In this article we will learn about complete guide of C# Constructors. Here we discuss what a constructor is and why it is required in C#, as well as different types of constructors and when to use which constructors, as well as some basic questions and answers about constructors. Please read my previous article about How to use cookies in ASP.Net Core- Complete Guide.
Constructors are the special method of the class which is used to initialize the data members of the new object. It is associated with a class and get automatically invoked when the classes instance (i.e., objects) are created.
Fundamental characteristics of Constructors
- The constructor of a class must have the same name as the class in which it resides.
- A class can have any number of constructors.
- A constructor can never be abstract, final, static and synchronized.
- A constructor doesn’t have a return type but neither the return type is void.
- Constructors can have access modifiers along with it to control its access i.e. which other class can call the constructor
Types of Constructors in C#
- Default Constructor
- Parameterized Constructor
- Copy Constructor
- Private Constructor
- Static Constructor
Default Constructor
If a class doesn’t have a constructor then a default constructor gets called when object is created. The default constructor is added to a class by default if you don’t add any constructor to your class.
- The default constructor should have public access.
- A default constructor is parameter less.
Example of Default Constructor
namespace Constructors
{
public class Employee
{
public int ID;
public string name;
public string designation;
public Employee() // Default Constructor
{
Console.WriteLine("Default Constructor is Called!");
ID = 123;
name = "John Smith";
designation = "Software Engineer";
}
}
public class Program
{
public static void Main()
{
Employee s = new Employee();
Console.WriteLine(s.name + "\n" + s.ID + "\n" + s.designation);
}
}
}
Output
Default Constructor is Called
John Smith
123
Software Engineer
Code Explanation
Here we have specified the default constructor ourselves, but even if we don’t do that, the compiler will automatically assign a default constructor to the class which is used for initialization of object of the class. That’s why you can find the “Default constructor is called” message is invoked first.
Parameterized Constructor
A constructor with at least one parameter is called as parameterized constructor.
namespace Constructors
{
public class Employee
{
public int ID;
public string name;
public string designation;
public Employee(int id, string names, string desig) // Default Constructor
{
Console.WriteLine("Default Constructor Called!");
ID = id;
name = names;
designation = desig;
Console.WriteLine(id + "\n" + name + "\n" + desig);
Console.WriteLine("\n");
}
}
public class Program
{
public static void Main()
{
Employee emp1 = new Employee(123, "John Smith","Software Engineer");
emp1 = new Employee(321, "Ronaldo Dev", "HR");
Console.WriteLine();
}
}
}
Output
Default Constructor Called!
123
John Smith
Software Engineer
Default Constructor Called!
321
Ronaldo Dev
HR
Code Explanation
Here we have specified the parametrized constructor with passing required parameters. And we set the two values into the class so we can see there is print two employee information’s. The console of text “Default constructor” is w printed twice due to we have initiate the class twice.
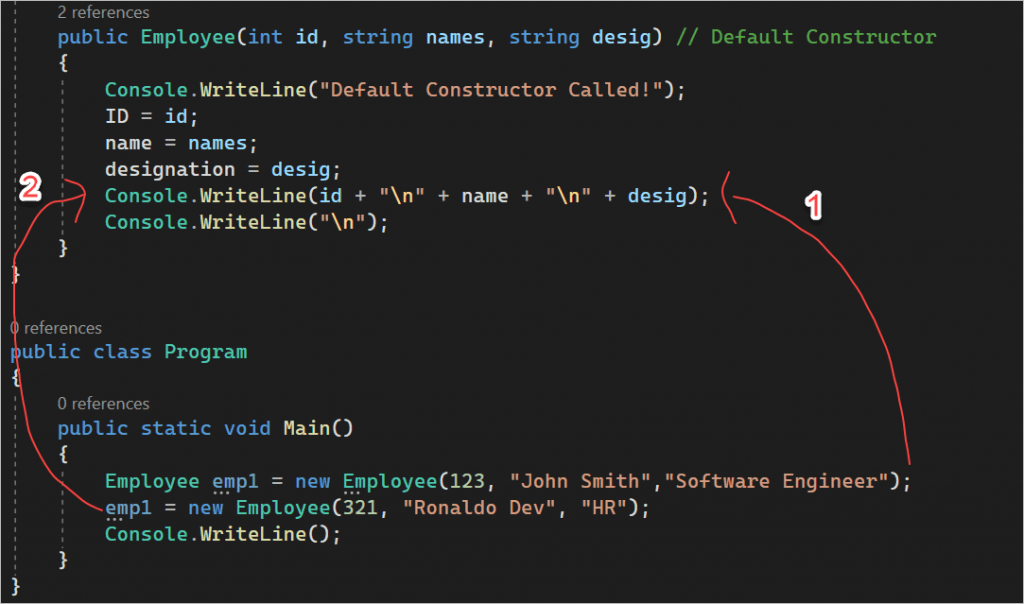
Copy Constructor
The copy constructor is used to create an object by copying all of its variables(attributes) from another object. This type of constructors are used for initializing a new instance from an existing one.
