In this article we are going to discuss about Worker Service in .Net Core. o learn step by step process about .NET Core and ASP .NET Core please follow the link. Please read my previous article CQRS pattern with MediatR in ASP.NET Core 5.0.
What is Worker Service in .NET Core
A worker service is a .NET project built using a template which supplies a few useful features that turn a regular console application into something more powerful. A worker service runs on top of the concept of a host, which maintains the lifetime of the application. The host also makes available some familiar features, such as dependency injection, logging and configuration. Worker services will generally be long-running services, performing some regularly occurring workload.
Create Worker Service in .NET Core
Like below we can create worker service in .NET Core from scratch.
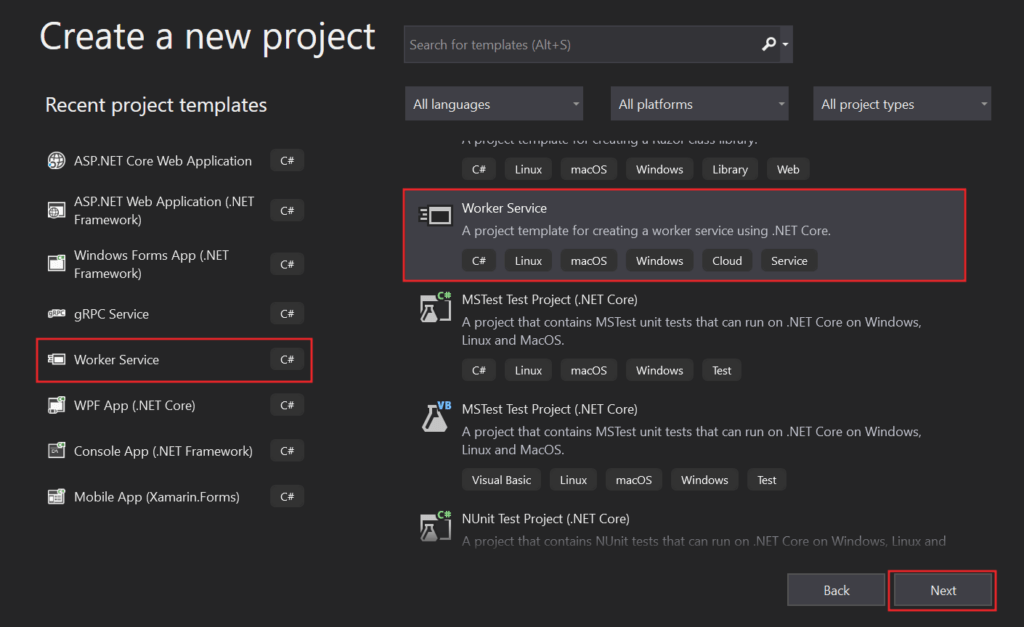
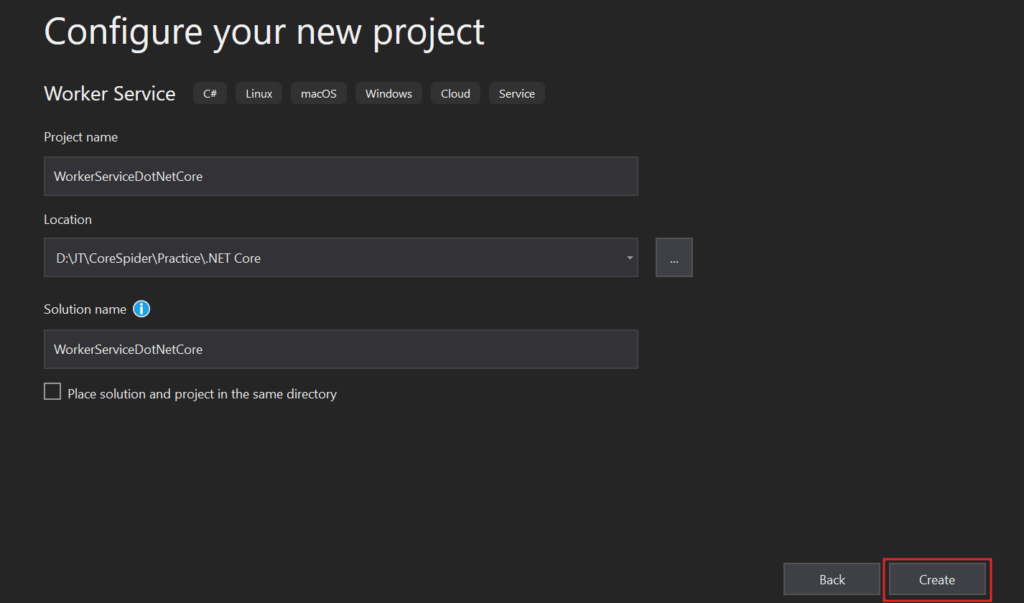
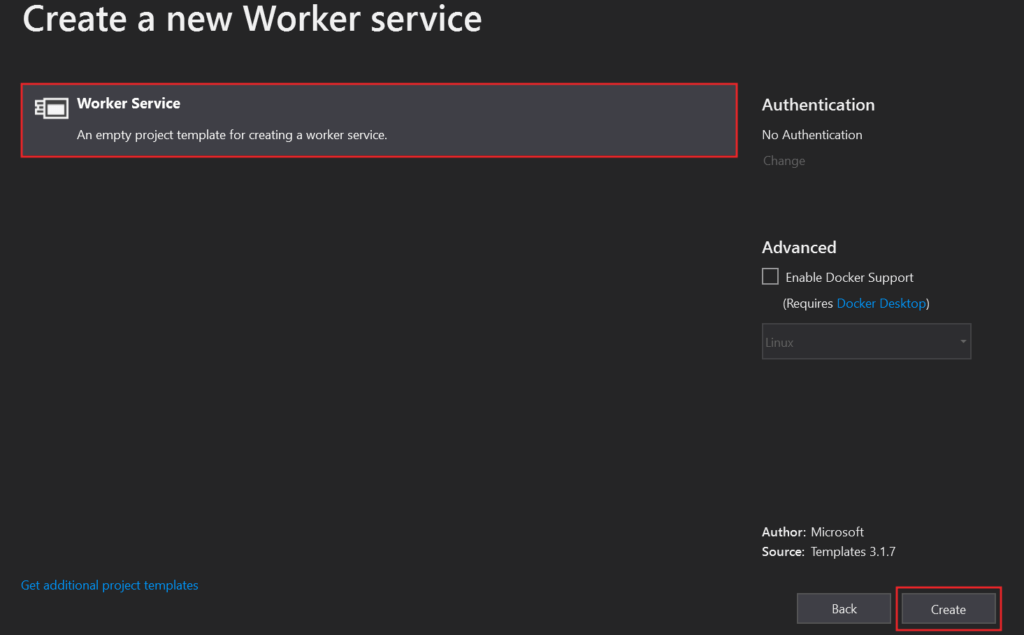
To use the Command Line, simply use the following command
dotnet new worker -o myworkerproject
Program.cs
This class consists of the required Main method entry point for .NET console applications. The .NET runtime expects to locate this method within the Program class when it starts your .NET application.
CreateHostBuilder
private method which creates an IHostBuilder
. The IHostBuilder interface defines a type which uses the builder pattern to produce an instance of an IHost
. The template creates a new HostBuilder by calling the static CreateDefaultBuilder
method on the Host class.
public class Program { public static void Main(string[] args) { CreateHostBuilder(args).Build().Run(); } public static IHostBuilder CreateHostBuilder(string[] args) => Host.CreateDefaultBuilder(args) .ConfigureServices((hostContext, services) => { services.AddHostedService<Worker>(); }); }
Worker.cs
The Worker
class is something new which you will not find in the default ASP.NET Core project template. This is where the magic of hosted services, combined with the host, provide the basis of a worker service.
