In this article, I will explain you how to Translate Language Using Google Translate API in ASP.Net MVC. You can see in the below picture the Google have a website named “https://translate.google.co.in/” that provided us to convert one language to another. The Google provide us to convert 100 languages for translation. Please read my all articles of .NET in this link.
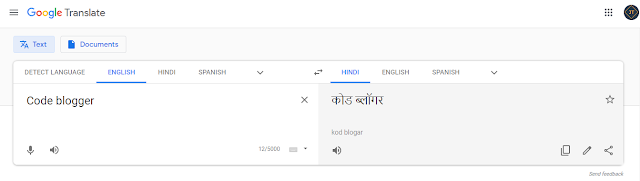
But question is how to create your own translator using Google API? I will explain all details step by step. For this we need the Google API and mind that as we are using Google API CDN in this example so internet connectivity is mandatory for this.
I will show you how the Google translator works and how it save into database using MVC with EF (Code-First Approach)
Prerequisite
• Google API
• MVC 4.0
• SQL Server
• HTML/Bootstrap
Creating ASP.Net MVC Project
Open Visual Studio 2017 -> Create New Project -> Set as Visual C# and Asp .Net Web Application (.Net Framework) ->Set the Project name as GoogleTranslateMVC -> Click Ok
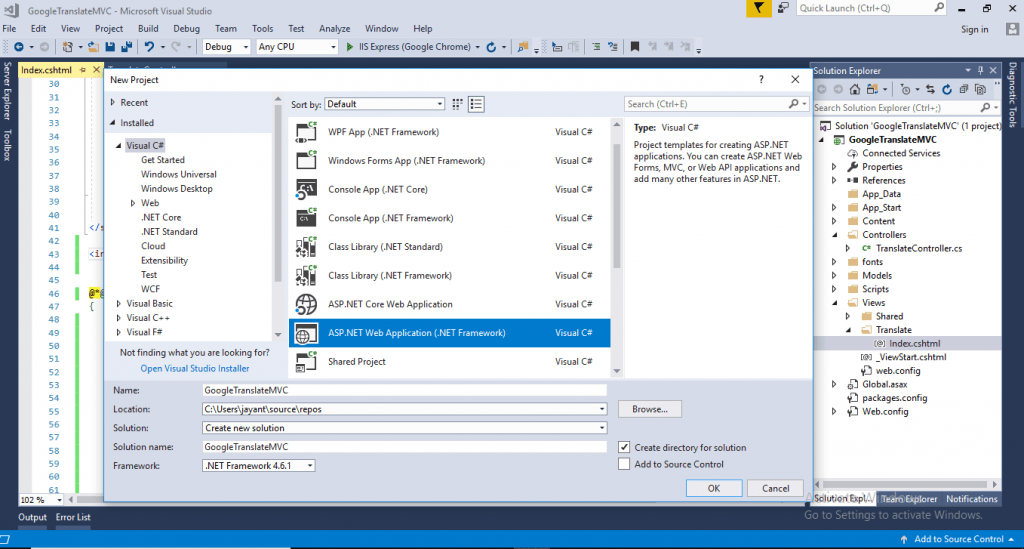
Then choose Empty as application and check the Checkbox as MVC as per the below image. This will take span of time to setup the application.