namespace Constructors
{
public class Employee
{
public int ID;
public string name;
public string designation;
public Employee(int id, string names, string desig) // Default Constructor
{
ID = id;
name = names;
designation = desig;
}
// Copy Constructor
public Employee(Employee e)
{
ID = e.ID;
name = e.name;
designation = e.designation;
}
}
public class Program
{
public static void Main()
{
Employee emp1 = new Employee(123, "John Smith","Software Engineer");
Console.WriteLine("Employee1 value is: " + emp1.ID + "\n" + emp1.name + "\n" + emp1.designation);
Console.WriteLine("\n");
// Another Employee by copying employee details
Employee emp2 = new Employee(emp1);
Console.WriteLine("Employee2 value is: " + emp2.ID + "\n" + emp2.name + "\n" + emp2.designation);
Console.WriteLine("\n");
emp2.ID = 1234;
emp2.name = "Ronaldo Dev";
emp2.designation = "HR";
Console.WriteLine("Employee2 value is: " + emp2.ID + "\n" + emp2.name + "\n" + emp2.designation);
Console.WriteLine();
}
}
}
Code Explanation
Here we have initialize the emp1
values and print the information and then the same emp1
data we passed as parametrized constructor to emp2
and print the result, you can see for both of the result same.
And with adding the emp2
values with new data it print the new record.
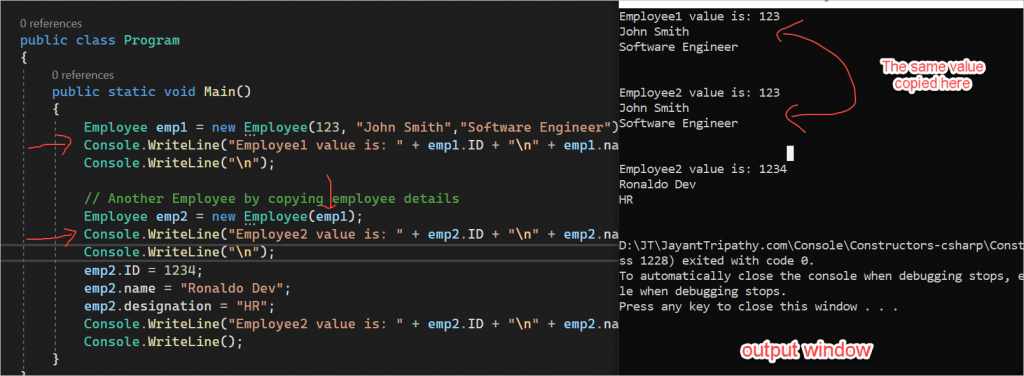
Private Constructor
A constructor that is preceded by a private access modifier is called a private constructor. It does not make it possible for other classes to inherit any data from this class (we can’t instantiate the class if the constructor is private). We will cover more on this concept when we will cover the concept of inheritance.
Following are its characteristics:
- Generally, private constructor is used in classes that contain static members only.
- We can’t create public and private constructors simultaneously in a class, both without parameters.
- We can’t instantiate the class with a private constructor.
- If we want to create an object of a class with private constructor then, we need to have public constructor along with it.
Points To Remember about C# Private Constructor:
- Using Private Constructor in C# we can implement the singleton design pattern.
- We need to use the private constructor in C# when the class contains only static members.
- Using a private constructor is not possible to create an instance from outside the class.
The main purpose of creating private constructor is to restrict the class from being instantiated when it contains every member as static.
public class Employee
{
public int ID;
public string name;
public string designation;
public int roll_no;
private Employee()
{
Console.WriteLine("Private Constructor");
}
public Employee(int id, string names, string desig)
{
ID = id;
name = names;
designation = desig;
}
}
public class Program
{
public static void Main()
{
//Employee emp1 = new Employee(); // it will generate an error because the constructor is inaccessible
Employee emp2 = new Employee(123, "John Smith", "Software Engineer");
Console.WriteLine("Employee value is: " + emp2.ID + "\n" + emp2.name + "\n" + emp2.designation);
}
}
Output
Employee value is: 123
John Smith
Software Engineer
Static Constructor
Static constructor will be invoked only once for all the instances of the class and it is invoked during the creation of the first instance of the class.