This class derives from the BackgroundService
abstract base class. The BackgroundService class implements an interface named IHostedService. BackgroundService includes an abstract method named ExecuteAsync which we must override in our subclass, just as the worker class, provided in the worker service template, does here. ExecuteAsync returns a Task which internally the BackgroundService expects to be some long-running workload. It will start the Task, which then runs in the background.
public class Worker : BackgroundService { private readonly ILogger<Worker> _logger; public Worker(ILogger<Worker> logger) { _logger = logger; } protected override async Task ExecuteAsync(CancellationToken stoppingToken) { while (!stoppingToken.IsCancellationRequested) { _logger.LogInformation("Worker running at: {time}", DateTimeOffset.Now); await Task.Delay(1000, stoppingToken); } } }
How Do I Register an IHostedService ?
The next logical question is, how do I register an IHostedService ? If we head back to the code from Program.cs, we’ll find out the code as services.AddHostedService();
public static IHostBuilder CreateHostBuilder(string[] args) => Host.CreateDefaultBuilder(args) .ConfigureServices((hostContext, services) => { services.AddHostedService<Worker>(); });
Creating Worker Service Programming
Let’s add 3 packages from Nuget to use Worker Service and Serilog.
Add Package in Worker Service Project using Nuget
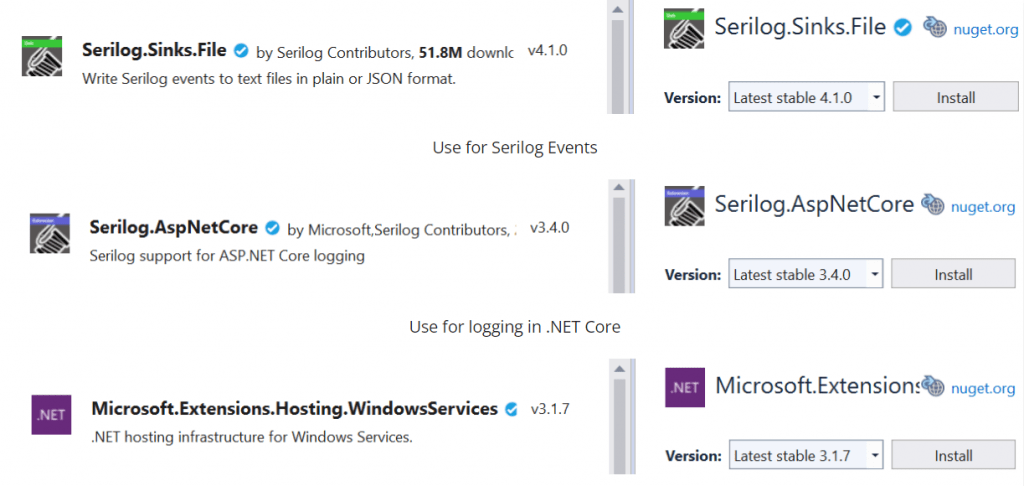
Modify Program.cs
public class Program { public static void Main(string[] args) { Log.Logger = new LoggerConfiguration() .MinimumLevel.Debug() .MinimumLevel.Override("Microsoft", LogEventLevel.Warning) .Enrich.FromLogContext() .WriteTo.File(@"C:\LogFile.txt") .CreateLogger(); try { Log.Information("Starting up the service"); CreateHostBuilder(args).Build().Run(); return; } catch (Exception ex) { Log.Fatal(ex, "There was a problem starting the serivce"); return; } finally { Log.CloseAndFlush(); } } public static IHostBuilder CreateHostBuilder(string[] args) { return Host.CreateDefaultBuilder(args) .UseWindowsService() .ConfigureServices((hostContext, services) => { services.AddHostedService<Worker>(); }) .UseSerilog(); } }
- We initiate a logger that should be logged the process into a TXT file that is to be created on C-drive (you may save it into as per your desired location).
- In try block we call the CreateHostBuilder method.
- In the web host builder method added .UseWindowsService() to register windows service.
- services.AddHostedService<Worker>() enable worker process.
