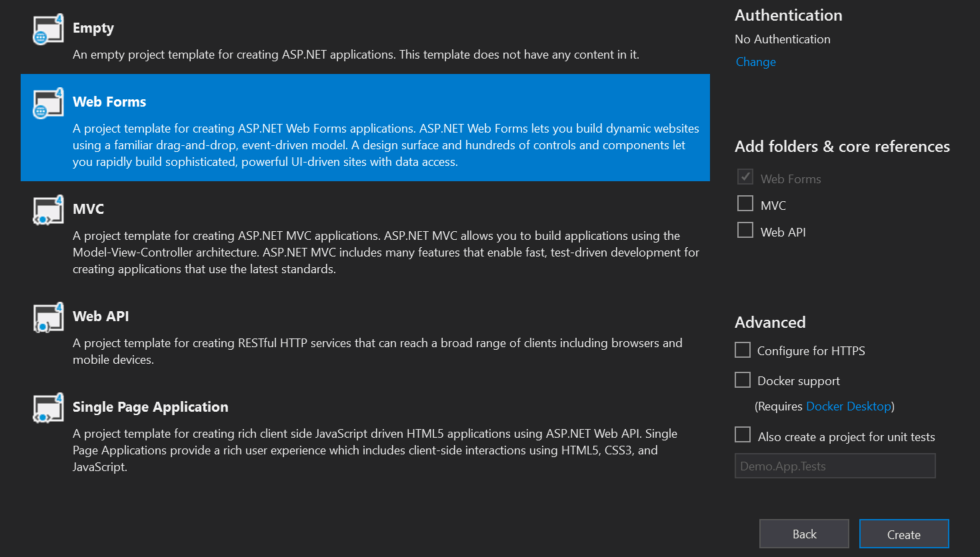
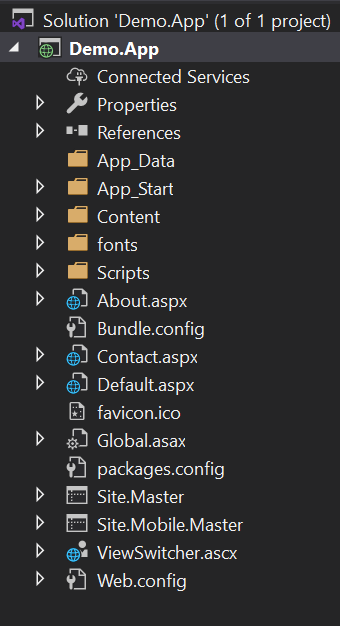
Create a Models Folder under Demo.App ASP.NET C# application
- Right Click on the Demo.App project and add a new folder named as “Models”.
- Under “Models” folder add a class name as “Calculator.cs” with required methods to perform addition, subtraction, multiplication and Division.
- And the sample code look like below,
public class Calculator { public int add(int x, int y) { return x + y; } public int sub(int x, int y) { return x - y; } public int mul(int x, int y) { return x * y; } public int div(int x, int y) { return x - y; } }
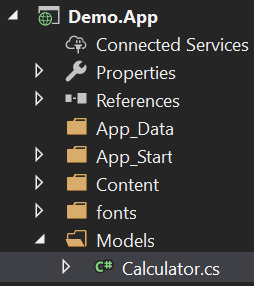
Impose Calculator method in Default page
<div class="jumbotron"> <div class="row"> <asp:Label ID="lbl_add" runat="server"></asp:Label> </div> <div class="row"> <asp:Label ID="labl_sub" runat="server"></asp:Label> </div> <div class="row"> <asp:Label ID="lbl_mul" runat="server"></asp:Label> </div> <div class="row"> <asp:Label ID="lbl_div" runat="server"></asp:Label> </div> </div>
public partial class _Default : Page { Calculator _calc = new Calculator(); protected void Page_Load(object sender, EventArgs e) { lbl_add.Text ="Addition Value of (100,5) is : " + _calc.add(100, 5).ToString(); labl_sub.Text = "Substraction Value of (100,5) is : " + _calc.sub(100, 5).ToString(); lbl_mul.Text = "Multiplication Value of (100,5) is : " + _calc.mul(100, 5).ToString(); lbl_div.Text = "Division Value of (100,5) is : " + _calc.div(100, 5).ToString(); } }
Creating a .NET Unit Testing Project
Before we create a test project, let’s remind the below high-level steps.