Following are its characteristics:
- Static constructor neither accepts parameters nor access modifiers.
- In a class, only one static constructor is allowed.
- Static constructor will invoke automatically, whenever we create the first instance of a class.
- It is used to initialize static fields of the class.
- A static constructor cannot be called directly.
- The user has no control over when the static constructor is executed in the program.
- A typical use of static constructors is when the class is using a log file and the constructor is used to write entries to this file.
namespace Constructors
{
public class Employee
{
public int ID;
public string name;
public string designation;
public int roll_no;
static Employee()
{
Console.WriteLine("Static Constructor");
}
public Employee()
{
Console.WriteLine("Default Constructor");
}
}
public class Program
{
public static void Main()
{
Employee emp1 = new Employee();
Employee emp2 = new Employee();
}
}
}
Output
Static Constructor
Default Constructor
Default Constructor
The above code shows that we initialize the class twice but only call the static constructor once during its lifetime.
Interview Question and Answers about Constructor in C#
What is a constructor in C#?
A special method of the class that is automatically invoked when an instance of the class is created is called a constructor.
The main use of constructors is to initialize the private fields of the class while creating an instance for the class. When you have not created a constructor in the class, the compiler will automatically create a default constructor of the class. The default constructor initializes all numeric fields in the class to zero and all string and object fields to null.
How many Constructors can be Defined in a Class in C#?
In C#, within a class, we can define any number of constructors. But the most important point that you need to remember is that each and every constructor must have a different signature. A different signature means the number, type, and parameter order should be different. So, in a class, we can define one no-argument constructor plus ‘n’ number of parameterized constructors in C#.
When do we need to provide the Constructor Explicitly?
If we want to execute some custom logic at the time of object creation, that logic may be object initialization logic or some other useful logic, then as a developer, we must provide the constructor explicitly in C#.
What is a User-Defined Default Constructor in C#?
The constructor which is defined by the user without any parameter is called the user-defined default constructor. This constructor does not accept any argument but as part of the constructor body, you can write your own logic.
When should we define a parameterized constructor in a class?
If we want to initialize the object dynamically with the user-given values or if we want to initialize each instance of a class with a different set of values then we need to use the Parameterized Constructor in C#. The advantage is that we can initialize each instance with different values.
Can we initialize non-static data members within a static constructor in C#?
It is not possible to initialize non-static data members within a static constructor, it raises a compilation error.
Can you create object of class with private constructor in C#?
No, object of a class having private constructor cannot be instantiated from outside of the class.
What is the advantages of static class in C#?
The advantage of using a static class is that the compiler can check to make sure that no instance members are accidentally added. The compiler will guarantee that instances of this class cannot be created. Static classes are sealed and therefore cannot be inherited. They cannot inherit from any class except Object
Difference between private constructor and static constructor in C#
Static constructor is called before the first instance of class is created, wheras private constructor is called after the first instance of class is created.
Static constructor will be executed only once, whereas private constructor is executed every time, whenever it is called.
Conclusion
In this article, we discussed various types of constructors in C# language with examples and also discussed possible interview questions and answers.
Leave behind your valuable queries and suggestions in the comment section below. Also, if you think this article helps you, do not forget to share this with your developer community. Happy Coding 🙂
You may Also Like
- Building Real-Time Messaging with Kafka and .NET Core
- How to Build a serverless CRUD app with Azure function and Cosmos DB
- .NET 8 Authentication with Identity in a Web API using Bearer Tokens and Cookies
- How to convert Text To Speech With Azure Cognitive Services using Angular and .Net Core
- CRUD operation using the repository pattern with .Net 8, Ef-Core, and MySQL
- How to use Response Compression in .NET Core
- How to Integrate GraphQL in .Net Core
- Upload Download and Delete files in Azure Blob Storage using ASP.NET Core and Angular
- How to upload files to Azure Blob Storage using Asp.Net Core Web API
- Integrate Paging in ASP.Net Core Web API- Beginner’s Guide
Jayant Tripathy
Coder, Blogger, YouTuberA passionate developer keep focus on learning and working on new technology.