Modify Worker.cs
public class Worker : BackgroundService { private readonly ILogger<Worker> _logger; private HttpClient client; public Worker(ILogger<Worker> logger) { _logger = logger; } public override Task StartAsync(CancellationToken cancellationToken) { client = new HttpClient(); return base.StartAsync(cancellationToken); } public override Task StopAsync(CancellationToken cancellationToken) { client.Dispose(); _logger.LogInformation("The service has been stopped..."); return base.StopAsync(cancellationToken); } protected override async Task ExecuteAsync(CancellationToken stoppingToken) { while (!stoppingToken.IsCancellationRequested) { var result = await client.GetAsync("https://www.corespider.com"); if (result.IsSuccessStatusCode) { _logger.LogInformation("CoreSpider is running and the Status code {StatusCode}", result.StatusCode); } else { _logger.LogError("CoreSpider is is down. Status code {StatusCode}", result.StatusCode); } _logger.LogInformation("Worker running at: {time}", DateTimeOffset.Now); await Task.Delay(10000, stoppingToken); } } }
- StartAsync is use to start the windows service. Here we initialize the HttpClient.
- StopAsync is use if the worker service is stopped.
- ExecuteAsync is the method where we implement our logic. Here we check if the website response is okay then do your stuff.
- Task.Delay(10000, stoppingToken) is use to delay the task for 1 minute.
- 10000 millisecond=1 minute according to your requirement you can set the intervals.
Windows Service management
To achieve best performance, we first need to build our application in the Release
mode.
The commands to create, start/stop, and delete a Windows Service are shown in the following code snippet.
:: Create a Windows Service sc create WorkerService DisplayName="WorkerServiceDotNetCore" binPath="C:\full\path\to\WorkerServiceDotNetCore.exe" :: Start a Windows Service sc start WorkerService :: Stop a Windows Service sc stop WorkerService :: Delete a Windows Service sc delete WorkerService
Open command shell as Run as Administrator
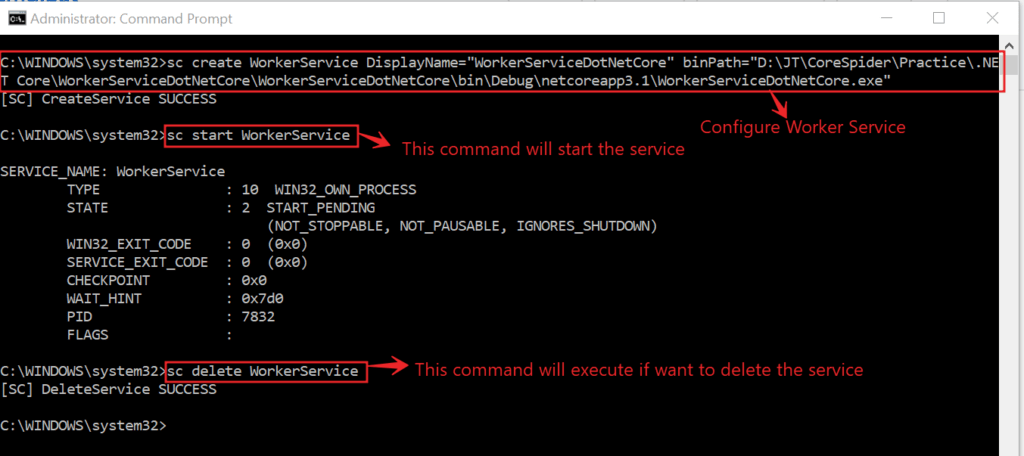
After run the above command you can see the worker service is configured on windows machine. To open the services on windows type in search bar of windows services.msc
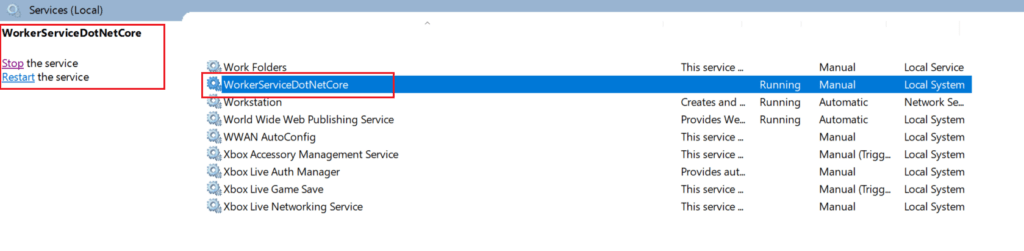
After running the worker service you can see the log file is generated in C-drive with interval of 1 minute.