Ensure the Demo.App is open in Visual Studio
To following below steps create the Unit Test project.
- Choose Unit Test template (We set the project name as Demo.Test)
- Add reference the Demo.APP in Unit test project
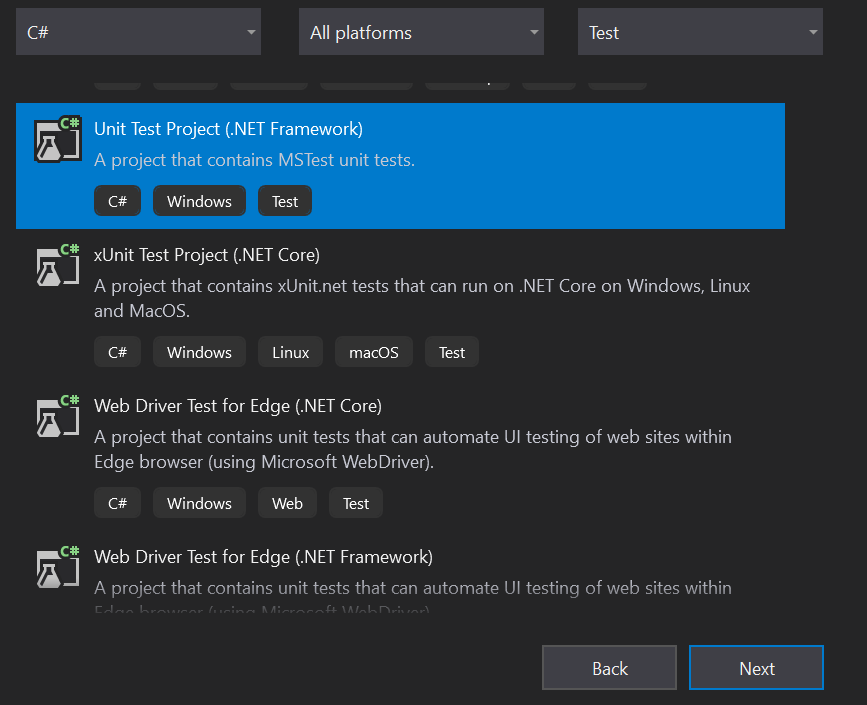
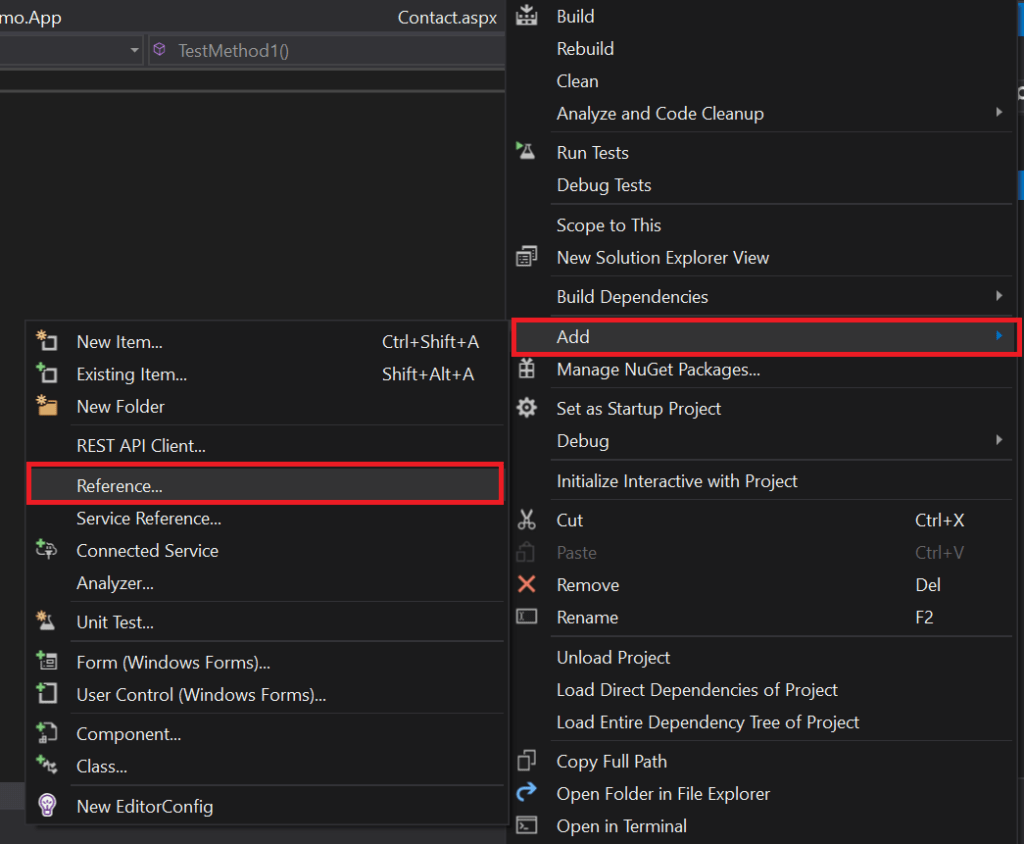
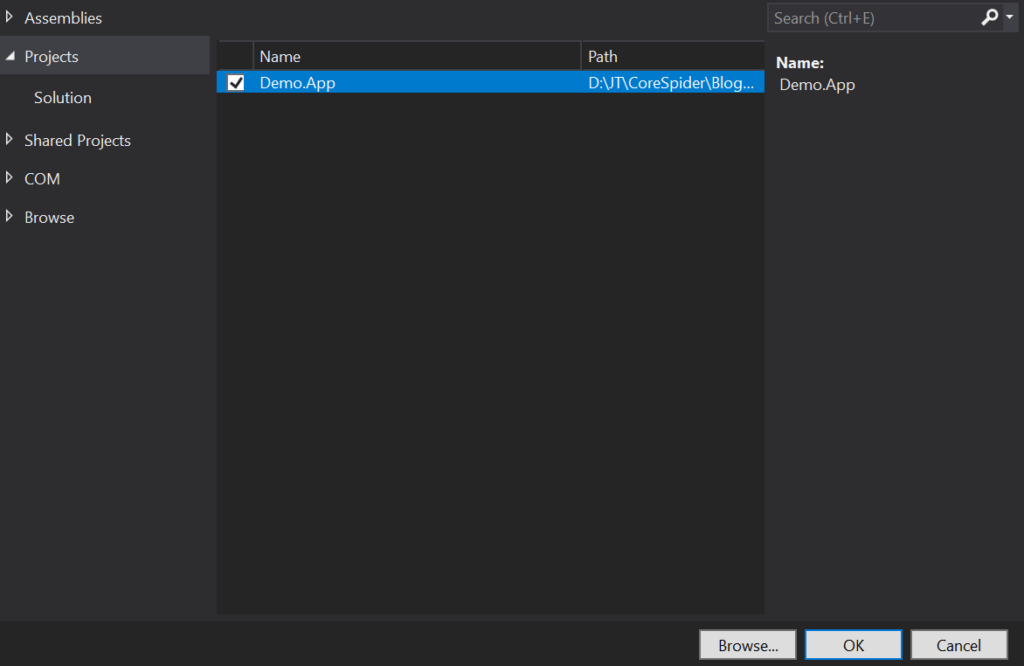
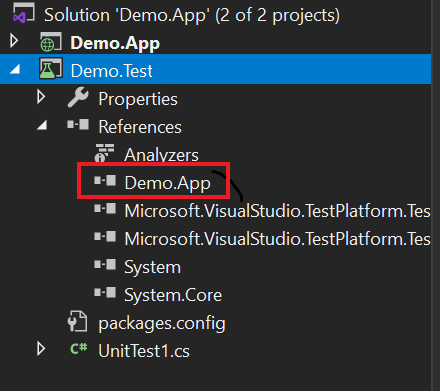
UnitTest1.cs file look like below
[TestClass] public class UnitTest1 { [TestMethod] public void TestMethod1() { Calculator _cal = new Calculator(); // addition Assert.AreEqual(_cal.add(10, 20),30); // substraction Assert.AreEqual(_cal.sub(20, 20), 0); // multiplication Assert.AreEqual(_cal.mul(10, 20), 200); // division Assert.AreEqual(_cal.div(20, 20), 0); } }
Code Explanation
- You can see we access the Calculator class and create the object.
- Call all the calculate method with the expected value and actual value.