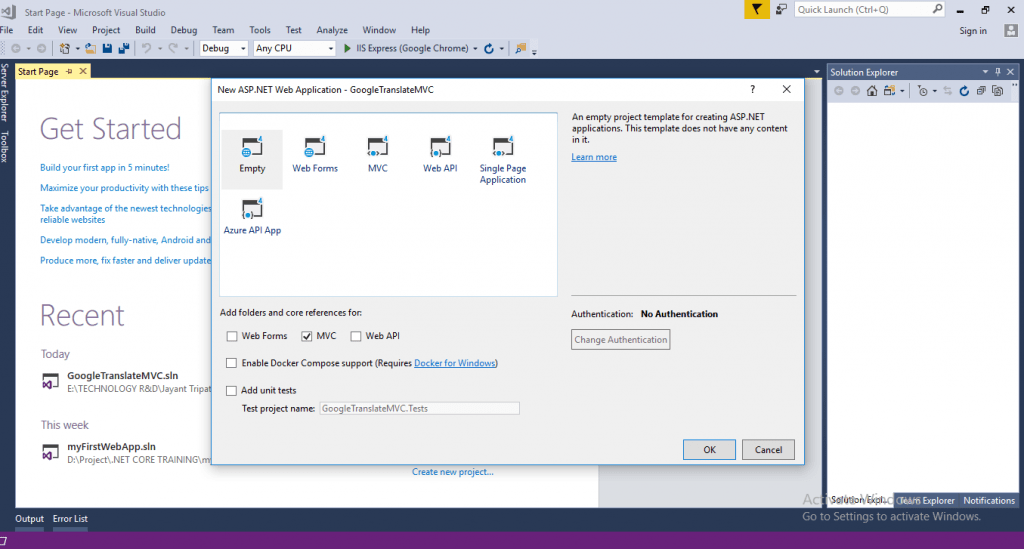
Now the application is ready and we add a Controller and create a view of that. Add a Controller named as TranslateController and in index action add View.
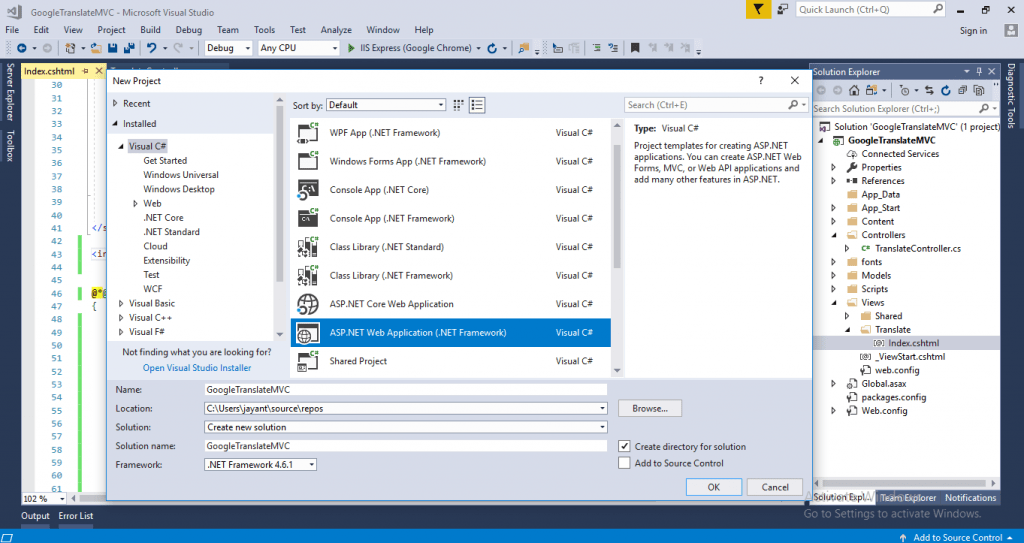
Adding the Google API CDN
<script type="text/javascript" src="https://www.google.com/jsapi"></script>
<script type="text/javascript">
// Load the google elements
google.load("elements", "1", {
packages: "transliteration"
});
function onLoad() {
var options = {
sourceLanguage:
google.elements.transliteration.LanguageCode.ENGLISH,
destinationLanguage:
[google.elements.transliteration.LanguageCode.HINDI],
transliterationEnabled: true
}
// Create a Instance on TransliterationControl
var control = new google.elements.transliteration.TransliterationControl(options);
control.makeTransliteratable(['HindiLanguage']);
}
google.setOnLoadCallback(onLoad);
</script>
Then add the Textbox like below to see the Translator and run the application.