2020-08-24 12:58:22.306 +05:30 [INF] CoreSpider is running and the Status code "OK" 2020-08-24 12:58:22.307 +05:30 [INF] Worker running at: "2020-08-24T12:58:22.3073606+05:30" 2020-08-24 12:58:35.012 +05:30 [INF] CoreSpider is running and the Status code "OK" 2020-08-24 12:58:35.013 +05:30 [INF] Worker running at: "2020-08-24T12:58:35.0134560+05:30" 2020-08-24 12:58:47.011 +05:30 [INF] CoreSpider is running and the Status code "OK" 2020-08-24 12:58:47.012 +05:30 [INF] Worker running at: "2020-08-24T12:58:47.0123668+05:30" 2020-08-24 12:58:58.158 +05:30 [INF] CoreSpider is running and the Status code "OK" 2020-08-24 12:58:58.159 +05:30 [INF] Worker running at: "2020-08-24T12:58:58.1588869+05:30" 2020-08-24 12:59:10.445 +05:30 [INF] CoreSpider is running and the Status code "OK" 2020-08-24 12:59:10.446 +05:30 [INF] Worker running at: "2020-08-24T12:59:10.4465566+05:30" 2020-08-24 12:59:21.705 +05:30 [INF] CoreSpider is running and the Status code "OK" 2020-08-24 12:59:21.705 +05:30 [INF] Worker running at: "2020-08-24T12:59:21.7056397+05:30" 2020-08-24 12:59:33.250 +05:30 [INF] CoreSpider is running and the Status code "OK" 2020-08-24 12:59:33.251 +05:30 [INF] Worker running at: "2020-08-24T12:59:33.2512991+05:30" 2020-08-24 12:59:44.848 +05:30 [INF] CoreSpider is running and the Status code "OK" 2020-08-24 12:59:44.849 +05:30 [INF] Worker running at: "2020-08-24T12:59:44.8495591+05:30" 2020-08-24 12:59:55.917 +05:30 [INF] CoreSpider is running and the Status code "OK" 2020-08-24 12:59:55.918 +05:30 [INF] Worker running at: "2020-08-24T12:59:55.9185238+05:30" 2020-08-24 13:00:07.912 +05:30 [INF] CoreSpider is running and the Status code "OK" 2020-08-24 13:00:07.912 +05:30 [INF] Worker running at: "2020-08-24T13:00:07.9127129+05:30" 2020-08-24 13:00:19.368 +05:30 [INF] CoreSpider is running and the Status code "OK" 2020-08-24 13:00:19.369 +05:30 [INF] Worker running at: "2020-08-24T13:00:19.3689720+05:30"
What Files are part of the worker service template ?
- Program.cs – Entry point for the console application. Creates and runs a host to manage the application lifetime and make a long-running service.
- appsettings.json – A JSON file which provides application configuration values.
- Worker.cs – Derives from the BackgroundService base class to define long-running workloads which are executed as background Tasks.
What are Worker Services
- Applications which do not require user interaction.
- Use a host to maintain the lifetime of the console application until the host is signalled to shut down. Turning a console application into a long-running service.
- Include features common to ASP.NET Core such and dependency injection, logging and configuration.
- Perform periodic and long-running workloads.
Conclusion
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
Related Articles
- How to use Response Compression in .NET Core
- How to migrate .Net Core 7 to .Net Core 8
- How to Integrate GraphQL in .Net Core
- Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular
- How to upload files to Azure Blob Storage using Asp.Net Core Web API
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.