- Expected value is that we pass to the method and actual value is depends upon expected result means let’s take an example Assert.AreEqual(_cal.add(10, 20),30); If we pass value as (10,20) then output should be 30. If the actual value is not 30 then the unit test is not pass. Like this others are work accordingly to their type.
- Let’s run the Unit test project to follow below steps.
Run Unit Test Project
To run the Unit test project follow below steps.
- Choose on the Menu Test and then select Run All Tests and if all the result is pass then below image is show as pass.
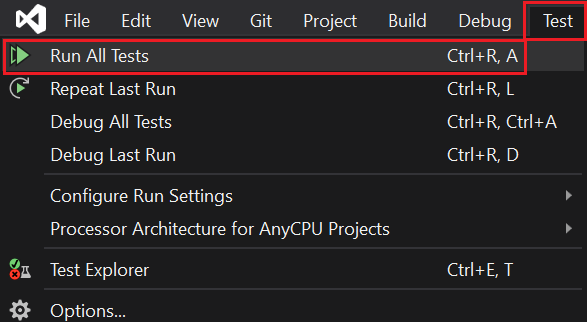
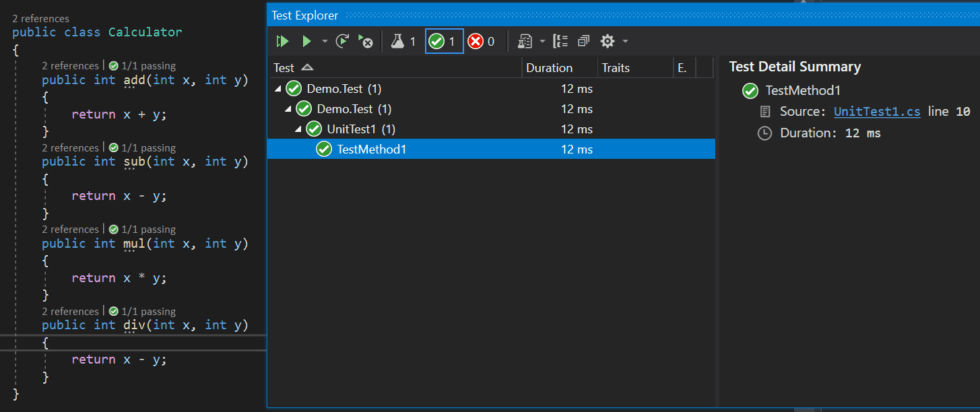
How to test the Unit testing Project is failed
Let’s make the change the expected value with wrong one and see how the test result is failed.
- You can see we make change on Assert.AreEqual(_cal.add(10, 20),35) the expected value is 10+20=30 but we make as 35.
- Like this Assert.AreEqual(_cal.sub(20, 20), 10) we make as 20-20=0 but we make as 10.
- And when we run you can see the below image the test result is clearly failed.
[TestClass] public class UnitTest1 { [TestMethod] public void TestMethod1() { Calculator _cal = new Calculator(); // addition Assert.AreEqual(_cal.add(10, 20),35); // substraction Assert.AreEqual(_cal.sub(20, 20), 10); // multiplication Assert.AreEqual(_cal.mul(10, 20), 200); // division Assert.AreEqual(_cal.div(20, 20), 0); } }
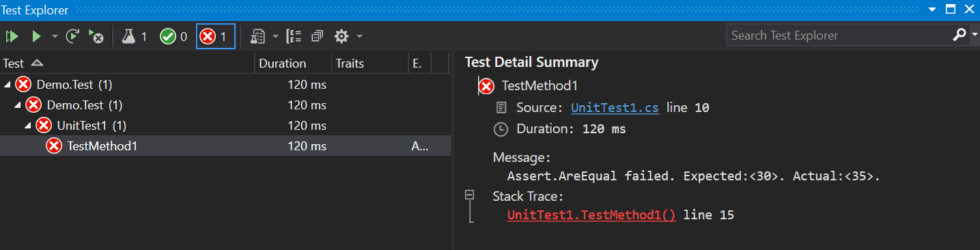
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Related Articles
- How to use Response Compression in .NET Core
- How to migrate .Net Core 7 to .Net Core 8
- How to Integrate GraphQL in .Net Core
- Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular
- How to upload files to Azure Blob Storage using Asp.Net Core Web API
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.