<input type="text" id="HindiLanguage" name="HindiLanguage" autocomplete="off" />
Run the application and the Output should like below, if we write anything in English it should automate translate to Hindi as we use destination language as Hindi:
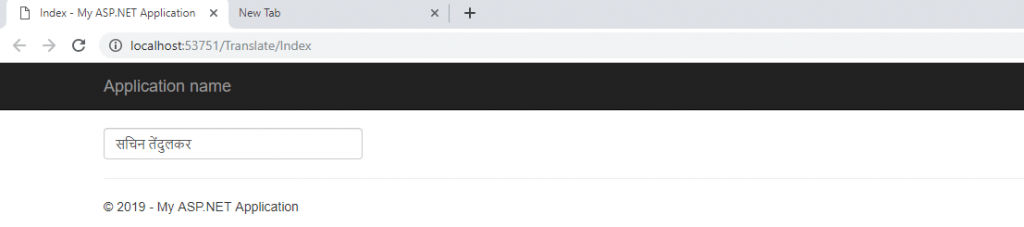
Creating Database Table
Now we save the data into database using MVC and EF.
1. Create a database and Table, the table script look like below. Here my database is “TestDB” and table name is “Translate” and I have added two columns, one is for Hindi and one is for Oriya
USE [TestDB]
GO
/****** Object: Table [dbo].[Translate] Script Date: 4/16/2019 1:48:59 PM ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE TABLE [dbo].[Translate](
[Id] [int] IDENTITY(1,1) NOT NULL,
[HindiLanguage] [nvarchar](500) NULL,
[OriyLanguage] [nvarchar](500) NULL,
CONSTRAINT [PK_Translate] PRIMARY KEY CLUSTERED
(
[Id] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
GO
Adding the Entity Framework Code First Approach
Create a Model class
After adding EF to the project then add a class of property to hold the languages. Below the class name is “Translate” also my table name is same, I map the table name.
namespace GoogleTranslateMVC.Models
{
[Table("Translate")]
public class Translate
{
[Key]
public int Id { get; set; }
public string HindiLanguage { get; set; }
public string OriyLanguage { get; set; }
}
}
Adding a Data Context class
We did EF Code-First approach here so declare the Data Context Class like below to establish with db.
using System;
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
using System.Web;
namespace GoogleTranslateMVC.Models
{
public class DataContext : DbContext
{
public DataContext() : base("name=mySqlConnection")
{
}
public DbSet<Translate> Translate { get; set; }
}
}
Adding the connection string
Add the Connection String in Web.config like below:
<connectionStrings>
<add name="mySqlConnection" connectionString="Data Source=SERVERNAME;
Initial Catalog=TestDB; Persist Security Info=True;User ID=sa;password=*****;
Pooling=false;Connect Timeout=300000" providerName="System.Data.SqlClient"></add>
</connectionStrings>
Adding Datacontext class inside Controller
Now add the DataContext Class reference into the “Translate” controller and the Index() look like this:
Add the Post method in “Transalte” Controller
public ActionResult Index()
{
using (var ctx = new DataContext())
{
var Lang = ctx.Translate.ToList();
return View(Lang);
}
}
[HttpPost]
public ActionResult Index(Translate tran)
{
using (var ctx = new DataContext())
{
ctx.Translate.Add(tran);
ctx.SaveChanges();
var languageList = ctx.Translate.ToList();
return View(languageList);
}
}
Adding the HTML content inside CSHTML
Now modify the Index.cshtml like below
1. Add the class
2. Add bootstrap for styling
3. Add MVC Beginform to submit
@model IEnumerable<GoogleTranslateMVC.Models.Translate>
@{
ViewBag.Title = "Index";
}
<link href="~/Content/bootstrap.min.css" rel="stylesheet" />
<script type="text/javascript" src="https://www.google.com/jsapi"></script>
<script type="text/javascript">
// Load the google elements
google.load("elements", "1", {
packages: "transliteration"
});
function onLoad() {
var options = {
sourceLanguage:
google.elements.transliteration.LanguageCode.ENGLISH,
destinationLanguage:
[google.elements.transliteration.LanguageCode.HINDI],
transliterationEnabled: true
}
var options2 = {
sourceLanguage:
google.elements.transliteration.LanguageCode.ENGLISH,
destinationLanguage:
[google.elements.transliteration.LanguageCode.TAMIL],
transliterationEnabled: true
}
// Create a Instance on TransliterationControl
var control = new google.elements.transliteration.TransliterationControl(options);
var control2 = new google.elements.transliteration.TransliterationControl(options2);
control.makeTransliteratable(['HindiLanguage']);
control2.makeTransliteratable(['OriyLanguage']);
}
google.setOnLoadCallback(onLoad);
</script>
@using(Html.BeginForm("index","Translate",FormMethod.Post))
{
<div class="container" style="padding:10px;">
<div class="panel panel-default">
<div class="panel-heading">
<h3 class="panel-title">Google translate</h3>
</div>
<div class="panel-body">
<div class="row">
<div class="col-md-3">
<input type="text" id="HindiLanguage" name="HindiLanguage" class="form-control" autocomplete="off" />
</div>
<div class="col-md-3">
<input type="text" id="OriyLanguage" name="OriyLanguage" class="form-control" autocomplete="off" />
</div>
<div class="col-md-6">
<input type="submit" id="btn_Submit" name="btn_Submit" class="btn btn-primary" value="Submit" />
</div>
</div>
</div>
</div>
</div>
}
@if (Model != null && Model.Count() != 0)
{
<div class="row">
<div class="col-md-12">
<table class="table table-condensed">
<thead>
<tr>
<th>Sl#.</th>
<th>Language1</th>
<th>Language2</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<th>@item.Id</th>
<th>@item.HindiLanguage</th>
<th>@item.OriyLanguage</th>
</tr>
}
</tbody>
</table>
</div>
</div>
}
All is done now, run the application and see the output like below,
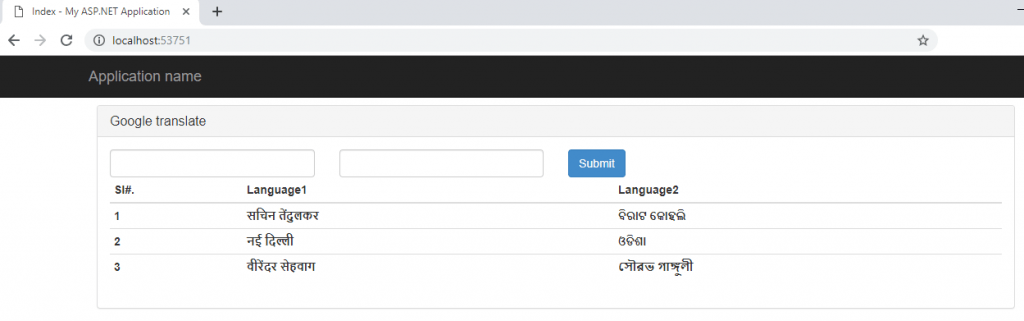
And the information are saved in database, the database output is like below,
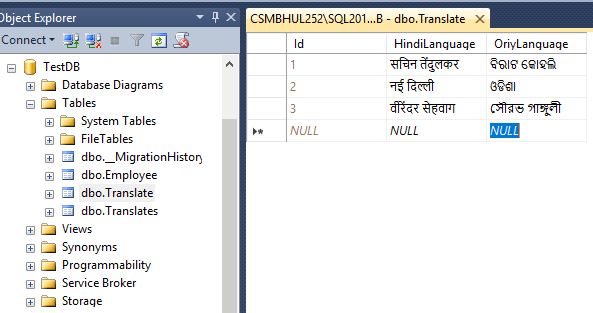
Conclusion
So in this article we learnt about Translate Language Using Google Translate API in ASP.Net MVC.
Hi, this is very nicely explained.
If we want to use the same method to translate web pages, in fact web applications, can we do?
Do you have any article on that?
Hi Deeraj, If I am not wrong you are asking using same method, if we can translate complete web page right ?
If Yes, then definitely let me try once.